A C++ code generator is a tool that automatically creates C++ code snippets based on user-defined parameters, streamlining the coding process and reducing manual errors.
Here’s an example of a simple C++ code generator that outputs a "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is a C++ Code Generator?
A C++ code generator is a specialized tool that automatically produces C++ source code based on a set of defined specifications or templates. Its primary purpose is to streamline the coding process by reducing the amount of repetitive boilerplate code developers must manually write. The benefits of using a code generator include time efficiency, allowing developers to focus on higher-level logic rather than mundane syntax, and consistency in code structure, which promotes good coding practices across large projects.
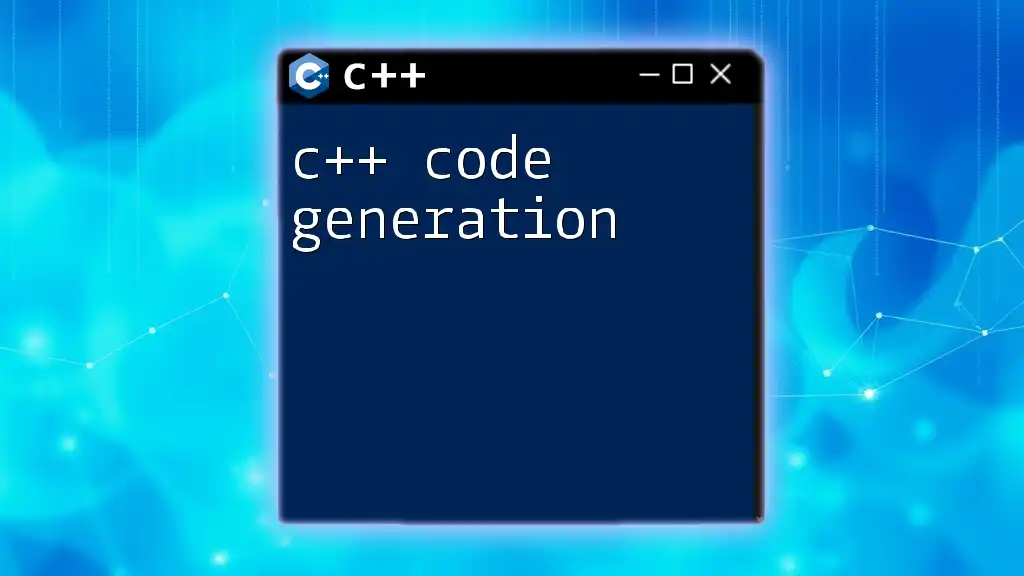
Why Use a C++ Code Generator?
One compelling reason to utilize a C++ code generator is the significant time savings it provides. By automating the generation of common code patterns, developers can reduce the development cycle and deploy applications much faster. Additionally, code generators help enhance consistency across different parts of a project or among different projects, reducing potential discrepancies.
Another crucial factor is the reduction of human error. Since code generation tools follow defined templates and specifications, they mitigate the risk of mistakes that can arise from manual coding, such as syntax errors or typographical mistakes.
