A C++ program is a set of instructions written in the C++ programming language that the computer can execute to perform specific tasks, such as the following example that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Introduction to C++ Programming
C++ is a powerful, high-level programming language that has shaped the landscape of software development since its creation in the early 1980s by Bjarne Stroustrup. As a language that supports object-oriented, imperative, and generic programming, C++ is widely recognized for providing a rich set of features that enable developers to create efficient and high-performance applications.
Learning C++ offers numerous benefits, including:
- Performance: C++ allows for system-level programming and is used in resource-critical applications such as game development and operating systems.
- Versatility: C++ is utilized in various industries, from finance to gaming, and is the backbone of many widely used applications and libraries.
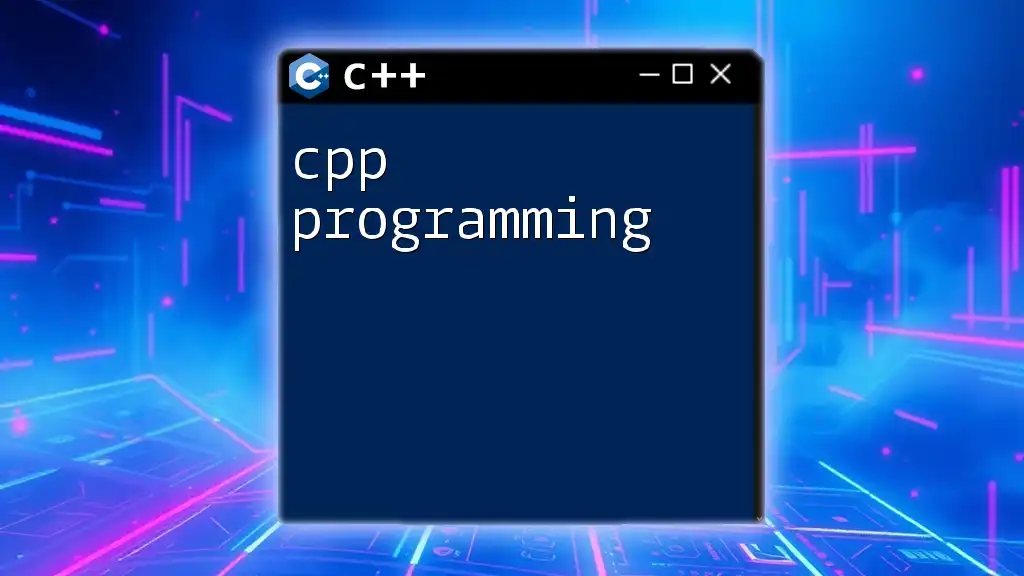
Setting Up Your C++ Development Environment
To begin your journey with a C++ program, it's essential to set up a suitable development environment.
Choosing the Right Compiler
A compiler converts your C++ code into an executable program. Some popular C++ compilers include:
- GCC (GNU Compiler Collection)
- Clang
- MSVC (Microsoft Visual C++)
To install GCC on a Unix-based system, you may use the package manager:
sudo apt install g++
Installing an Integrated Development Environment (IDE)
While you can use a simple text editor, an Integrated Development Environment (IDE) enhances your productivity with features like code completion, debugging tools, and syntax highlighting. Recommended IDEs for C++ programming include:
- Visual Studio
- Code::Blocks
- Eclipse
To install Code::Blocks, follow these steps:
- Download the installer from the official website.
- Run the installer and follow the instructions to complete the installation.
Compiling Your First C++ Program
Now that you have an IDE or text editor set up, let’s create your first C++ program.
Here’s a simple "Hello, World!" example in C++:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
To compile this program, use the command line:
g++ hello.cpp -o hello
./hello
This will display "Hello, World!" in your terminal.
