The `std::pair` in C++ is a simple container that stores two heterogeneous values as a single unit, allowing for easy manipulation and retrieval of the stored values.
#include <iostream>
#include <utility> // for std::pair
int main() {
std::pair<int, std::string> myPair(1, "Hello");
std::cout << "First: " << myPair.first << ", Second: " << myPair.second << std::endl;
return 0;
}
Understanding C++ Pairs
What is a Pair?
A pair in C++ is a simple and efficient way to store two related values. Each pair can hold two values of potentially different data types. The concept of a pair is fundamental as it allows developers to group related items together, making coding more intuitive and organized.
Core Characteristics of Pairs
A pair is defined using the `std::pair` template from the C++ Standard Library. The two values in a pair are referred to as `first` and `second`. Pairs serve as a bridge between primitive data types and more complex structures, providing a lightweight solution when the use of full-fledged classes or structures seems unnecessary.
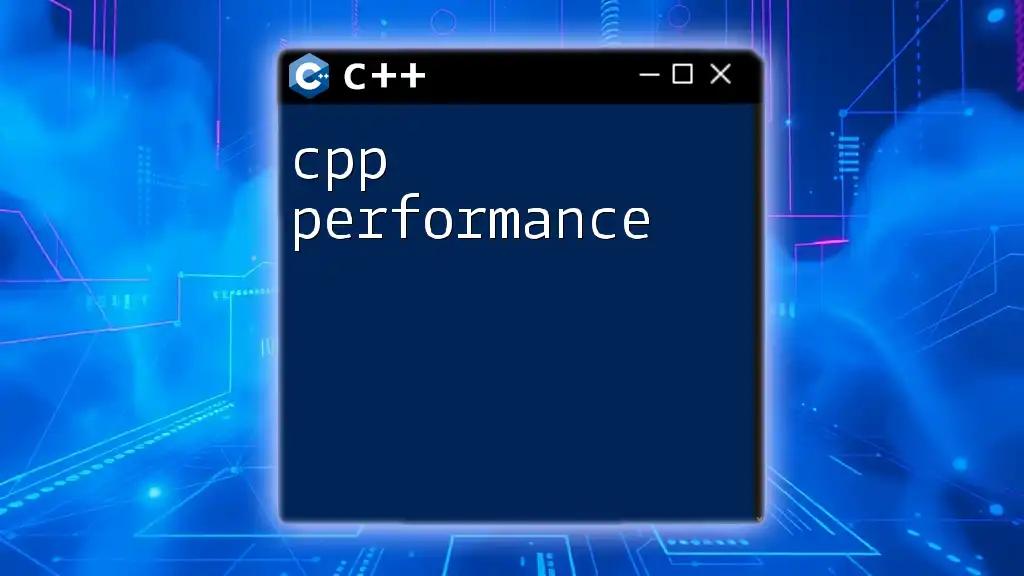
The Basics of C++ Pairs
How to Include the Pair Header
To utilize pairs in your C++ code, you must include the appropriate header file. The required header is found in the `<utility>` library. This library provides the definition for the `std::pair` class.
#include <utility>
Declaring a Pair
Declaring a pair is straightforward. You use the template `std::pair<Type1, Type2>` where `Type1` and `Type2` are the data types of the two values you want to store. For example, you can declare a pair that holds an integer and a string.
std::pair<int, std::string> myPair;
Initializing Pairs
Pairs can be initialized in several ways, offering flexibility based on your coding style or needs.
-
Using Constructors: You can initialize a pair directly during declaration.
std::pair<int, std::string> myPair1(1, "Hello");
-
Using `make_pair()`: This function simplifies the creation of pairs without explicitly specifying types.
auto myPair2 = std::make_pair(2, "World");
These methods provide you with the ability to succinctly create pairs, enhancing code readability and maintainability.
