In C++, declaring an object involves specifying the object type followed by its name, allowing you to instantiate that object for use in your program.
Here's a simple example:
class MyClass {
public:
void display() {
std::cout << "Hello, World!" << std::endl;
}
};
// Declaring an object of MyClass
MyClass myObject;
Understanding Objects in C++
What is an Object in C++?
An object in C++ is an instance of a class. A class serves as a blueprint, defining the properties (data members) and behaviors (member functions) that its objects will have. When a class is declared, memory is allocated only for the class itself. It’s when we create an object from this class that memory is allocated for its attributes.
For example, consider a class named `Car`:
class Car {
public:
std::string model;
int year;
void displayInfo() {
std::cout << "Model: " << model << ", Year: " << year << std::endl;
}
};
Here, `Car` is a class, and an instance of `Car`, such as `myCar`, can hold specific data for `model` and `year`.
The Role of Constructors
Constructors are special member functions used to initialize objects of a class. A constructor is called when an object is created, and it can have parameters for customizable initialization.
For instance, the `Car` class can be enhanced with a constructor:
class Car {
public:
std::string model;
int year;
Car(std::string m, int y) : model(m), year(y) {}
void displayInfo() {
std::cout << "Model: " << model << ", Year: " << year << std::endl;
}
};
In this code, when we create a `Car` object, we can specify its model and year.
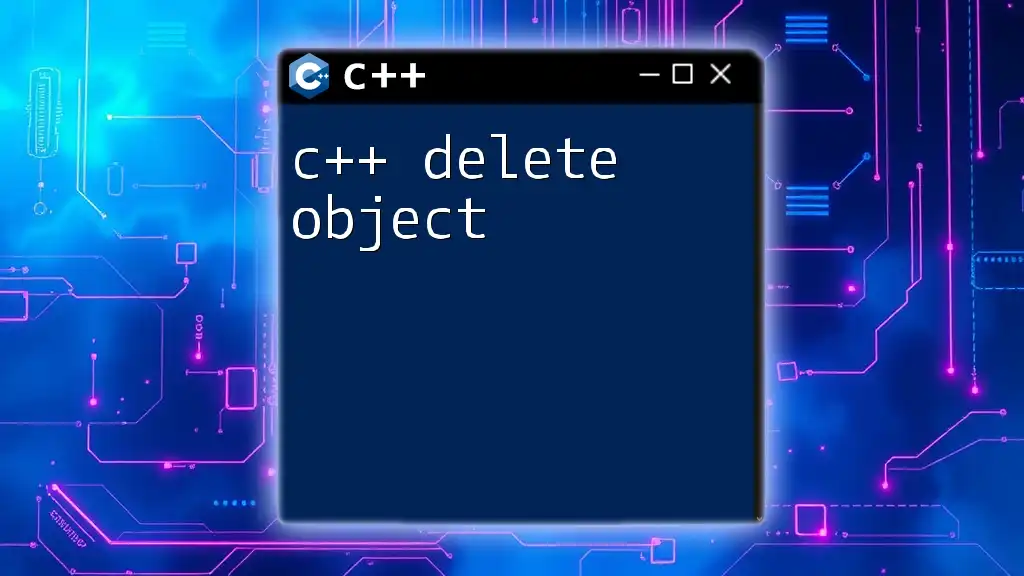
How to Declare an Object in C++
The Syntax for Declaring an Object
To declare an object in C++, you simply specify the class name followed by the object name. The basic syntax looks like this:
ClassName objectName;
For example:
Car myCar;
Creating an Object Using the Default Constructor
If a class has a default constructor (a constructor with no parameters), you can create an object without passing any arguments:
class Car {
public:
std::string model;
int year;
Car() { // Default constructor
model = "Unknown";
year = 0;
}
};
Car myDefaultCar; // Created using default constructor
Creating an Object with Parameters
When using a parameterized constructor, pass arguments during the object creation:
Car myCar("Toyota", 2021); // Created using a parameterized constructor
This effectively initializes `myCar` with the model "Toyota" and the year 2021.
C++ Declare Object: Stack vs Heap
Understanding where objects are allocated is crucial. Objects can be allocated on the stack or the heap, affecting their lifespan and memory management.
