To effectively test C++ code, you can write a simple program that includes basic input and output functionality, allowing you to ensure your code behaves as expected. Here's an example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding the Basics of C++ Code Testing
What is C++ Code Testing?
C++ code testing involves evaluating the correctness and functionality of software written in C++. It is a crucial step in the development process aimed at identifying errors, ensuring software reliability, and enhancing overall code quality. The key objectives of testing include verifying that the code behaves as expected, preventing regression of features, and validating user requirements.
Familiarity with common terminology is essential for understanding C++ code testing. Key terms include:
- Unit Tests: Tests that validate individual components or functions of the code.
- Integration Tests: Tests that assess the interactions between different components within the application.
- Functional Tests: Tests that examine if the software meets specified requirements.
Types of C++ Code Testing
Unit Testing is the most fundamental type of testing. It focuses on testing small, isolated pieces of code, typically a single function or class, to ensure they operate correctly on their own. For example, using a popular testing framework like Google Test, a simple unit test might look like this:
#include <gtest/gtest.h>
// Function to be tested
int Add(int a, int b) {
return a + b;
}
// Unit test
TEST(AdditionTest, PositiveNumbers) {
EXPECT_EQ(Add(2, 3), 5);
EXPECT_EQ(Add(0, 0), 0);
}
Integration Testing, on the other hand, checks how different modules of the application interact with each other. It ensures that the combined functionality of multiple components works as intended. Here’s an integration testing example that validates the coordination between two functions:
#include <gtest/gtest.h>
int Multiply(int a, int b) {
return a * b;
}
// Function using Multiply
int AddAndMultiply(int a, int b, int c) {
return Add(a, b) * c; // Add function from earlier
}
// Integration test
TEST(IntegrationTest, AddAndMultiply) {
EXPECT_EQ(AddAndMultiply(2, 3, 4), 20); // (2 + 3) * 4
}
Functional Testing assesses the software against its functional requirements. It often involves executing specific scenarios that users will encounter. For example, if a user expects a particular interface response, functional tests should validate that expectation through structured test cases.
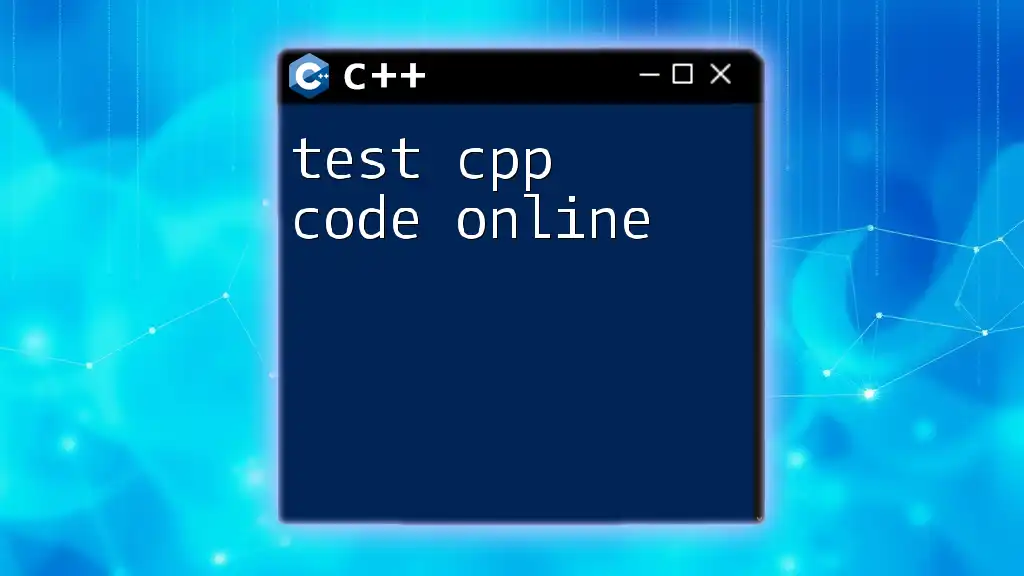
Setting Up Your C++ Testing Environment
Tools Required for Testing C++ Code
Selecting the right tools plays a pivotal role in C++ code testing. Popular frameworks such as Google Test, Catch2, and CPPUTest are widely used for creating and managing tests.
