C++ code formatting is the practice of organizing and structuring code to enhance readability and maintainability, ensuring that proper indentation, spacing, and consistent naming conventions are used throughout.
Here’s an example of well-formatted C++ code:
#include <iostream>
int main() {
int number;
std::cout << "Enter a number: ";
std::cin >> number;
if (number % 2 == 0) {
std::cout << number << " is even." << std::endl;
} else {
std::cout << number << " is odd." << std::endl;
}
return 0;
}
Understanding C++ Code Formatting
What is Code Formatting?
C++ code formatting refers to the practice of designing the code structure in a uniform manner that enhances its readability and maintainability. The format includes not just the arrangement of lines but also how code blocks, functions, and expressions are structured. Proper code formatting is essential in collaborative programming environments where multiple developers work on the same project.
Why Code Formatting Matters
Adhering to good C++ code formatting standards promotes readability, making it easier for developers to understand the logic of the code. This becomes particularly crucial when revisiting code after a period of time or when someone else is trying to comprehend your work. It also enhances collaboration; when everyone follows the same rules, it minimizes misunderstandings and reduces the chances of errors. Additionally, well-formatted code simplifies the process of debugging, as the structure helps pinpoint issues quickly.
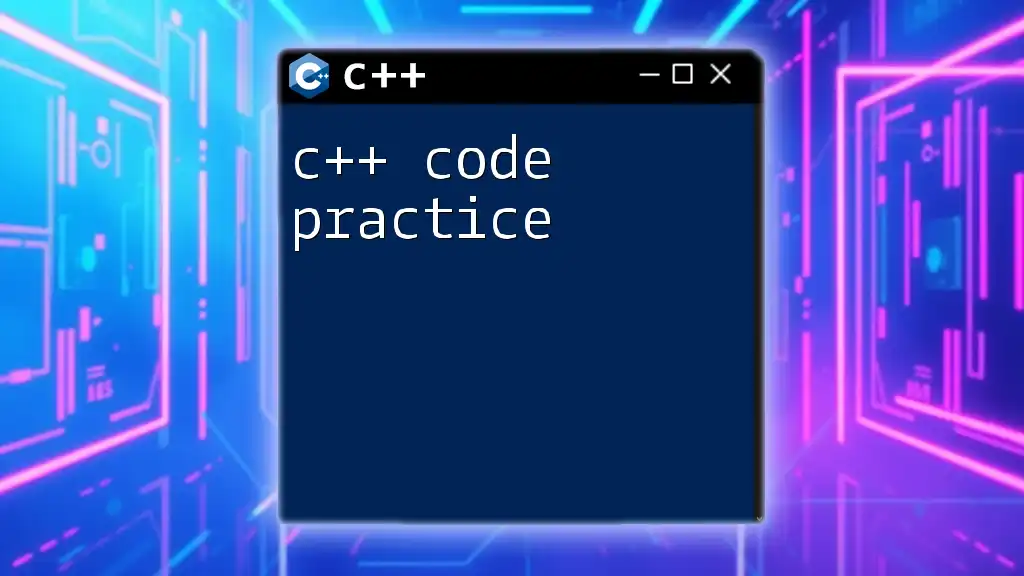
Basic Formatting Rules for C++
Indentation
Consistent indentation is one of the cornerstones of good C++ code formatting. Proper indentation helps highlight code blocks and dictates the logical flow. A common practice is to indent using either tabs or spaces, but it's crucial to select one and remain consistent throughout your code. Poor formatting can lead to confusion, as shown in the following examples:
// Poorly formatted
if(a>b) {
doSomething();
}
// Well-formatted
if (a > b) {
doSomething();
}
In the first snippet, the lack of indentation makes it difficult to see the hierarchy of the code, while the second version provides clarity and logical structure.
