In C++, a map is an associative container that stores elements in key-value pairs, allowing for efficient retrieval based on keys. Here's an example of how to use a map in C++:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> ageMap;
ageMap["Alice"] = 30;
ageMap["Bob"] = 25;
std::cout << "Alice's age: " << ageMap["Alice"] << std::endl;
return 0;
}
Understanding C++ Maps
What is a Map in C++?
A map in C++ is a sophisticated data structure that associates unique keys with specific values, forming a collection of key-value pairs. This allows for efficient retrieval, addition, and removal of data. Maps are notably different from other data structures like arrays and vectors, where elements are indexed numerically instead of through unique keys.
Characteristics of Maps
Maps function as associative arrays, providing a way to store data in a manner that allows for dynamic sizing. One of their most crucial features is the uniqueness of keys; no two entries can contain the same key, thereby enforcing a structure that ensures the integrity of relationships between keys and their associated values. Under the hood, maps are typically implemented using balanced tree structures like Red-Black trees, which aid in maintaining order and efficiency in operations.
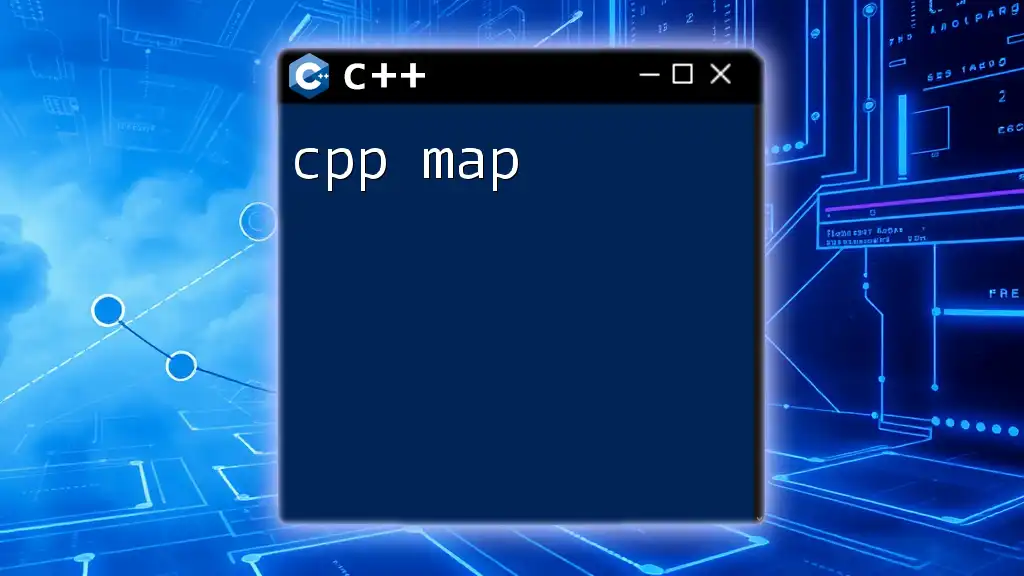
The C++ Standard Library Map
Overview of `std::map`
The C++ Standard Library provides `std::map`, which is an associative container that holds elements formed by a combination of keys and mapped values. To use maps, you must include the appropriate header file by adding the line:
#include <map>
The map’s internals manage keys in a sorted manner based on their associated values, ensuring that any insertion or retrieval operates efficiently.
Key Features of `std::map`
One of the defining features of `std::map` is its ability to automatically sort keys. This allows for orderly storage and quick access to elements. The complexity of operations like insertion, deletion, and retrieval is log(N), making maps an optimal choice for scenarios where these attributes are critical.
Additionally, `std::map` offers robust support for iterators, enabling developers to traverse the map comfortably, whether from beginning to end or in reverse order.