Google Test is particularly revered for its rich feature set, usability, and seamless integration into various environments. To install Google Test, you can often utilize package managers like `vcpkg` or clone the repository directly from GitHub.
Writing Your First C++ Test
Once your testing environment is set up, the next step is writing your first test case. Here’s a simple example that demonstrates the testing process:
#include <gtest/gtest.h>
int Subtract(int a, int b) {
return a - b;
}
// Test case
TEST(SubtractionTest, BasicAssertions) {
EXPECT_EQ(Subtract(10, 5), 5);
EXPECT_NE(Subtract(20, 10), 10);
}
In this code, we defined a function `Subtract` and created a test case that checks if the function behaves correctly by validating both equality and inequality.
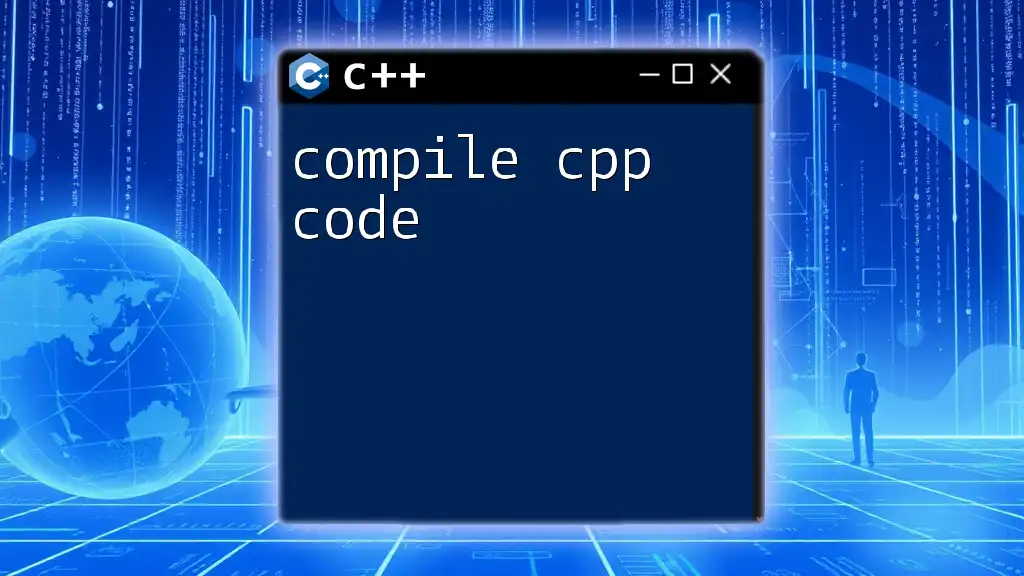
Using a C++ Code Tester
What is a C++ Code Tester?
A C++ code tester is a tool that automates the process of running your tests and checking results. It simplifies the process of validating code correctness and plays a crucial role in efficient software development.
Popular C++ Code Testing Tools
Google Test remains the most popular choice among C++ developers due to its comprehensive documentation and community support. Here’s how writing tests using Google Test looks:
#include <gtest/gtest.h>
TEST(StringLengthTest, BasicAssertions) {
std::string str = "Hello World!";
EXPECT_EQ(str.length(), 12);
}
Catch2 is another robust testing framework. It stands out for its simple setup and header-only approach. Here is an example of using Catch2:
#define CATCH_CONFIG_MAIN
#include <catch.hpp>
TEST_CASE("String length test") {
std::string str = "Catch2";
CHECK(str.length() == 6);
}
CPPUTest is particularly beneficial for unit testing in embedded systems. Here’s a simple CPPUTest test case:
#include <CPPUTest/CommandLineTestRunner.h>
TEST_GROUP(MathFunctions) {};
TEST(MathFunctions, AddTest) {
CHECK(Add(2, 3) == 5);
}
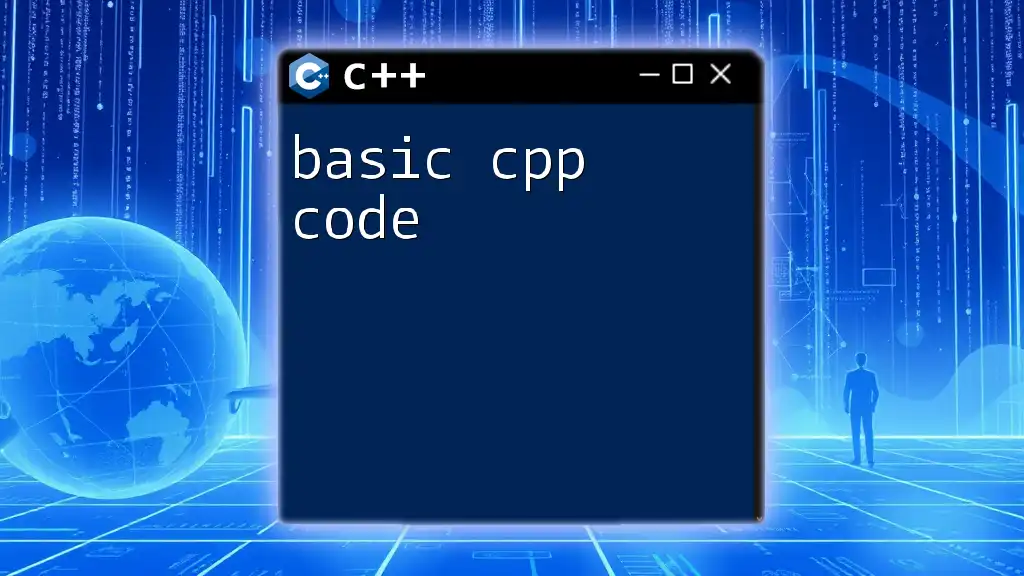
Writing Effective Tests
Best Practices for C++ Code Testing
Effective testing leads to maintainable and reliable code. Here are some best practices:
-
Clarity and Conciseness: Write tests that are easy to understand. Each test should focus on a single aspect of the function.
-
Maintain Test Readability: Use meaningful names for your test cases. Make sure someone else can understand the purpose behind each test.
-
Use of Assertions: Assertions are vital in testing, as they validate expected outcomes against actual results. For instance:
TEST(AdditionTest, HandlesPositiveInput) {
EXPECT_TRUE(Add(3, 7) == 10); // Validates the result
EXPECT_FALSE(Add(5, 5) == 11); // Validates an expectation that should be false
}
Common Pitfalls in C++ Testing
Avoid frequent mistakes that can lead to inefficiencies. Common pitfalls include:
-
Overly Complex Tests: Tests should not test multiple behaviors at once. Focus on one scenario at a time to avoid confusion.
-
Ignoring Edge Cases: Always test edge conditions, such as empty inputs or maximum/minimum values.
By addressing these pitfalls upfront, you can significantly enhance the quality of your testing suite.
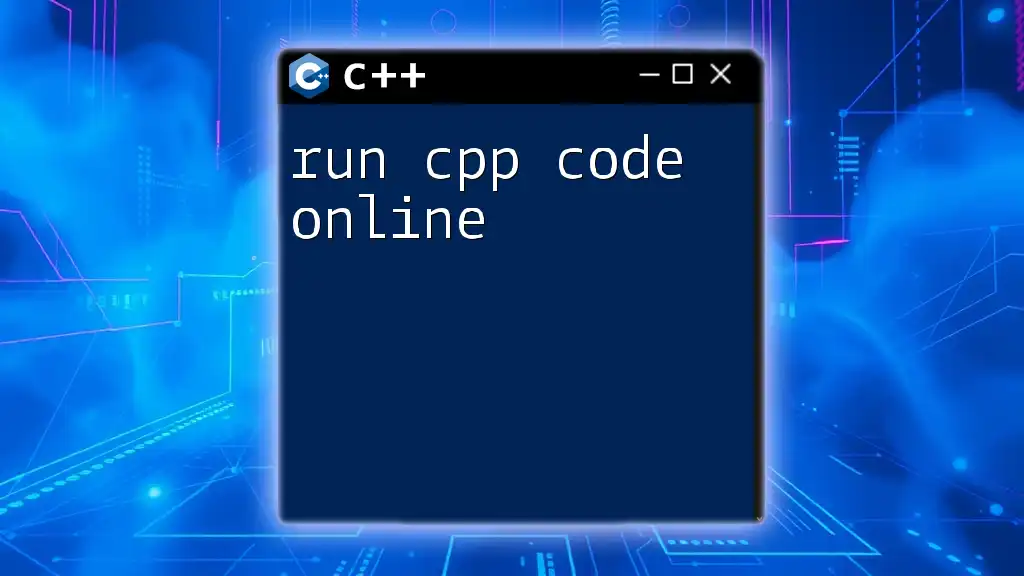
Running and Automation of Tests
Running Your C++ Tests
Running tests can often be achieved effortlessly through the command line. For example, to run tests using Google Test, you compile the test files and execute the output file. A common command would look like:
g++ -o my_tests my_tests.cpp -lgtest -lpthread
./my_tests
Interpreting test results is straightforward, with successful assertions typically yielding no output, while failures will display detailed error messages.
