CPP forms refer to the various ways in which C++ code can be structured, including function declarations, classes, and templates, to facilitate clear and efficient programming.
Here's a simple code snippet demonstrating a C++ function form:
#include <iostream>
// Function to add two numbers
int add(int a, int b) {
return a + b;
}
int main() {
std::cout << "Sum: " << add(5, 3) << std::endl; // Displays the sum
return 0;
}
Understanding C++ Forms
Definition of Forms
C++ forms are structures used in graphical user interfaces (GUIs) to gather and present data from users. They play a crucial role in creating intuitive applications by providing a straightforward way for users to interact with the software. Unlike standard input/output operations, which rely on console-based interactions, forms facilitate a seamless exchange of information through various controls and components.
Benefits of Using Forms
Using forms in applications comes with numerous benefits:
-
User Experience: Forms enhance the user interface by allowing users to input data visually, making interactions more engaging. A well-designed form can significantly improve how users perceive and utilize your software.
-
Data Validation: Forms can implement various validation techniques ensuring that the entered data meets specific criteria before processing, effectively reducing errors and enhancing data integrity.
-
Efficiency in Data Handling: Forms enable efficient data collection and retrieval, permitting users to submit multiple fields of information at once, streamlining the process for both them and the developers.
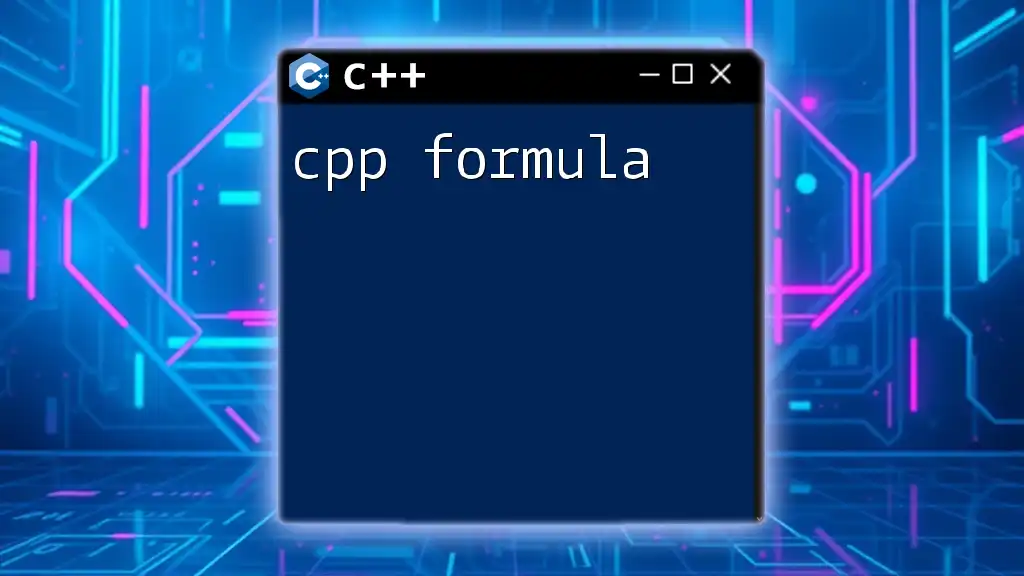
Building C++ Forms
Setting Up Your Environment
Before you start building C++ forms, you must choose the right libraries and frameworks that best suit your project needs. Popular libraries like Qt and wxWidgets provide an array of tools to create functional and visually appealing forms.
Basic Structure of a C++ Form
Header Files
To get started with creating forms, you need to include the necessary libraries. This often involves including standard header files such as:
#include <iostream>
#include <string>
These inclusions allow you to leverage C++ functionalities, such as standard input/output operations and string handling.
Class Definition
You will typically define a form as a class. This encapsulates all the functionalities and properties related to that form. Here’s an example of a simple form class:
class UserForm {
private:
std::string username;
std::string password;
public:
// Constructor and methods
};
Creating Your First Form
Using Qt Framework
Creating a GUI form using the Qt Framework involves a few straightforward steps:
-
Setting Up the Project: Initialize your Qt project and set up the main application.
-
Creating the Window: Here’s a minimal example to create a basic application window:
#include <QApplication>
#include <QWidget>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QWidget window;
window.setWindowTitle("Simple Form");
window.show();
return app.exec();
}
This code defines the basic structure of a Qt application and displays a simple window titled "Simple Form".
