To check C++ code for errors and ensure it functions correctly, you can use a simple example that includes a basic compilation and output verification process.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // Check if code prints "Hello, World!"
return 0;
}
Understanding C++ Code Quality
Code quality refers to the properties that make code maintainable, understandable, and functional. In C++, it is essential to uphold certain standards due to the language's complexity and performance-critical nature. The key factors influencing code quality include readability, maintainability, and performance.
Benefits of Checking C++ Code
Checking your C++ code is crucial for several reasons:
-
Improved Performance and Reliability: By identifying and addressing issues early, you can ensure that your application runs more efficiently and reliably under various conditions.
-
Enhanced Security: Code checking plays a critical role in identifying vulnerabilities that can be exploited. Ensuring your code is free from common pitfalls is integral to building a secure application.
-
Greater Readability: Clean code is easier to read and understand, which is necessary for effective collaboration among team members and future maintainers.
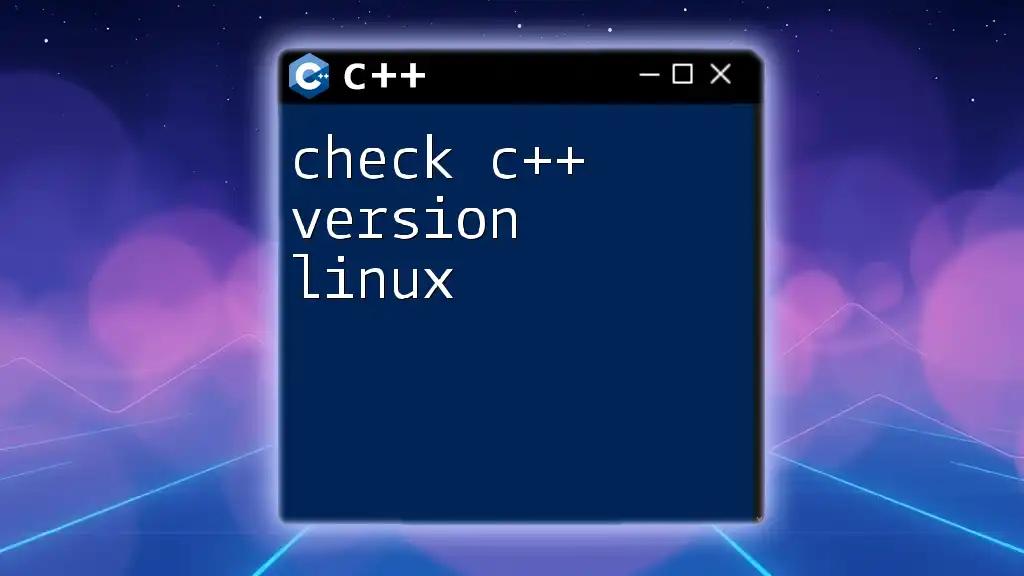
Common C++ Code Checking Techniques
Static Code Analysis
Static code analysis refers to the examination of code without executing it. This process helps to identify potential bugs, enforce coding standards, and evaluate complexity.
Popular Static Code Analysis Tools
-
CPPcheck CPPcheck is a widely-used static analysis tool for C++. It's capable of detecting various issues such as memory leaks, mismatched types, and improper function calls. To use CPPcheck, follow these steps:
-
Installation: CPPcheck can usually be installed via your package manager or downloaded from its [official website](http://cppcheck.sourceforge.net/).
-
Usage Example: To check a C++ file, you would run:
cppcheck my_code.cpp
CPPcheck reports issues directly on the command line, allowing for direct feedback.
-
-
Clang Static Analyzer The Clang Static Analyzer is part of the Clang project and offers a powerful way to find bugs in C++ code. To install it, you typically need to have Clang already set up. Here's how to use Clang Static Analyzer:
scan-build make
This command will build your project and analyze it simultaneously, providing you with a report highlighting potential issues.
