Simple C++ programs are basic scripts that demonstrate fundamental programming concepts, such as input/output operations and control structures.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Getting Started with C++
Setting Up Your Environment
Before diving into simple C++ programs, it's essential to have the right environment set up. You’ll need an Integrated Development Environment (IDE) or a compiler to write and execute your code. Popular choices include:
- Visual Studio: A powerful IDE with extensive support for C++.
- Code::Blocks: A user-friendly option for beginners.
- g++ on Linux: A command-line compiler that’s great for those who prefer a minimalist approach.
Follow the installation instructions specific to your selected tool to ensure a smooth setup.
Basic Syntax Overview
C++ has a specific syntax that you need to familiarize yourself with. Here's a quick overview of the critical components of a C++ program:
- Structure of a C++ Program: Most C++ programs start with `#include` directives, followed by the `main()` function where execution begins.
- Data Types: C++ supports various data types, including `int`, `float`, `char`, and `string`. Understanding these will allow you to define variables effectively.
- Variables and Operators: C++ uses variables to store values and operators for performing operations on those values. For example:
- Arithmetic operators: `+`, `-`, `*`, `/`.
- Comparison operators: `==`, `!=`, `<`, `>=`.
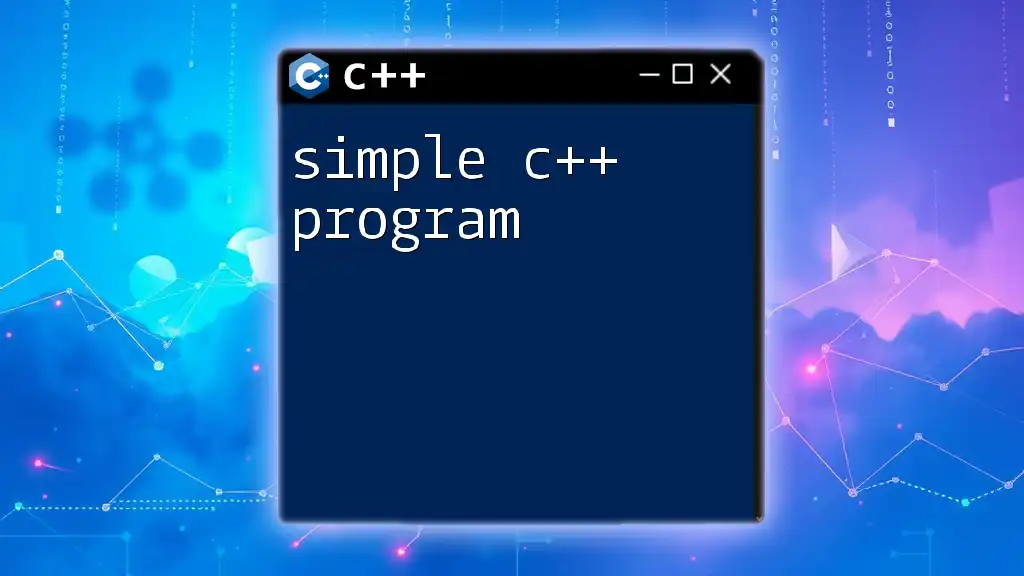
Writing Your First Simple C++ Program
Hello World Example
Let's start with the classic "Hello World" program, the most basic illustration of how to output text to the console.
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this example:
- The line `#include <iostream>` tells the compiler to include the standard input/output stream library, which is essential for output via `cout`.
- The `main()` function is where the program begins to execute.
- `cout` is used to print "Hello, World!" to the console, followed by `endl`, which moves the cursor to a new line.
This simple program demonstrates the basic syntax and structure of a C++ application.
