In 2024, cpp contributions will focus on enhancing community collaboration and simplifying access to libraries, enabling developers to create efficient and robust applications using streamlined command syntax.
Here's an example of a simple C++ program that demonstrates how to print "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Contributions
What Are C++ Contributions?
In the context of C++, contributions encompass any efforts made to improve the language, its libraries, or its community. This can include:
-
Code Contributions: This covers a wide range of activities such as developing new libraries, fixing bugs, or optimizing existing code to enhance performance. For instance, if you identify a performance bottleneck in the standard library, you could write a patch to address it.
-
Documentation and Tutorials: Clear and comprehensive documentation is vital for any programming language. By creating tutorials, writing guides, or updating documentation, you are helping others navigate the complexity of C++. Good documentation can significantly lower the learning curve for new developers.
-
Community Engagement: Engaging with the C++ community is equally important. This can involve answering questions in forums, mentoring new contributors, or attending and participating in C++ conferences. The strength of a programming ecosystem often lies in its community support.
Why Contributions Matter
The value of contributions to C++ cannot be overstated. They are essential for improving language features and libraries, making the ecosystem richer and more robust. Contributions ensure the following:
-
Enhanced Performance: As technology evolves, so do the expectations for performance. Contributions that optimize existing code or introduce efficient algorithms can greatly enhance the performance of C++ applications.
-
Community Growth: By participating in the community, you help in building a network of developers who support each other. This not only fosters innovation but also narrows the gap for newcomers to join the fold.
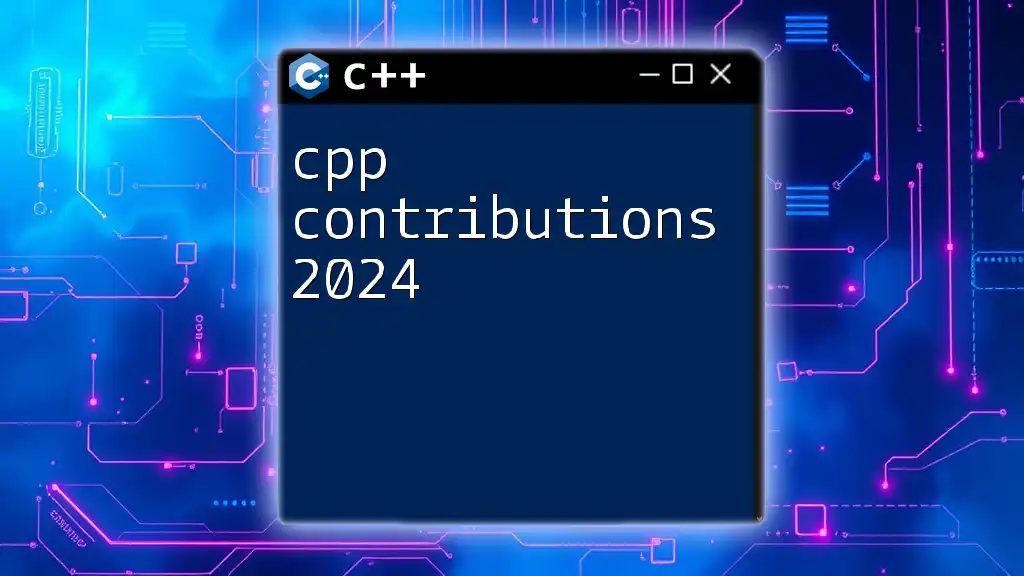
Getting Started with C++ Contributions
Setting Up Your Environment
Before making contributions, it's crucial to establish a suitable development environment. Here are some tools and resources that can aid your setup:
-
Recommended IDEs: Popular IDEs for C++ development include Visual Studio, CLion, and Eclipse CDT. Each has its strengths: Visual Studio is known for its excellent debugging tools, while CLion offers superb code analysis features.
-
Version Control with Git: Understanding how to use Git is fundamental. Whether for version control or collaboration, Git lets you track changes, revert to previous states, and collaborate efficiently with others.
Creating a Development Workflow
An effective development workflow will help maintain organization and clarity in your contributions:
-
Adopt Coding Standards: Follow established coding standards such as the Google C++ Style Guide to ensure consistency and readability across the codebase.
-
Testing and Iteration: Implement unit tests for your code to catch errors early and ensure functionality. This iterative approach not only enhances the quality of your contribution but also builds trust with maintainers.
Finding Contribution Opportunities
Open Source Projects to Consider
There is a wealth of open-source projects that welcome contributions. Therapeutic platforms like GitHub host numerous repositories where you can lend a helping hand. Some notable projects include:
- Boost Libraries: Enhance the functionality of C++ by contributing to this widely used library collection.
