C++ contributions refer to the collaborative efforts of developers to enhance the C++ programming language and its ecosystem through code contributions, documentation improvements, and community engagement.
Here's a basic code snippet demonstrating a C++ function that can serve as a simple contribution example:
#include <iostream>
// Function to add two integers
int add(int a, int b) {
return a + b;
}
int main() {
std::cout << "The sum of 3 and 5 is: " << add(3, 5) << std::endl;
return 0;
}
What Are C++ Contributions?
Contributions in the context of C++ refer to the various ways developers can enhance, optimize, and expand upon existing C++ projects, languages, or community resources. These contributions can manifest in several ways, including code contributions, documentation contributions, and community engagement.
Types of Contributions
Code Contributions involve writing new libraries, frameworks, or tools to support the C++ ecosystem. This can also mean implementing new features directly within the C++ language or existing codebases.
Documentation Contributions play a pivotal role in making C++ more accessible. Improving tutorials, guides, and in-line comments within the code helps demystify C++ for newcomers while aiding existing users in better understanding complex features.
Community Engagement is about being active in discussions on online forums, participating in meetups, and contributing insights that can foster a collaborative environment. This engagement can lead to a richer community where ideas and solutions are shared freely.
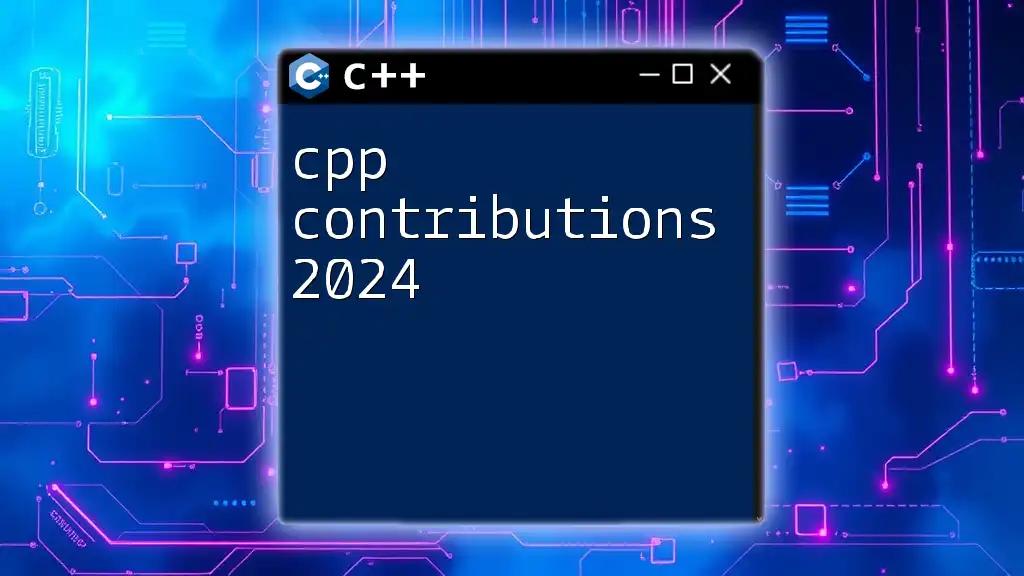
Why Contribute to C++?
Contributing to C++ comes with numerous benefits that can significantly impact both personal and professional development.
Skill Development
One of the foremost reasons to contribute is the skill development aspect. Hands-on coding allows you to learn from real-world scenarios while engaging in peer reviews exposes you to different coding philosophies and techniques.
Building a Network
Contributing enables you to build a network of contacts within the industry. Whether you're working on a collaborative project or merely participating in forums, these interactions can lead to collaborations, mentorships, and even job opportunities.
Advancement of the Language
Being involved in contributions allows you to directly influence the evolution of C++. You can take part in discussions that shape future standards, propose improvements, and be an active player in refining the language.