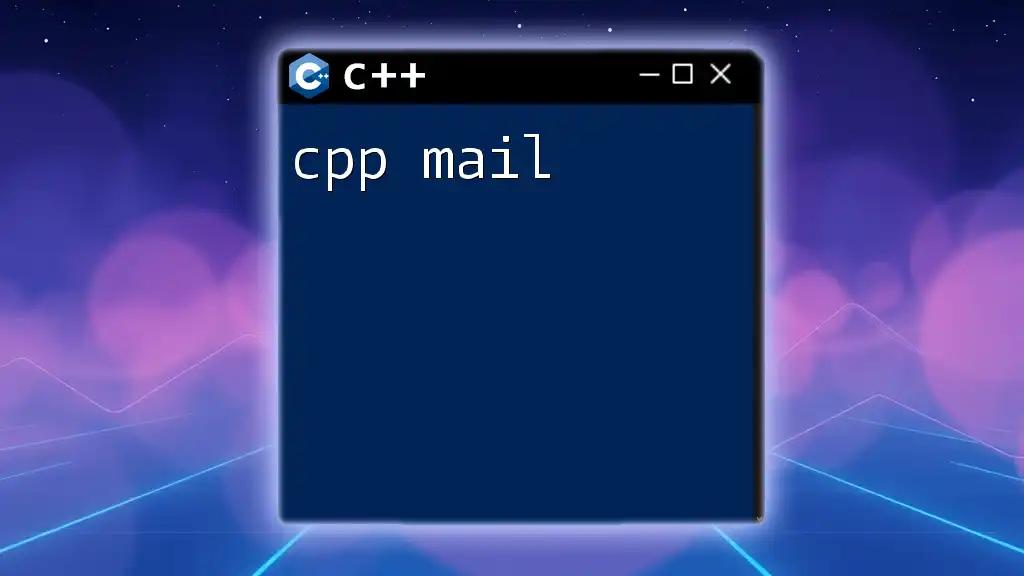
Working with C++ Pairs
Accessing Elements in a Pair
Once a pair is created, accessing its elements is simple. Pairs expose two public members: `first` and `second`. You can access and manipulate these elements directly.
int num = myPair1.first; // Accessing the first element
std::string str = myPair1.second; // Accessing the second element
Modifying Pair Elements
C++ pairs allow modification of their elements after initialization. The `first` and `second` members can be assigned new values at any time.
myPair1.first = 10;
myPair1.second = "Updated Hello";
This flexibility enables developers to adapt the contents of a pair as the program executes.
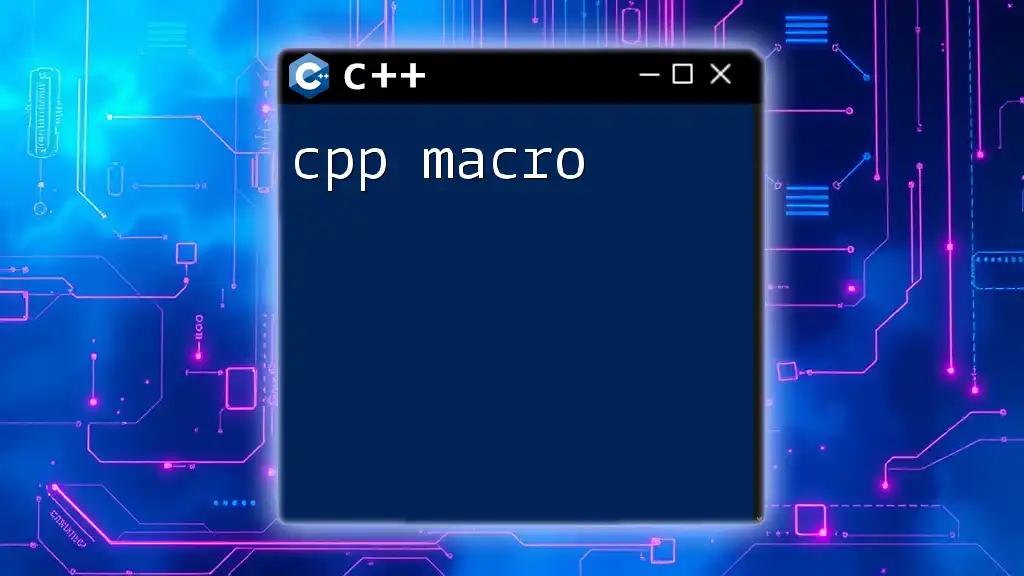
Pairs in Standard Template Library (STL)
Using Pairs in STL Containers
Pairs are versatile and often employed within STL containers. For instance, they can be stored in vectors or maps, providing a way to maintain key-value relationships.
std::vector<std::pair<int, std::string>> vec;
vec.push_back(std::make_pair(1, "One"));
This usage pattern allows you to create collections of pairs, which are particularly useful for managing sets of related data.
Sorting Pairs
Pairs can be manipulated using STL algorithms. When you store pairs in a vector, you can sort them based on the first element (or the second, depending on your criteria) with ease.
std::sort(vec.begin(), vec.end());
Using the `sort` function from the `<algorithm>` header allows you to quickly organize your pairs, which can be crucial for data processing tasks.
