Unlock the basics of C++ programming with our free C++ course designed to teach you essential commands quickly and concisely. Here's a simple example of a C++ program that outputs "Hello, World!":
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++?
C++ is a general-purpose programming language that was developed as an extension of the C programming language. It was created by Bjarne Stroustrup at Bell Labs in the early 1980s. C++ has become a fundamental language in software development due to its performance efficiency and versatility. Some of its essential features include:
- Object-Oriented Programming (OOP): C++ supports OOP principles, enabling developers to create modular and reusable code.
- Standard Template Library (STL): STL provides a rich set of methods for managing data structures and algorithms, making coding easier and more efficient.
Why Learn C++?
Learning C++ can open doors to numerous career opportunities. It is widely used in various domains such as:
- Game development: Many game engines, including Unreal Engine, are written in C++.
- Systems programming: C++ is a preferred choice for developing operating systems and system-level applications due to its ability to interact closely with hardware.
- Embedded systems: C++ plays a crucial role in programming embedded systems for devices ranging from home appliances to automotive systems.
C++ also offers performance advantages over many modern programming languages, with a strong focus on efficiency and speed. Its versatility means it can be used across different fields, making it a valuable skill for aspiring developers.
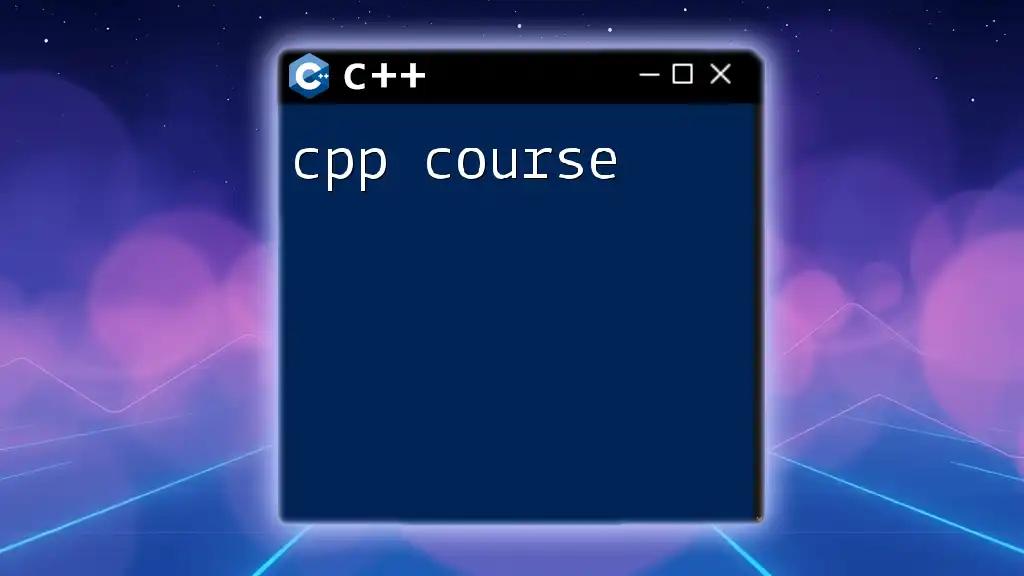
Course Structure
In this free C++ course, you will find a concise and practical learning approach. The course is designed to include:
- Short video tutorials that focus on specific concepts.
- Quizzes to reinforce your understanding as you progress.
- Hands-on coding exercises that enable you to practice what you learn immediately.
The structured layout ensures that you build a solid foundation while keeping the learning process engaging and manageable.