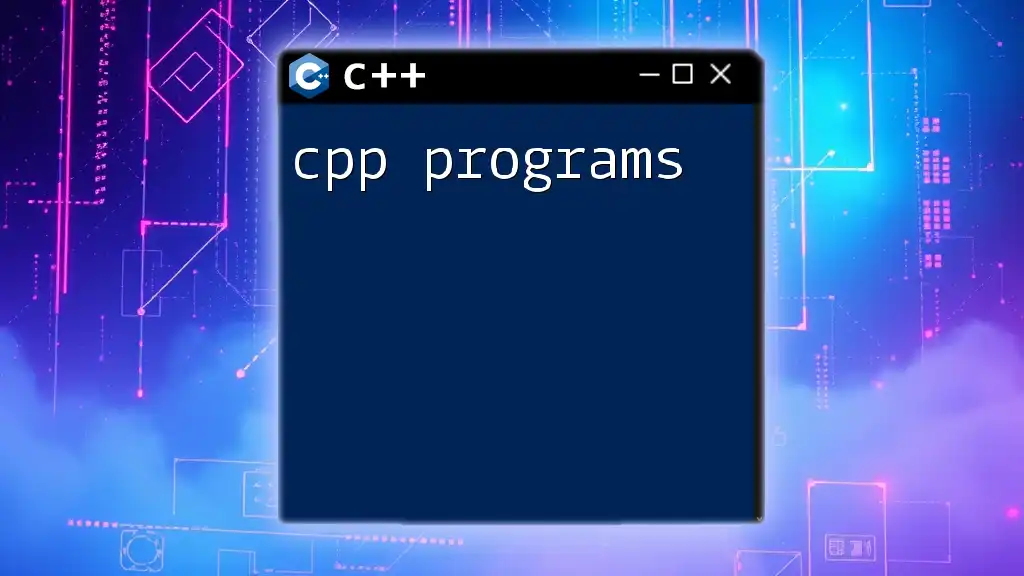
Understanding C++ Syntax and Structure
Every C++ program consists of a basic structure that must be followed.
Basic Structure of a C++ Program
A typical C++ program contains the following components:
- Headers: Imported libraries are included at the top, such as `<iostream>`, which is necessary for input and output operations.
- Namespaces: Use of the `using namespace std;` statement allows direct access to the standard library without prefixing with `std::`.
- Main Function: The entry point of every C++ program is the `main` function.
Here is the structure annotated:
#include <iostream> // Header
using namespace std; // Namespace
int main() { // Main function
// Your code goes here
return 0; // Exit status
}
Data Types in C++
C++ offers a variety of data types, allowing developers to define the type of data that variables can hold. Basic data types include:
- int: For integers.
- char: For characters.
- float: For floating-point numbers.
- double: For double-precision floating-point numbers.
Additionally, C++ allows you to create user-defined data types using `struct` and `class`, enabling complex data structures that model real-world entities.
Variables and Constants
Variables in C++ are created by declaring with a specific data type:
int age = 30;
const int MAX_VALUE = 100; // Constant
Using `const` or `#define`, you can employ constants that prevent accidental modification throughout your program.
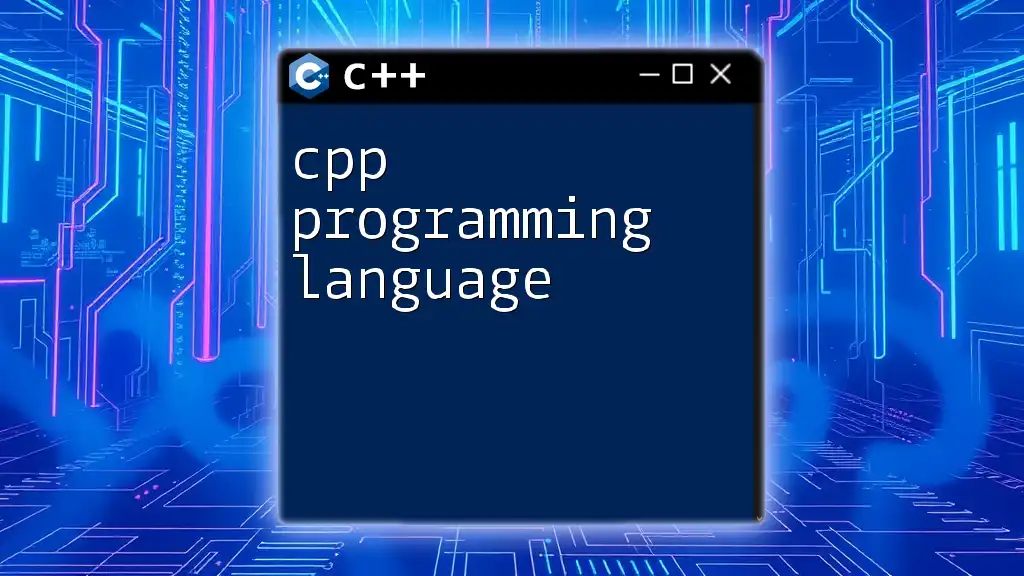
Control Structures in C++
Control structures govern the flow of your C++ program.