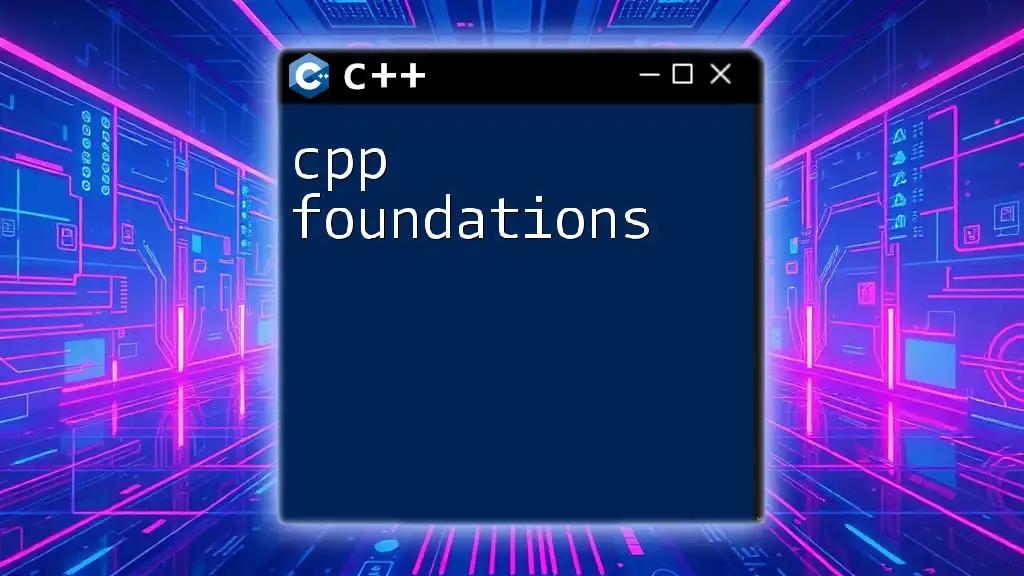
Major Platforms for C++ Contributions
Finding the right platform to contribute to is essential for maximizing your impact within the C++ community.
GitHub
GitHub serves as the primary repository for open-source C++ projects. Developers can explore numerous projects and get involved in contributing code, debugging, or documentation.
To illustrate, if you want to contribute to a project like the C++17 standard library, you could navigate to its GitHub repository, identify open issues, and start addressing them. This process often begins with forking the project, making local changes, and then submitting a pull request for review.
C++ Standards Committee
The C++ Standards Committee is crucial for maintaining and evolving the C++ standards. Getting involved with this committee often requires a bit more commitment, but for those passionate about the language, it can be incredibly rewarding. Potential contributors can review proposals, comment, or even submit their own ideas regarding improvements.
Stack Overflow and Online Forums
Online forums and platforms like Stack Overflow offer another avenue for contributing through knowledge sharing. By answering questions, providing code examples, or reviewing solutions, users not only aid others but also solidify their understanding of C++ concepts.
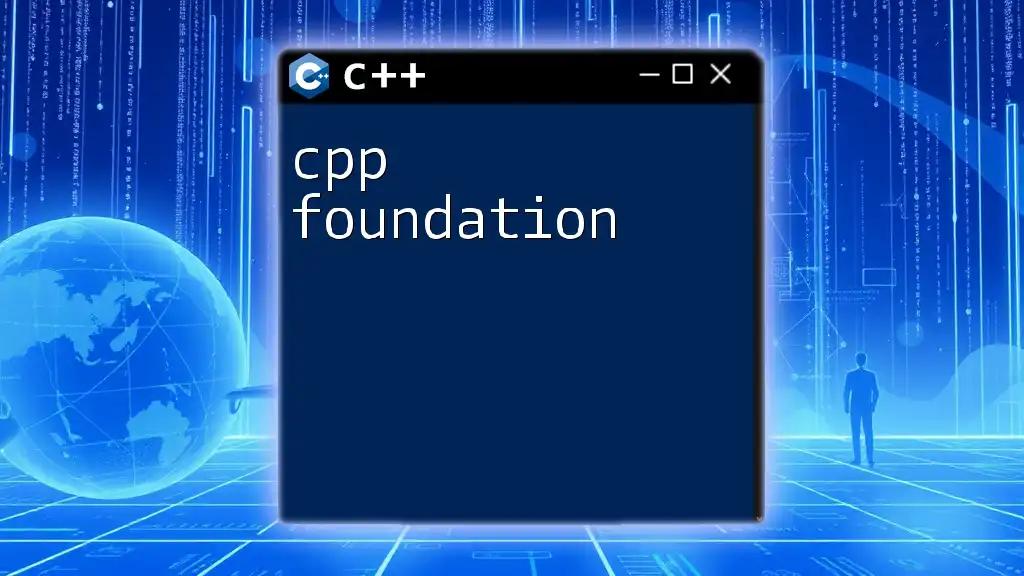
How to Get Started with Contributing to C++
Starting your journey in C++ contributions can seem daunting, but breaking it down into smaller, manageable steps can simplify the process.