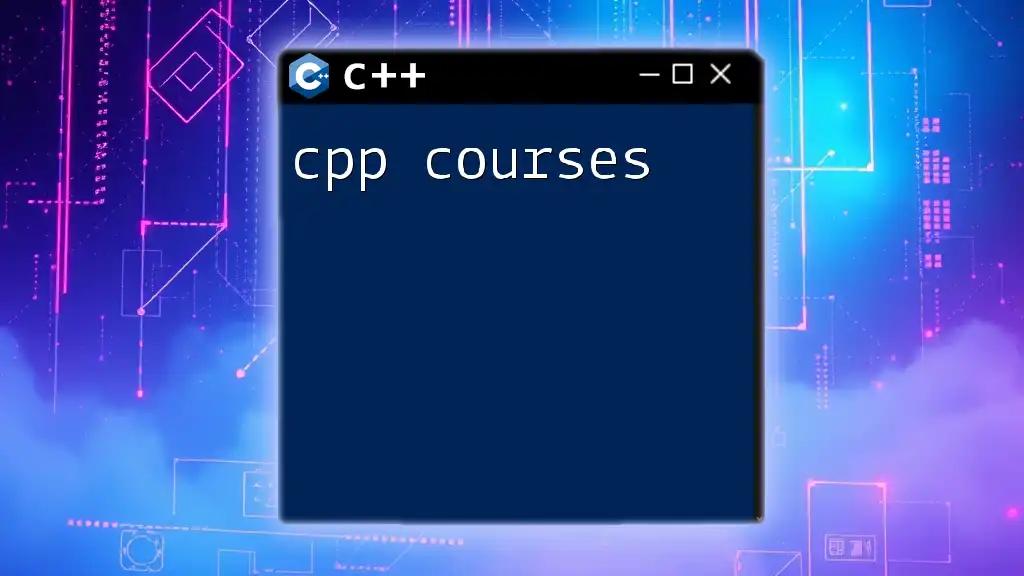
Getting Started
Setting Up Your Development Environment
To kick off your C++ journey, setting up your development environment is crucial. Here are some recommended Integrated Development Environments (IDEs):
- Visual Studio: A robust environment with excellent debugging tools.
- Code::Blocks: A lightweight and user-friendly IDE that is suitable for beginners.
- CLion: A powerful cross-platform IDE with extensive coding assistance features.
Installation Steps: Follow the instructions provided on the respective IDE's official website to download and install the software.
Writing Your First C++ Program: "Hello, World!"
Once your IDE is set up, it’s time to write your first C++ program. Open your IDE and create a new project, then input the following code:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
When you run the above code, you should see the output "Hello, World!" displayed in the console, marking the successful start of your programming journey.
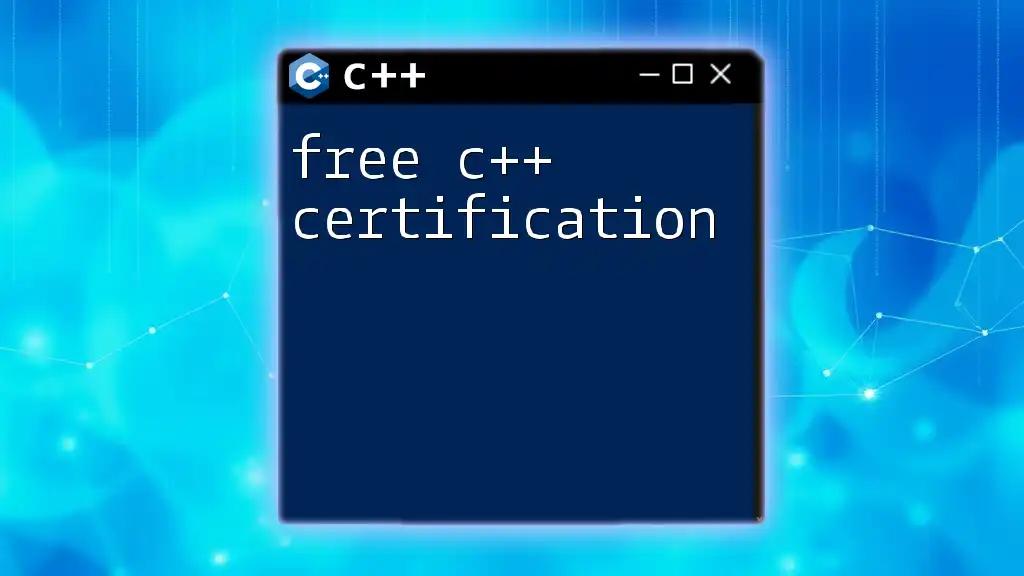
Core Concepts of C++
Basic Syntax
Understanding the basic syntax of C++ is vital. This involves learning about variables, data types, and operators. In C++, you can declare a variable like so:
int age = 30;
float salary = 50000.50;
In this example, `age` is an integer variable, and `salary` is a floating-point variable. Familiarizing yourself with these types will help you understand how data is stored and manipulated in C++.
Control Structures
C++ provides various control structures that dictate the flow of execution in a program.
