C++ programs are collections of instructions written in the C++ programming language that are executed to perform specific tasks or computations.
Here's an example of a simple C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Basics
What is C++?
C++ is a powerful, high-level programming language that was developed by Bjarne Stroustrup in the early 1980s as an extension of the C language. It provides features that support object-oriented programming, which allows for better code organization and reuse. C++ has evolved over the years into a widely-used language in various domains, including systems programming, game development, and application software.
One significant aspect of C++ is its performance. It is close to the hardware, allowing for fine-tuned optimizations, which makes it a preferred choice for performance-critical applications.
Setting Up the C++ Environment
To begin writing C++ programs, a proper development environment is essential. Here’s how you can set it up:
-
Choosing an IDE: Some popular Integrated Development Environments (IDEs) for C++ include:
- Visual Studio: A comprehensive IDE that provides tools for building Windows applications.
- Code::Blocks: Ideal for beginners and those looking for a lightweight option.
- Eclipse: A versatile IDE that can be configured for C++.
-
Installation Steps: Each IDE has its own installation process. Generally, you would download the installer, follow the setup instructions, and customize the installation as needed for your requirements.
-
Compiling & Running a C++ Program: After setting up your IDE, write your first C++ program and observe how to compile and run it. Compiling translates your code into machine language, while running executes the program.
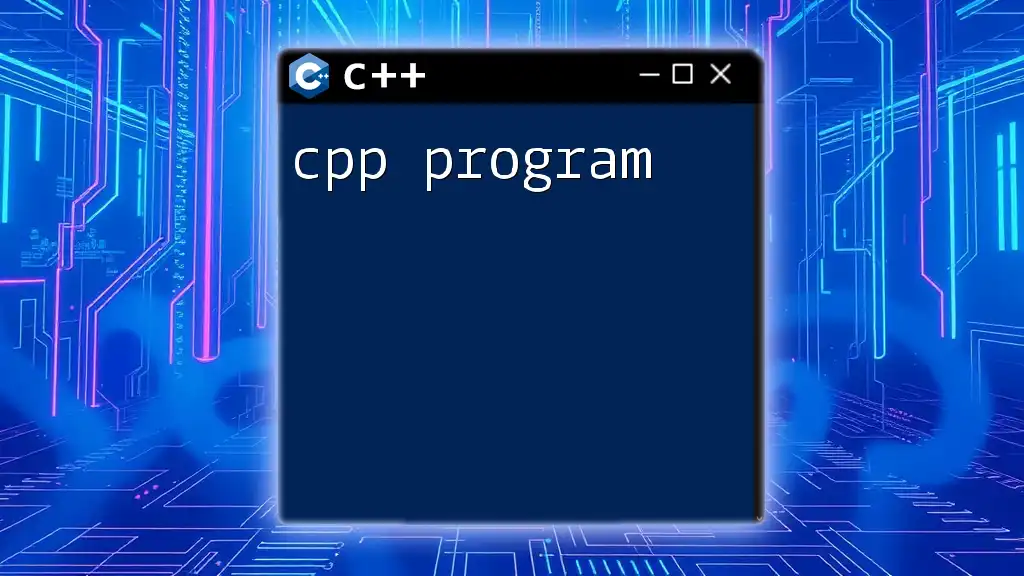
Anatomy of a C++ Program
Basic Structure
A C++ program consists of various components that work together to perform tasks effectively:
- Headers: These are files that provide definitions of functions and macros. For example, using `#include <iostream>` allows you to use input and output functions from the standard library.
- Main Function: This is the entry point of every C++ program. The syntax looks like this:
int main() {
// code goes here
return 0;
}
- Statements: Statements are individual instructions that tell the program what to execute. Each statement must end with a semicolon.
Example: Hello World Program
The quintessential example of a C++ program is the “Hello World” application. Here’s a simple implementation:
#include <iostream>
using namespace std;
int main() {
cout << "Hello World!" << endl;
return 0;
}
In this code snippet:
- `#include <iostream>` imports the I/O stream library for console output.
- `using namespace std;` allows us to use standard library names without prefixing them with `std::`.
- `cout` is used to print text to the console, while `endl` adds a new line.