Conditional Statements
C++ provides several conditional statements that determine which block of code to execute based on specified conditions.
if Statement
With an `if statement`, you can execute code based on true/false conditions:
if (age >= 18) {
cout << "Adult" << endl;
} else {
cout << "Minor" << endl;
}
switch Statement
The `switch statement` offers an alternative to `if` for multiple conditions:
switch (day) {
case 1:
cout << "Monday" << endl;
break;
case 2:
cout << "Tuesday" << endl;
break;
default:
cout << "Invalid day" << endl;
}
Loops
Loops allow you to execute a block of code multiple times based on conditions.
for Loop
The `for loop` is commonly used when the number of iterations is known:
for (int i = 0; i < 10; i++) {
cout << i << endl; // Prints numbers 0-9
}
while Loop
The `while loop` continues as long as a specified condition is true:
while (condition) {
// Code to execute as long as condition is true
}
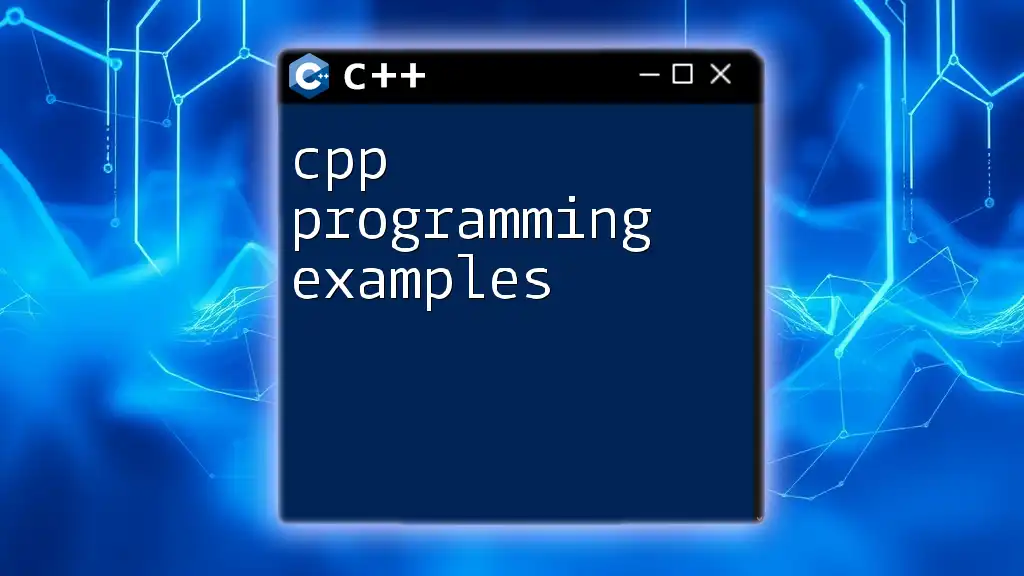
Functions in C++
Functions in C++ enable modular and reusable code design, allowing you to define operations that can be called multiple times.
Defining and Calling Functions
You define a function's return type, name, and parameters:
int add(int a, int b) {
return a + b;
}
Function Overloading
Function overloading lets you create multiple functions with the same name but different parameters:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
This is particularly useful for performing similar operations on different data types.