Understanding Existing Codebases
When diving into an existing project, familiarize yourself with the repository. Opening a project in an Integrated Development Environment (IDE) helps you navigate the structure of the codebase and understand the design principles it follows.
Finding Issues to Work On
Utilize GitHub’s issue tracker to find projects suitable for your skill level. Often, repositories label beginner-friendly issues, making it easier for newcomers to identify where they can contribute.
Making Your First Contribution
Making your first contribution can be incredibly fulfilling. Here’s how to do it:
- Fork the Project: This creates your own copy where you can freely make changes.
- Clone the Repository Locally: You can work on the project in your local development environment.
- Make Changes: After identifying an issue, write your code. For example, if you're fixing a bug in a function, it might look like this:
int add(int a, int b) {
return a + b; // Basic addition function
}
After you have made your changes, test thoroughly to ensure everything works as expected.
- Commit Your Changes: Use clear and concise commit messages that describe what you’ve accomplished.
- Submit a Pull Request: Once you are satisfied with your changes, you can submit a pull request to the original repository for review.
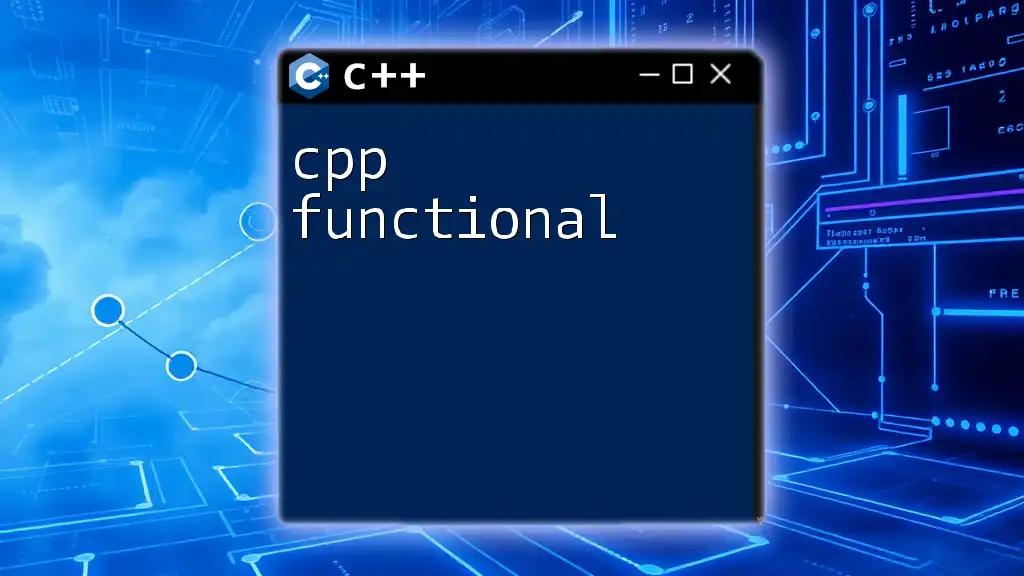
Best Practices for Contributing
Writing Quality Code
Ensuring that your code is clean and readable is critical. Adopting naming conventions, consistent formatting, and modularity will not only help others read your code but will also speed up the requirement.