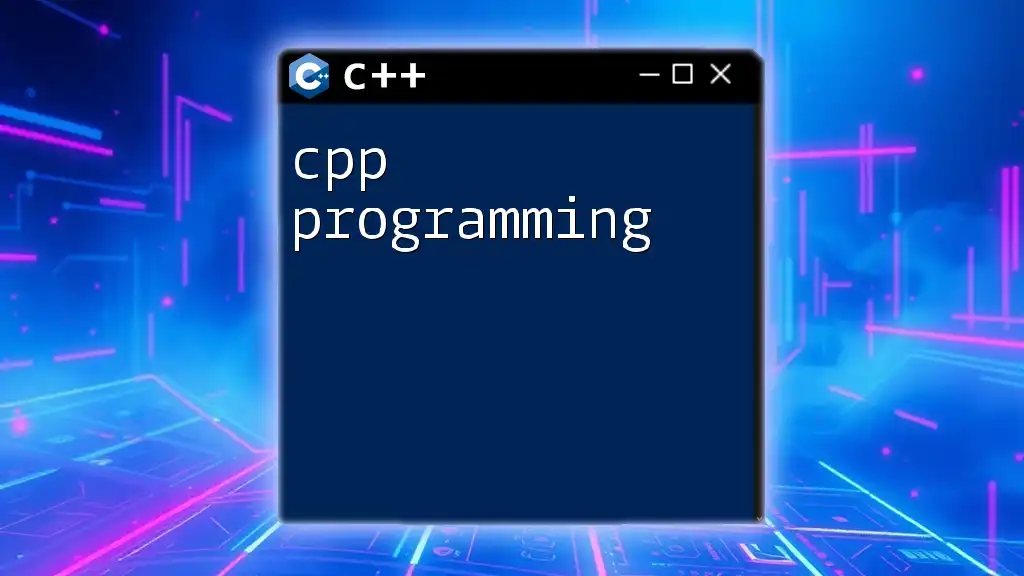
Core Concepts of C++ Programming
Data Types in C++
C++ offers several built-in data types:
-
Primitive Types: These include `int` for integers, `float` for floating-point numbers, and `char` for characters. Understanding data types is crucial as they determine how much memory is allocated and what kind of operations can be performed.
-
Derived Types: Arrays and functions fall into this category. Arrays store a collection of items of the same type, while functions encapsulate reusable logic.
-
User-defined Types: These include structures, classes, and enumerations, providing more complex data modeling capabilities.
Variables and Constants
Declaring a variable in C++ is straightforward. You must specify the type followed by the variable name:
int age = 25;
const float pi = 3.14;
In this example, `age` is a variable, while `pi` is a constant. Understanding scope and lifetime is crucial; variables defined within a function are local and cease to exist once the function execution ends.
Operators in C++
Operators in C++ are symbols that perform operations on variables and values. Here’s a quick overview:
- Arithmetic Operators: Include addition (`+`), subtraction (`-`), multiplication (`*`), and division (`/`).
- Comparison Operators: Such as equal to (`==`), not equal (`!=`), greater than (`>`), and less than (`<`).
- Logical Operators: Include AND (`&&`), OR (`||`), and NOT (`!`).
Control Structures
Conditional Statements
Control flow in C++ often relies on conditional statements, which allow the program to make decisions:
- If-Else Statements: Control the flow of the program based on conditions. For example:
if (age >= 18) {
cout << "Adult" << endl;
}
else {
cout << "Minor" << endl;
}
- Switch Cases: Useful for selecting among multiple options based on a variable’s value.
Loops
Loops help iterate over a block of code multiple times:
- For Loop: Effective for counter-controlled loops:
for (int i = 0; i < 5; i++) {
cout << i << endl;
}
- While Loop: Repeats as long as a specified condition is true:
int i = 0;
while (i < 5) {
cout << i << endl;
i++;
}
- Do-While Loop: Similar to the `while` loop but guarantees at least one iteration.