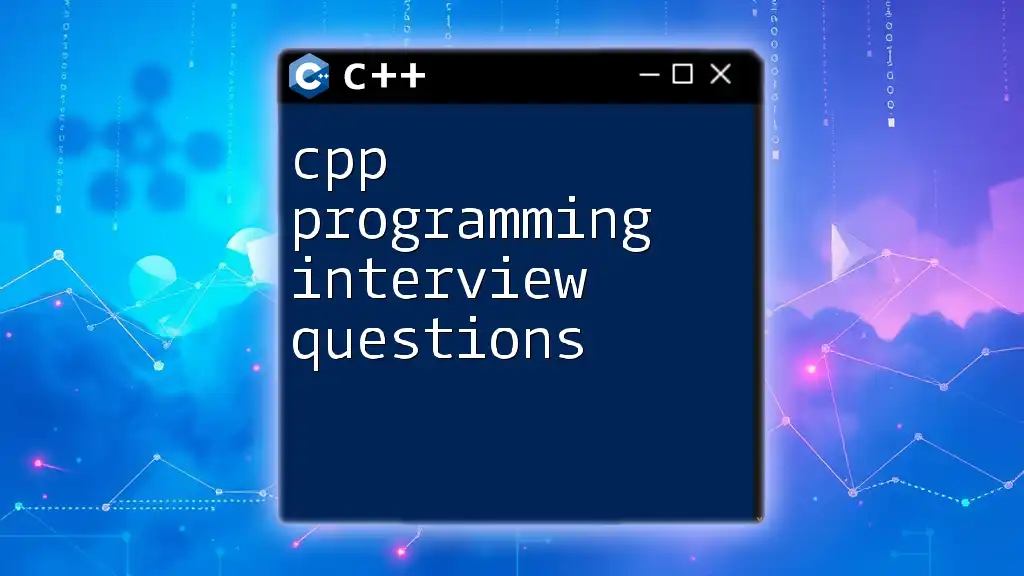
Object-Oriented Programming in C++
C++ fundamentally supports Object-Oriented Programming (OOP), which models real-world entities using classes and objects.
Introduction to OOP Concepts
OOP concepts include:
- Encapsulation: Bundling the data and methods that operate on that data together.
- Inheritance: Creating new classes from existing ones.
- Polymorphism: Allowing classes to be defined with shared interfaces.
Creating and Using Classes
A class in C++ defines a blueprint for objects.
class Circle {
public:
float radius;
float area() {
return 3.14 * radius * radius;
}
};
You can create an object of the `Circle` class and use its methods.
Inheritance and Polymorphism
C++ supports inheritance, allowing one class to inherit properties from another:
class Shape {
public:
virtual void draw() { }
};
class Circle : public Shape {
public:
void draw() override { /* Draw Circle */ }
};
This example demonstrates how shape characteristics can be inherited and specialized.
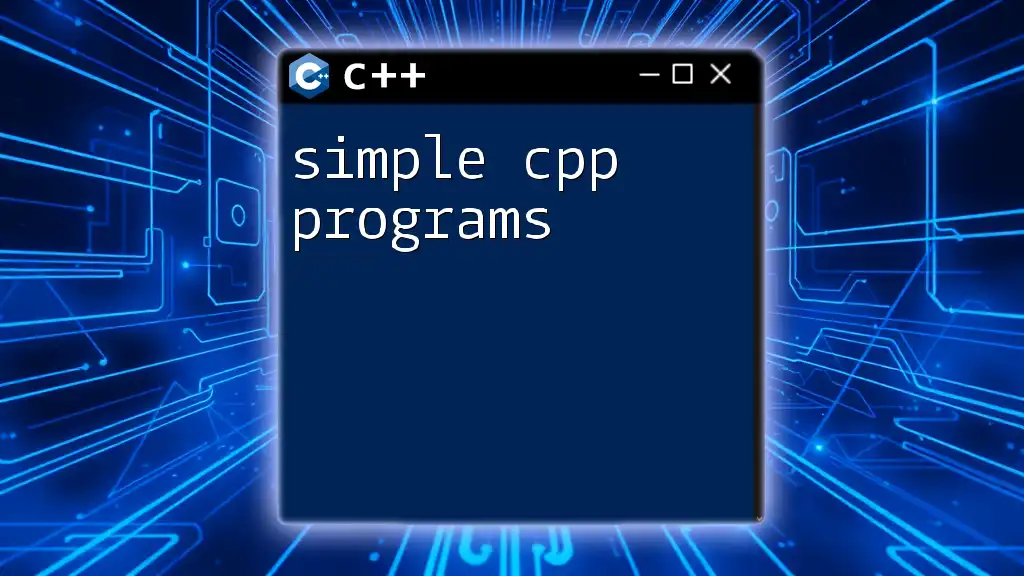
Working with Libraries and Standard Template Library (STL)
Leveraging libraries and the Standard Template Library (STL) can enhance your development efficiency.
