"CPP foundations" refers to the essential principles and basic constructs of the C++ programming language, which empower developers to write efficient and effective code.
Here’s a basic example demonstrating the syntax for defining a simple function in C++:
#include <iostream>
void greet() {
std::cout << "Hello, World!" << std::endl;
}
int main() {
greet();
return 0;
}
Understanding C++
What is C++?
C++ is a powerful programming language that evolved from C, adding object-oriented features. Created in the early 1980s by Bjarne Stroustrup, C++ combines the efficiency of C with the abstraction capabilities of object-oriented programming (OOP). Today, it plays a pivotal role in various sectors, from system programming to game development and beyond.
Key Features of C++
C++ is distinguished by several key features that enhance its capability and versatility:
Object-Oriented Programming (OOP)
C++ is built around the concept of classes and objects, which facilitates code reusability and modularity. Fundamental concepts include:
- Classes: Templates for creating objects. They encapsulate data and functions that operate on the data.
- Objects: Instances of classes that hold specific data.
- Inheritance: The mechanism of deriving new classes from existing ones. This promotes code reuse and the establishment of a hierarchical relationship.
- Polymorphism: The ability for different classes to be treated as instances of the same class through a common interface, often using virtual functions.
Example of a basic class structure:
class Animal {
public:
virtual void speak() {
std::cout << "Animal speaks" << std::endl;
}
};
Standard Template Library (STL)
STL is a robust feature of C++ that provides a set of template classes for data structures and algorithms. Key components include containers (like vectors and lists), iterators, and algorithms.
Example using std::vector to store numbers:
#include <vector>
#include <iostream>
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
Memory Management
C++ provides fine-grained control over memory. Understanding pointers, references, and dynamic memory management is crucial.
Example using `new` and `delete`:
int* ptr = new int(10); // dynamically allocate memory for an int
std::cout << *ptr << std::endl; // prints 10
delete ptr; // free the allocated memory
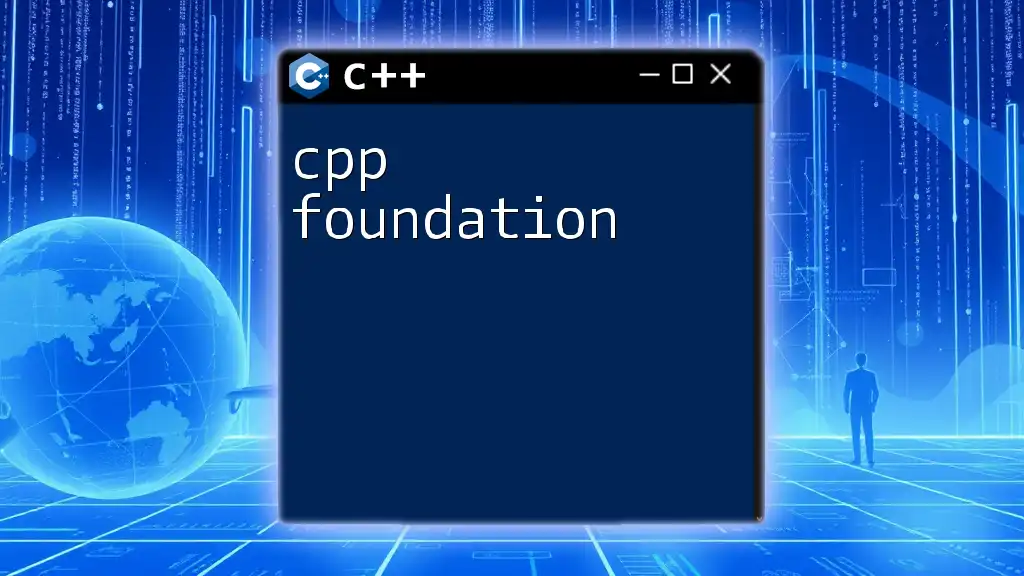
Setting Up Your Environment
Choosing a Compiler
The first step in programming with C++ is choosing an appropriate compiler. Popular options include GCC (GNU Compiler Collection), MSVC (Microsoft Visual C++), and Clang. Each has its strengths and platform compatibility, so it's important to select one that fits your development needs.
Integrated Development Environments (IDE)
A good IDE can significantly streamline your coding process. Here are some recommended options:
- Visual Studio: Offers powerful debugging tools and intelligent code completion.
- Code::Blocks: A free, open-source IDE that's lightweight and customizable.
- CLion: A JetBrains product that boasts intelligent coding assistance and smart refactorings.
Compiling Your First C++ Program
Creating your first C++ program is an exciting milestone. Here’s a step-by-step guide to writing, compiling, and running your Hello World program:
- Create a new file named `hello.cpp`.
- Insert the following code:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
- Open your terminal or command prompt.
- Navigate to the directory containing `hello.cpp`.