Dynamic Code Analysis
Dynamic code analysis involves testing the program while it is running, which allows for the discovery of errors that occur in real-time.
Tools for Dynamic Code Checking
-
Valgrind Valgrind is a powerful tool for memory debugging, memory leak detection, and profiling. To check a program for memory leaks with Valgrind, you’d use:
valgrind --leak-check=full ./my_program
Valgrind provides detailed information about memory usage, helping you identify leaks and improper memory handling.
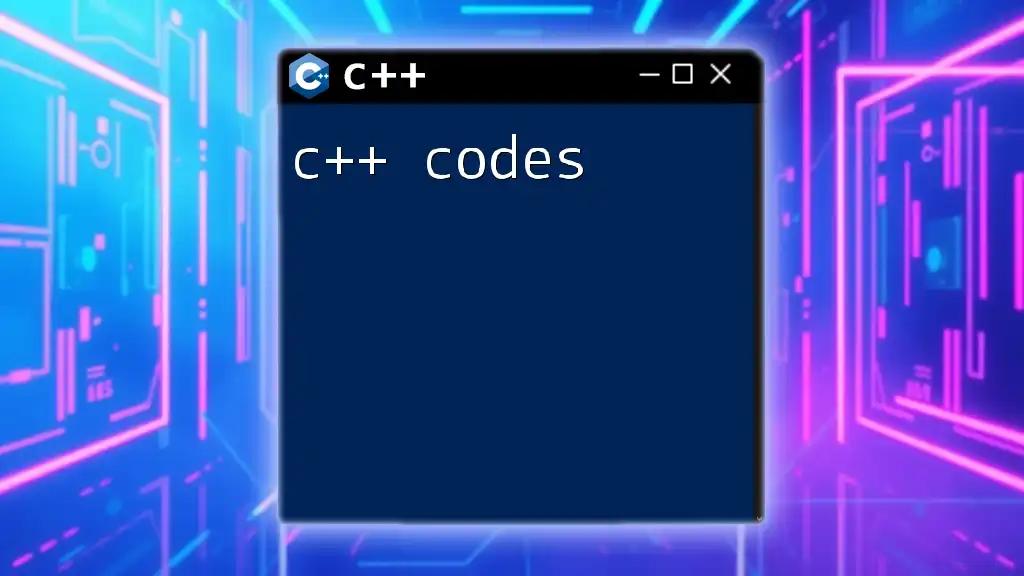
Best Practices for C++ Code Checking
Manual Code Reviews
Code reviews are an essential practice in software development. They ensure that code adheres to standards and encourage learning among team members. Here are practices to conduct effective code reviews:
-
Establish Clear Guidelines: Define what aspects need to be checked, including style, logic, and documentation.
-
Foster a Collaborative Environment: Encourage constructive feedback and discussions about code improvements, making reviews a learning experience for all.
Writing Unit Tests
Unit tests are crucial for validating individual components of your code. They help catch bugs early in the development process and ensure that changes do not introduce new errors.
Example of Writing a Simple Unit Test
Using a popular framework like Google Test, you can easily create unit tests. Here’s a simple example that tests an addition function:
#include <gtest/gtest.h>
int Add(int a, int b) {
return a + b;
}
TEST(AddTest, HandlesPositiveInput) {
ASSERT_EQ(Add(1, 2), 3);
}
This unit test confirms that the `Add` function works as expected with positive integers. Writing comprehensive tests is essential for ongoing software reliability.
Continuous Integration
Continuous integration (CI) integrates code into a shared repository multiple times a day. It automates the process of checking C++ code to ensure quality and functionality throughout the development lifecycle.
Integrating Code Checking into CI
By setting up a CI pipeline, you can run static analysis tools and tests automatically with each code commit. Here’s a simple example of a CI configuration using GitHub Actions:
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up C++ environment
run: sudo apt-get install g++ cppcheck
- name: Run cppcheck
run: cppcheck .
