C++ declaration defines a variable, function, or class by specifying its name and type without allocating memory or implementing functionality.
int myNumber; // Declares an integer variable named myNumber
Understanding Declarations
What is a Declaration?
A declaration in C++ is a statement that introduces a name into the program, indicating the type of the entity being declared. It informs the compiler about the existence and type of variables, functions, classes, and more. Importantly, a declaration does not allocate memory or define the entity; that’s reserved for definitions.
In C++, it's crucial to note the difference between a declaration and a definition. While a declaration specifies the type and name, a definition also allocates memory. For instance, declaring a variable does not allocate memory until it is defined.
Types of Declarations
C++ comprises various types of declarations, each serving different purposes:
- Variable Declarations: Introduce variables.
- Function Declarations: Define functions.
- Class Declarations: Introduce user-defined types.
- More on Data Types: Specify different data storage types.
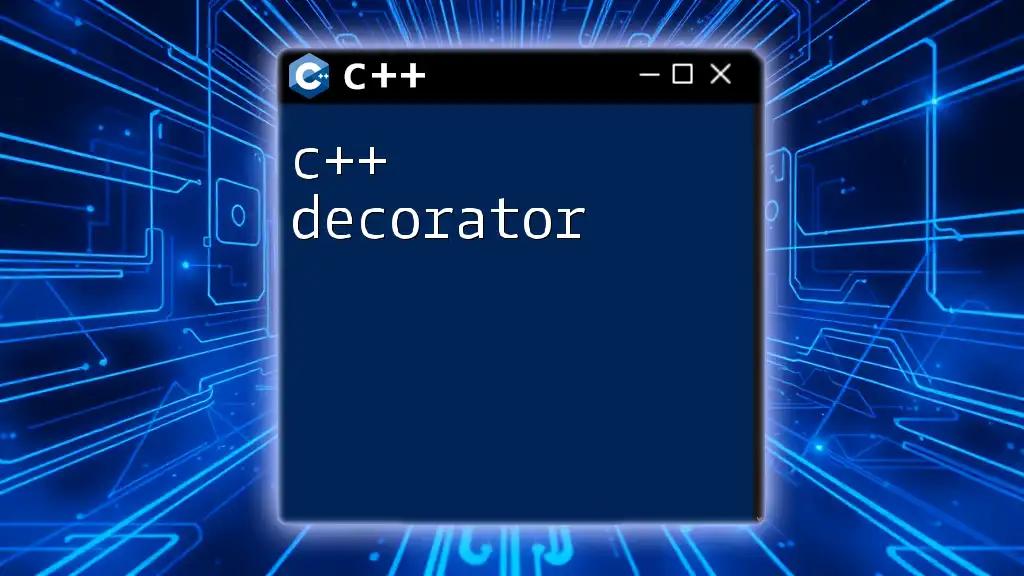
Variable Declarations
Basic Syntax
To declare a variable in C++, you must specify its type followed by its name. For example:
int x;
In this instance, `int` indicates that `x` is an integer variable. Understanding the syntax is essential as it sets the foundation for variable manipulation in your programs.
Initialized Declarations
You can also declare and initialize a variable simultaneously, which provides a value right from the start. For example:
int y = 10;
This line not only declares `y` as an integer but also initializes it to the value of 10. Initialization is a fundamental practice that prevents unintended behaviors due to using uninitialized variables.
Multiple Declarations
C++ allows you to declare multiple variables in a single line, which can enhance code readability and conciseness. Here's how you can do that:
int a = 5, b = 10, c = 15;
In this case, three integer variables are declared and initialized at once, which is particularly useful for related variables.
Scope and Lifetime
Understanding the scope and lifetime of variables is critical in managing memory effectively. A local declaration is limited to the block in which it is defined, whereas a global declaration persists throughout the program. Here’s a quick example:
int globalVar; // Global declaration
void function() {
int localVar; // Local declaration
}
In this example, `globalVar` can be accessed anywhere in the program, while `localVar` is restricted to `function()`. Consequently, managing variable scope wisely can prevent errors and enhance code clarity.
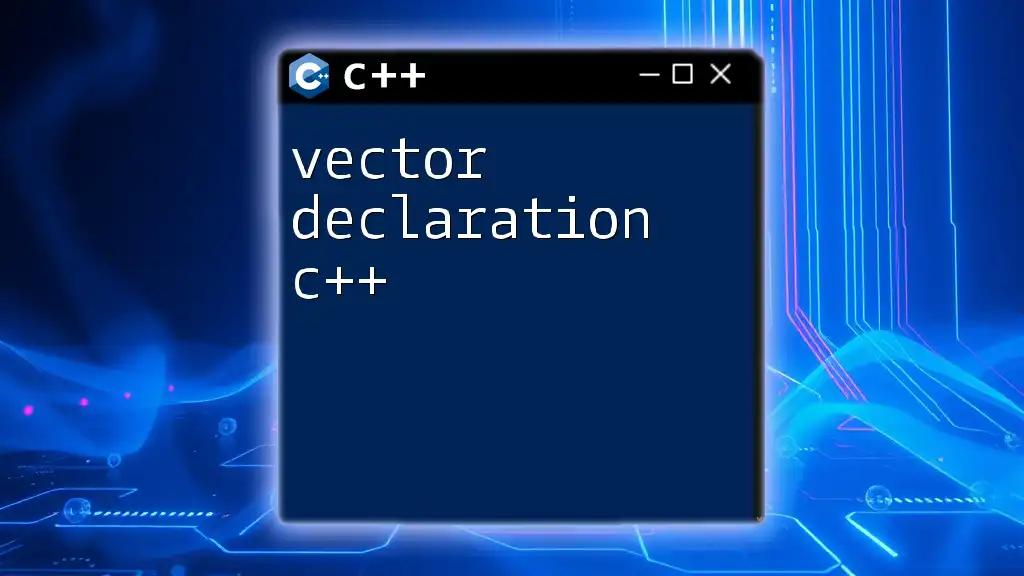
Function Declarations
Function Declaration Basics
A function declaration introduces a function's name, return type, and parameters, allowing the compiler to know about the function before its actual definition appears. Here’s an example:
void myFunction();
This snippet declares a function named `myFunction` that does not return any value (void).
Return Types and Parameters
It’s crucial to specify not only the return type but also any parameters that the function will accept. For instance:
int add(int a, int b);
This declaration indicates that the `add` function takes two integer parameters and returns an integer. Each parameter's type must be defined, allowing for type-checking during compilation.
Function Overloading
C++ supports function overloading, enabling the definition of multiple functions with the same name differentiated by parameter types and/or counts. Take a look at this example:
int add(int a, int b);
double add(double a, double b);
In this case, two `add` functions exist: one for integers and another for doubles. This capability enhances code usability and readability.