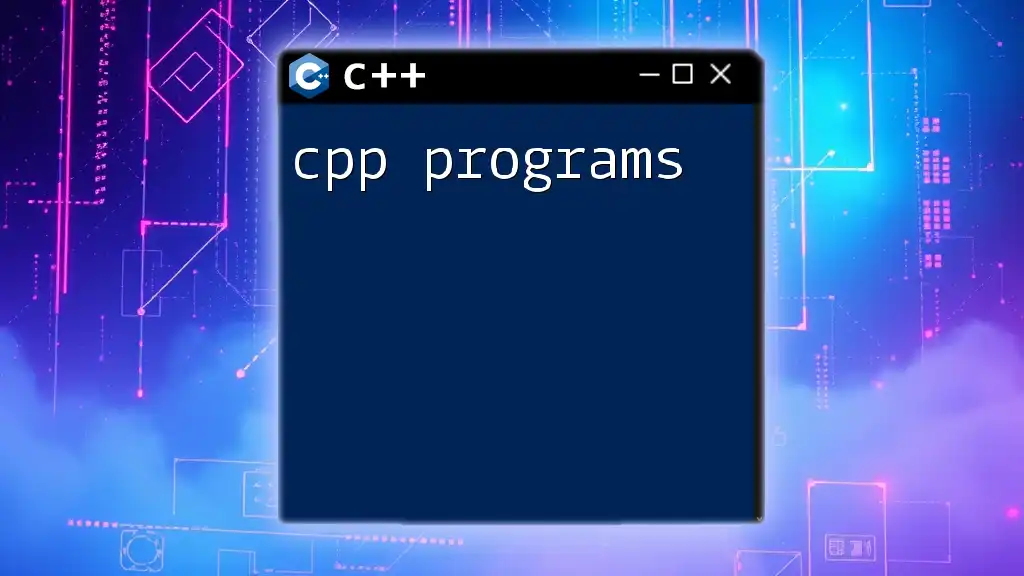
Control Structures in C++
Conditional Statements
Conditional statements are vital when you need to execute different actions based on varying conditions. One straightforward implementation is the if-else statement.
int num;
cout << "Enter a number: ";
cin >> num;
if (num > 0) {
cout << "Positive number" << endl;
} else if (num < 0) {
cout << "Negative number" << endl;
} else {
cout << "Zero" << endl;
}
In this code:
- The user inputs a number, which is stored in the variable `num`.
- Depending on the value of `num`, the program outputs whether it's positive, negative, or zero, demonstrating how to use logical conditions effectively.
Loops
Loops allow repeated execution of a block of code, which can be useful for tasks like counting or iterating through data.
For Loop Example
A common loop is the for loop, which is used when the number of iterations is known.
for (int i = 1; i <= 5; i++) {
cout << "Iteration: " << i << endl;
}
This loop prints a message five times, demonstrating control flow and iteration.
While Loop Example
The while loop is useful when the number of iterations is not predetermined.
int j = 1;
while (j <= 5) {
cout << "Iteration: " << j << endl;
j++;
}
This loop continues as long as the condition `j <= 5` holds true, showcasing another method for executing repeated tasks.
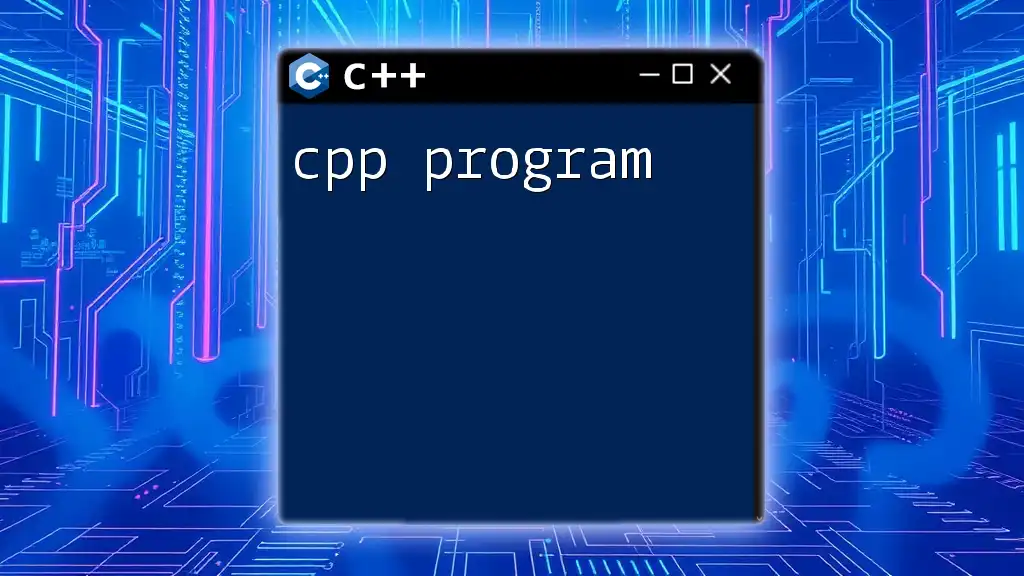
Functions in C++
Functions play a crucial role in organizing and reusing code, enhancing readability and maintainability.
Creating and Using Functions
Here’s a simple function that adds two integers:
int add(int a, int b) {
return a + b;
}
int main() {
cout << "Sum: " << add(5, 3) << endl;
return 0;
}
In this example:
- The function `add` takes two integer parameters and returns their sum.
- Within `main()`, the function is called, and its result is printed. This exemplifies the power of functions for encapsulating behavior and performing specific tasks.