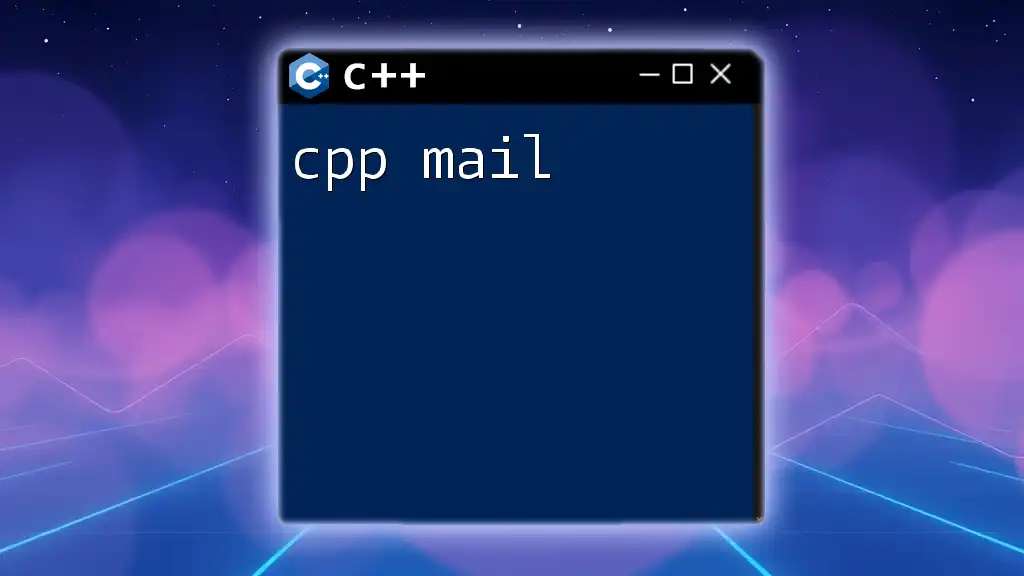
Types of Maps in C++
`std::map` vs `std::unordered_map`
While `std::map` sorts keys, `std::unordered_map` stores them in an arbitrary order, enabling average constant-time complexity for lookups. When the order of elements is not relevant, `std::unordered_map` can outperform its ordered counterpart.
Here’s a simple code snippet demonstrating both types:
std::map<int, std::string> orderedMap;
std::unordered_map<int, std::string> unorderedMap;
orderedMap.insert({1, "One"});
unorderedMap[2] = "Two";
In this example, `orderedMap` will keep its entries sorted by keys, while `unorderedMap` does not guarantee any order.
Other Map Variants
You can also utilize `std::multimap`, which allows for multiple values per key. This means you can have non-unique keys but still maintain a sorted order. Similarly, `std::unordered_multimap` provides an unordered collection with non-unique keys, ideal for specific applications where duplicates are acceptable.
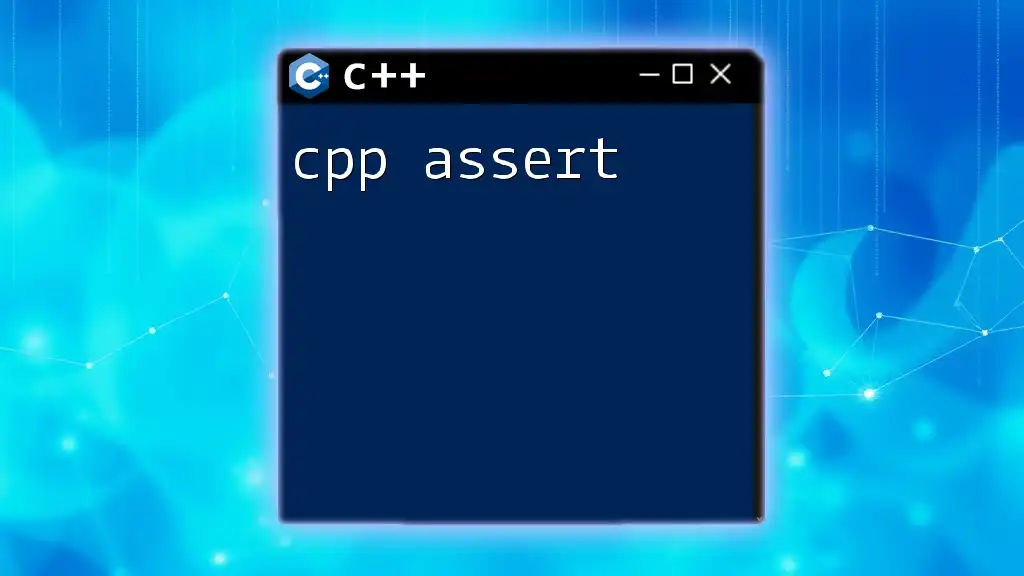
How to Use Maps in C++
Declaring and Initializing a Map
To work with maps in C++, you must first declare them. A typical declaration looks like this:
std::map<int, std::string> myMap;
You can initialize a map with specific values upon declaration:
std::map<int, std::string> myMap = {{1, "One"}, {2, "Two"}, {3, "Three"}};
Inserting Elements into a Map
C++ provides multiple ways to insert elements into a map. One common method is using the `insert()` function:
myMap.insert(std::make_pair(4, "Four"));
Another way to add elements is through the array-like syntax `[]`, which is more straightforward:
myMap[5] = "Five";
This method will automatically create an entry if the key doesn't already exist.
