In 2024, C++ contributions focus on enhancing language features and libraries, aimed at improving performance, safety, and simplicity for developers. Here's a basic example of a modern C++ feature, the range-based for loop, which simplifies iteration over containers:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (const auto& num : numbers) {
std::cout << num << " ";
}
return 0;
}
The Current State of C++ in 2024
Overview of the C++ Standard
As we step into 2024, the C++ programming language continues to evolve. The C++20 standard has introduced several impactful features such as concepts, ranges, and coroutines, enhancing both the functionality and usability of the language. These features have paved the way for C++23, which is expected to build upon the advancements made in C++20, offering developers even more tools to write efficient and elegant code. Understanding the standard's evolution is crucial in realizing the importance of contributions as they help shape the future of C++.
The Importance of Community Contributions
Contributions from the community are vital for the growth and improvement of C++. From bug fixes and feature enhancements to tutorials and documentation, community efforts fuel the development of libraries, frameworks, and tools that many developers rely on daily. The C++ Standards Committee, comprised of dedicated individuals from various backgrounds, plays a central role in this ecosystem, but it is the community's contributions that often lead to innovative solutions and rapid advancements.

Types of C++ Contributions
Code Contributions
Code contributions are at the heart of the C++ community. They involve adding new features, fixing bugs, and improving existing codebases. Many notable C++ projects, such as Boost and the LLVM project, began as community initiatives. These contributions not only enhance the code’s functionality but also help foster collaboration among developers.
An example of a simple code contribution could be adding a new algorithm to the standard library. When you contribute code, it’s important to follow existing coding standards and ensure that the new code integrates smoothly with the existing codebase.
Documentation and Tutorials
Good documentation is crucial for the usability of any software. As valuable as the code itself, thorough documentation aids both new and experienced developers in understanding how to use libraries and frameworks effectively. Contributing to documentation can involve improving existing content, writing guides, or even translating documentation into different languages.
Platforms like GitHub and ReadTheDocs are great places to start contributing. If you’re interested in writing tutorials, focus on topics that can help others get started or troubleshoot common issues. A well-written tutorial can significantly reduce the learning curve for newcomers.
Bug Reporting and Feature Requests
Reporting bugs and suggesting new features is another excellent way to contribute to C++. When encountering an issue, it's important to document it clearly. Describe how to reproduce the bug and any relevant information. Many projects maintain a dedicated space for issues, like GitHub issues, where users can report problems directly.
Offering suggestions for new features can also be incredibly valuable. Think critically about how the language or a library could be improved and present your ideas constructively to the project maintainers.
Community Involvement
Community involvement is essential to the C++ ecosystem. Engaging in forums, mailing lists, and discussion groups can give you insights into the latest trends, challenges, and opportunities in the field. Some popular community forums include Stack Overflow, the C++ subreddit, and dedicated C++ Discord servers. Participating in these platforms not only helps you learn but also allows you to assist others, fostering a supportive environment.
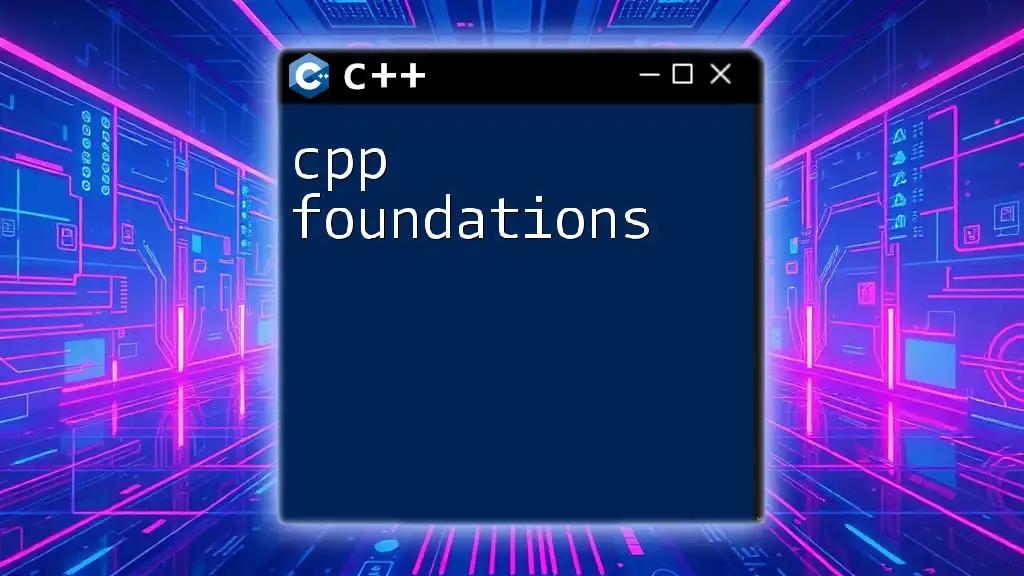
Ways to Contribute in 2024
Open Source Projects
Open source projects provide a fantastic avenue for contributions. With thousands of repositories on platforms like GitHub, finding a project that resonates with your interests is easier than ever. Engaging with a project can start with simple tasks, such as fixing typos or resolving minor bugs. Many projects also label issues suitable for newcomers, making it easier to get started.