Class Declarations
Introduction to Classes
A class acts as a blueprint for creating objects (instances), encapsulating data and functions. By declaring a class, you define a custom type to model real-world entities.
Basic Syntax of Class Declaration
The syntax to declare a class begins with the `class` keyword followed by the class name and the class body within curly braces. Here’s an example:
class MyClass {
public:
void myMethod();
};
In this declaration, `MyClass` is defined with one public method `myMethod`. The visibility of class members (private, public, protected) significantly affects their accessibility from outside the class.
Member Variables and Methods
You can include member variables and methods within a class declaration. Here’s an example:
class MyClass {
private:
int x;
public:
void setX(int val);
};
In this case, `MyClass` has a private integer member `x` and a public method `setX` to set its value. This encapsulation characteristic of classes helps in maintaining control over data access and modifications.
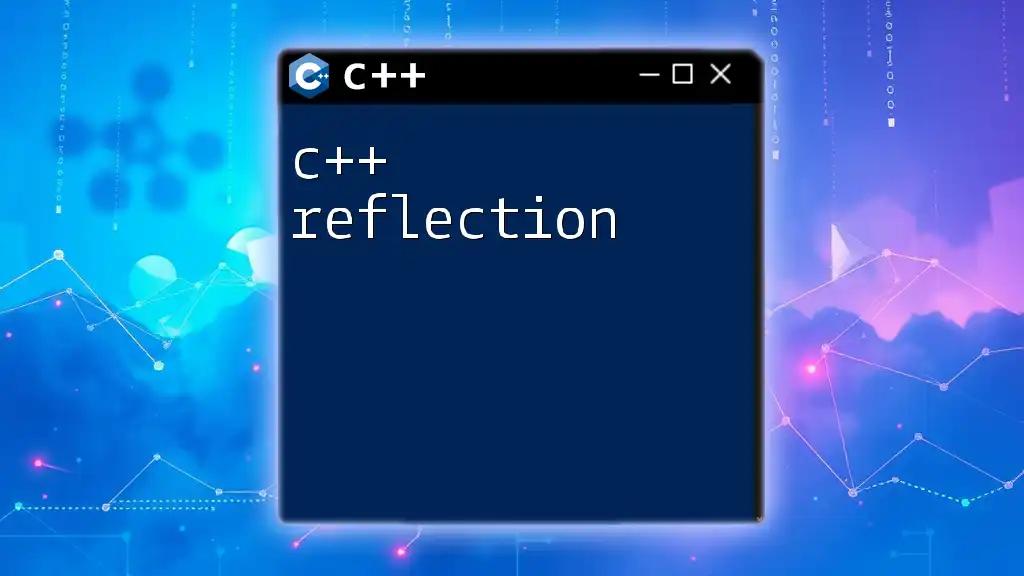
Advanced Declaration Concepts
Forward Declarations
Forward declarations are useful when a program involves circular dependencies between classes or functions. It allows one to declare a class or function before its complete definition appears. For example:
class MyClass; // Forward declaration
This technique informs the compiler that `MyClass` will be defined later, thereby avoiding compilation errors.
Static Declarations
In C++, declaring a variable as static changes its storage duration to static, meaning it retains its value between function calls. Here’s an example:
static int myStaticVar;
A static variable can only be accessed within the same file if declared at file scope, leading to encapsulation and preventing namespace pollution.
Const and Volatile Declarations
The keywords const and volatile play a crucial role in any declaration. A constant value cannot be altered after its declaration, whereas a volatile variable can change unexpectedly outside the control of the program. For instance:
const int maxLimit = 100;
volatile int sensorData;
In this example, `maxLimit` cannot be modified, while `sensorData` may vary due to hardware changes, emphasizing the need for careful consideration when using these qualifiers.

Conclusion
C++ declarations are a fundamental aspect of the language, forming the basis for variables, functions, and classes. By adhering to good declaration practices, you can write more maintainable, understandable, and efficient codes. Regular practice and experimentation will help solidify your grasp of this essential concept, leading to mastery in your C++ journey.

Further Reading and Resources
For those looking to deepen their understanding of C++ declarations, consider exploring recommended books, online courses, and official documentation. Engaging with community forums and support can also provide valuable insights and assistance as you refine your skills.