In C++, the `new` operator is used to dynamically allocate memory for an object or an array, returning a pointer to the allocated space.
// Example of creating a new object of a class called 'MyClass'
MyClass* obj = new MyClass();
Understanding C++ Objects
What is an Object in C++?
An object in C++ is an instance of a class, which represents a data structure that can contain both data and functions. Classes provide a blueprint for creating objects, encapsulating attributes and behaviors relevant to the specific type of object. Objects are fundamental to object-oriented programming (OOP), allowing developers to model real-world entities and their interactions.
The relationship between objects and classes is crucial: a class defines the properties (attributes) and methods (functions) that an object can have. For instance, if we have a class named Car, an object would represent a specific car, such as a red Toyota Corolla.
The Role of Constructors
Constructors are special member functions that initialize objects of a class. They are invoked automatically when a new object is created and are essential for setting up an object's initial state.
In C++, there are several types of constructors:
- Default constructors: Initialize objects without any parameters.
- Parameterized constructors: Allow customization of an object's properties at creation.
- Copy constructors: Create a new object as a copy of an existing object.
Understanding constructors is crucial for effective object management, ensuring that objects start their life in a valid state.
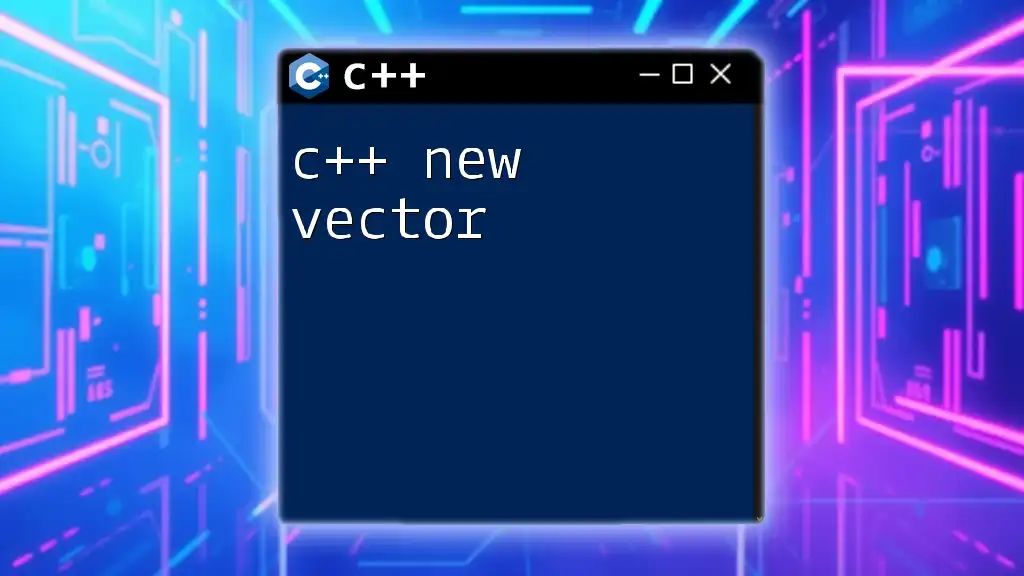
Creating a New Object in C++
Syntax for Creating New Objects
To create a new object in C++, you can use the following syntax:
className objectName;
This creates an object on the stack, which is automatically managed and destroyed when it goes out of scope. However, if you require an object to persist beyond the scope of its creator, you can allocate it on the heap using the `new` operator:
className* objectName = new className();
Objects allocated on the heap must be explicitly destroyed using `delete` to prevent memory leaks.
Basic Example of a New Object
Here’s a simple example demonstrating how to create an object:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Dog myDog; // Stack allocation
myDog.bark();
return 0;
}
In this example, we define a `Dog` class with a `bark` method that prints "Woof!". In the `main` function, we create a `Dog` object named `myDog` on the stack and call its `bark` method. The object `myDog` is automatically destroyed when it goes out of scope.
Using the `new` Operator
Using the `new` operator facilitates dynamic memory allocation. This allows objects to remain in existence until you decide to delete them.
Advantages of Dynamic Memory Allocation
Creating objects on the heap can be useful when:
- You do not know the number of objects you wish to create at compile time.
- You need objects to persist outside the function scope where they were created.
Code Snippet: Creating an Object with `new`
Dog* myDog = new Dog(); // Heap allocation
myDog->bark();
delete myDog; // Freeing the allocated memory
In this example, a `Dog` object is created on the heap. We utilize the `->` operator to call the `bark` method on this pointer. Once done, it's crucial to call `delete` to free the allocated memory and prevent memory leaks.