Using Standard Libraries
C++ includes numerous standard libraries that facilitate various operations. Common libraries such as `<vector>`, `<map>`, and `<algorithm>` provide built-in functionalities that can save time.
Introduction to STL Containers
STL encompasses several data containers:
#include <vector>
std::vector<int> numbers;
numbers.push_back(10); // Adding element
The vector container dynamically resizes itself to accommodate fluctuations in data size.
Algorithms in STL
STL also incorporates numerous algorithms, such as `sort` or `find`, to manipulate and search through data efficiently.
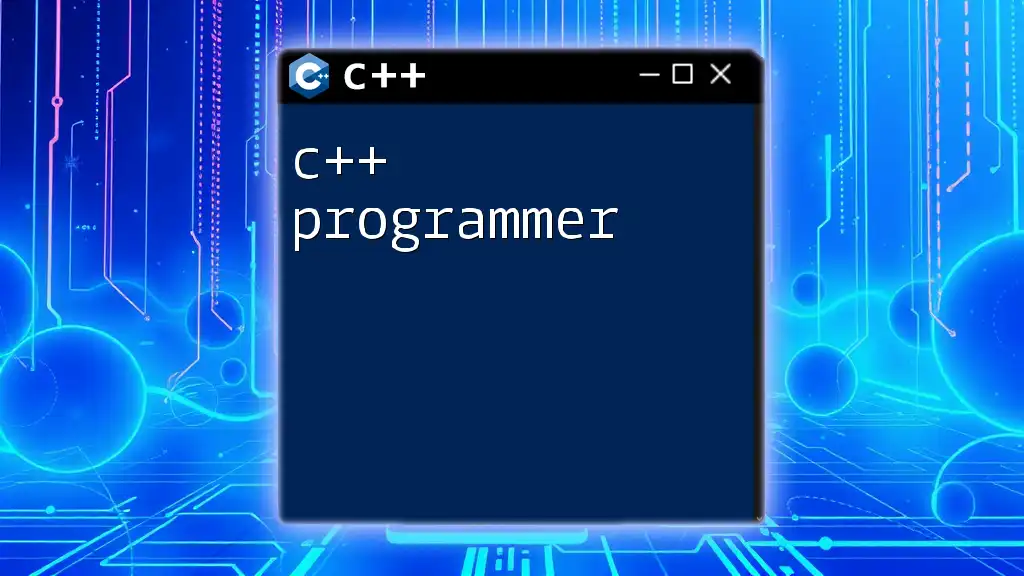
Debugging and Error Handling
Debugging is an essential skill in programming. Understanding common errors can streamline this process.
Common C++ Errors
Errors in C++ can be categorized into:
- Compilation Errors: Issues that the compiler identifies before the program runs.
- Runtime Errors: Problems that occur while the program is executing.
Using Debugging Tools
You can use tools like GDB for debugging in terminal or utilize debugging features in IDEs such as breakpoints and variable inspection.
Error Handling with Exceptions
Error handling is crucial to managing unexpected behaviors. C++ provides exception handling using `try` and `catch` blocks:
try {
// Code that may throw an exception
} catch (exception &e) {
cout << e.what() << endl; // Handle exception
}
This allows more robust code that gracefully handles potential errors.