- Compile your program using the following command:
g++ hello.cpp -o hello
- Run the compiled program:
./hello
This should output:
Hello, World!
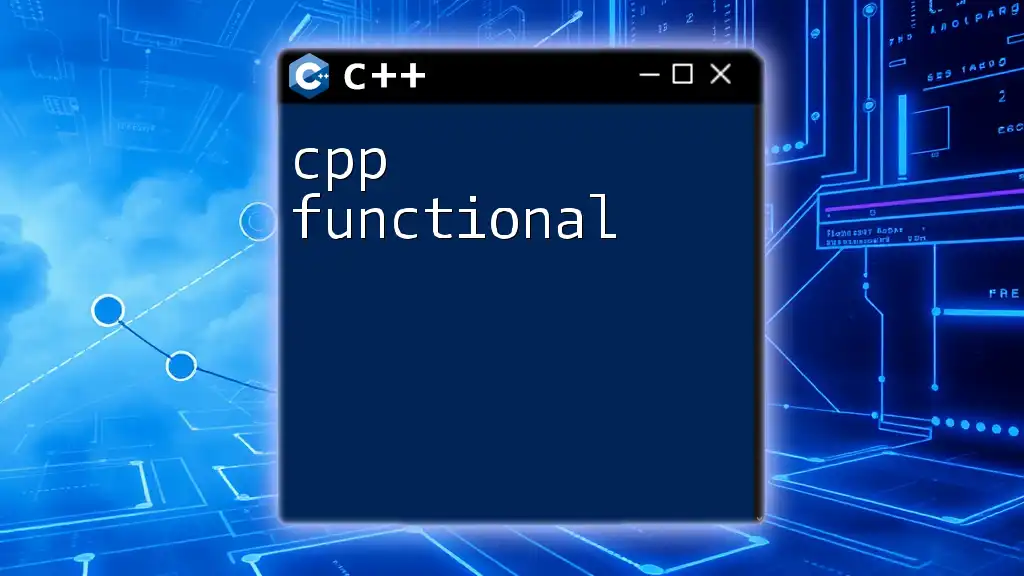
C++ Basics
Data Types and Variables
Understanding data types is fundamental in C++. The language supports a range of built-in types, including:
- int: For integers, e.g., `int age = 30;`
- float: For floating-point numbers, e.g., `float pi = 3.14;`
- char: For characters, e.g., `char initial = 'A';`
- double: For double-precision floating-point numbers, e.g., `double largeNumber = 1.2e10;`
Example: Declaration and initialization of different data types:
int a = 5;
float b = 3.14;
char c = 'A';
Operators in C++
C++ supports a variety of operators:
- Arithmetic Operators: `+`, `-`, `*`, `/`, `%`
- Relational Operators: `==`, `!=`, `>`, `<`, `>=`, `<=`
- Logical Operators: `&&`, `||`, `!`
- Bitwise Operators: `&`, `|`, `^`, `~`, `<<`, `>>`
Example: Simple arithmetic operations:
int sum = a + 10; // addition example
bool isEqual = (a == 5); // relational example
Control Structures
Conditional Statements
Control the flow of your program using if, else if, and else statements. They allow you to execute code conditionally based on logic.
Example of a basic if-else structure:
if (a > 10) {
std::cout << "a is greater than 10" << std::endl;
} else {
std::cout << "a is less than or equal to 10" << std::endl;
}
Loops
C++ supports multiple types of loops to execute a block of code repeatedly:
- for loop: Ideal when the number of iterations is known.
- while loop: Useful when the number of iterations is not predetermined.
- do-while loop: Similar to while, but guarantees at least one execution.