- LLVM: A powerful compiler framework that offers opportunities for optimization and features enhancements.
Identifying Areas in Need
Finding areas ripe for contribution requires a bit of detective work. Here’s how to approach it:
-
Explore Issues and Feature Requests: Most projects have an "issues" section where maintainers highlight bugs or feature requests. Engage with these threads to see how you can help.
-
Community Feedback: Pay attention to discussions within community forums. Often, users will highlight pain points or missing features that could guide your contributions.
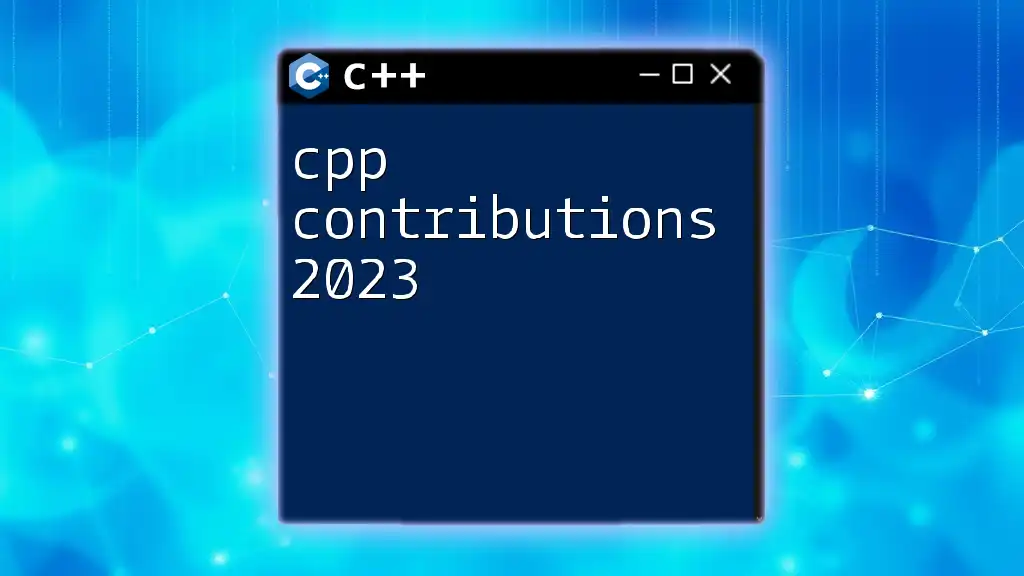
Best Practices for C++ Contributions
Coding Standards and Guidelines
Contributing code means adhering to community coding standards to ensure consistency across the project. Focusing on a few key aspects can significantly improve your contribution:
-
Conform to Standards: Adhering to established coding guidelines, like the Google C++ Style Guide, can prevent style inconsistencies that detract from the readability of your code.
-
Writing Clean and Maintainable Code: Clear code is easier to maintain and debug. Consider the following example that illustrates refactoring for clarity:
Before:
void calculate(int x) {
if(x % 2 == 0) {
std::cout << "Even" << std::endl;
}
}
After:
void printIfEven(int number) {
if (number % 2 == 0) {
std::cout << "Even" << std::endl;
}
}
With the refactored version, the function name immediately conveys its purpose, enhancing readability.
Effective Communication
Clear communication can dramatically improve your contributions:
- Documenting Your Work: Always write clear commit messages. A well-crafted commit message can help maintainers understand your modifications quickly. For example:
Fix memory leak in buffer management
- Engaging with the Community: Take time to engage with other developers through forums like Stack Overflow or the C++ Reddit community. Ask questions, offer help, and discuss new ideas. This engagement builds relationships that can spur collaboration on future projects.