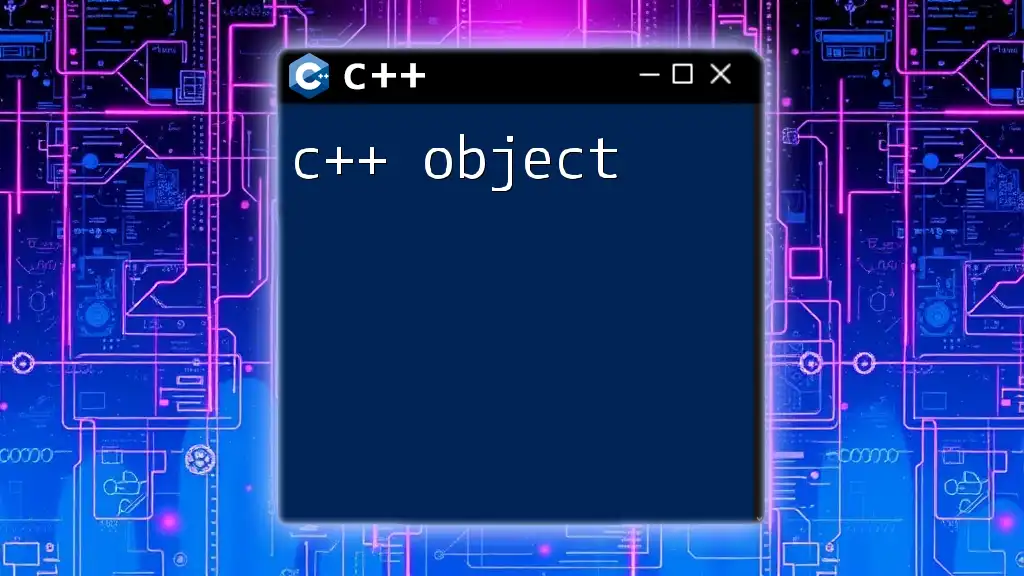
Working with Object Properties and Methods
Accessing Properties
In C++, class members can be declared as public or private. Public members can be accessed from outside the class, while private members cannot. This encapsulation forms a core principle of OOP.
To access and modify properties, you typically define getter and setter methods to control how values are accessed and modified.
Code Snippet: Using Properties in an Object
class Cat {
public:
std::string name;
void meow() {
std::cout << name << " says Meow!" << std::endl;
}
};
int main() {
Cat myCat;
myCat.name = "Whiskers";
myCat.meow();
return 0;
}
Here, we define a `Cat` class with a public property `name` and a method `meow()`. We create an instance of `Cat`, set the `name`, and call `meow()` to display its value. This demonstrates how to work with object properties effectively.
Invoking Methods on New Objects
Object methods can be invoked using the dot operator (`.`) for stack-allocated objects and the arrow operator (`->`) for heap-allocated objects. It’s important to ensure methods are adapted to their context, especially regarding const-correctness, meaning you should define methods that do not modify member variables as `const`.
Code Snippet: Complex Method Manipulation
class Bird {
public:
void fly() {
std::cout << "Flapping wings!" << std::endl;
}
};
int main() {
Bird* sparrow = new Bird();
sparrow->fly();
delete sparrow;
return 0;
}
In this snippet, we create a `Bird` class with a `fly()` method. The object `sparrow` is dynamically allocated and flies before being deleted, ensuring unmanaged memory does not accumulate.
