In C++, you can work with dates using the `<chrono>` library, which provides a straightforward way to represent and manipulate time.
Here’s a simple example of getting the current date and time:
#include <iostream>
#include <chrono>
#include <ctime>
int main() {
auto now = std::chrono::system_clock::now();
std::time_t now_time = std::chrono::system_clock::to_time_t(now);
std::cout << "Current date and time: " << std::ctime(&now_time);
return 0;
}
Understanding Dates in C++
What is a Date?
In programming, a date represents a specific point in time, usually defined in a human-readable format such as YYYY-MM-DD. Dates are critical in various applications, from planning events to managing transactions and logging activities. Understanding how to manipulate and utilize dates effectively can significantly enhance the functionality and usability of any software.
C++ Date Libraries
C++ offers several libraries for handling dates. Two of the most notable are:
- `<chrono>`: This header provides a comprehensive set of tools for time duration and date calculations, based on modern C++ standards.
- `<ctime>`: A more traditional C-style encoding that offers basic functions for time manipulation and retrieval.
Each library comes with its specific use cases, so knowing when to apply them enhances efficiency in your programming tasks.

Using `<chrono>` for Date Manipulation
Overview of `<chrono>`
The `<chrono>` library is a modern and highly flexible tool for performance-aware date manipulation. It provides various types such as:
- `std::chrono::system_clock`: Represents the system-wide real-time clock.
- `std::chrono::duration`: Represents a time duration with a defined unit (seconds, minutes, etc.).
By leveraging these types, you can perform sophisticated date operations smoothly and effectively.
Getting Current Date and Time
Retrieving the current date and time can be accomplished simply with a few lines of code. Here’s how you can do it:
#include <iostream>
#include <chrono>
#include <ctime>
int main() {
auto now = std::chrono::system_clock::now();
std::time_t now_time = std::chrono::system_clock::to_time_t(now);
std::cout << "Current Date and Time: " << std::ctime(&now_time);
return 0;
}
In this example, `std::chrono::system_clock::now()` retrieves the current date and time as a point in time. The `std::chrono::system_clock::to_time_t(now)` function translates this point into a time_t object, which can subsequently be formatted into a human-readable string using `std::ctime()`.
Calculating Durations
C++ allows you to calculate the difference between two dates easily. Here’s an example of getting a future date by adding days to the current one:
#include <iostream>
#include <chrono>
int main() {
auto today = std::chrono::system_clock::now();
auto future_date = today + std::chrono::days(30);
std::cout << "Current Date: " << std::chrono::system_clock::to_time_t(today) << "\n";
std::cout << "Future Date (30 days later): " << std::chrono::system_clock::to_time_t(future_date) << "\n";
return 0;
}
In this case, `std::chrono::days(30)` adds 30 days to the current date. Understanding how to manipulate time durations empowers developers to build applications that require date and time forecasting.
Formatting Dates
For better user display, formatting dates is essential. You can utilize `std::put_time` from `<iomanip>` to achieve this:
#include <iomanip>
#include <iostream>
#include <chrono>
int main() {
auto now = std::chrono::system_clock::now();
std::time_t now_time = std::chrono::system_clock::to_time_t(now);
std::tm* now_tm = std::localtime(&now_time);
std::cout << "Formatted Date: " << std::put_time(now_tm, "%Y-%m-%d %H:%M:%S") << "\n";
return 0;
}
The `std::put_time` function allows you to specify a format for the output, where `%Y`, `%m`, `%d`, etc., represent year, month, and day, respectively. This flexibility helps tailor the output to match user expectations.