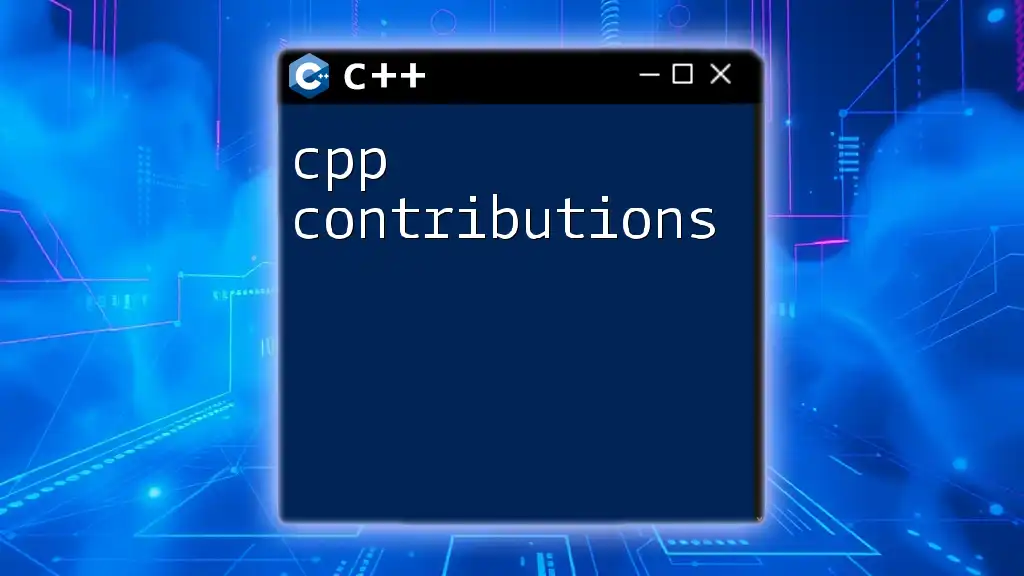
Example Contribution Process
Step-by-Step Guide to Contributing
If you’re new to contributing, here’s a concise step-by-step guide to get you started:
Finding a Project
Begin by browsing repositories on platforms like GitHub. Look for projects that have an active community and clear contribution guidelines.
Forking and Cloning Repositories
Once you've chosen a project, fork it to your account and clone it locally. Use the following commands:
git clone https://github.com/yourusername/projectname.git
Making Changes and Testing
Make your changes, remembering to keep your commits focused. After modifying a file, test your changes thoroughly. For instance, consider creating simple functionality:
void greet() {
std::cout << "Hello, C++ Community!" << std::endl;
}
Run your tests to ensure everything functions as expected.
Submitting a Pull Request
Once your changes are ready, submit a pull request (PR). Follow any templates provided by the project and be respectful in your communication. This step allows maintainers to review your work, improve it with constructive feedback, and ultimately accept your contribution.
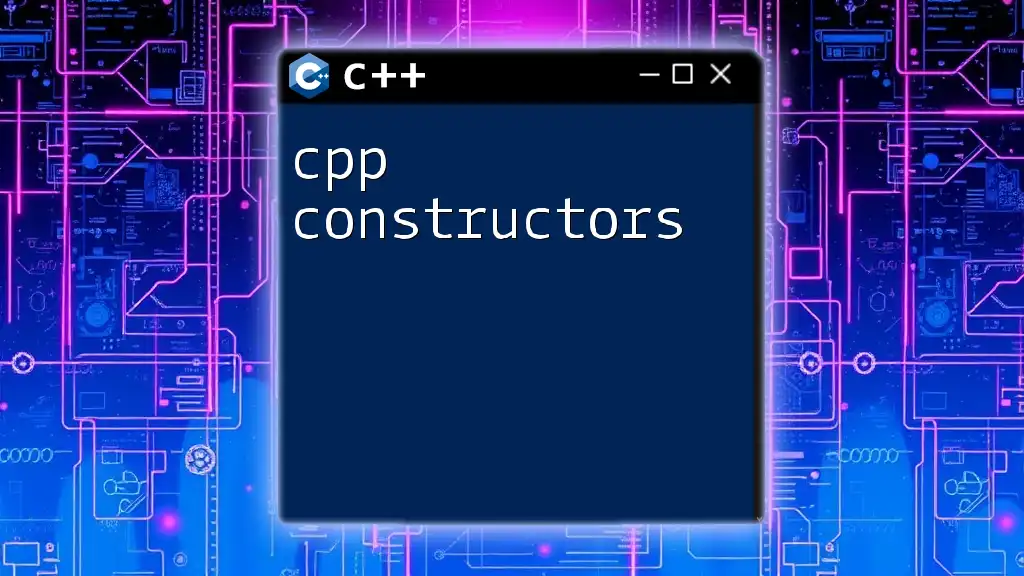
Trends to Watch in 2024
Upcoming C++ Standards
With every year, the C++ landscape evolves, particularly with new standards emerging. C++23 has introduced several key features, such as:
- Modules: A new way to organize code and improve compilation speeds.
- Coroutines: Simplifying asynchronous programming, making it more intuitive.
These changes open exciting doors for contributions, as developers will require updated libraries, tools, and documentation to harness the full potential of these features.
Emerging Technologies
Stay informed about integrating C++ with modern technologies. The rise of AI and machine learning means that C++ can play a significant role in building performant applications in these fields. Contributions can focus on creating wrappers for popular AI libraries or optimizing existing algorithms to leverage new hardware capabilities.