Stack Allocation: Objects declared on the stack are automatically destroyed when they go out of scope. For example:
void function() {
Car myStackCar("Honda", 2020); // Stack allocation
} // myStackCar is destroyed here
Heap Allocation: With dynamic memory allocation using the `new` keyword, objects can persist beyond the scope they were created in:
Car* myHeapCar = new Car("Ford", 2019); // Heap allocation
Remember to deallocate memory when it’s no longer needed:
delete myHeapCar; // Freeing the allocated memory
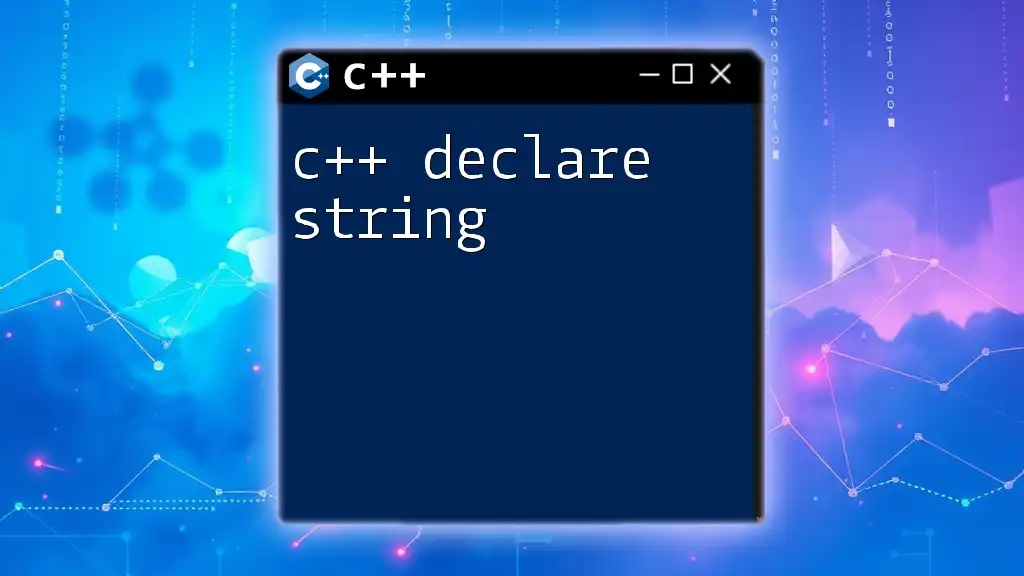
How to Make an Object in C++
Understanding Object Initialization
When creating an object, initializing its members is crucial to prevent undefined behavior. C++ offers various methods to initialize attributes upon object creation, including:
-
Direct Initialization
- This is used when constructor syntax is employed.
-
Copy Initialization
- Example:
Car anotherCar = myCar; // Copy constructor invoked
-
List Initialization (C++11 and later)
- Example:
Car myListCar{"Nissan", 2022};
Using C++11 Features
Modern C++ features enable more efficient object creation with smart pointers, improving memory management:
Using `std::make_unique` (for unique pointers):
#include <memory>
std::unique_ptr<Car> myUniqueCar = std::make_unique<Car>("BMW", 2023);
Common Mistakes When Creating Objects
Not Initializing Objects
Uninitialized objects can lead to unpredictable program behavior. Always ensure that your objects are initialized properly when declared. For example, failing to initialize an object might leave attributes with garbage values, leading to erroneous outputs.
Copying Objects Incorrectly
When copying objects, understanding how the copy constructor and assignment operator works is crucial. A common pitfall is performing shallow copies which may lead to shared pointers referencing the same memory location.