int i = 0;
do {
cout << i << endl;
i++;
} while (i < 5);
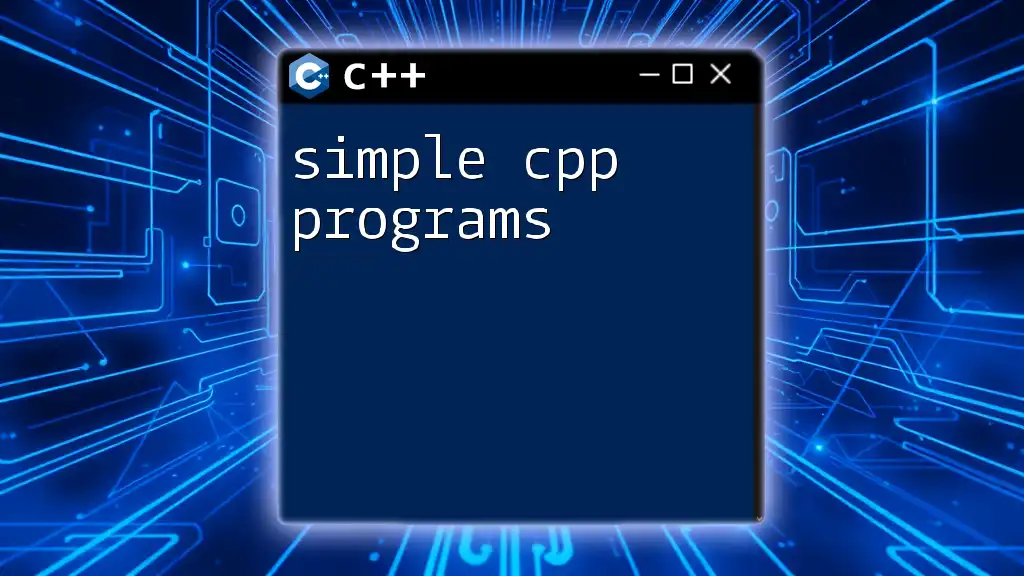
Functions in C++
Understanding Functions
Functions are blocks of code that perform a specific task. They promote code reusability and modular design. The syntax for defining a function is as follows:
return_type function_name(parameters) {
// Code to execute
}
For example, a simple function could look like this:
void greet() {
cout << "Hello, User!" << endl;
}
Function Overloading
C++ supports function overloading, allowing multiple functions with the same name yet different parameters. This feature enhances functionality without altering an existing function's name.
void display(int n) {
cout << n << endl;
}
void display(string str) {
cout << str << endl;
}
Inline Functions
Inline functions are those that are expanded in line when they are invoked, rather than being called through traditional function calls. This can increase performance by reducing function call overhead.
inline int square(int x) {
return x * x;
}
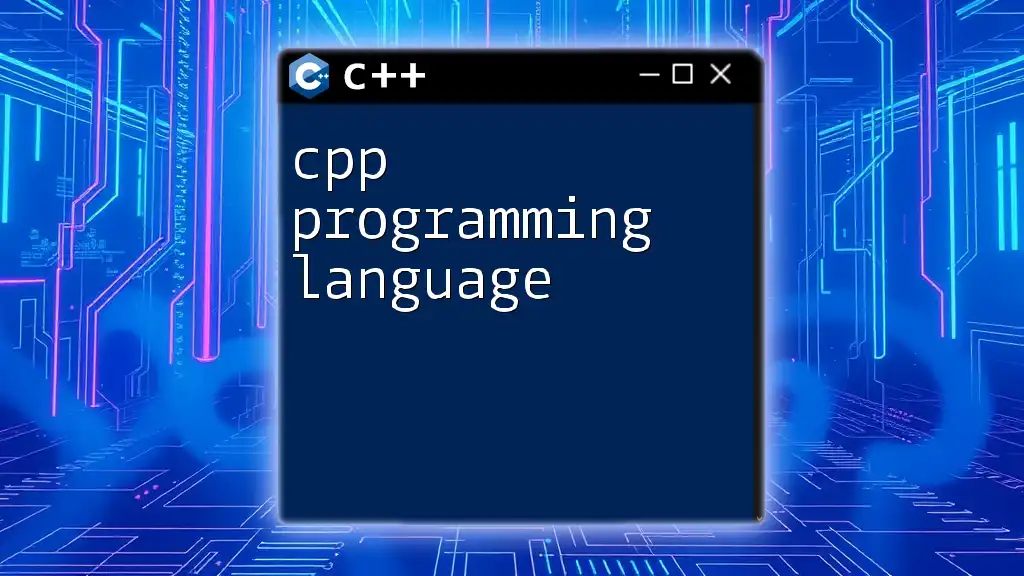
Object-Oriented Programming in C++
Introduction to OOP
C++ is designed with Object-Oriented Programming (OOP) principles in mind. The main principles include:
- Encapsulation: Restricting access to certain properties and methods from outside interference and misuse.