Writing Blogs and Articles
Sharing your knowledge through blogs and articles contributes significantly to the community. Writing about your experiences and insights allows you to teach others and promote best practices. Additionally, it helps reinforce your understanding of C++. When crafting your articles, ensure they are well-structured, clear, and contain practical examples that readers can follow.
Participating in Conferences and Meetups
Conferences remain a premier platform for networking and showcasing contributions. In 2024, major C++ conferences such as CPPCon and Meeting C++ will host talks, workshops, and discussions regarding the latest advancements and contributions to the language. Attending these events is an excellent way to meet other contributors, learn from experts, and share your own work with a broader audience.
Mentor and Educate
Mentorship is a powerful tool in the community. If you feel experienced enough, consider taking on a mentor role. Helping guide newcomers not only reinforces your own knowledge but also fosters a sense of community and support. Through mentorship, you can share your expertise, provide valuable feedback, and inspire the next generation of C++ developers.

Tools and Best Practices for C++ Contributions
Version Control Systems
Using a version control system like Git is essential for effective collaboration. Git allows you to track changes, revert to previous versions, and collaborate efficiently with others. Familiarize yourself with basic Git commands such as:
git clone [repository URL]
git commit -m "Descriptive message"
git push origin [branch name]
These commands form the foundation of version control, ensuring your contributions are well-managed and documented.
Coding Standards and Style Guides
Maintaining coding standards is imperative for collaborative projects. Guidelines such as the Google C++ Style Guide or the C++ Core Guidelines help ensure consistency and readability. Using tools like linters can assist you in adhering to these standards, catching potential problems before they reach the production stage.
Testing and Quality Assurance
Writing tests for your contributions is crucial to maintaining the integrity of the codebase. Effective testing practices help catch bugs early in the development lifecycle. Popular testing frameworks such as Google Test provide a comprehensive suite for writing and running tests. Here's an example of a simple test:
#include <gtest/gtest.h>
int Add(int a, int b) {
return a + b;
}
TEST(AddTest, PositiveNumbers) {
EXPECT_EQ(Add(1, 2), 3);
EXPECT_EQ(Add(5, 5), 10);
}
Creating such tests verifies the functionality of your code and encourages others to implement tests for their contributions.

Recognizing Contributions
Contribution Recognition Programs
Various programs exist to recognize significant contributions made by developers in the C++ community. Recognition boosts morale and motivation, encouraging individuals to keep contributing and improving. From community awards to public acknowledgment at conferences, these programs can provide visibility and appreciation for your efforts.
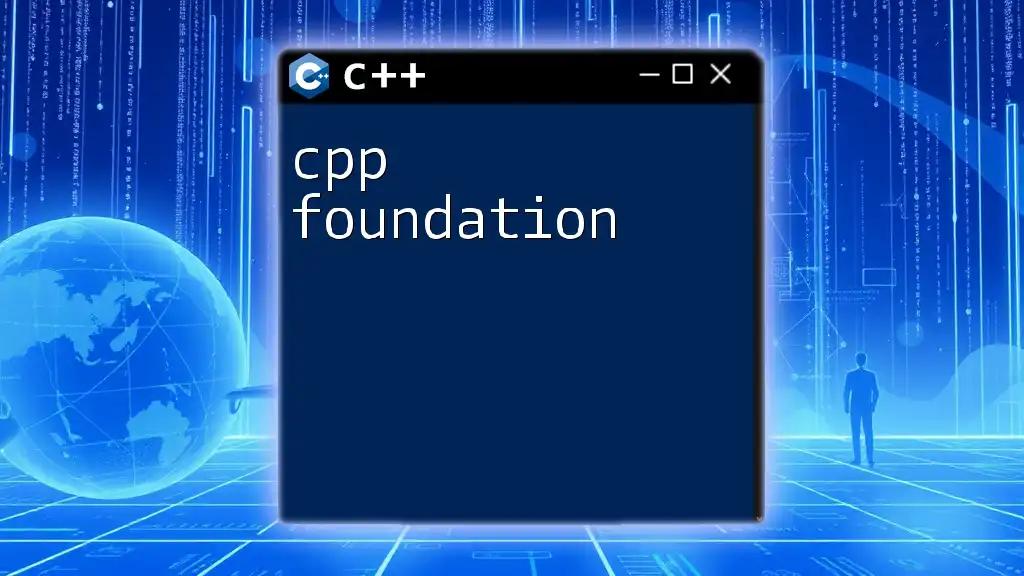
Conclusion
C++ contributions in 2024 are not only vital for the language's development but also for personal growth within the community. Engaging in various forms of contributions—be it code, documentation, or mentorship—helps enrich the C++ ecosystem and enhances your skills as a developer.