Conditionals
Conditionals help in making decisions. Here’s an example using an `if` statement:
if (age >= 18) {
std::cout << "You are an adult." << std::endl;
} else {
std::cout << "You are a minor." << std::endl;
}
Loops
Loops enable repeated execution of code. A simple `for` loop example is as follows:
for (int i = 0; i < 5; i++) {
std::cout << "Iteration: " << i << std::endl;
}
This loop will print out the iteration count from 0 to 4, demonstrating how you can execute repetitive tasks efficiently.
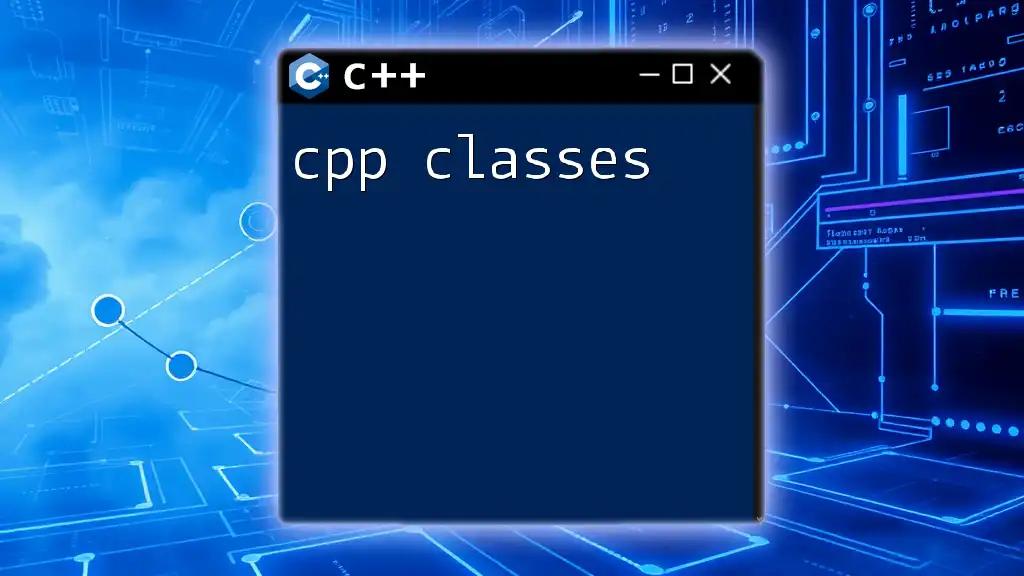
Functions in C++
Defining Functions
Functions are essential for organizing your code into reusable parts. Here is how to define a simple function:
void greet() {
std::cout << "Welcome to the Free C++ Course!" << std::endl;
}
You can call this function from your `main()` function to display the greeting message.
Function Overloading
C++ allows you to have multiple functions with the same name but different parameter types or counts. Here’s a basic example:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
In this code, the same function name `add` is used for both integer and double parameters, showcasing function overloading effectively.
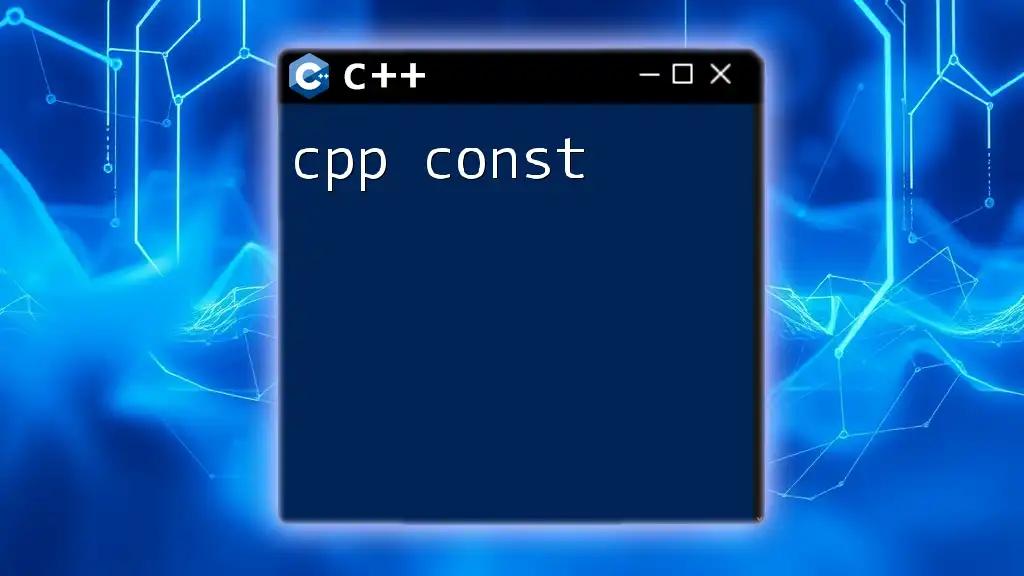
Object-Oriented Programming
Classes and Objects
One of the standout features of C++ is its embrace of Object-Oriented Programming. A class acts as a blueprint for creating objects. Here’s a simple example:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
Dog myDog;
myDog.bark();
In this example, we create a class `Dog` with a method `bark`, then instantiate it and call the method.