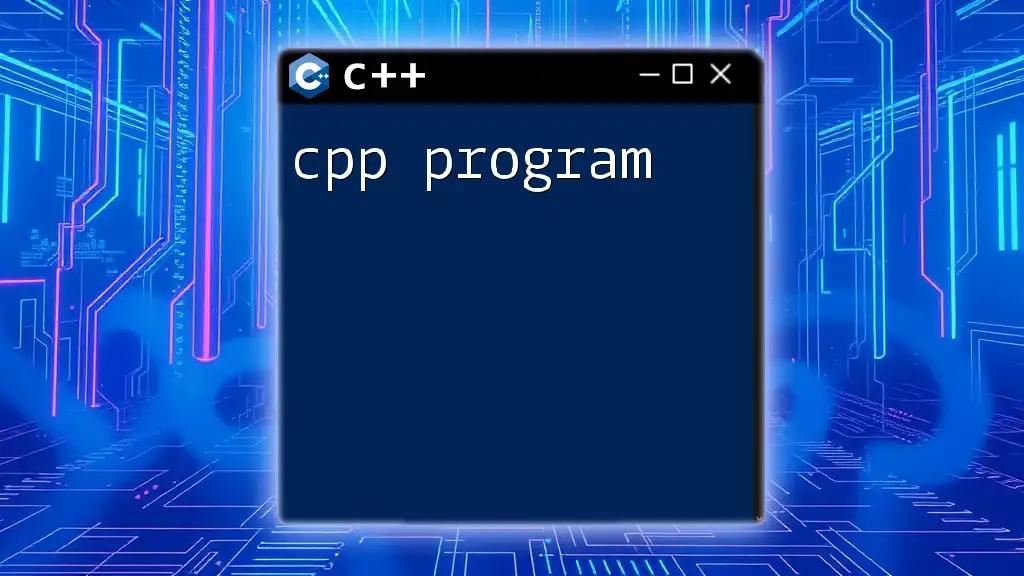
Advanced Pair Techniques
Pair Comparison
Comparing pairs directly is straightforward. The built-in comparison operators (`==`, `!=`, `<`, `>`, `<=`, `>=`) can be utilized to determine the relationship between two pairs based on their elements.
std::pair<int, int> pair1(1, 2);
std::pair<int, int> pair2(1, 3);
bool areEqual = (pair1 == pair2); // Compares pairs
This functionality is particularly useful in algorithms that require sorting or checking for duplicates.
Nested Pairs
Using pairs within pairs can enhance the ability to represent complex data structures in a manageable way. This is known as nesting.
std::pair<int, std::pair<int, int>> nestedPair(1, std::make_pair(2, 3));
Nested pairs allow you to encapsulate additional data, making them useful in certain problem-solving scenarios where relationships between multiple levels of data are required.
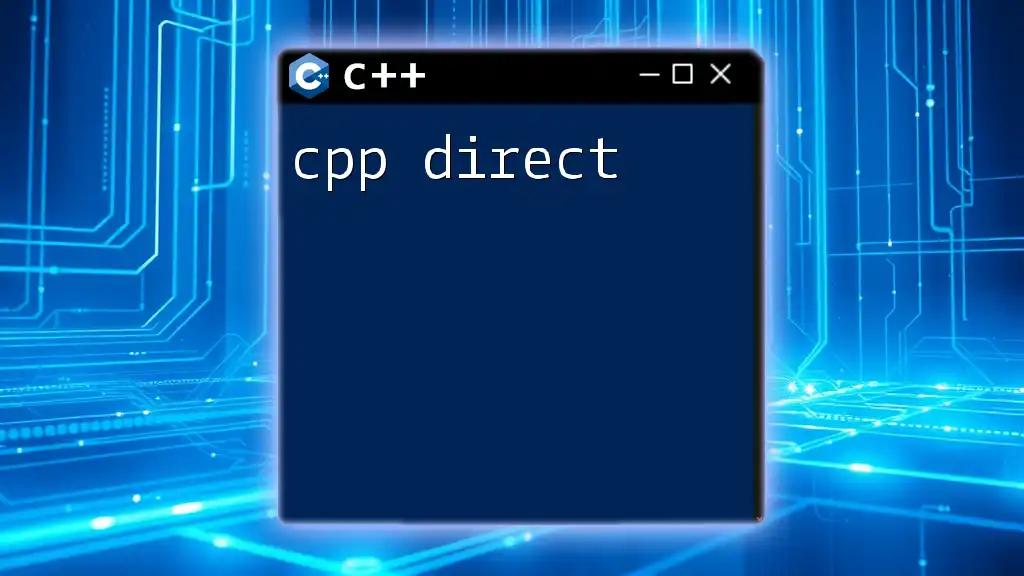
Real-World Use Cases of C++ Pairs
Key-Value Pairs in Maps
Pairs form the foundational element of maps in C++. Maps are associative containers that store elements in key-value pairs. Each key corresponds to a value, making data retrieval efficient.
std::map<std::string, int> ageMap;
ageMap["Alice"] = 30;
Maps leverage pairs' properties to provide a clear mapping of one piece of data to another, streamlining data management.
Pairing Data in Algorithms
In more complex algorithms, such as graph traversal or optimization methods like Dijkstra's Algorithm, pairs are often used to represent vertices and edge weights. This usage underscores the importance of pairs in programming, especially when handling relational data effectively.
