Our CPP course offers a streamlined approach to mastering C++ commands through quick and concise lessons, empowering you to write efficient code with ease.
Here's a simple example of a C++ command:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What to Expect from a C++ Course
In a C++ course, you'll often encounter a structured learning approach designed to simplify the complexities of programming.
Course Structure
Typically, courses are divided into modules that cover foundational to advanced topics. The duration may vary, ranging from a few weeks to several months. Online courses provide flexibility, allowing you to learn at your own pace, while in-person classes can foster direct interaction with instructors and peers.
Target Audience
C++ courses cater to a wide range of learners, from complete novices with no prior programming experience to seasoned developers seeking to refine their skills. The course content may also be tailored to specific fields such as game development, systems programming, and application software.
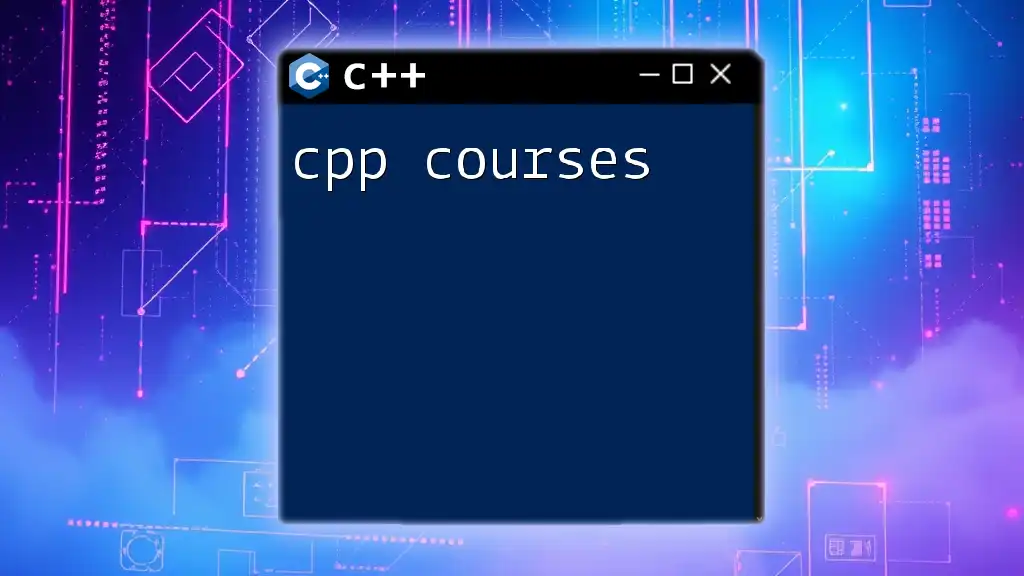
Key Topics Covered in a C++ Course
Fundamental Concepts of C++
The backbone of any C++ course lies in understanding fundamental concepts. These may include:
Variables and Data Types
A variable is a storage location identified by a name, which can hold a value. Familiarizing yourself with common data types such as `int`, `float`, `char`, and `string` is crucial.
int age = 25;
float salary = 50000.50;
char grade = 'A';
In this snippet, we declare a variable for age, store a salary with decimal precision, and represent a single character grade. Understanding these basics will help you manipulate data efficiently.
Control Structures
Control structures such as if-else statements, switch cases, and loops — including `for`, `while`, and `do-while` — allow you to dictate the flow of your programs.
for (int i = 0; i < 5; ++i) {
std::cout << i << std::endl;
}
In this example, a loop iterates from 0 to 4, printing each number. Control structures form the backbone of decision-making in your applications.
Functions in C++
Functions are essential for breaking down complex problems into manageable parts. They enable code reuse and improve organization.
int add(int a, int b) {
return a + b;
}
In this function, we return the sum of two integers. Learning to create, declare, and utilize functions properly is pivotal for effective coding.
Object-Oriented Programming (OOP) in C++
Object-oriented programming is a core offering of C++. Within a C++ course, students delve into:
Classes and Objects
Classes serve as blueprints for creating objects. A class encapsulates data and functionality, allowing for better code management.
class Car {
public:
void start() {
std::cout << "Car started!" << std::endl;
}
};
The `Car` class above illustrates the process of defining a simple class and its member method. Understanding how to create and manipulate classes is vital for object-oriented design.