Accessing and Modifying Elements
Accessing elements is simple. By using the key, you get the associated value:
std::string value = myMap[1]; // "One"
You can also modify an existing entry without needing to check if the key exists, as attempting to access a non-existent key through this method initializes it to a default value (which is empty in the case of strings).
To check for the existence of a key before accessing, use the `find()` method, which returns an iterator to the found element or `end()` if it does not exist:
auto it = myMap.find(6);
if (it != myMap.end()) {
// Key exists, access it
} else {
// Key does not exist
}
Deleting Elements from a Map
The `erase()` method removes an element using its key:
myMap.erase(4); // Removes the entry with key 4
Attempting to erase a non-existent key has no effect, making this operation safe to use without prior checks.
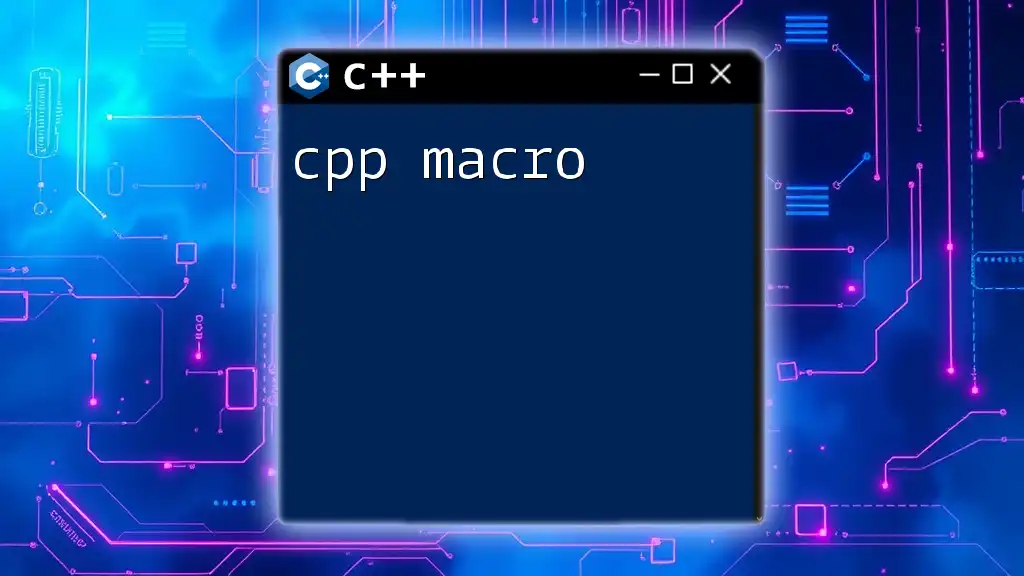
Iterating Over Maps
Iterating with Iterators
You can utilize iterators to loop through a map efficiently. Here’s an example demonstrating this approach:
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << "Key: " << it->first << ", Value: " << it->second << std::endl;
}
This code snippet prints each key-value pair in the map.