Example of a simple for loop:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
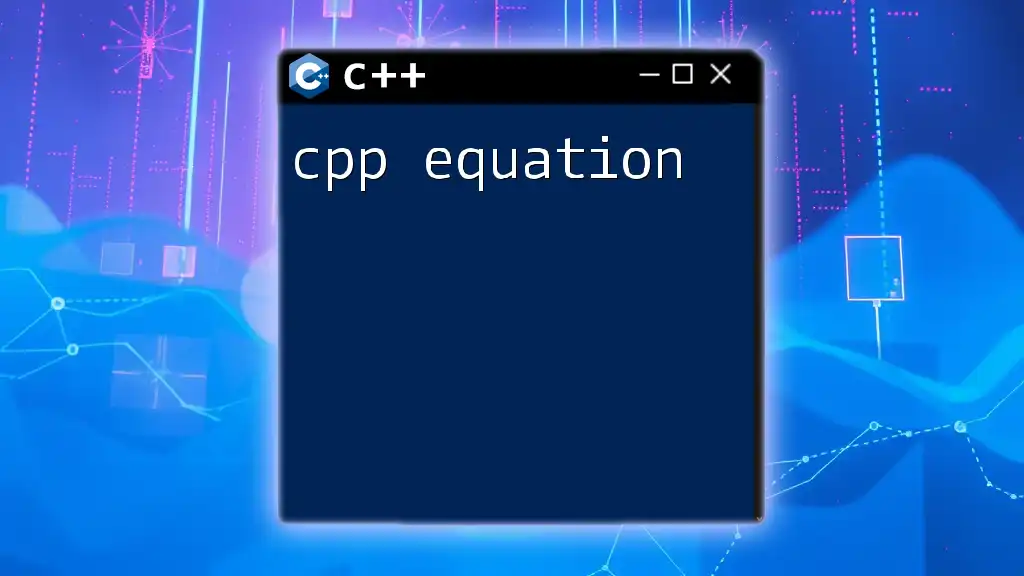
Functions in C++
Defining Functions
Functions are reusable blocks of code designed to perform specific tasks. You can define a function using the following syntax:
return_type function_name(parameters) {
// body of the function
}
Example: A simple function to calculate the square of a number:
int square(int x) {
return x * x;
}
Function Overloading
C++ allows multiple functions to share the same name, as long as their parameter types or counts are different. This feature is known as function overloading.
Example: Overloaded functions for different data types:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
Lambda Expressions
Lambda expressions provide a concise way to represent anonymous functions. They are particularly useful for passing functions as arguments to algorithms.
Example: Using a lambda for sorting:
#include <vector>
#include <algorithm>
std::vector<int> nums = {4, 2, 3};
std::sort(nums.begin(), nums.end(), [](int a, int b) { return a < b; });
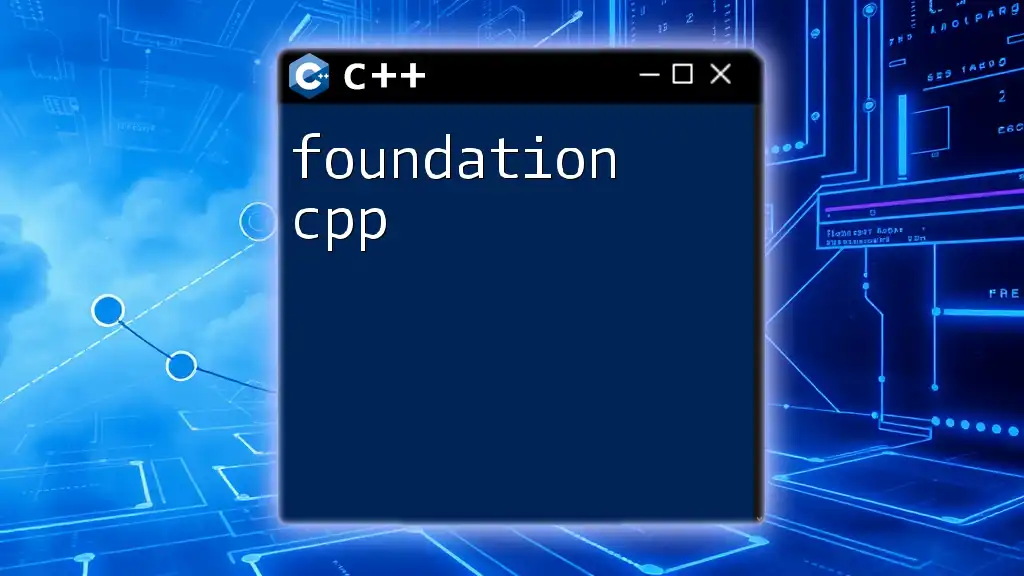
Object-Oriented Programming in C++
Understanding Classes and Objects
Classes serve as blueprints for creating objects, which hold their own data and functionality.
Example of creating a simple `Car` class:
class Car {
public:
std::string brand;
void honk() {
std::cout << "Honk!" << std::endl;
}
};
Inheritance
Inheritance allows for the creation of new classes based on existing ones, forming a hierarchical relationship. This promotes code reuse and enhances maintainability.
Example of base and derived class:
class Vehicle {
public:
void move() {
std::cout << "The vehicle moves" << std::endl;
}
};
class Bike : public Vehicle {
public:
void ringBell() {
std::cout << "Ring ring!" << std::endl;
}
};
Polymorphism and Encapsulation
Polymorphism allows methods to do different things based on the object that it is acting upon. Encapsulation restricts access to certain components of a class, exposing only necessary parts.