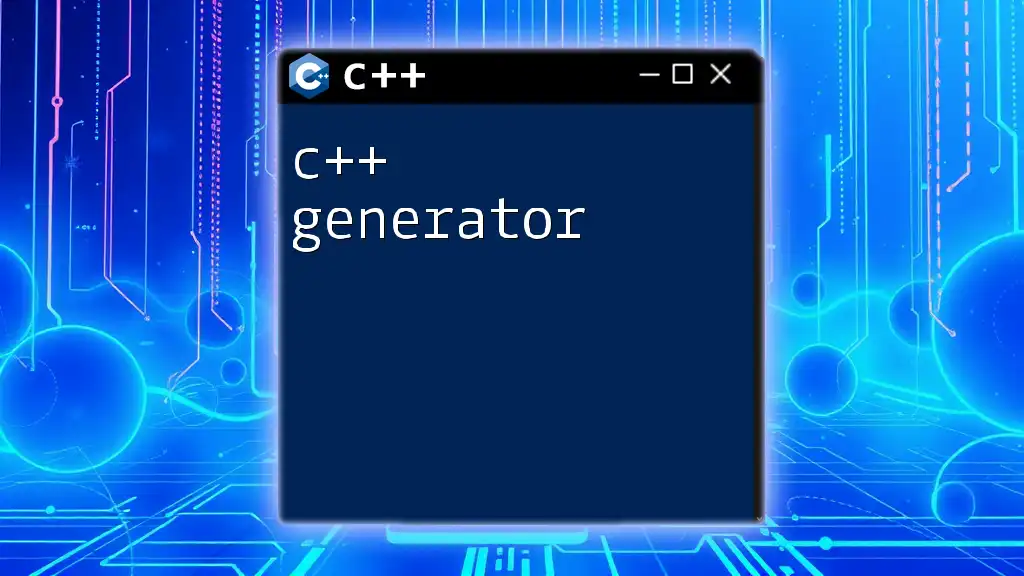
Types of C++ Code Generators
High-Level Code Generators
High-level code generators focus on generating C++ code from abstract models and design specifications. These tools allow developers to define a structure or behavior visually, which the generator then translates into actual code.
Overview and Features
High-level code generators typically feature the ability to produce full class definitions, create interfaces, and implement data structures. These tools often work best when integrated into development environments or modeling software.
Popular High-Level C++ Code Generators
- Qt Designer: This interactive tool allows developers to create user interfaces visually, generating corresponding C++ code.
- Enterprise Architect: A comprehensive UML modeling tool that can produce C++ code from UML diagrams.
Use Cases
High-level code generators are particularly useful in rapid application development, where speed and efficiency are paramount. They are ideal for developers working on prototypes or applications that require frequent updates.
Low-Level Code Generators
Low-level code generators focus on generating specific code snippets or libraries within the C++ ecosystem. These tools often work by providing templates that developers can fill in with custom logic or data.
Overview and Features
Low-level code generators allow fine-tuning and detailed control over the generated code while still saving time on repetitive tasks. They often offer a range of templates tailored to specific designs and implementations.
Popular Low-Level C++ Code Generators
- CodeSmith: A versatile code generation tool that supports various languages, including C++.
- C++ Builder: This development environment includes a powerful code generation engine, which assists in creating C++ applications.
Use Cases
Low-level code generators are excellent for embedded systems and performance-critical applications, where precise and optimized code is necessary.
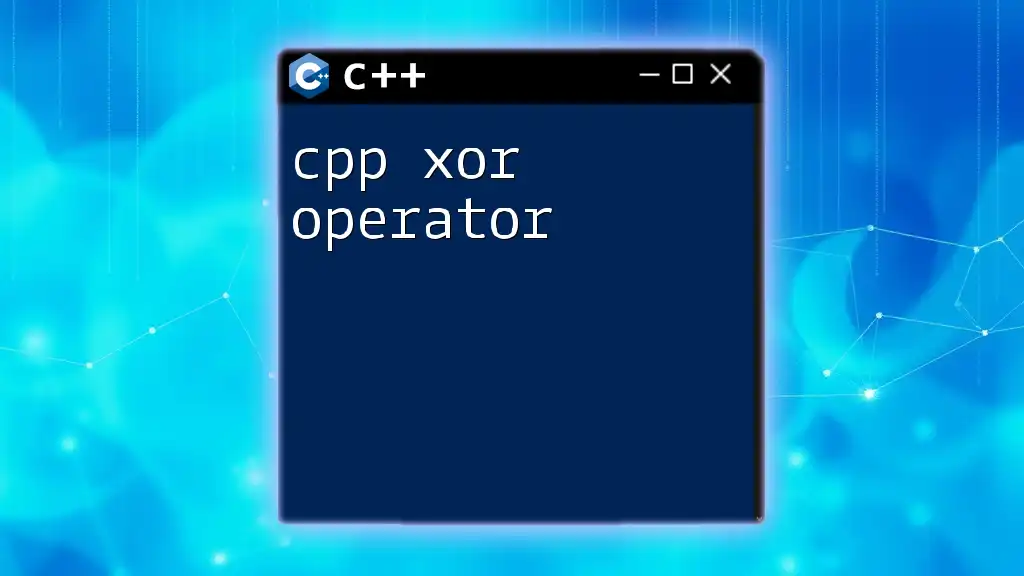
How C++ Code Generators Work
Input Specifications
Understanding the different input formats is crucial in utilizing a C++ code generator effectively. Input specifications often include model definitions, configuration files, or code templates that clearly define the desired output.