This pipeline installs necessary tools and runs cppcheck, helping maintain code quality.
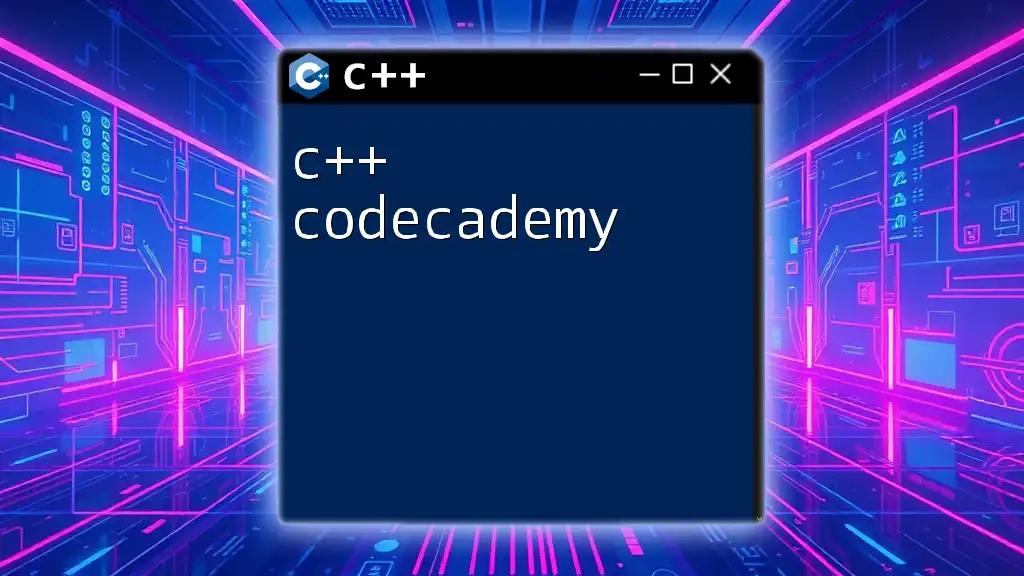
Advanced C++ Code Checking Techniques
Profiling and Performance Analysis
Profiling involves measuring the performance of your code to identify bottlenecks. Profiling tools help you understand where your application spends most of its time or uses excessive resources.
Profiling Example with gprof
gprof is a profiling program used to analyze the performance of C++ applications. To use gprof:
- Compile your code with the profiling option:
g++ -pg my_code.cpp -o my_program
- Run your program:
./my_program
- Generate the gprof report:
gprof my_program gmon.out > analysis.txt
This results in a detailed report that helps pinpoint performance issues.
Version Control Practices
Version control, such as Git, is vital for tracking changes in your codebase. It allows multiple developers to work simultaneously while managing conflict resolution effectively.
Benefits of Git for Code Checking
-
Collaborative Development: Branching strategies can isolate features, allowing for thorough code checking before merging changes.
-
History Tracking: Git maintains a history of changes, making it easier to review modifications and understand the evolution of the code.