Range-based For Loop
If you prefer cleaner and more concise syntax, C++11 introduced range-based for loops, allowing you to iterate through a map easily:
for (const auto& pair : myMap) {
std::cout << "Key: " << pair.first << ", Value: " << pair.second << std::endl;
}
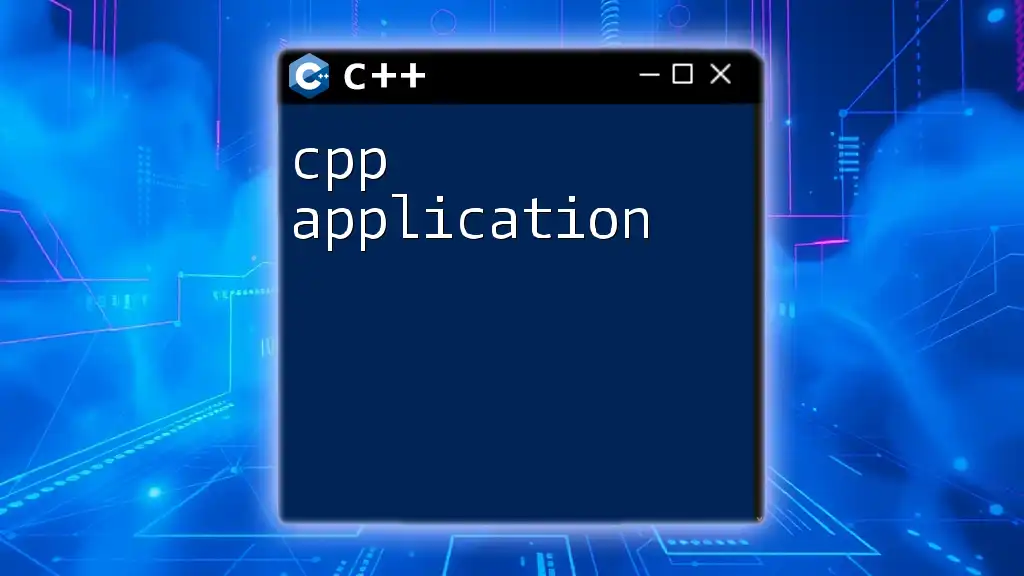
Advanced Operations on Maps
Searching in Maps
Searching within maps is straightforward and efficient. Given the tree structure, the time complexity for search operations is logarithmic. For example, retrieving an element is seamless, as shown previously with the access via `[]`.
Merging and Swapping Maps
Merging two maps can be done using the `insert()` method, which allows you to add elements from one map to another:
std::map<int, std::string> anotherMap = {{6, "Six"}, {7, "Seven"}};
myMap.insert(anotherMap.begin(), anotherMap.end());
The `swap()` function allows for the exchange of contents between two maps efficiently:
std::map<int, std::string> tempMap;
myMap.swap(tempMap);
Custom Key Types
You can create custom keys in maps by using structures or classes. To achieve this, ensure your custom key type has overloaded comparison operators, which `std::map` uses to maintain order.