Inheritance and Polymorphism
Inheritance allows new classes to inherit properties and methods from existing classes, fostering code reusability. Polymorphism, on the other hand, permits methods to do different things based on the object invoking them.
class Vehicle {
public:
virtual void honk() {
std::cout << "Vehicle honking!" << std::endl;
}
};
class Bike : public Vehicle {
public:
void honk() override {
std::cout << "Bike honking!" << std::endl;
}
};
The above code snippet illustrates a base class `Vehicle` and derived class `Bike`, showcasing how a child class can override a parent's method. Mastery of these concepts allows for greater flexibility and elegant design in your programs.
Advanced C++ Features
After grasping the fundamentals, a C++ course often progresses to advanced topics.
Templates and STL (Standard Template Library)
Templates are a mechanism that allows functions and classes to operate with generic types. Mastering them can drastically improve the efficiency of your code. The Standard Template Library (STL) provides useful data structures and algorithms.
#include <vector>
std::vector<int> nums = {1, 2, 3, 4, 5};
This snippet showcases how to use a vector from STL, which is a dynamic array that automatically resizes. Being proficient with STL is instrumental in modern C++ software development.
Exception Handling
Exception handling is crucial for developing robust applications. It allows you to gracefully handle runtime errors without crashing your program.
try {
// code that may throw
} catch (const std::exception& e) {
std::cout << e.what() << std::endl;
}
In this example, the `try` block contains code that might generate an exception, while the `catch` block handles it gracefully, displaying an error message. Familiarizing yourself with these processes will enhance your programming reliability.
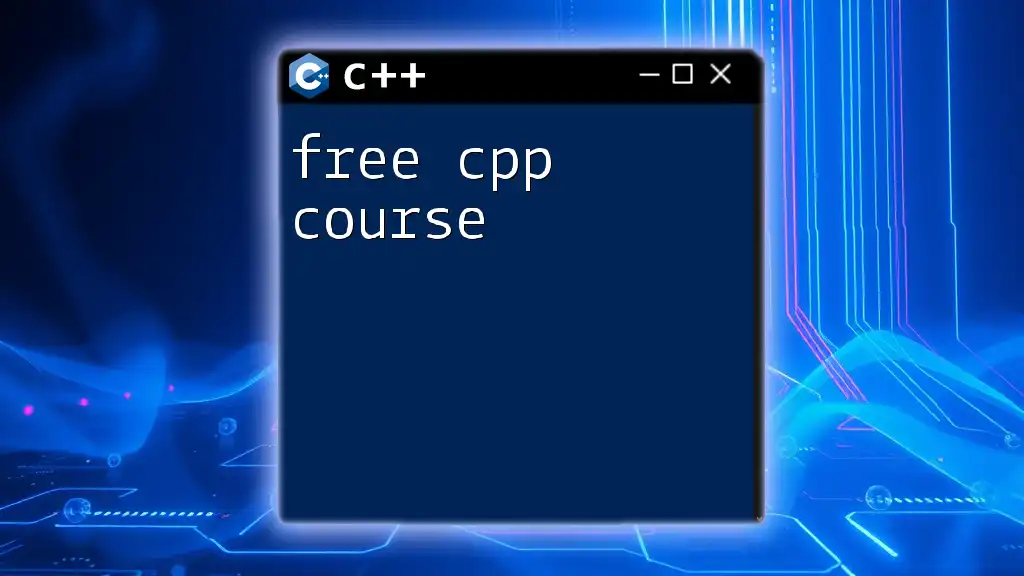
Best Practices for Learning C++
Hands-On Projects
To fully absorb what you've learned, practical experience is invaluable. Engaging in hands-on projects helps solidify concepts and improve your problem-solving abilities. Start with simple applications, such as creating a calculator or a basic game like Snake, to apply your knowledge in a real-world context.
Resources and Tools
Select the right Integrated Development Environment (IDE) for your learning path. Platforms like Visual Studio and Code::Blocks offer extensive features that can streamline your coding process.
In addition, tap into online communities and forums, such as Stack Overflow and Reddit, for support and collaboration, which are excellent for troubleshooting. To supplement your learning, explore various materials such as books, tutorial websites, and video lectures tailored to C++.