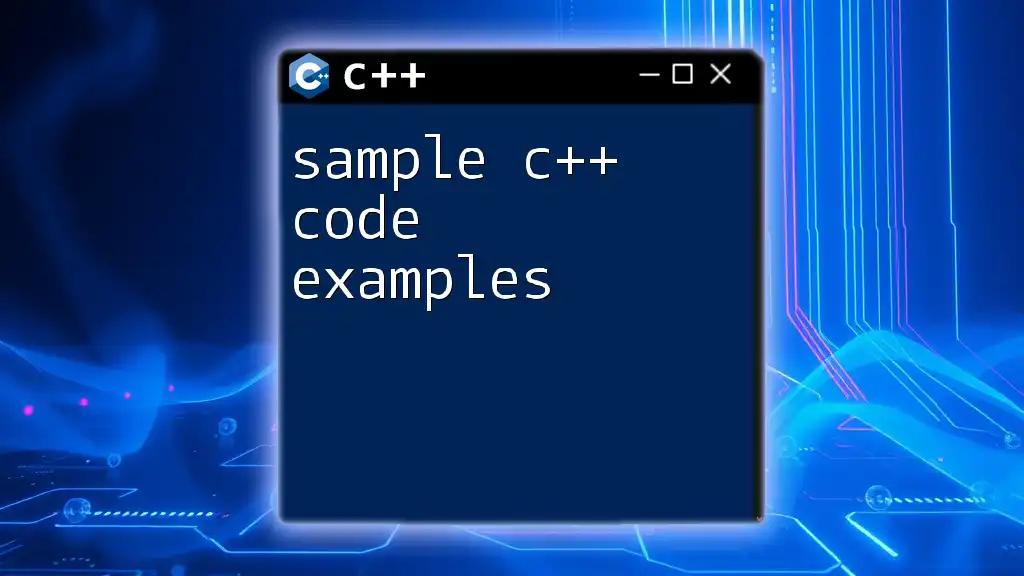
Conclusion
Checking C++ code is not merely a process but a robust practice that ensures the reliability and efficiency of your applications. By utilizing various tools and methodologies such as static and dynamic analysis, fostering manual reviews, writing comprehensive unit tests, and leveraging continuous integration, you establish a solid foundation for high-quality code. Implementing these practices today will not only enhance your current projects but also set you on the path to becoming a skilled C++ developer prepared for future challenges.
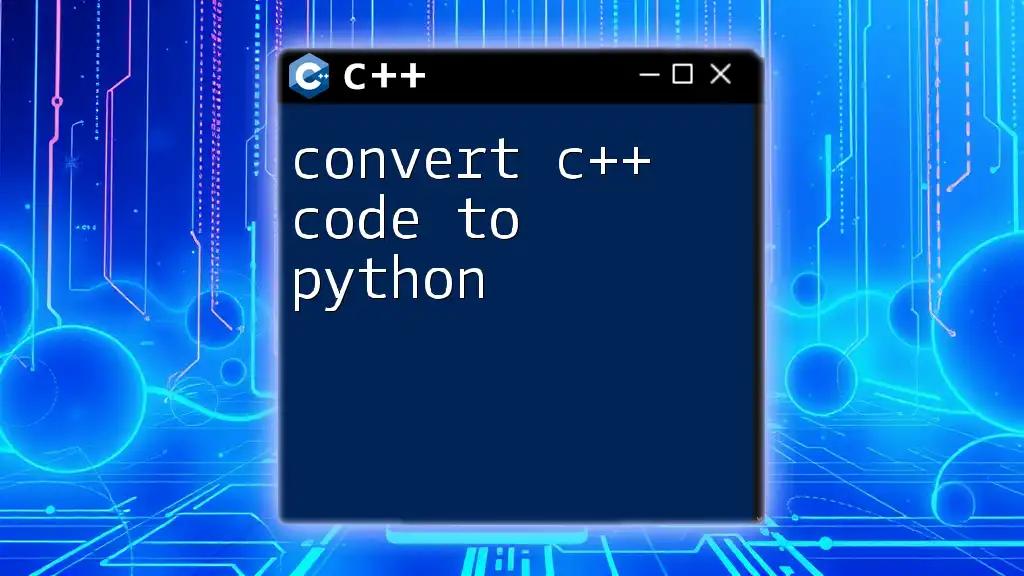
Additional Resources
To further assist you in checking C++ code effectively, consider exploring the following resources:
- CPPcheck Documentation: Official guidelines and usage tips.
- Clang Static Analyzer Guide: Comprehensive resources on installation, usage, and troubleshooting.
- Google Test Documentation: In-depth tutorials on writing unit tests for C++.
- Valgrind Manual: A detailed manual on memory debugging and profiling.
Explore these resources to broaden your understanding and mastery of C++ coding practices!