Here’s a simple example of a custom key:
struct CustomKey {
int id;
bool operator<(const CustomKey& other) const {
return id < other.id;
}
};
std::map<CustomKey, std::string> myCustomMap;
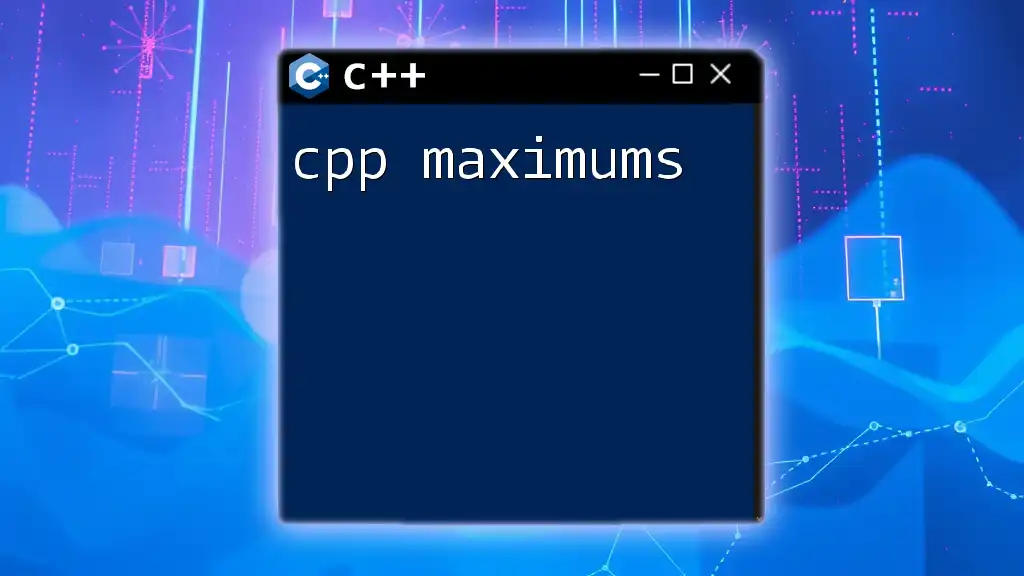
Common Use Cases for C++ Maps
Usage in Real-world Applications
C++ maps are versatile and find application in numerous areas. They are often used for storing configuration settings where unique parameters (keys) map to their respective values. Additionally, they can be invaluable in counting occurrences of elements, making frequency tables, or implementing lookup tables that require quick access times.
Performance Considerations
Choosing between a map and other data structures hinges on your specific needs. If retrieval time is a priority and you require ordered elements, `std::map` is often the appropriate choice. Familiarity with the time complexities of various operations will equip you to make optimal decisions based on the context of your programming tasks.
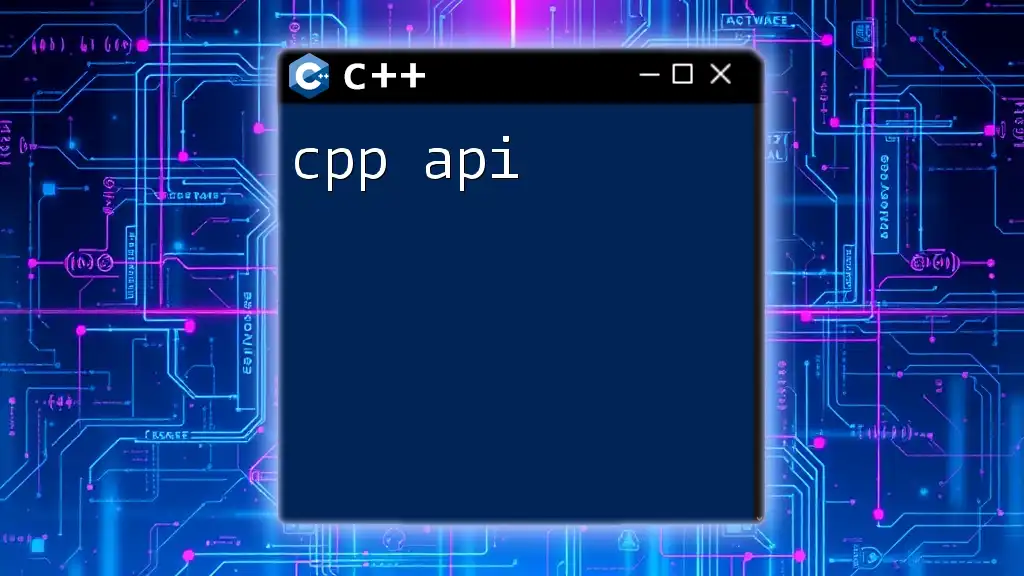
Conclusion
Recap of Key Points
In this comprehensive guide, you’ve learned about C++ maps, their features, types, usage, and nuances. Armed with this foundation, you can now explore maps further, apply them in your projects, and solve various programming challenges with confidence.
Further Learning Resources
To deepen your understanding, consider exploring recommended books, online courses, and the C++ documentation available through the Standard Library resources.
Call to Action
Now it’s your turn! Put your knowledge of C++ maps into practice. Tackle coding challenges that utilize maps, build simple projects, and gain hands-on experience to solidify your understanding. Happy coding!