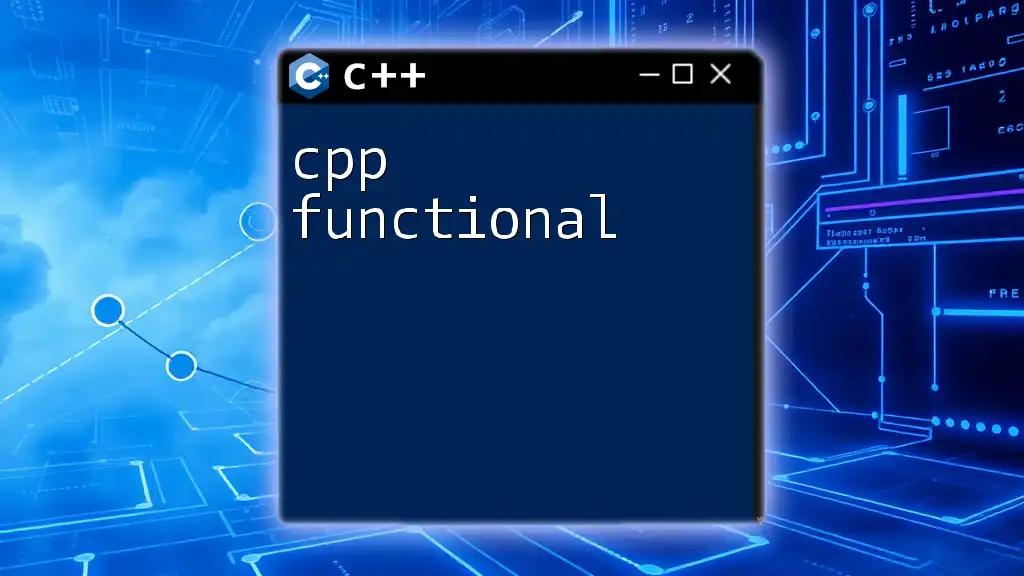
Call to Action
Explore the diverse opportunities available for C++ contributions, whether you’re a seasoned developer or new to the field. The C++ community is thriving, and your participation can make a significant difference.
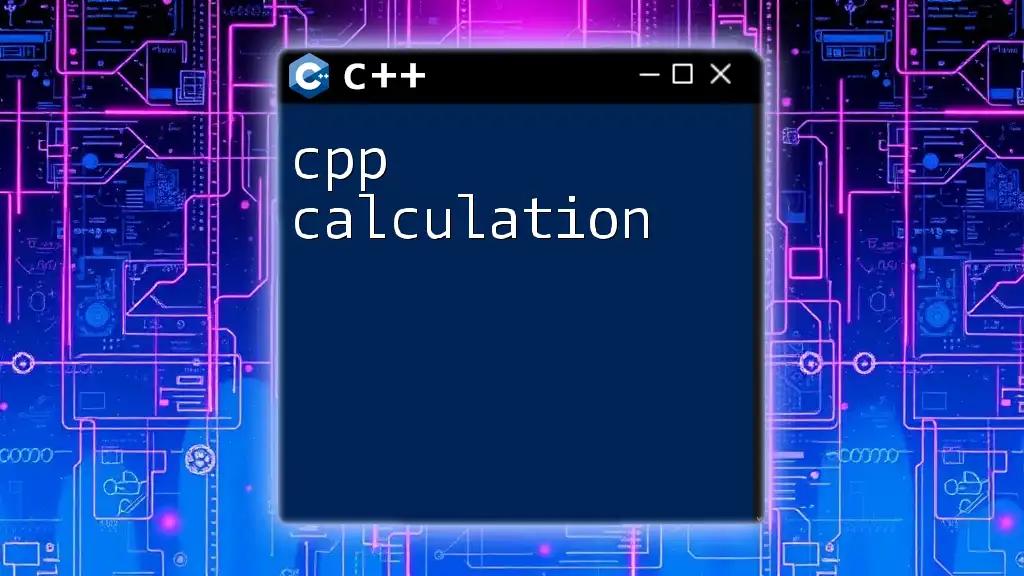
Additional Resources
To further foster your journey in contributing to C++, consider checking out recommended books, online courses, and active communities. Get involved today, and be part of the next exciting chapter in the C++ story!