In 2023, cpp contributions focus on enhancing code efficiency and developer productivity through innovative libraries and optimized algorithms. Here's a simple example of a C++ command demonstrating the use of a standard library algorithm:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 3, 8, 1, 2};
std::sort(numbers.begin(), numbers.end());
for(int n : numbers) std::cout << n << " ";
return 0;
}
Understanding C++ Contributions
What are C++ Contributions?
C++ contributions encompass a variety of actions that individuals and organizations take to enhance the C++ programming language and its ecosystem. This may include writing and improving code, providing documentation, creating tutorials, and contributing to libraries and frameworks. Contributions can play a crucial role in keeping C++ relevant and robust in a rapidly changing programming landscape.
Why Contributions Matter
Contributions to C++ are vital for several reasons. They not only foster innovation and growth within the C++ community but also provide numerous benefits to the contributors. When developers engage in contributing, they enhance their own skills, expand their professional network, and gain recognition among peers. Being part of a collaborative effort can drive motivation and encourage personal growth – quite essential for any programmer's career trajectory.
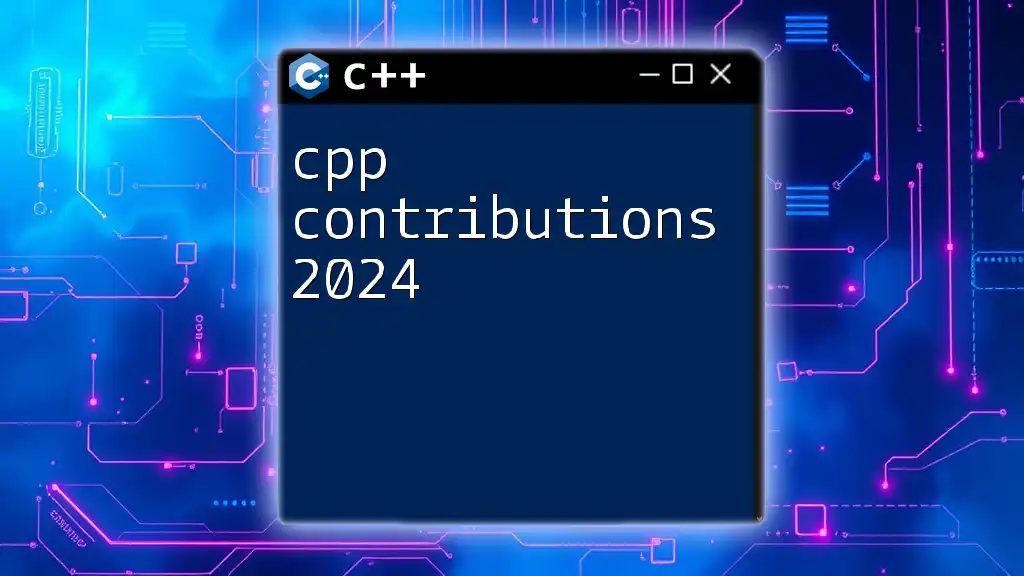
Major Contributions in 2023
Key Features Introduced
C++23 Overview
The release of C++23 is a landmark event in the programming world, characterized by its substantial enhancements and novel features. It brings improvements that focus on making the language more expressive and easier to use.
- Ranges: C++23 introduces ranges, which facilitate working with collections of data more intuitively. The syntax is cleaner and allows for more functionality like filtering and mapping. Here’s an example of how to use ranges:
#include <iostream>
#include <vector>
#include <ranges>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
// Using the views from ranges
auto even_numbers = numbers | std::views::filter([](int n) { return n % 2 == 0; });
for (int n : even_numbers) {
std::cout << n << " "; // Output: 2 4
}
}
- Calendar and Time Zone Library: C++23 introduces a robust calendar and time zone library that simplifies managing dates and times. This is crucial for applications involving scheduling or time-sensitive transactions. Example usage:
#include <chrono>
#include <iostream>
int main() {
auto now = std::chrono::system_clock::now();
std::cout << "Current time in seconds since epoch: " << std::chrono::duration_cast<std::chrono::seconds>(now.time_since_epoch()).count() << std::endl;
}
- Pattern Matching: This feature provides a more efficient way to manage complex object patterns directly in the code and enhances readability. An example of basic pattern matching:
#include <iostream>
#include <variant>
int main() {
std::variant<int, float, std::string> v = 42;
switch (v.index()) {
case 0: std::cout << "Integer: " << std::get<int>(v); break;
case 1: std::cout << "Float: " << std::get<float>(v); break;
case 2: std::cout << "String: " << std::get<std::string>(v); break;
}
}
Community Contributions
Open Source Projects
The open-source movement is at the core of C++ contributions. Major projects, such as Boost and LLVM, have welcomed significant enhancements throughout 2023, shaping the capabilities of C++.