Line Length
Maintaining a reasonable line length (typically between 80-120 characters) can significantly improve code readability. When lines exceed this limit, they can wrap in editors, making it harder to read. To enhance clarity, long lines can be split into multiple lines by breaking at logical points. For instance:
// Excessive length on one line
if (someLongFunctionName(argument1, argument2, argument3, argument4, argument5)) {
doSomething();
}
// Improved formatting
if (someLongFunctionName(argument1, argument2,
argument3, argument4,
argument5)) {
doSomething();
}
Whitespace Usage
The effective use of whitespace can greatly enhance readability. Here are a few guidelines:
- Use spaces around operators (`+`, `-`, `=`, etc.) and after commas.
- Ensure there's space between control keywords and parentheses.
Avoid cluttering your code with too many spaces. Consider the following examples of proper and improper whitespace usage:
// Improper
for(int i=0;i<10;i++) { performAction(i); }
// Proper
for (int i = 0; i < 10; i++) {
performAction(i);
}
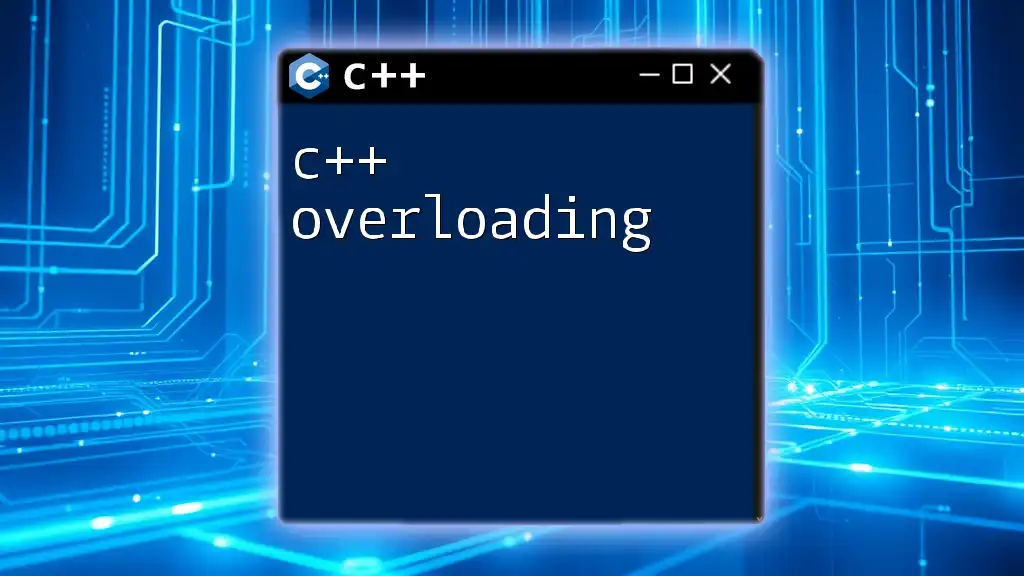
Advanced Formatting Techniques
Bracing Styles
Different programming teams often adopt different bracing styles. The two popular styles include:
- K&R Style: The opening brace is on the same line.
- Allman Style: The opening brace starts on a new line.
Visualizing these styles can help you decide which to use, as shown below:
K&R Style Example
if (condition) {
execute();
}
Allman Style Example
if (condition)
{
execute();
}
Commenting Code
Good comments are essential for providing context and explaining complex logic. Always strive for clear and concise comments. Systematic commenting can involve:
- Inline comments that describe single lines or operations.
performAction(i); // Perform action on index 'i'
- Block comments that explain larger portions of code or higher-level concepts.
/*
This function performs a specific action based on the value of 'index'.
It is essential for ensuring user input is processed correctly.
*/
void performAction(int index) {
// ...