Inheritance and Polymorphism
Inheritance allows a new class to inherit properties and methods from an existing class, while polymorphism allows methods to do different things based on the object it is acting upon.
Templates
Templates in C++ are a powerful feature that allows functions and classes to operate with generic types. Here’s a simple template example:
template <typename T>
T add(T a, T b) {
return a + b;
}
This function can now be used with any data type (int, float, etc.), making your code flexible and reusable.
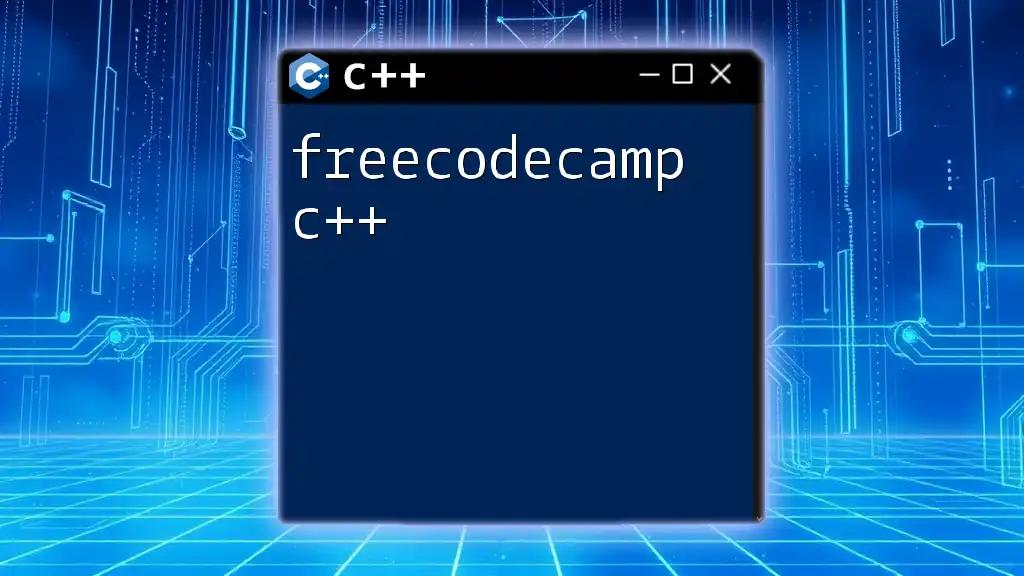
Advanced Topics (Optional)
For those who wish to delve deeper, the following topics are beneficial:
- Pointers and References: Critical for memory management and performance.
- File Handling: Learn how to read from and write to files.
- Exception Handling: Understand how to manage errors gracefully in your code.

Course Bonuses
Enrolling in this free C++ course grants access to several bonuses, such as:
- Additional resources to deepen your learning.
- Exclusive community support to help with any questions or challenges.
- Ideas for projects to practice your skills and apply what you've learned.

Conclusion
In this guide, we've covered essential topics that lay the groundwork for your journey into C++. As you progress, remember to keep practicing and experimenting with code.
With dedication and effort, you'll become proficient in C++, unlocking numerous opportunities in your programming career.

FAQs
-
What are the prerequisites for this C++ course? A basic understanding of programming concepts will help but is not necessary to begin.
-
How long will the course take to complete? The timeline varies; however, the course's design emphasizes concise and effective learning, so you can progress at a comfortable pace.
Dive in, and let's begin this exciting journey together in the world of C++!