-
Boost Library Updates: The Boost libraries have been improved with new algorithms and enhanced functionality, reflecting community-driven contributions. These updates include additional utility functions and improved performance metrics.
-
LLVM Enhancements: As a fundamental infrastructure for compiler design, LLVM saw substantial contributions related to overall compilation performance, optimizations, and support for new architectures. Developers utilized optimization goals to help maximize efficiency.
Documentation and Education
An often overlooked area of contribution is documentation, yet it plays an essential role in making new features accessible to all users. In 2023, several key contributors have enhanced official documentation across various C++ projects, offering clarity and guidance.
Useful guides and resources created this year have greatly aided understanding, such as:
- C++ Core Guidelines: An effort to help developers write safer and more efficient C++ code.
- Online Tutorials: Several community members have produced video tutorials and written content that demystifies new features introduced in C++23.
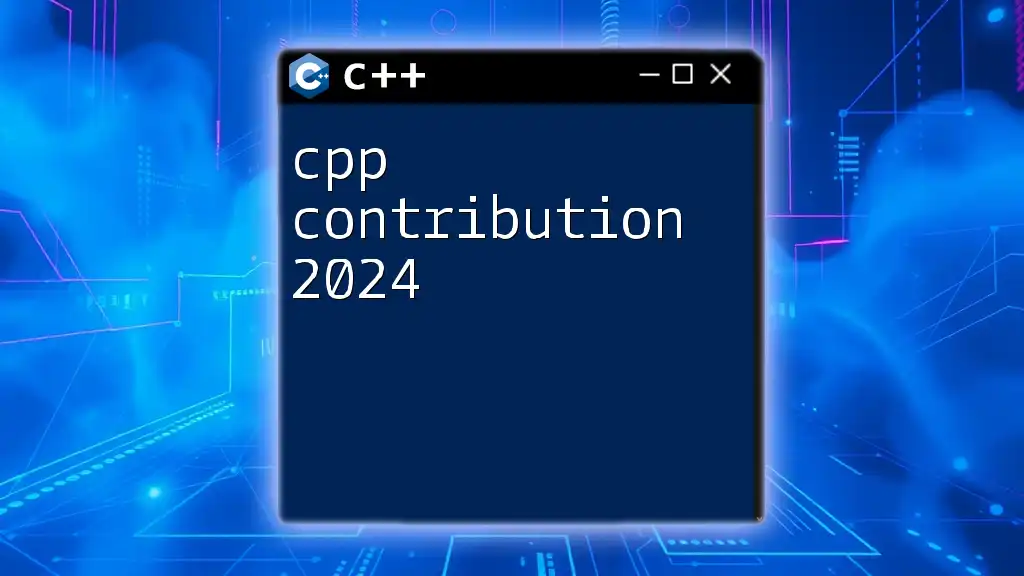
Tools and Frameworks
Updated Development Tools
In 2023, popular C++ tools have seen significant upgrades to support new C++ standards, enhancing productivity and code quality.
-
Compilers: Notably, new versions of GCC, Clang, and MSVC have rolled out with first-class support for C++23 features. With improved debugging tools and error diagnostics, programmers are equipped to write cleaner code more easily.
-
IDEs: Integrated Development Environments like Visual Studio and CLion have added functionalities for quick navigation and more intuitive coding practices. Code completion mechanisms have been improved, leading to a more productive coding experience.
Popular C++ Frameworks
C++ frameworks are the backbone of most C++ projects, and updates in popular frameworks significantly influence development. For instance:
-
Qt Framework: The Qt framework has seen a range of improvements and expanded functionalities, particularly around QML and asynchronous programming, making it even more robust for cross-platform application development.