Automating Your C++ Tests
Automation is essential in modern development workflows. Continuous Integration (CI) processes ensure that tests run automatically with every code update. Popular CI tools like Jenkins or GitHub Actions facilitate this:
- Setting Up a CI Tool: You can create a `.yaml` file for GitHub Actions that outlines how tests run.
- Triggering Tests on Push: Your configuration could automatically trigger tests upon a commit to the repository.
Here's an example of a simple GitHub Actions workflow for C++ tests:
name: C++ Testing Pipeline
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout Code
uses: actions/checkout@v2
- name: Install g++
run: sudo apt-get install g++
- name: Compile Tests
run: g++ -o my_tests my_tests.cpp -lgtest -lpthread
- name: Run Tests
run: ./my_tests
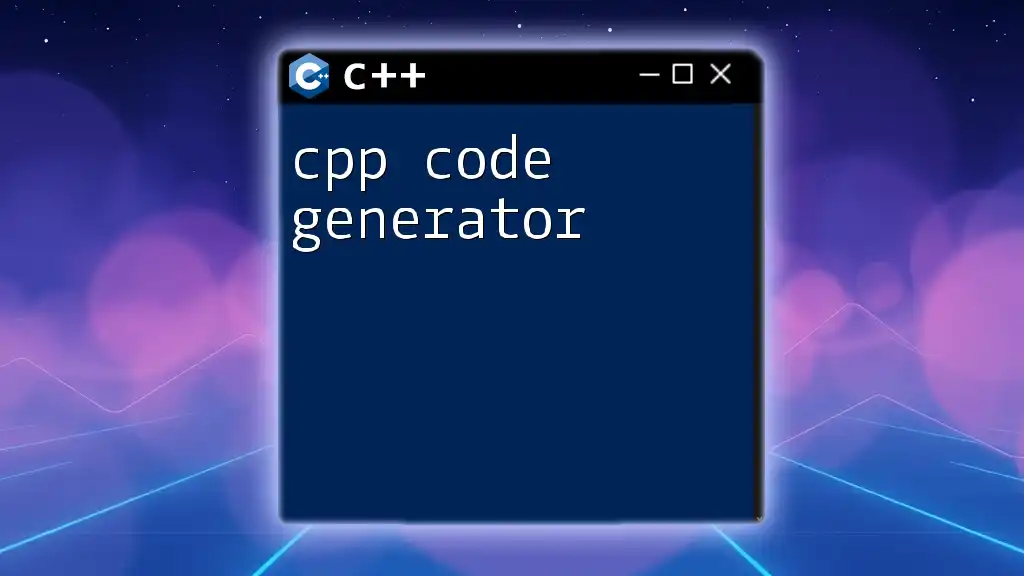
Debugging Failed Tests
Common Issues Leading to Test Failures
Identifying why tests fail is part of the testing process. Common causes may include:
- Logical errors in the code
- Incorrect test setup or initialization
- Unhandled scenarios in the code
Using Debugging Tools
A variety of debugging tools are available when working with C++. For instance, GDB (GNU Debugger) allows you to step through code and monitor variable values. Combining the power of a debugger with test cases can clarify failures, as you can analyze the stack and variable states leading to a test's failure.
Regardless of the testing methodology or framework, consistently applying these practices and understanding these principles will empower you to thoroughly test C++ code effectively. By improving your testing skills, you can produce reliable, maintainable, and high-quality C++ code that serves its intended purpose without unexpected behaviors.