Example of shallow vs deep copy:
class Person {
public:
std::string name;
// Shallow copy (default behavior)
Person(const Person& other) : name(other.name) {}
};
// Deep copy implementation
class PersonDeep {
public:
std::string* name;
PersonDeep(const std::string& n) {
name = new std::string(n);
}
~PersonDeep() {
delete name;
}
PersonDeep(const PersonDeep& other) {
name = new std::string(*other.name); // Deep copy
}
};
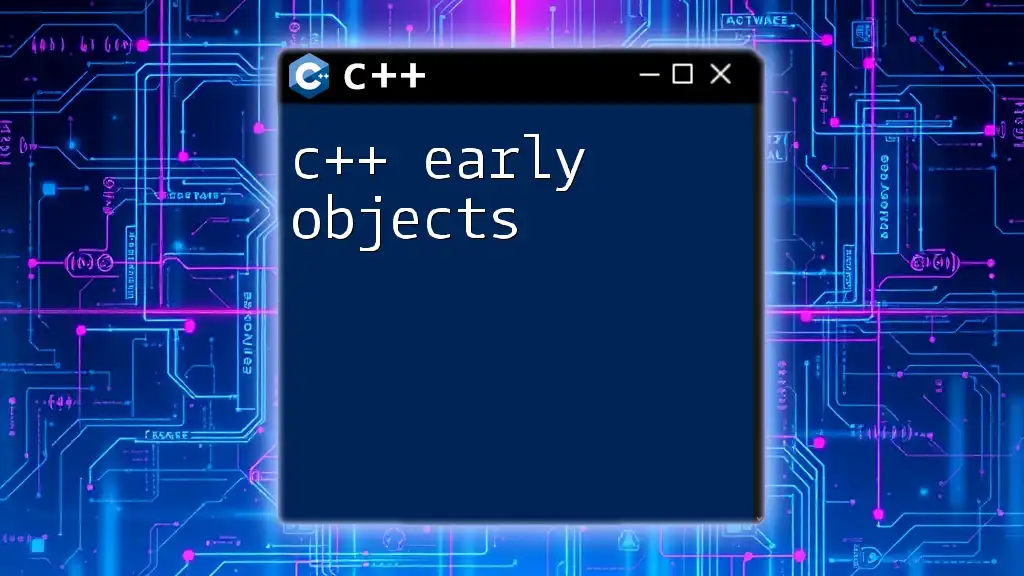
Advanced Object Creation Techniques
Aggregation and Composition
These are design patterns to manage object relationships. In aggregation, an object can exist independently, while in composition, the presence of the contained object depends on the containing object.
class Engine {
public:
Engine() {}
};
class CarWithEngine {
Engine engine; // Composition
public:
CarWithEngine() {}
};
Here, `CarWithEngine` contains `Engine` as part of its structure, and if `CarWithEngine` is destroyed, so is the `Engine`.
Polymorphic Behavior
Polymorphism allows objects to be treated as instances of their parent class. For this, virtual functions must be employed in the base class.
class Vehicle {
public:
virtual void sound() {
std::cout << "Generic vehicle sound" << std::endl;
}
};
class Car : public Vehicle {
public:
void sound() override {
std::cout << "Car beep!" << std::endl;
}
};
Vehicle* myVehicle = new Car();
myVehicle->sound(); // Outputs: Car beep!
delete myVehicle; // Clean up
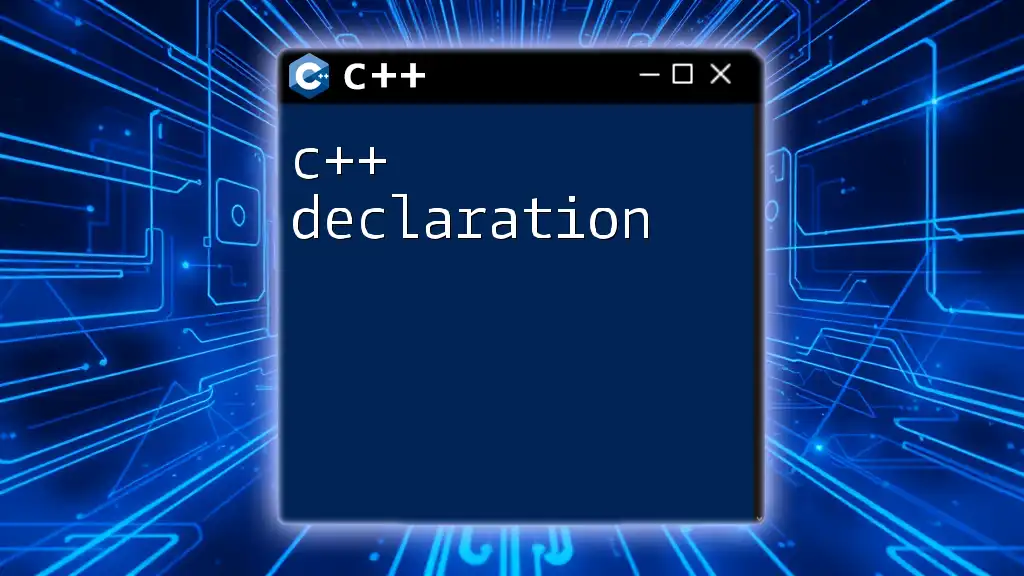
Conclusion
In this comprehensive guide, we explored the intricacies of how to c++ declare object by discussing the definitions, syntax, initialization methods, and advanced techniques such as polymorphism and memory management. Understanding these concepts is essential to leveraging the full power of C++ and enhancing your programming skills.