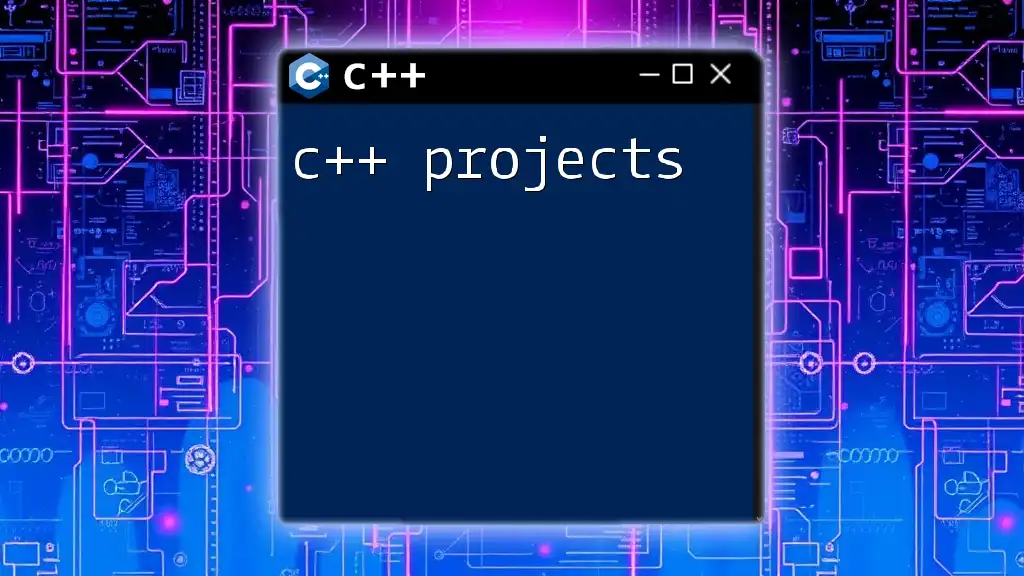
Best Practices for Creating Objects
Memory Management Considerations
Memory management is a critical aspect of C++ programming. Whenever you allocate memory using `new`, you must be diligent about releasing that memory with `delete`. Failing to do so can lead to memory leaks, progressively diminishing available memory.
Introduction to Smart Pointers
With modern C++, it’s advisable to utilize smart pointers provided in the C++11 standard. Smart pointers automate memory management and help prevent memory leaks by ensuring that memory is freed when the smart pointer goes out of scope.
Types of smart pointers include:
- `std::unique_ptr`: Represents sole ownership of an object, which automatically deletes the object when the pointer goes out of scope.
- `std::shared_ptr`: Represents shared ownership of an object, with multiple pointers capable of managing the same resource.
- `std::weak_ptr`: A non-owning smart pointer that does not affect the reference count of a `std::shared_ptr`.
Code Snippet: Using Smart Pointers
#include <memory>
int main() {
std::unique_ptr<Dog> puppy = std::make_unique<Dog>();
puppy->bark();
// No need to manually delete, memory is automatically managed
return 0;
}
In this example, a `std::unique_ptr` is used to manage the `Dog` object. When `puppy` goes out of scope at the end of `main`, the object is automatically deleted, making memory management straightforward and safe.
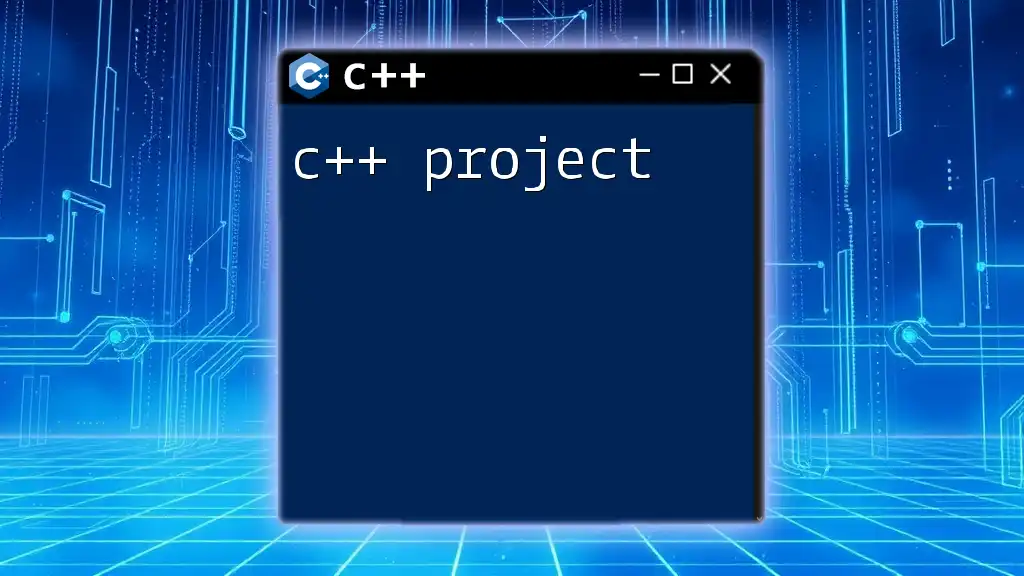
Conclusion
Recap of Key Concepts
Creating a new object in C++ involves understanding not only the syntax but also the underlying principles of management and memory allocation. By grasping constructors, stack vs. heap allocation, and best practices, you can effectively create and manipulate objects in your programs.
Call to Action
With this guide, you should feel empowered to practice creating objects in C++. Explore further resources on advanced C++ programming to solidify your understanding and use of objects effectively in your applications. Happy coding!