Here’s a before-and-after example of basic code refactoring:
Before:
void f(int x){
if(x>0){
cout << "Positive";
}
}
After:
void printPositiveMessage(int number) {
if (number > 0) {
std::cout << "Positive" << std::endl;
}
}
Documentation and Comments
Don’t underestimate the importance of thorough documentation. Comprehensive comments and documentation enhance understanding and help future contributors navigate your work. Standards like Doxygen can guide you in writing professional documentation.
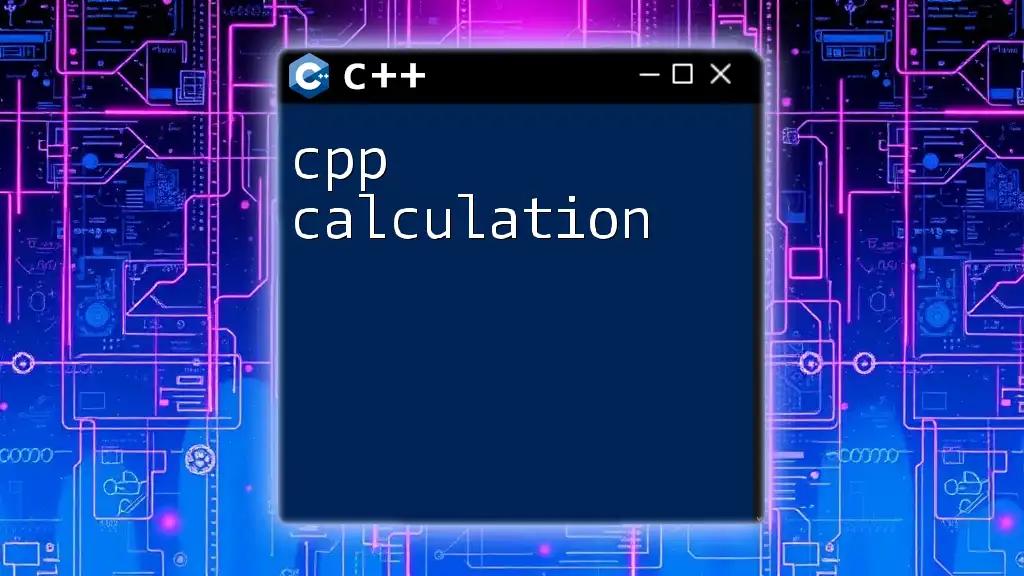
Real-life Examples of C++ Contributions
Case Study 1: Boost Libraries
The Boost Libraries are a prime example of successful C++ contributions. Originating from hundreds of contributors, the libraries' modular nature allows for extensive usability while also exemplifying high-quality development practices. Each library within Boost typically has its own set of maintainers who manage contributions, making it an excellent model for new contributors.
Case Study 2: OpenCV
OpenCV, an open-source computer vision library, has evolved dramatically due to contributions from users worldwide. By engaging with their community, contributors have implemented enhancements and new features, demonstrating how individual contributions can lead to significant advancements in software.
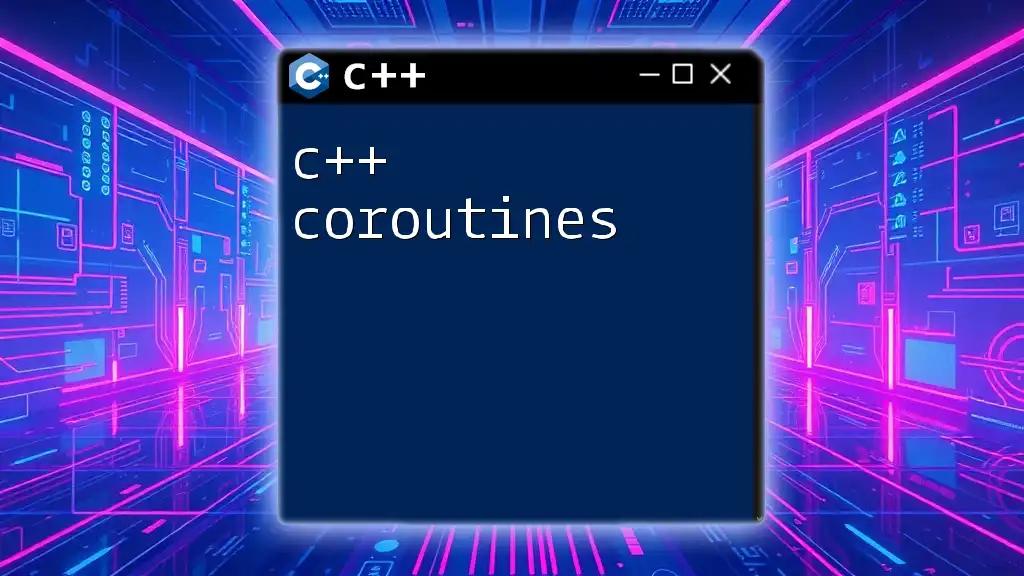
Challenges of Contributing to C++
While contributing can be rewarding, it’s important to acknowledge the challenges you may face.