Example of Input Specification
Here’s a simple input specification in JSON format that defines a class structure:
{
"className": "User",
"attributes": {
"id": "int",
"name": "std::string",
"email": "std::string"
}
}
Code Generation Process
The code generation process typically follows several steps:
- Parsing the Input: The generator begins by parsing the input specifications to understand the desired code structure.
- Transformation: It then transforms this information into actual code components, taking care to maintain proper syntax and structure.
- Output Code Generation: Finally, the generator produces the output code, effectively creating a fully functional C++ class based on the provided specifications.
Example Code Generation Process
Consider the provided JSON input for a `User` class. A high-level overview shows how this input is transformed into a C++ class definition, maintaining type and structure integrity.
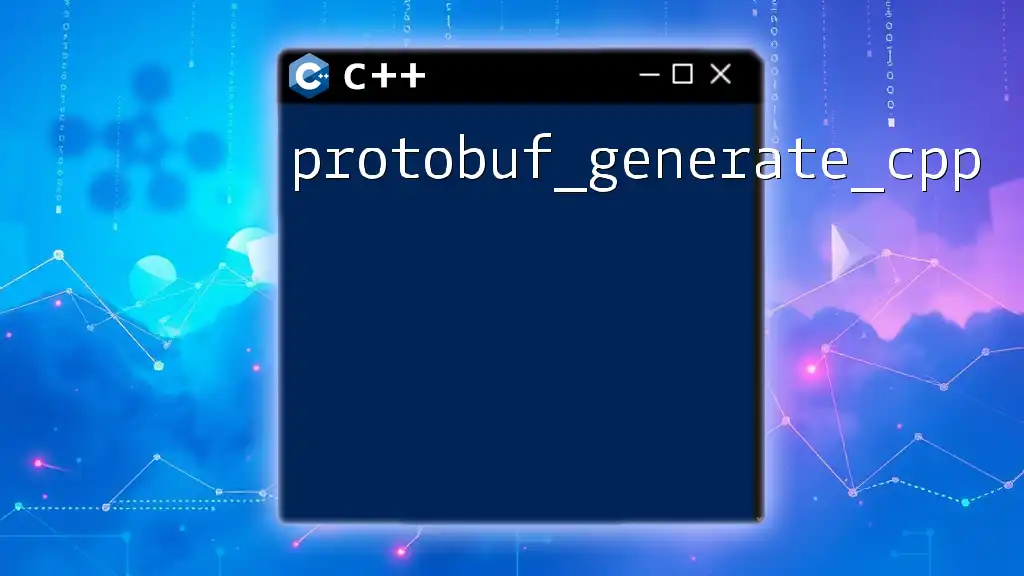
Using C++ Code Generators Effectively
Best Practices
To maximize the benefits of a C++ code generator, developers should keep these best practices in mind:
- Maintain Readability in Generated Code: Although the code is auto-generated, it is essential for it to be readable and maintainable.
- Customize Templates for Specific Use Cases: Tailoring templates to fit project needs can significantly enhance productivity and reduce the need for post-generation modifications.
- Regularly Update Input Specifications: Keeping input specifications current ensures the generated code remains relevant and suitable for evolving project requirements.
Common Pitfalls to Avoid
While code generators are powerful, developers should be cautious of over-relying on automated outputs. It's vital to balance automation with manual coding to maintain flexibility and adaptability within projects. Additionally, ignoring the quality of the generated code could lead to issues down the line. It’s imperative to review and refactor generated code as necessary to adhere to coding standards.