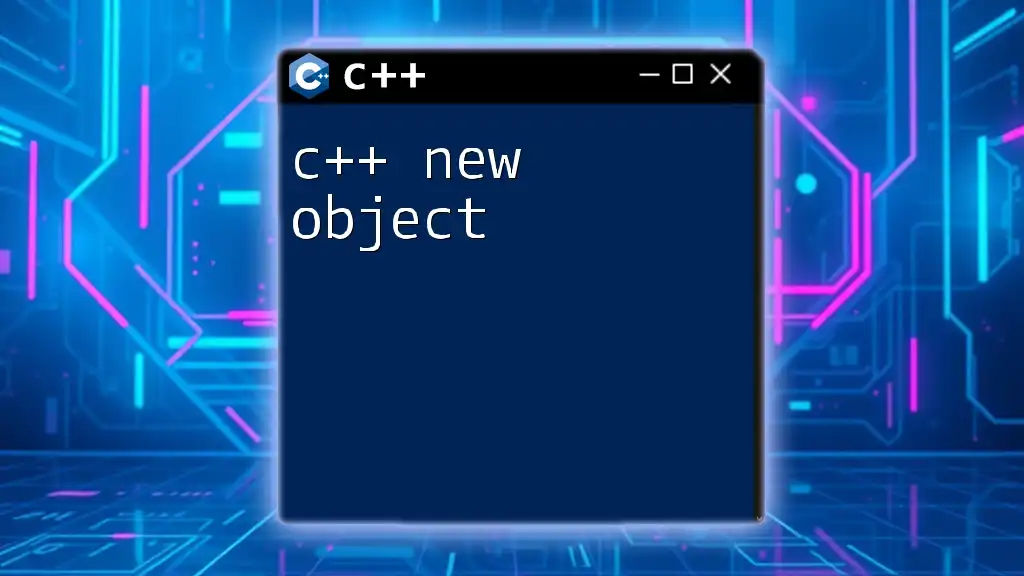
Additional Resources
For further learning, certain books and online resources can be beneficial. Exploring programming platforms with practical exercises can reinforce your skills as you apply what you've learned about creating objects in C++.
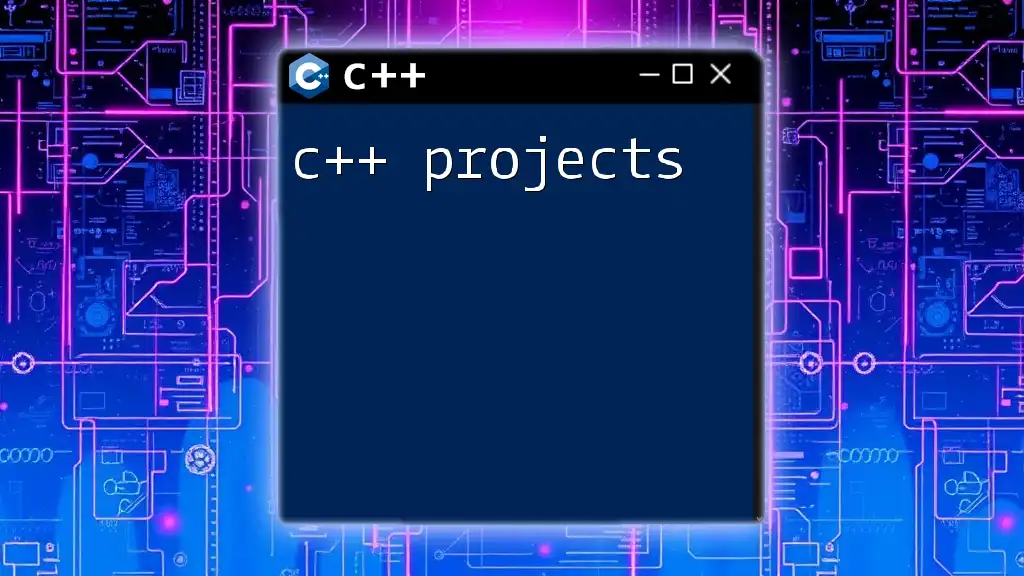
Call to Action
Feel free to subscribe for more insightful C++ tutorials, and share your experiences or questions regarding object creation in the comments!