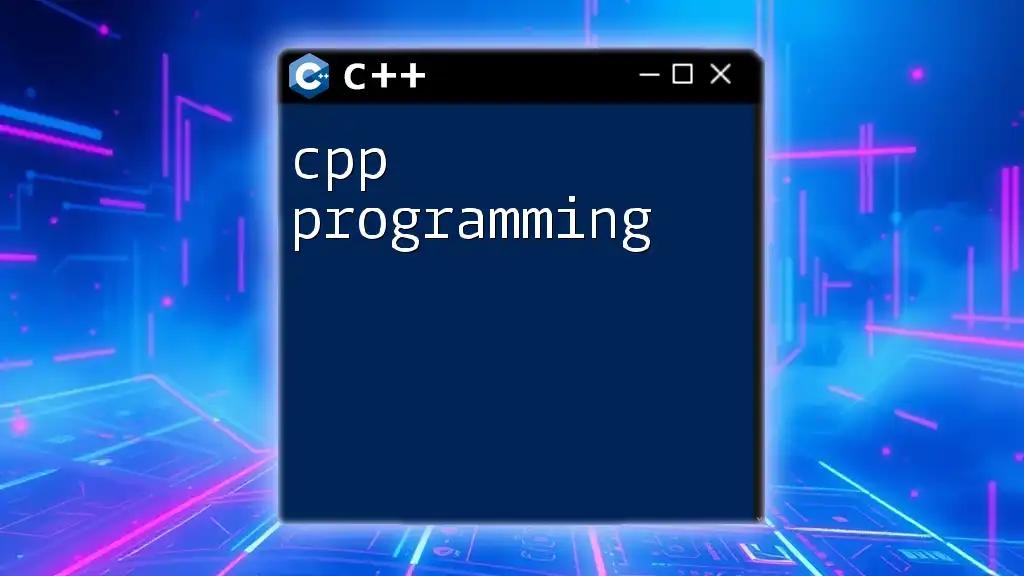
Best Practices for Using Pairs
When to Use Pairs
Utilize pairs when you need to group two related values succinctly. They are ideal for simple relationships and when creating complex data structures may be overkill. In scenarios where data is minimal and clarity is essential, prefer pairs over more intricate constructs.
Common Pitfalls to Avoid
While pairs are beneficial, they can lead to confusion if overused in highly complex scenarios. Be cautious with nested pairs; ensure their use adds clarity rather than complicating the code structure. Additionally, consider performance implications; pairs can sometimes induce overhead in large data processing tasks.
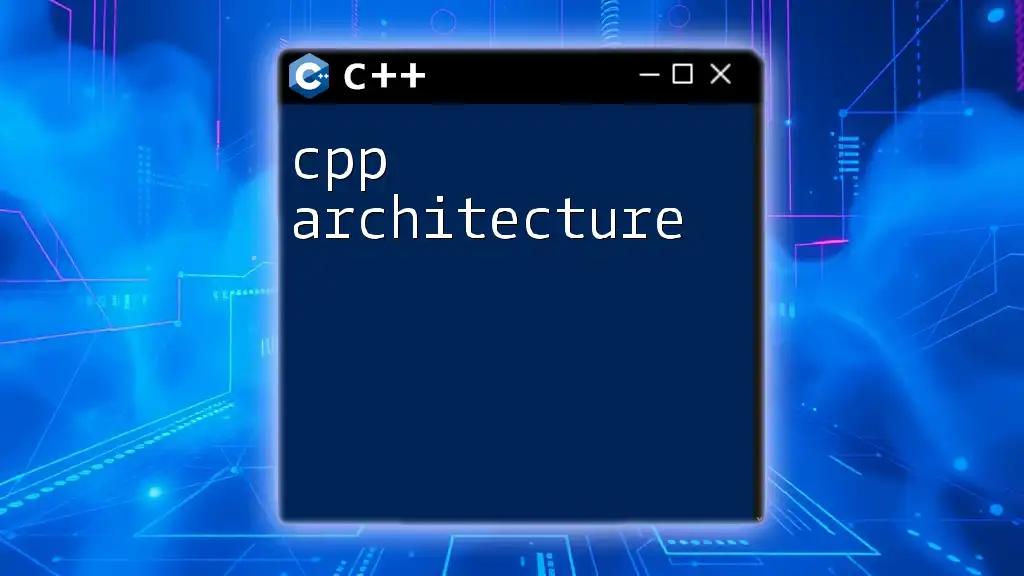
Conclusion
Recap of Key Points
C++ pairs represent a powerful yet simple tool for storing related data. Understanding their utility, from basic declaration to more advanced use cases, allows developers to enhance their programming efficiency and data management techniques.
Call to Action
Experiment with pairs in your C++ projects to gain familiarity with their capabilities. Start by using pairs in simple applications, then gradually incorporate them into more complex algorithms and data structures. Happy coding!