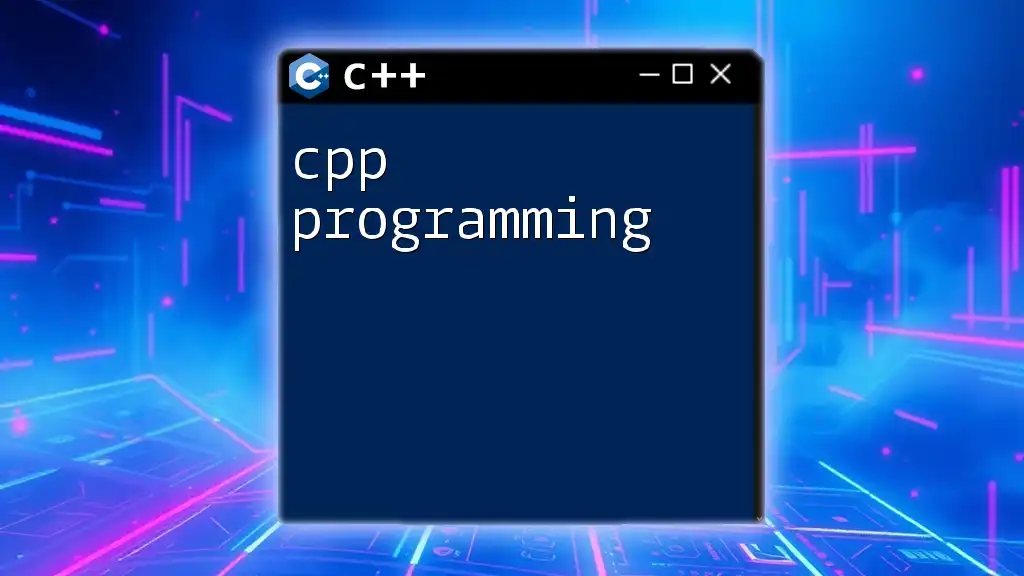
Basic Input/Output in C++
Using cin and cout
Input and output are fundamental operations in C++. This section introduces how to interact with users through the console.
Interactive User Input Example
Here is how to take user input and provide output:
string name;
cout << "Enter your name: ";
cin >> name;
cout << "Hello, " << name << "!" << endl;
In this code:
- The program prompts the user to enter their name, stored in the variable `name`.
- It then greets the user using the input received, demonstrating basic interaction through the console.
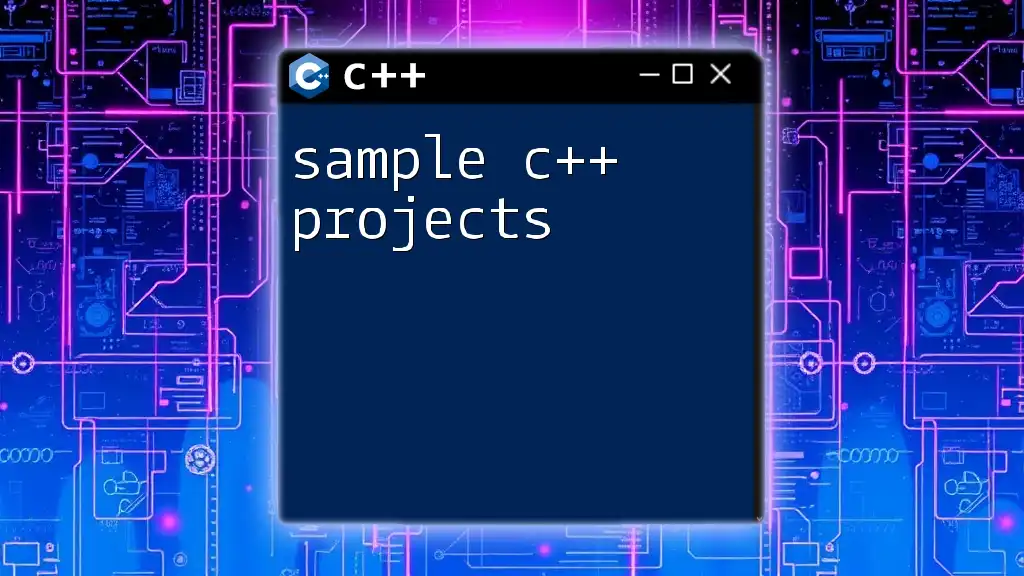
Simple Data Structures in C++
Understanding Arrays
Arrays are contiguous memory locations that store multiple values of the same type. They are essential for managing collections of data.
Array Example
Here’s how to declare and access an array:
int arr[5] = {1, 2, 3, 4, 5};
for (int i = 0; i < 5; i++) {
cout << arr[i] << " ";
}
cout << endl;
In this example:
- An integer array `arr` is defined and initialized with five values.
- A loop is used to iterate through the array and print each value, illustrating how to work with data structures in C++.