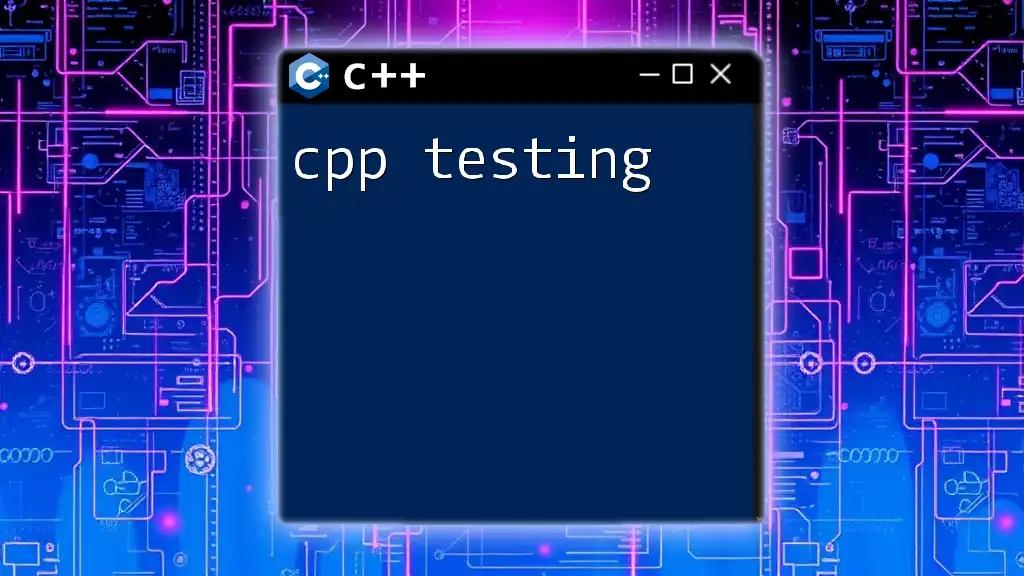
Using `<ctime>` for Date Manipulation
Overview of `<ctime>`
The `<ctime>` library provides a classic approach to date handling with a set of functions that cater to simpler use cases. Unlike `<chrono>`, it doesn’t use modern C++ constructs, making it less flexible but easier for quick dates manipulation.
Basic Functions in `<ctime>`
To get the current local time, you can use the following code snippet:
#include <iostream>
#include <ctime>
int main() {
std::time_t now = std::time(0);
std::cout << "Current local time: " << std::ctime(&now);
return 0;
}
This example showcases how `std::time(0)` fetches the current time in seconds since the epoch, and `std::ctime()` converts it into a human-readable string.
Manipulating Time with `<ctime>`
You can also convert a `tm` structure to epoch time and back. Here’s how to perform this conversion:
#include <iostream>
#include <ctime>
int main() {
std::tm timeinfo = {};
timeinfo.tm_year = 2023 - 1900; // Year since 1900
timeinfo.tm_mon = 0; // January
timeinfo.tm_mday = 1; // 1st day
std::time_t created_time = std::mktime(&timeinfo);
std::cout << "Epoch time (from tm structure): " << created_time << "\n";
return 0;
}
In this snippet, `std::mktime` converts the structured date into epoch time, providing a necessary bridge between structured and time-based programming.

Best Practices for Date Handling in C++
Choosing the Right Library
When dealing specifically with C++ dates, it’s essential to evaluate whether to use `<chrono>` or `<ctime>`. Use `<chrono>` for modern applications requiring performance optimization and precision, while `<ctime>` is suitable for legacy applications or simple tasks.
Avoiding Common Pitfalls
When working with dates in C++, be mindful of potential pitfalls:
-
Time Zones: Always account for time zone differences if your application spans multiple regions. Using `<chrono>` mitigates some issues by standardizing time representations.
-
Date Validation: Ensure that entered or calculated dates make sense (e.g., February 30 is invalid). Implement checking mechanisms to handle this and avoid runtime errors.
In conclusion, mastering C++ dates allows developers to manage temporal data effectively, enhancing application robustness and user experience. Whether you choose modern constructs provided by `<chrono>` or the traditional methods of `<ctime>`, understanding their capabilities and limitations is essential for successful date manipulation in C++.