Understanding Legacy Code
Many C++ projects involve legacy code, which can be daunting and complex. Familiarizing yourself with common design patterns and principles can help you navigate through older codebases effectively.
Keeping Up with Changes
C++ is a dynamic language with regular updates. Staying informed about new features, libraries, and standards is crucial for effective contributions. Resources like the C++ Super-FAQ and CPPCon sessions provide valuable insights for continuous learning.
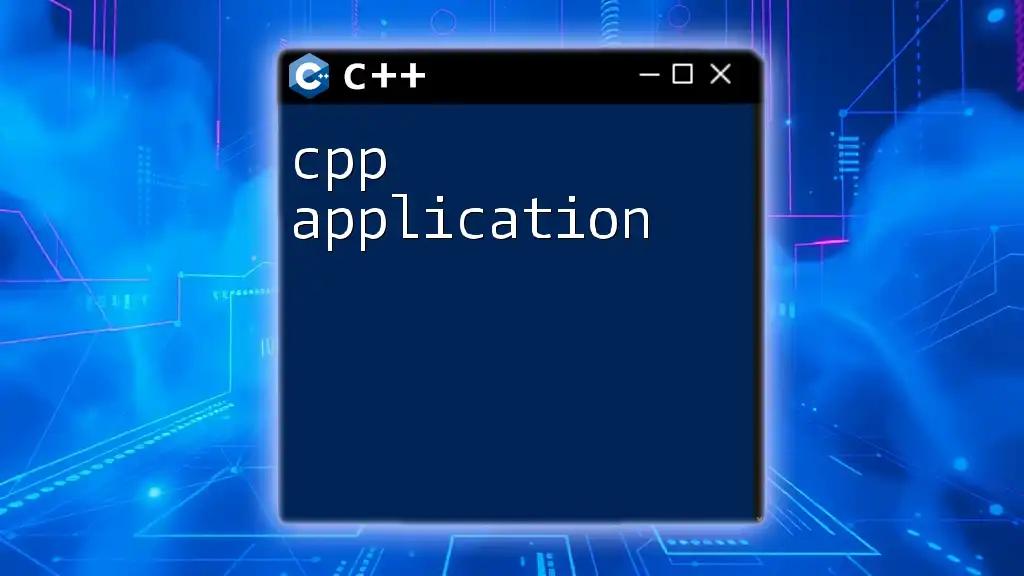
Conclusion
Contributing to C++ is not merely about writing code; it's about engaging with a vibrant community, learning new skills, and influencing the future of a powerful programming language. The doors are open for anyone willing to participate, so dive in and start making your mark in the world of C++ contributions.