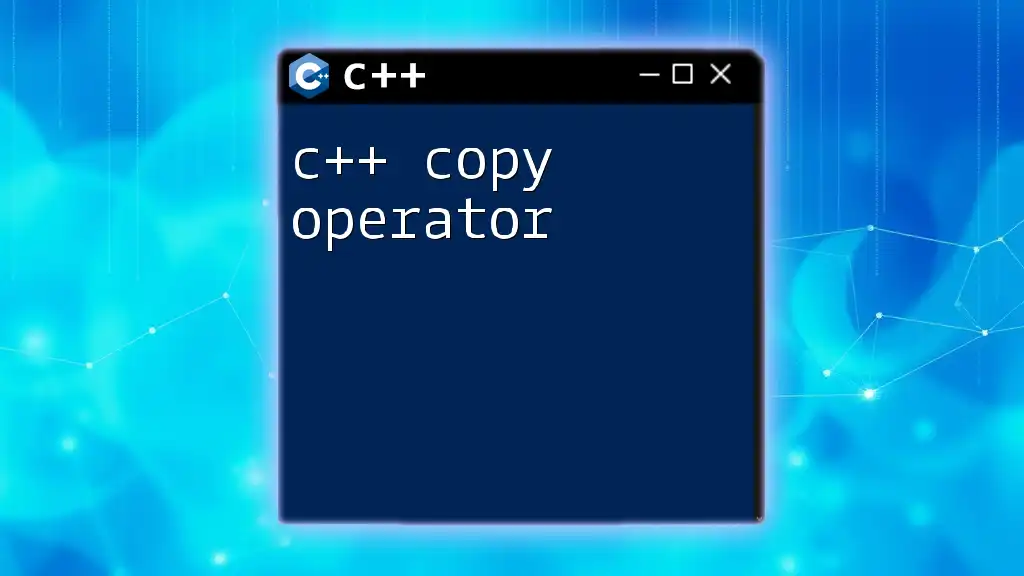
Popular C++ Code Generators Options
Overview of Leading Tools
Among the various tools available, two stand out for their capabilities:
- Visual Studio Code Generator: Functions seamlessly within Visual Studio, enabling developers to create templates that populate code with ease.
- CMake: Primarily a build system generator, it also offers features to create project-specific C++ code based on user-defined configurations.
Comparison of Tools
To provide clarity, here are some pros and cons of these popular C++ code generators:
-
Visual Studio Code Generator:
- Pros: Integrated development, comprehensive support, high customizability.
- Cons: Steeper learning curve for beginners.
-
CMake:
- Pros: Excellent for project management, widely used in open-source projects.
- Cons: Primarily a build tool, requiring additional steps for code generation.
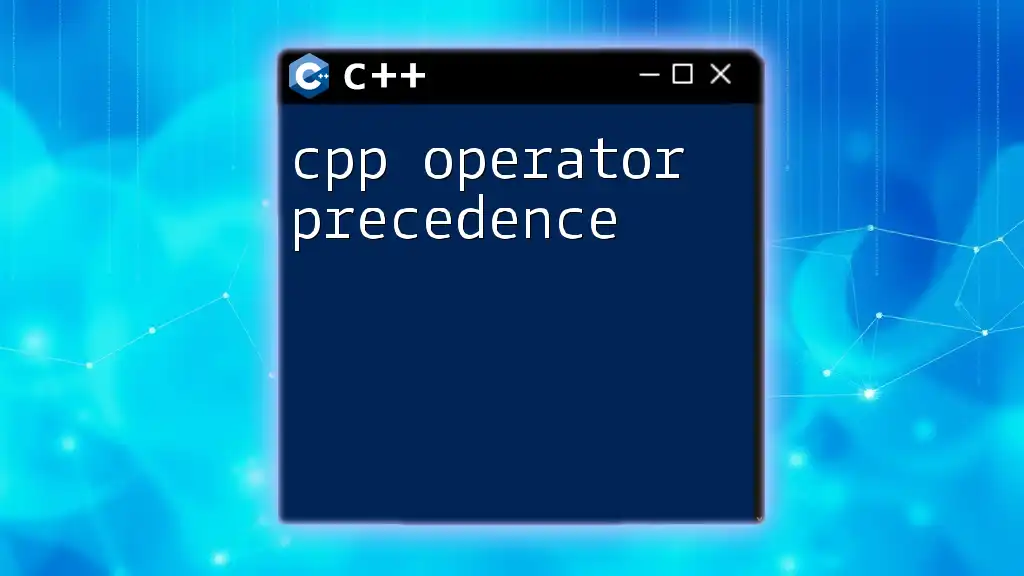
Building Your Own C++ Code Generator
Why Build Your Own?