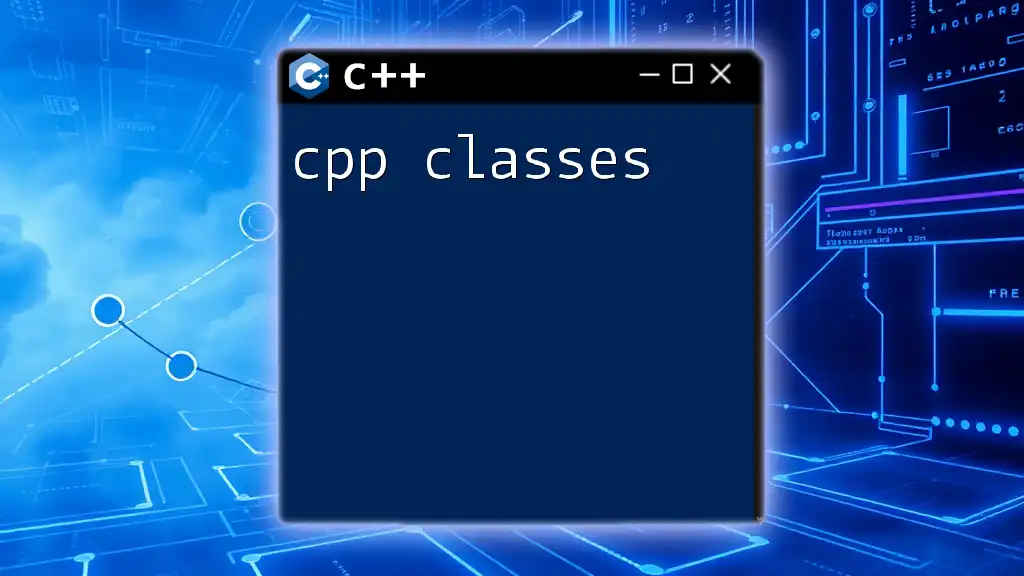
Conclusion
Participating in a C++ course can be a transformative experience for individuals seeking to broaden their programming proficiency. Learning C++ not only provides you with the skills necessary to develop software across multiple domains but also lays a solid foundation for understanding other programming languages.
Embarking on this journey will equip you with tools that can advance your career and navigate the ever-evolving landscape of technology. Don’t wait — enroll in a C++ coding course today to take your first step towards becoming a proficient C++ programmer!
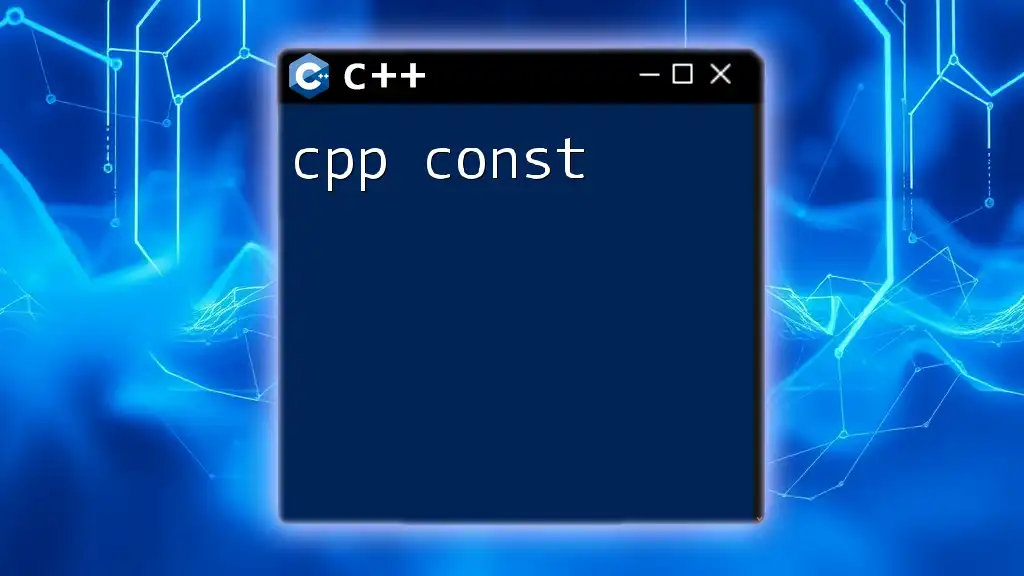
FAQ Section
Common Questions About C++ Courses
How Long Does It Take to Learn C++?
The time required varies based on individual commitment and prior experience, but a structured course can typically cover the basics in a few weeks.
What Are the Job Opportunities Post-Course Completion?
Skills in C++ open doors across various sectors, including game development, systems programming, and software engineering.
How Do I Choose the Right C++ Course for Me?
Consider your current skill level, desired learning format (online or in-person), and specific interests within the C++ ecosystem to find a suitable course.