User Input Fields
C++ forms typically include multiple input fields. Common field types include text boxes, checkboxes, and radio buttons. For instance, adding username and password fields in your form can be done in this way:
QLineEdit *usernameField = new QLineEdit;
QLineEdit *passwordField = new QLineEdit;
These lines create two `QLineEdit` objects, which serve as text input fields for the user.
Form Layouts
Grid Layout vs. Vertical Box Layout
Effective layout design is critical for usability. You can choose from various layout arragements. Here’s an overview:
-
Grid Layout: This type allows organizing multiple widgets in a grid-like structure, which is helpful for aligning labels and input fields.
-
Vertical Box Layout: This layout stacks widgets vertically. It is simpler for forms when you want a straightforward, linear arrangement.
To implement these layouts, you could use code snippets like the following:
QGridLayout *gridLayout = new QGridLayout;
gridLayout->addWidget(new QLabel("Username:"), 0, 0);
gridLayout->addWidget(usernameField, 0, 1);
Handling User Input
Signal and Slot Mechanism
Qt's signal and slot mechanism is a powerful feature that helps in connecting user actions to application logic. For example, when a user clicks a button, you can connect that action to a function handling form submission:
connect(submitButton, &QPushButton::clicked, this, &UserForm::onSubmit);
This line ensures that when `submitButton` is clicked, the `onSubmit` method of the `UserForm` class is executed.
Data Validation Techniques
One of the most critical aspects of forms is validating user data. This can be completed through various methods. For instance, you may wish to check if an email address is in the correct format:
if (!emailPattern.match(emailField->text())) {
// Show error message
}
Using regular expressions or built-in validation routines allows for effective error prevention.
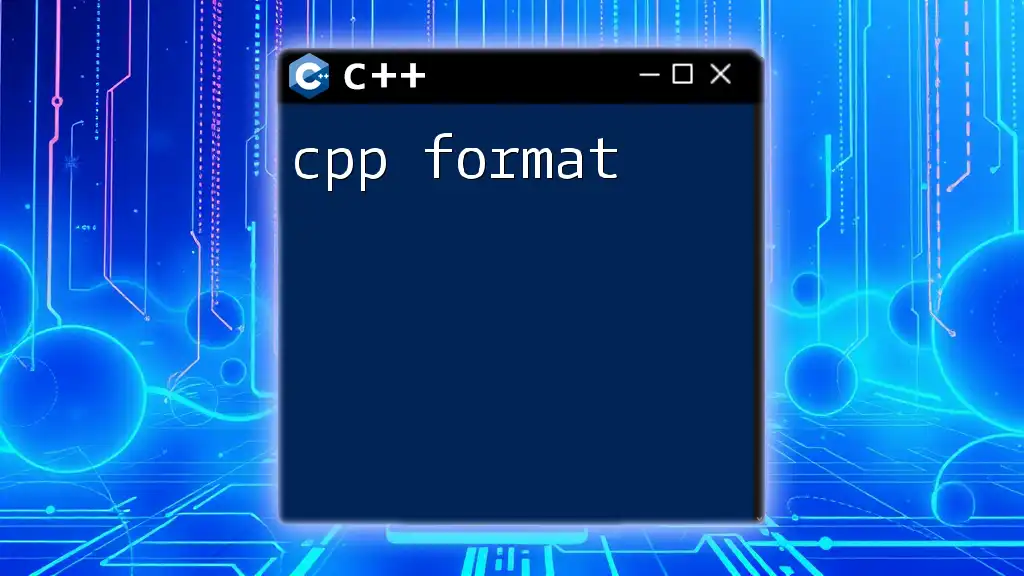
Advanced Form Features
Dynamic Form Creation
In more advanced applications, you may need to create forms dynamically based on user input. This flexibility lets you tailor the user experience and manage varying data requirements efficiently.
Integrating with Databases
Connecting forms to databases enables persistent data storage, allowing submitted form data to be saved for later retrieval. For example, after validating the form input, you might execute an SQL insert command in response to the user's submission.
// SQL Insert Command after form validation
This integration is crucial for applications like registration systems, feedback forms, and more.