-
Boost Libraries: Bug fixes and enhancements have strengthened the usability and capability of the Boost Libraries, ensuring that they remain a staple in C++ developers' toolkits.
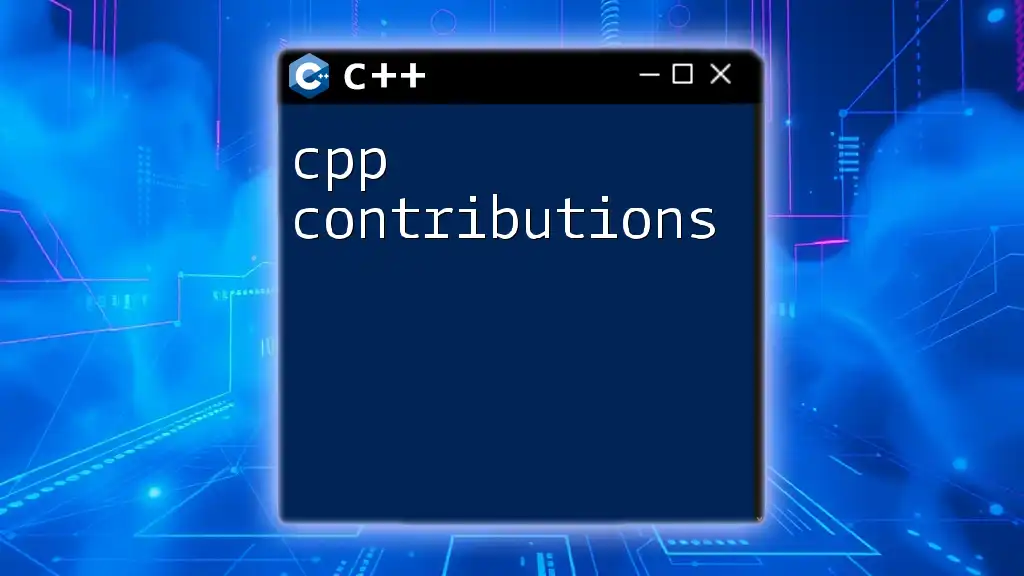
Contributing to C++ in 2023
How to Get Started
Finding a Project
Entering the realm of contributions can be daunting, but the right project can make all the difference. Resources such as GitHub and GitLab are gold mines for finding open-source C++ projects. Spend time browsing repositories tagged with “C++” or “help wanted” to identify where your skills can be best utilized.
Writing Great Code
Quality is paramount when contributing to C++. Here are a few critical tips for ensuring your submissions stand out:
- Adhere to coding standards: Familiarize yourself with the C++ coding conventions to maintain consistency across projects.
- Proper documentation: Glancing through a codebase usually isn’t enough; well-documented contributions help ensure that your work is easily understood by other developers.
Community Involvement
Joining C++ Communities
Networking within the C++ community can yield tremendous benefits. Seek out various forums, Slack groups, and online communities dedicated to C++. Engaging with these groups allows for knowledge sharing, problem-solving, and collaboration on projects.
Participating in Events
Attending C++ conferences and workshops remains one of the best ways to immerse yourself in the community. Events in 2023 have focused on exploring new features, sharing best practices, and fostering collaboration. By attending, you can connect with experienced developers and contribute to lively discussions on the future of C++.