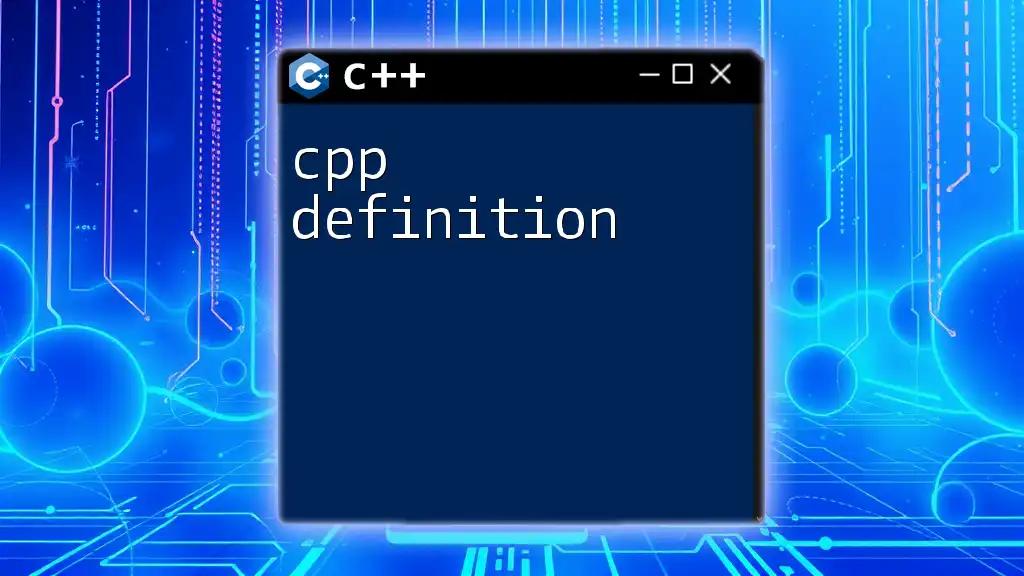
Additional Resources
For those eager to delve deeper, a list of essential resources includes:
- C++ Community on GitHub
- C++ Super-FAQ
- Books like "The C++ Programming Language" by Bjarne Stroustrup
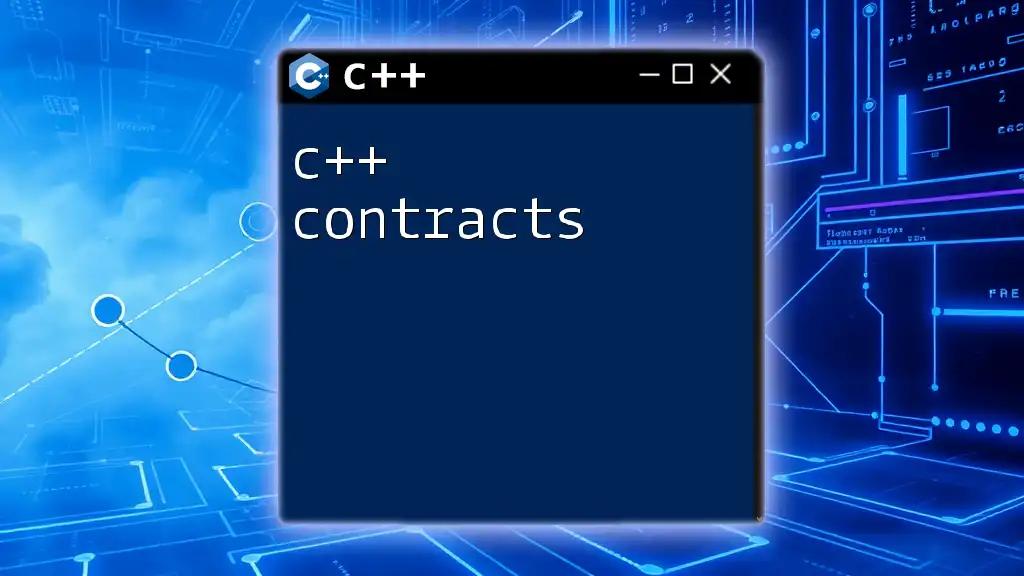
FAQ Section
Addressing common questions about C++ contributions can help demystify the process for beginners.
-
What should I focus on when making my first contribution? Start with smaller issues in well-maintained repositories. Build confidence before tackling larger projects.
-
How can I overcome barriers to contributing? Engage with community members, participate in discussions, and attend local meetups to gain support and knowledge.
By addressing these points, new contributors can navigate their entry into the world of C++ contributions with greater ease, hoping to inspire a future generation of C++ developers.