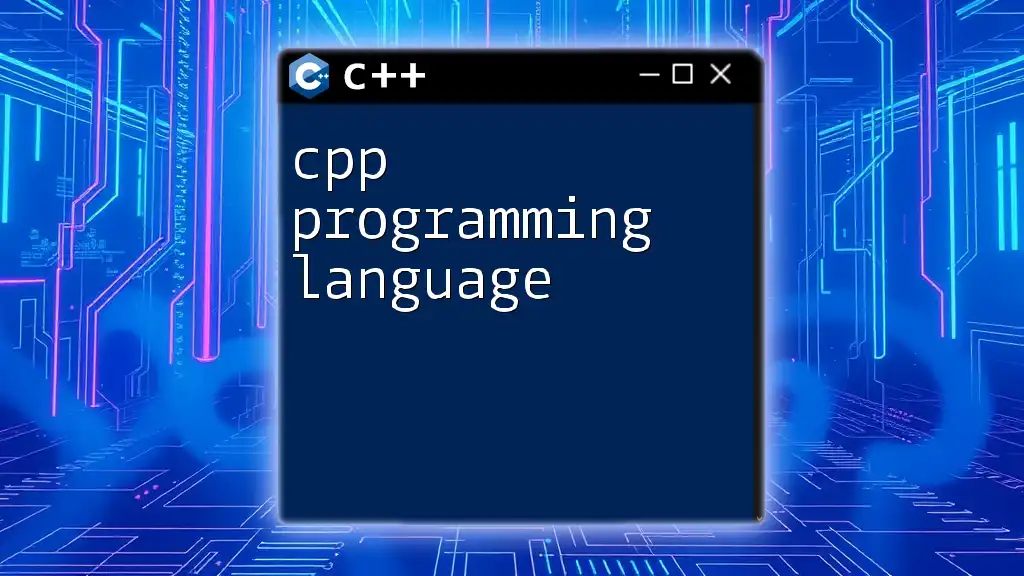
Conclusion
In summary, mastering simple C++ programs is a critical step toward becoming proficient in coding. By starting with basic concepts such as output, control structures, functions, input/output handling, and basic data structures, you lay the groundwork for tackling more advanced programming challenges.
As you practice these concepts, challenge yourself with additional simple programs like a calculator or a number guessing game. The journey of learning C++ is exciting, and every simple program you write brings you one step closer to becoming an expert programmer.
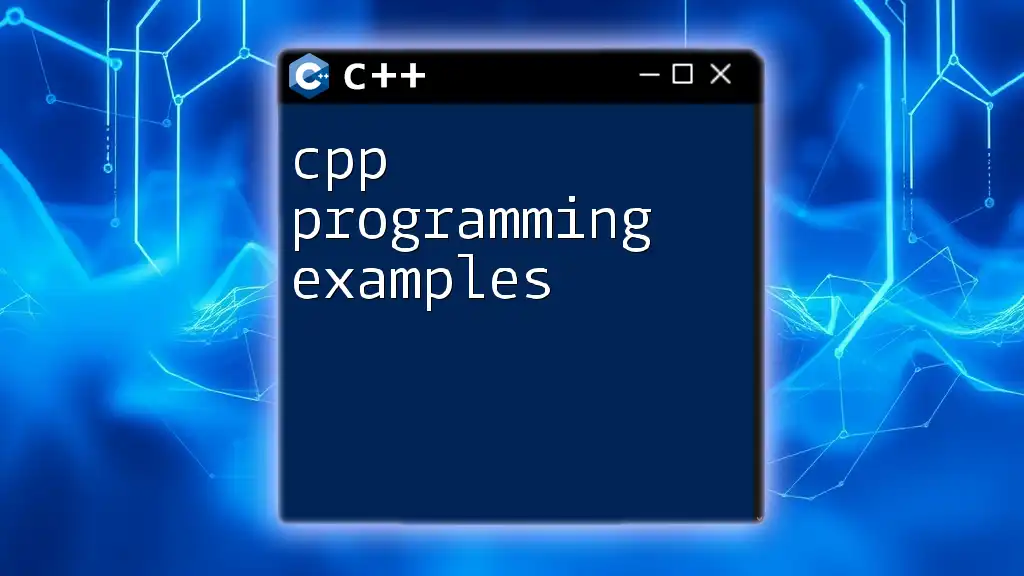
Additional Resources
To further your understanding and skills, consider exploring these resources:
- Tutorials and documentation available online to deepen your knowledge.
- Recommended books that cater to beginners and seasoned programmers alike.
- Programming communities online to engage with others, share your simple C++ programs, and seek help as needed.
With consistent practice and exploration, your proficiency in C++ will undoubtedly flourish!