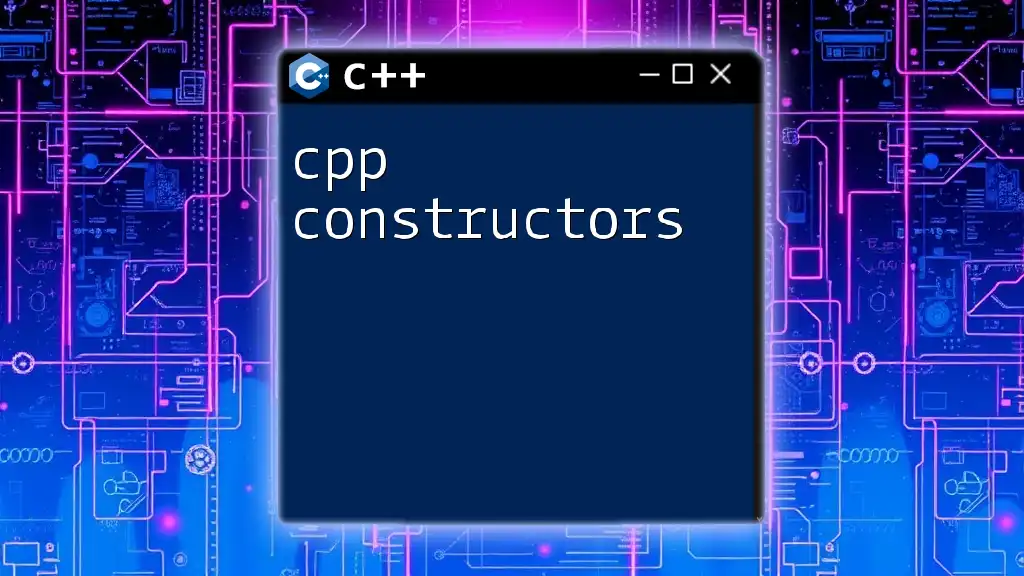
Challenges in C++ Contributions
Common Hurdles
Contributing to C++ is a rewarding yet challenging venture. Common obstacles include understanding existing codebases, compatibility issues, and maintaining clarity in documentation. While these roadblocks can be daunting, many developers find that collaborating with others or seeking mentorship plays a crucial role in overcoming them.
Navigating Legacy Code
Contributing to legacy code can be tricky, yet it offers valuable experience. Here are some best practices to consider:
- Understand the context: Familiarize yourself with the architecture and purpose of the legacy system before making changes.
- Test thoroughly: Always ensure that your modifications integrate smoothly with the existing code by running comprehensive tests.
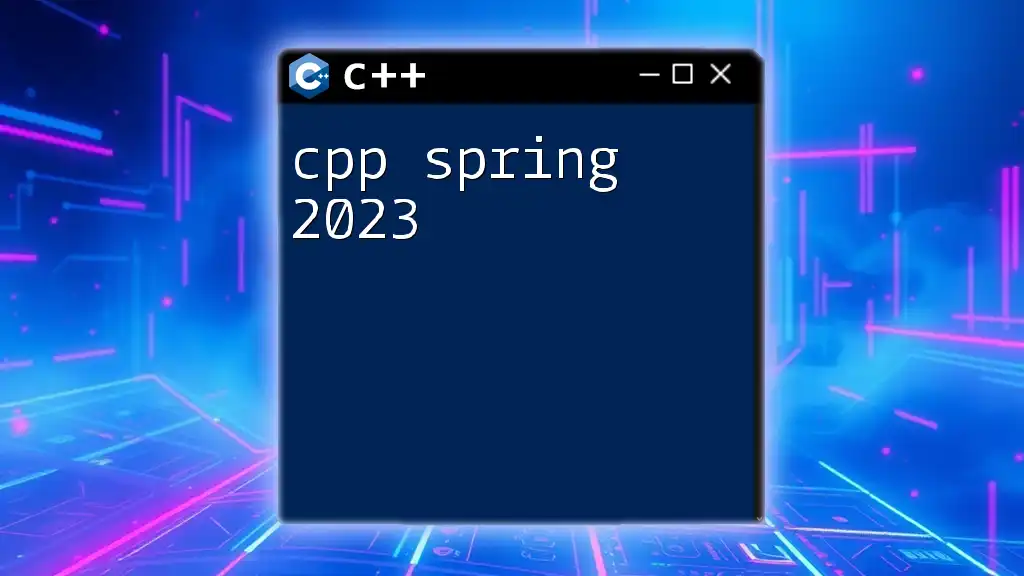
Conclusion
The landscape of C++ contributions in 2023 is vibrant and flourishing. With numerous enhancements to the language, substantial community efforts, and a range of tools available, it’s an exciting time to be involved in C++. Whether you're an experienced developer or just starting, engaging in contributions can lead to personal growth and greater impact on the C++ community.