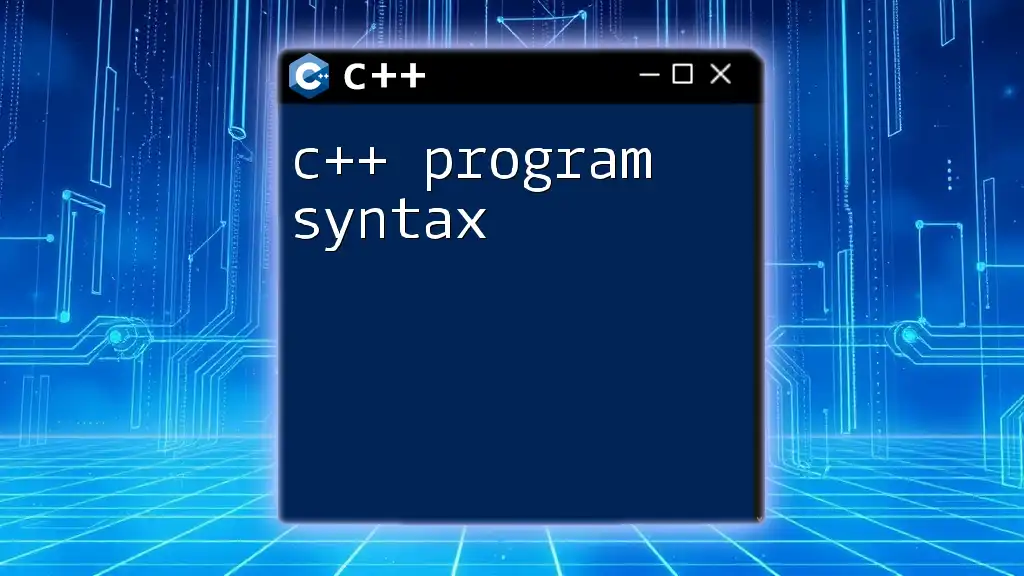
Best Practices in C++ Programming
Adhering to best practices can enhance your programming efficiency and code maintainability.
Code Readability
Maintainability is critical; thus, focus on:
- Write meaningful comments.
- Choose clear and descriptive names for variables and functions.
Memory Management
C++ requires explicit memory management:
int* ptr = new int; // Dynamic memory allocation
delete ptr; // Freeing allocated memory
It's essential to avoid memory leaks by pairing every `new` with a `delete`.
Version Control Basics
Utilizing Git for version control is advantageous for managing code changes, collaboration, and maintaining project history.
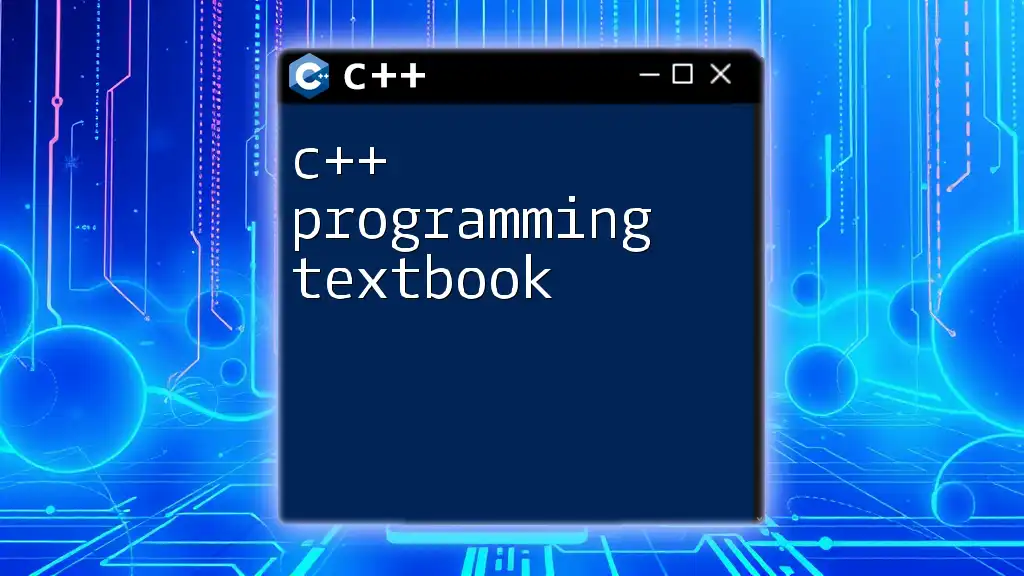
Conclusion
Learning C++ and building C++ programs opens an array of opportunities in software development. By mastering the principles outlined, you’ll be well-equipped to tackle complex programming challenges. Continuous practice and exploring additional resources will further enhance your skills. As you embark on your C++ programming journey, don’t hesitate to reach out for help or just to share your thoughts!