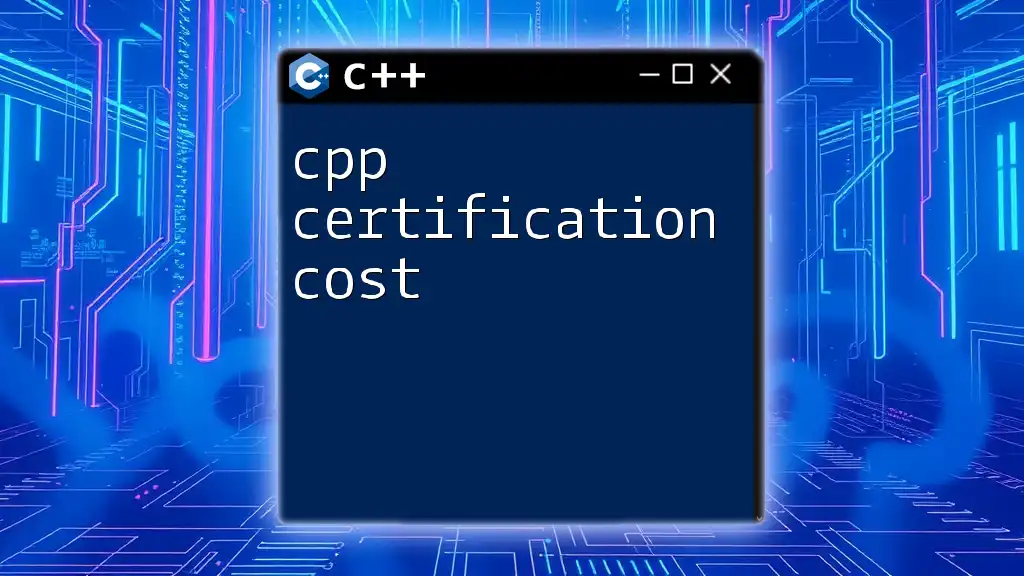
Conclusion
The C++ community thrives on contributions. Whether you are fixing bugs, writing documentation, or mentoring others, your efforts have a lasting impact on the ecosystem. As we approach 2024, I encourage you to jump in and start contributing. The benefits of involvement include not just enhancing your skills but also forming valuable connections within a vibrant community.
Remember, every small contribution counts, so don’t hesitate to share your knowledge and code!
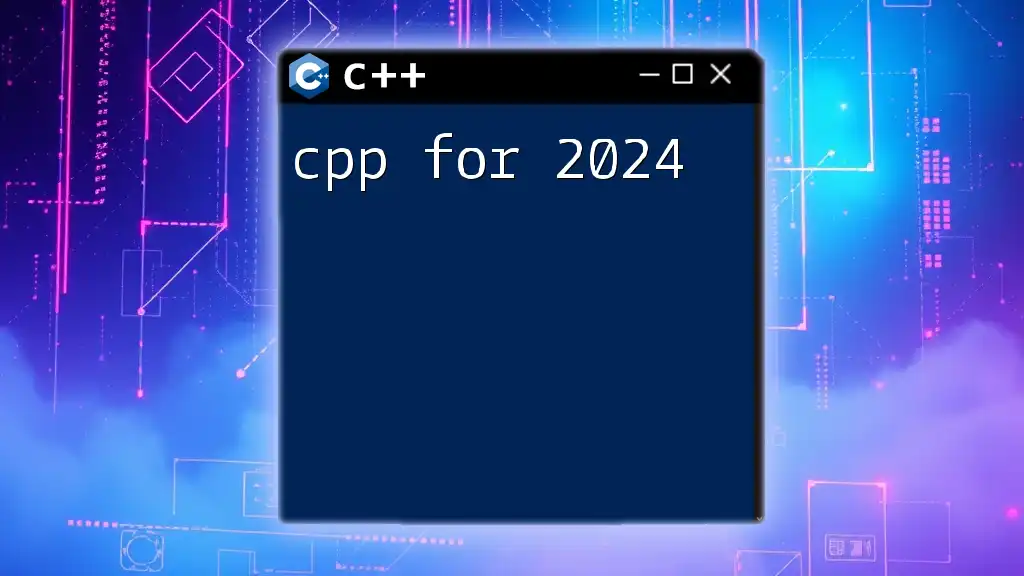
Additional Resources
For those eager to deepen their understanding and involvement, consider the following:
- C++ Resources: Books, online courses, and coding challenges tailored for C++ development.
- Community Forums: Join discussions on platforms like Stack Overflow, Reddit, or specialized C++ forums to share experiences and seek guidance.
Embrace the spirit of collaboration, and let’s make cpp contribution 2024 a memorable year!