Creating a custom C++ code generator allows developers to address unique needs in their projects. Tailoring a generator ensures that the output aligns perfectly with specific coding styles and practices, enhancing both productivity and code quality.
Key Steps in Development
The initial steps for building your C++ code generator include choosing a programming language for implementation, such as Python or C#, and defining the overall structure of your input specifications for optimal parsing.
Example of a Simple C++ Code Generator
Below is an example of a basic Python function for generating a C++ class definition based on given arguments:
def generate_class(class_name, attributes):
class_definition = f"class {class_name} {{\n"
for attr, attr_type in attributes.items():
class_definition += f" {attr_type} {attr};\n"
class_definition += "};"
return class_definition
# Example Usage
print(generate_class('User', {'id': 'int', 'name': 'std::string'}))
The function `generate_class` takes a class name and its attributes, creating a structured C++ class definition.
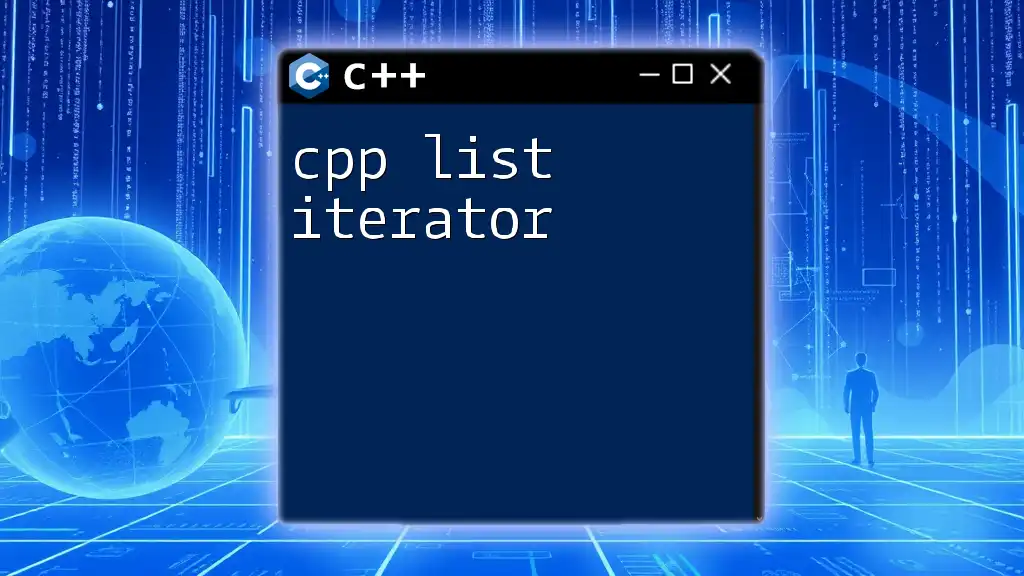
Conclusion
In summary, C++ code generators serve an essential role in modern software development. They enhance productivity, maintainability, and consistency in codebases. By leveraging the power of code generation tools and practices, developers can streamline their workflows and focus on creating innovative solutions.