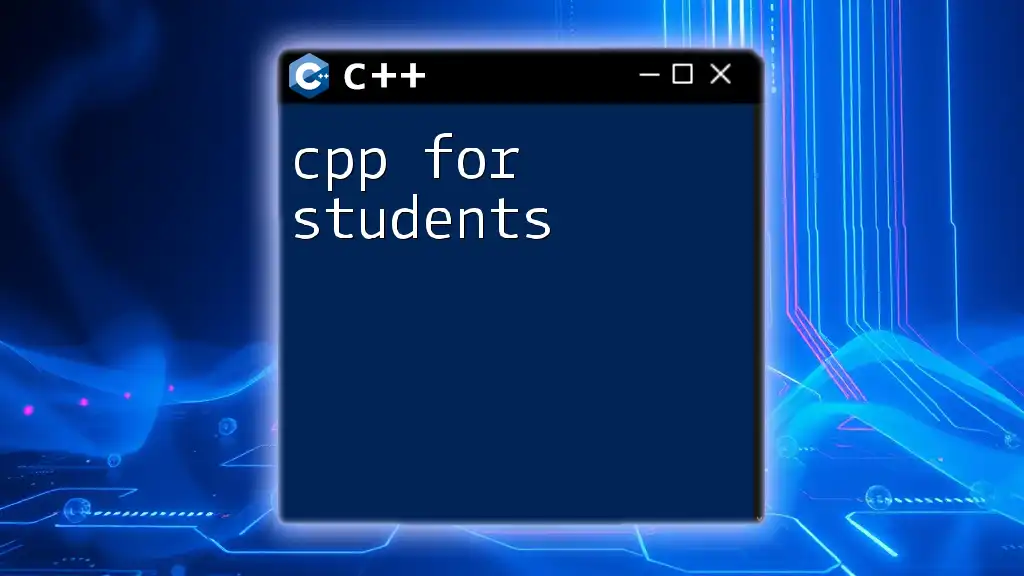
Best Practices for Designing C++ Forms
User-Centered Design Principles
Designing forms with the user in mind is essential. Ensure that your forms are intuitive, easy to navigate, and accessible. Utilize consistent labeling, clear instructions, and logical groupings of related fields to enhance usability.
Error Handling and Feedback
Providing users with immediate feedback is a cornerstone of good form design. Display clear error messages for invalid data and ensure users know what is required to correct their entries. For instance:
if(inputField.isEmpty()) {
errorLabel.setText("This field cannot be empty!");
}
This example provides direct feedback to users when they neglect to fill in a required field.
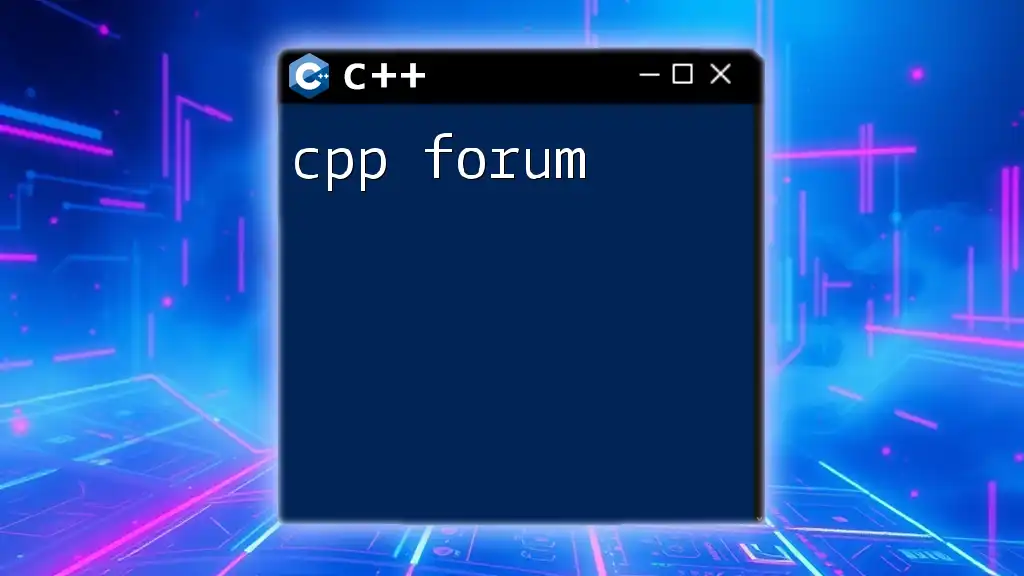
Conclusion
In conclusion, C++ forms are an integral part of modern software applications, facilitating user interaction and data handling. By understanding how to build, manage, and enhance forms using C++, developers can greatly improve the user experience and application performance. Engage with the concepts discussed here, and start creating your own user-friendly forms that elevate your projects to new heights.