- Inheritance: The ability to inherit properties and methods from existing classes, allowing for code reusability.
- Polymorphism: The ability of different classes to be treated as instances of the same class through a common interface.
Classes and Objects
A class is a blueprint for creating objects. Here’s how to define a class in C++:
class Car {
public:
string brand;
void honk() {
cout << "Honk! Honk!" << endl;
}
};
Objects can be created from classes:
Car myCar;
myCar.brand = "Toyota";
myCar.honk();
Constructors and Destructors
Constructors are special member functions invoked when a class instance is created. Destructors are invoked when an object goes out of scope, managing resource cleanup.
class Car {
public:
Car() {
cout << "Car created!" << endl;
}
~Car() {
cout << "Car destroyed!" << endl;
}
};
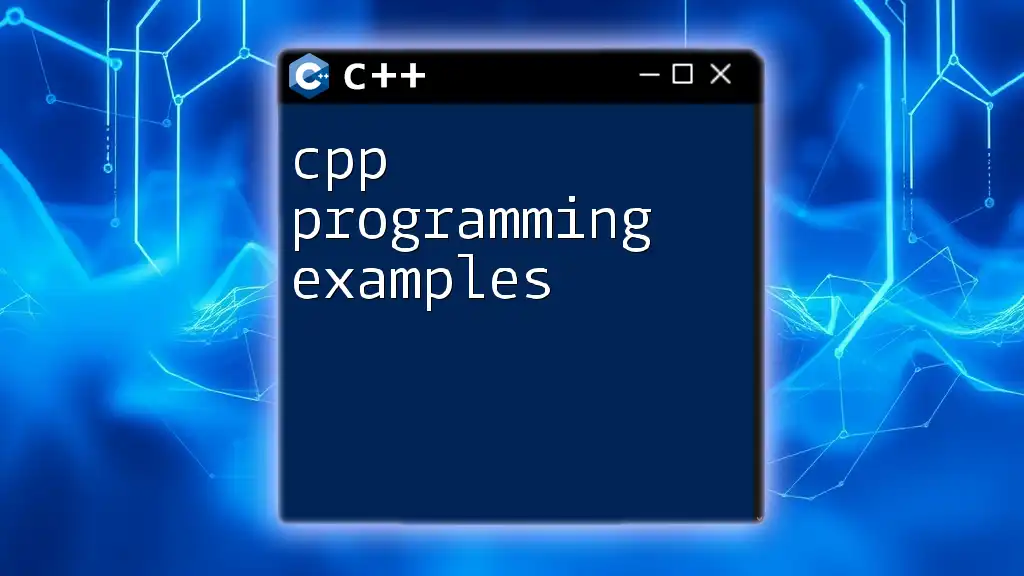
Standard Template Library (STL)
Introduction to STL
The Standard Template Library (STL) in C++ is a powerful set of C++ template classes to provide generic classes and functions. It helps improve code efficiency and simplicity with minimal coding effort.
Using STL Containers
Vectors
Vectors are dynamic arrays that can grow in size:
#include <vector>
vector<int> numbers = {1, 2, 3, 4, 5};
You can add or remove elements, ensuring that memory management is handled automatically.
Maps
Maps store key-value pairs, allowing for quick lookups:
#include <map>
map<string, int> ageMap = {{"Alice", 30}, {"Bob", 25}};
Accessing elements and iterating through maps is straightforward and efficient.