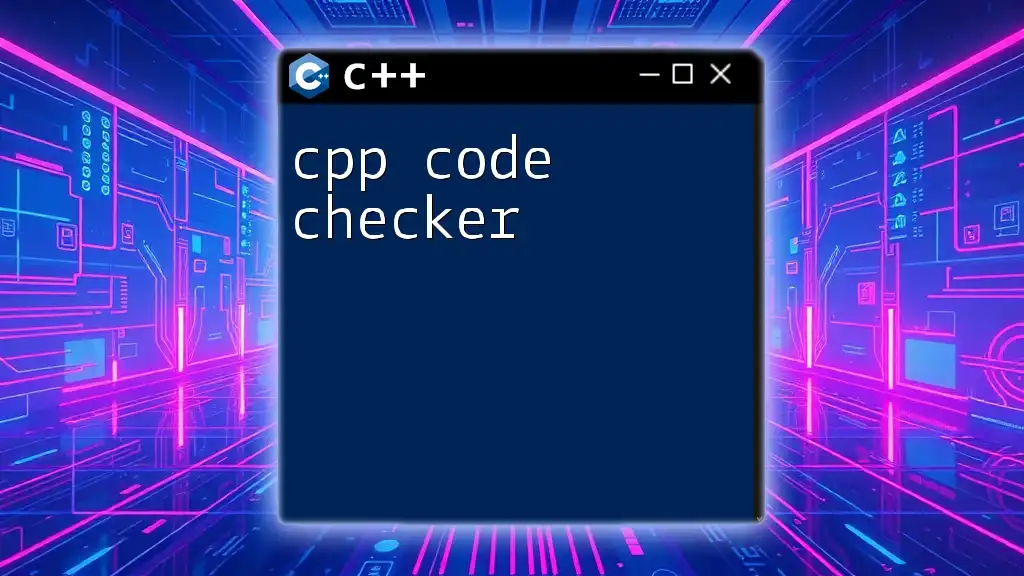
Additional Resources
For further reading on C++ code generation, consider exploring recommended books, online courses, and documentation tailored to C++. Engaging with community forums and groups will also help you stay updated with the latest tips and tools in the fast-evolving world of C++ programming.
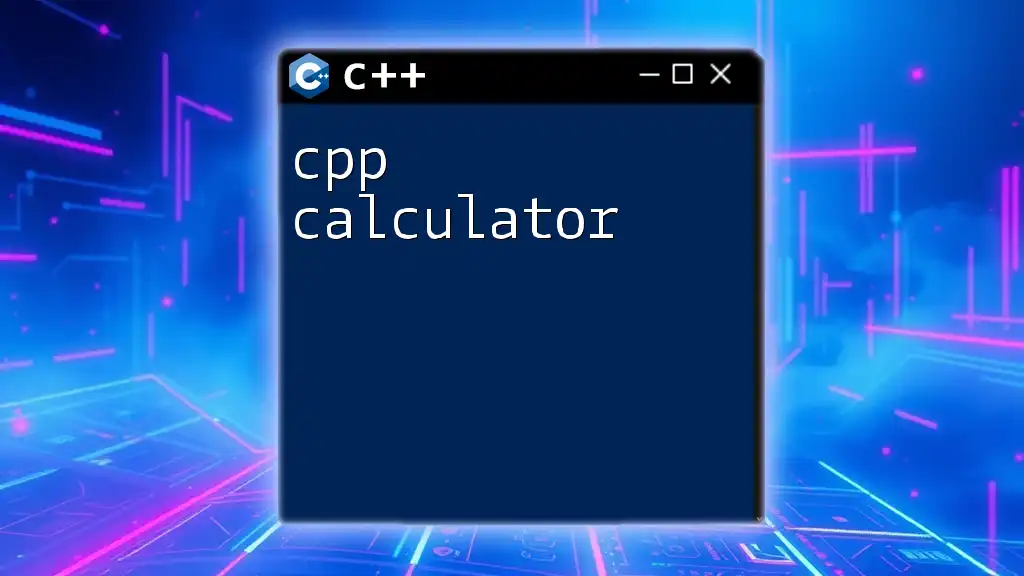
Call to Action
Join our C++ Code Generation Workshop to deepen your understanding of coding techniques and explore practical applications of C++ code generators. Explore hands-on activities and enhance your skills in this vital area of development!