}
Function Formatting
Structuring function definitions clearly is vital. Each function should have a clear purpose, and its signature should be descriptive enough to communicate its functionality. The parameters should be named meaningfully, enhancing the understandability of the code. For example:
// Poorly named function
void f(int a, int b) {
// Do something
}
// Well-named function
void calculateSum(int firstNumber, int secondNumber) {
// Calculate the sum of two numbers
}
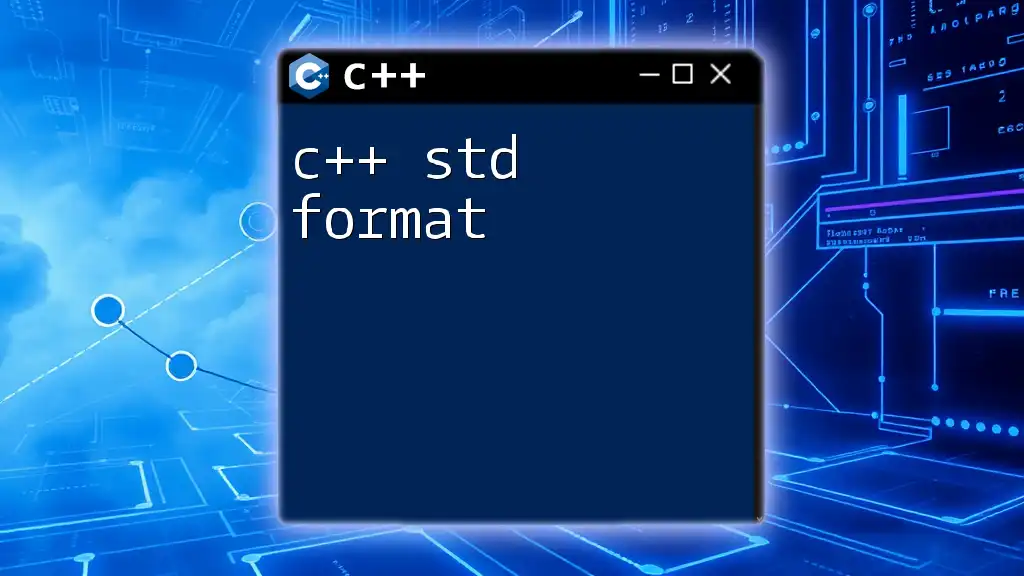
Using C++ Code Formatter Tools
What is a C++ Code Formatter?
A C++ code formatter is a tool designed to automatically format your source code according to specific style rules. This automation ensures consistency across different segments of code and saves developers time spent on manual formatting.
Popular C++ Code Formatters
Among the most popular tools are ClangFormat and Astyle. Both support custom configuration files and can be integrated into various editors and IDEs.
- ClangFormat: This tool offers extensive configurability and allows you to adhere to style guidelines like Google’s C++ style guide. It's particularly useful in larger codebases.
- Astyle: Known for its simplicity, Astyle provides straightforward configuration options to set your preferred formatting style for tabs and spaces.
How to Configure a Code Formatter
Configuring a code formatter typically involves creating a configuration file (like `.clang-format` for ClangFormat) where you define your preferred styles. An example configuration could look like this:
BasedOnStyle: Google
IndentWidth: 4
ColumnLimit: 120
UseTab: Never
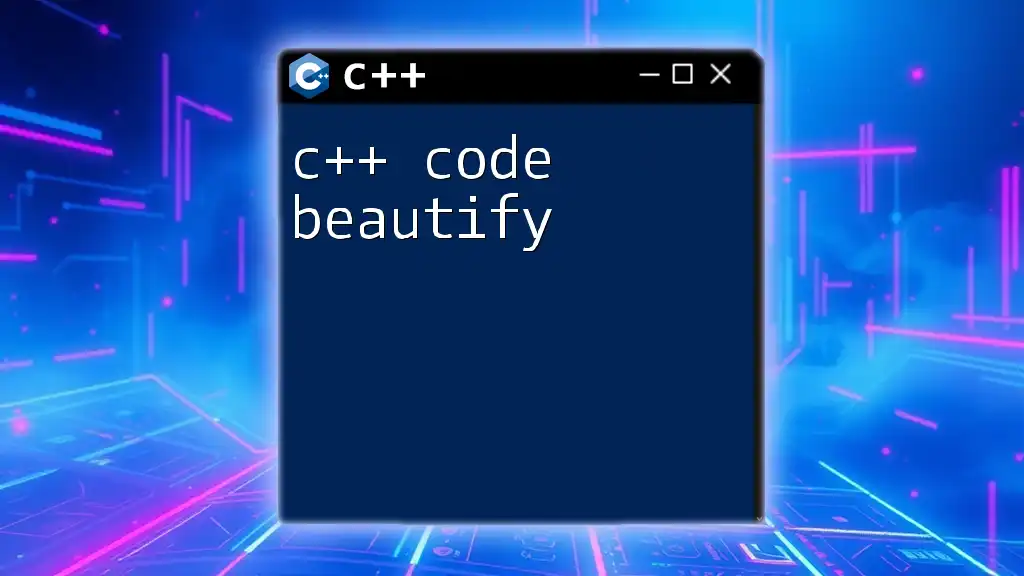
Best Practices for C++ Code Formatting
Consistency is Key
Consistency in code formatting enhances collaboration and reduces the cognitive load when reading code. Adopting a particular style guide—like Google's or LLVM’s—can help ensure everyone on your team is on the same page.
Utilizing a Code Review Process
Incorporating formatting checks into the code review process helps maintain standards. Reviewers should check for formatting issues alongside logic and functionality, fostering a culture of quality.
Making Use of IDE Features
Many modern Integrated Development Environments (IDEs) offer built-in formatting features. Familiarizing yourself with these functionalities can save precious time. For instance, Visual Studio and Code::Blocks have formatting options that can be applied to entire files or selected code blocks quickly.
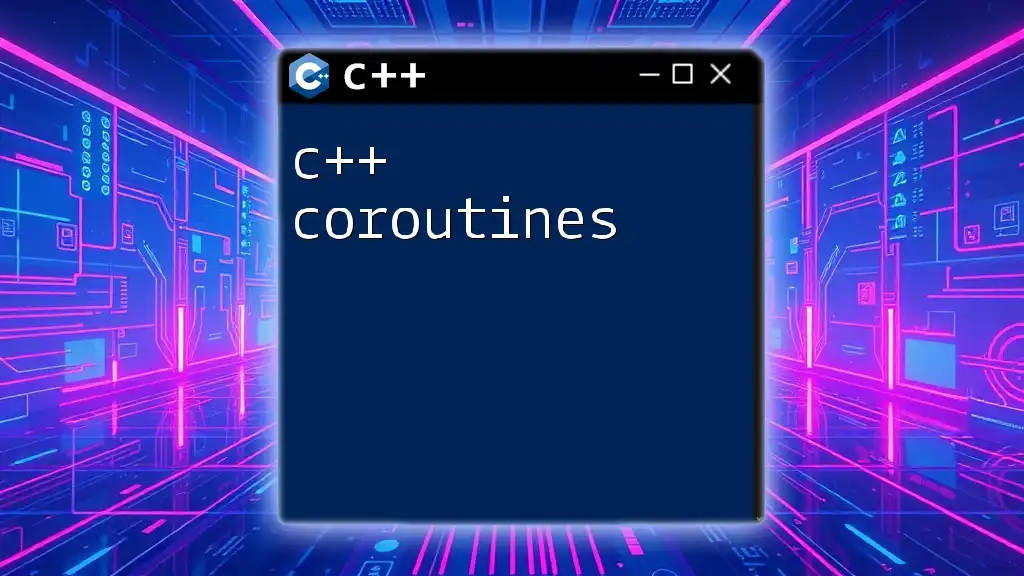
Conclusion
Implementing proper C++ code formatting is more than just making code look good; it’s about improving readability, maintaining organization, and enhancing collaboration. By adhering to established formatting rules and using available tools, developers can create code that is not only functional but also clean and professional. Make the choice to adopt robust code formatting practices now, and it will pay dividends in your programming career.