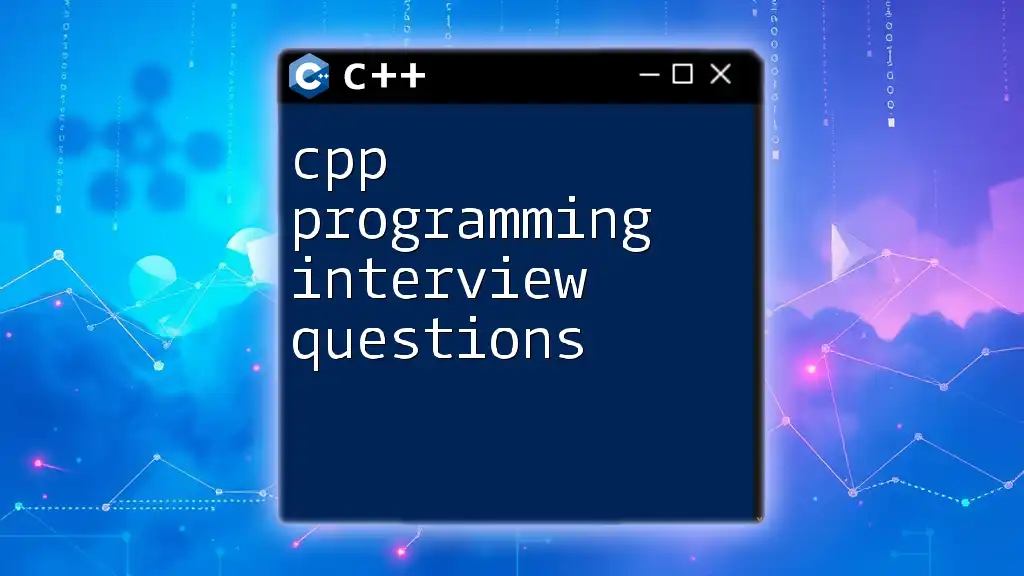
Debugging and Best Practices
Essential Debugging Techniques
Debugging is an essential skill for any programmer. It involves identifying and fixing errors within your code. Common errors include syntax errors (misspelled keywords, missing semicolons) and runtime errors (accessing out-of-range array indices, null pointer dereferencing). Use the debugging tools provided by your IDE to step through code and inspect variable states during execution.
Best Practices in C++ Programming
Following best practices improves code quality. Here are some tips:
- Code Readability: Use meaningful variable names, follow standard naming conventions, and add comments where necessary.
- Code Optimization: Optimize algorithms being careful to strike a balance between code complexity and performance.
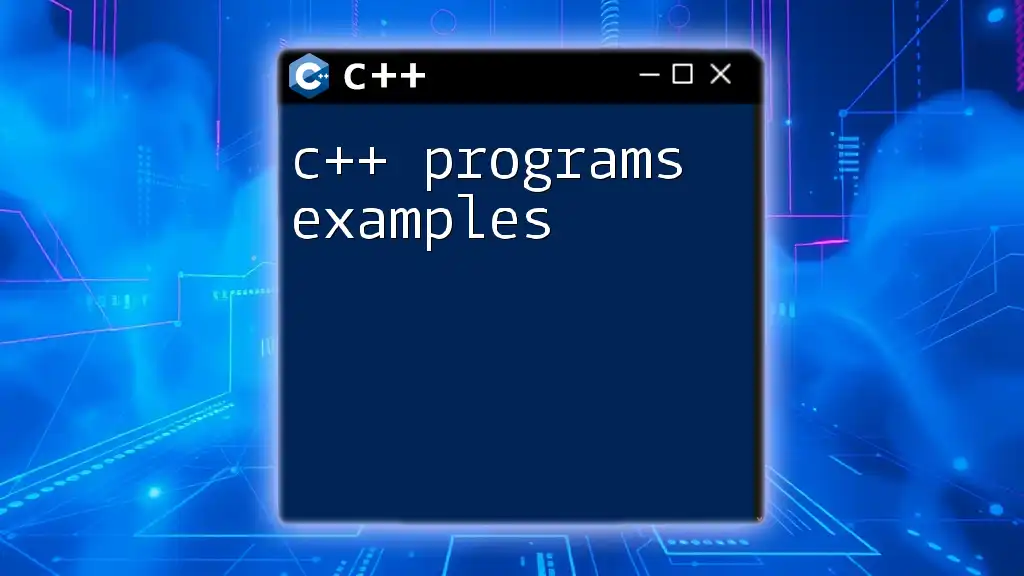
Conclusion
In this guide, we covered a comprehensive overview of C++ programs, from their basic structure and core concepts to the advantages of object-oriented programming and STL. Mastery of these concepts will cement your foundations in C++, enabling you to tackle more complex programming challenges.
To further your journey in learning, consider exploring more advanced topics and practical applications of C++ through hands-on programming exercises in our C++ courses.