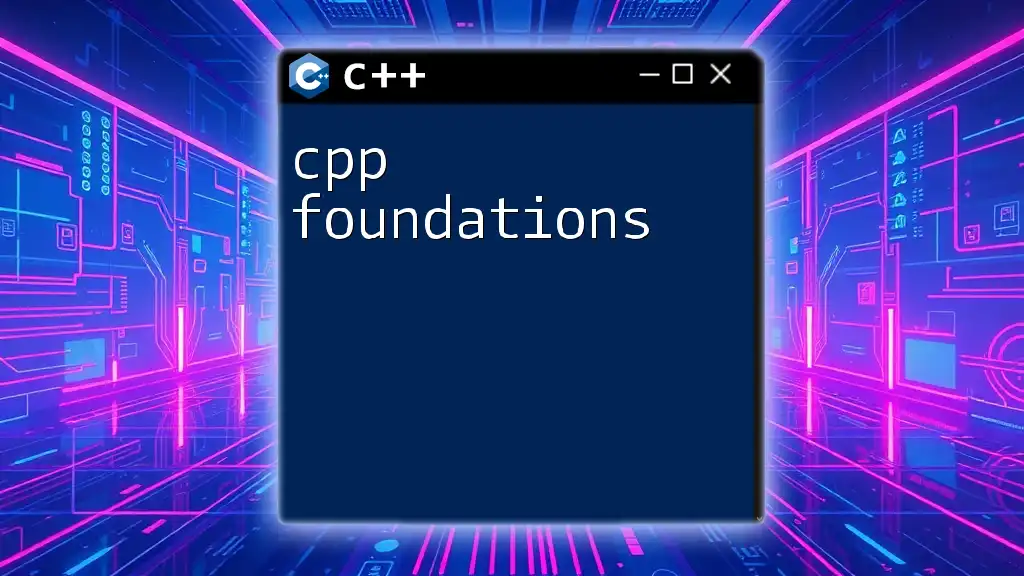
Resources
For those interested in furthering their C++ knowledge and getting involved in contributions, numerous resources are available:
- C++ Core Guidelines: A comprehensive set of guidelines for writing safe and efficient C++ code.
- Official C++ standards documentation: Stay updated with the latest principles and features.
- Online forums and communities: Join platforms like Stack Overflow, C++ Reddit, and specialized Discord servers for ongoing discussions and support.
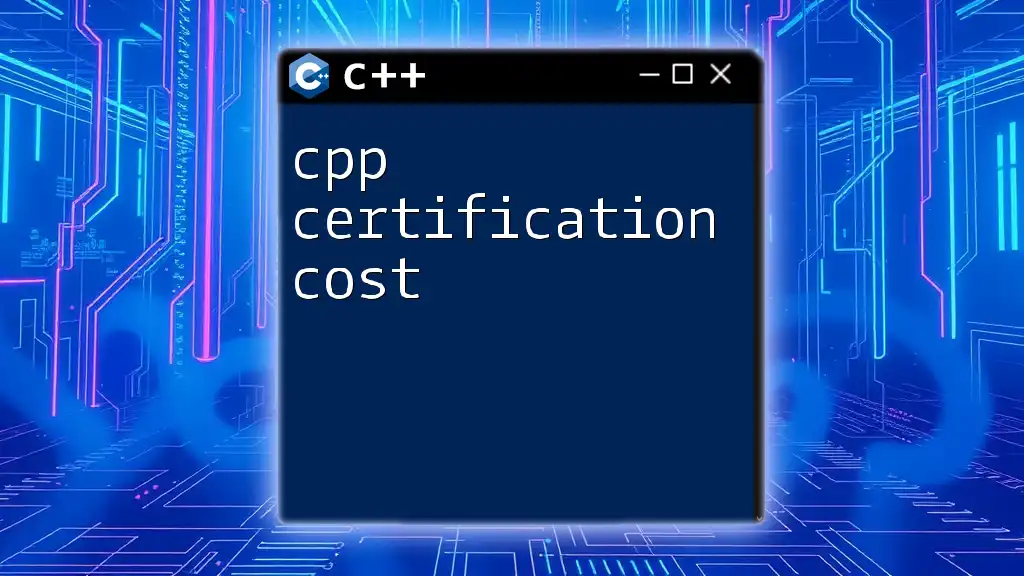
Call to Action
We invite you to share your thoughts and experiences in the comments below. Have you contributed to C++ this year? What challenges did you face? Let’s inspire one another to continue promoting innovation in the C++ community!