Example of polymorphism with virtual functions:
class Animal {
public:
virtual void speak() {
std::cout << "Animal speaks" << std::endl;
}
};
class Dog : public Animal {
public:
void speak() override {
std::cout << "Woof!" << std::endl;
}
};
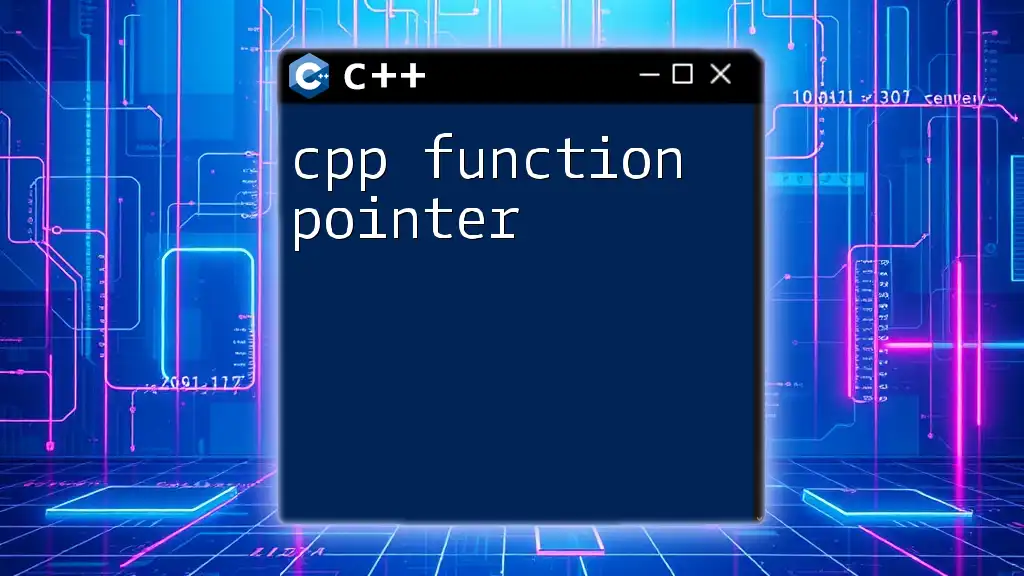
Error Handling in C++
Understanding Exceptions
Error handling in C++ can be efficiently managed through exception handling mechanisms. Using `try` and `catch` blocks allows programs to continue functioning smoothly in the event of an error.
Example of try-catch blocks:
try {
throw std::runtime_error("An error occurred");
} catch (const std::exception& e) {
std::cout << e.what() << std::endl;
}
Best Practices for Error Handling
Effective error management is crucial for robust application development. It is vital to catch exceptions and provide meaningful error messages for easier debugging. Always prioritize resource cleanup, especially in dynamic memory management.
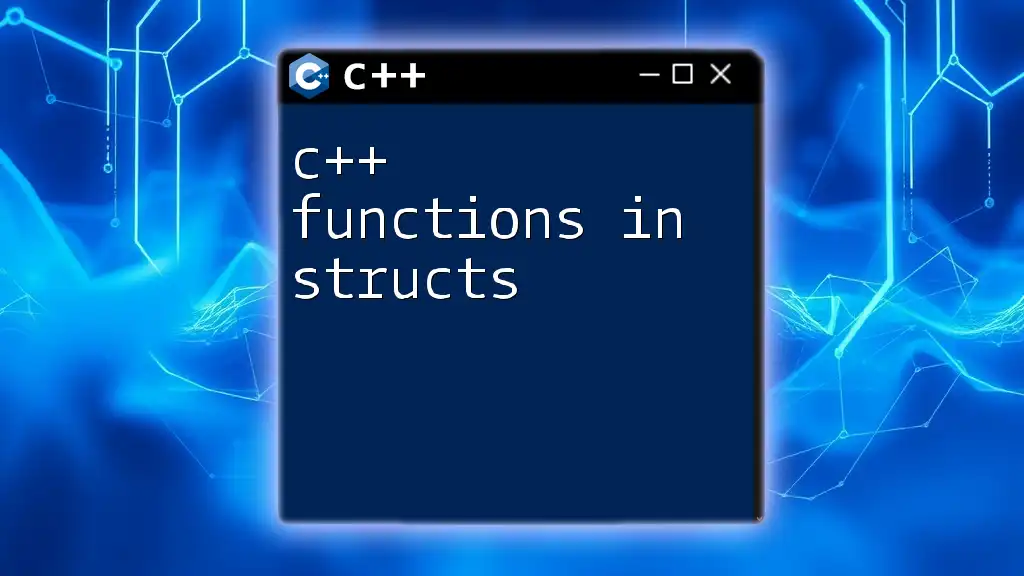
Conclusion
In summary, this guide has explored the C++ foundations, covering essential topics from data types and functions to OOP principles and error handling. By mastering these fundamentals, you can build a solid base for further learning and application in more complex C++ programming scenarios.
Next Steps
For those eager to deepen their knowledge, consider exploring advanced topics such as smart pointers, multithreading, and design patterns. Remember, practice is key to mastering C++. Engage in practical projects, partake in coding challenges, and leverage online resources to enhance your skills effectively!