C++ object composition is a design principle where a class is composed of one or more objects from other classes, allowing for code reuse and better organization of complex behaviors.
Here’s a simple code snippet illustrating object composition in C++:
#include <iostream>
#include <string>
class Engine {
public:
void start() {
std::cout << "Engine started." << std::endl;
}
};
class Car {
private:
Engine engine; // Composition: Car has an Engine
public:
void start() {
engine.start(); // Delegating the start action to the Engine
std::cout << "Car is ready to go!" << std::endl;
}
};
int main() {
Car myCar;
myCar.start();
return 0;
}
Understanding C++ Object Composition
What is Object Composition in C++?
C++ object composition is a design principle allowing developers to create complex types by combining simpler objects. Instead of creating a new class through inheritance, object composition allows a class to contain instances of other classes, leading to flexible and maintainable code structures.
This technique encourages code reuse, enhancing modularity and separation of concerns. It is particularly important in scenarios where you want to model real-world relationships between objects.
Differences Between Object Composition and Inheritance
Object composition and inheritance are both techniques for achieving code reuse, but they differ significantly. Inheritance establishes an "is-a" relationship (e.g., a Dog is an Animal), while composition creates a "has-a" relationship (e.g., a Car has an Engine).
Choosing when to use composition over inheritance is crucial for maintaining a clean and efficient design. If your design requires shared behavior among multiple classes, inheritance might be a suitable choice. However, if you want to create a more flexible architecture, composition is often preferable.
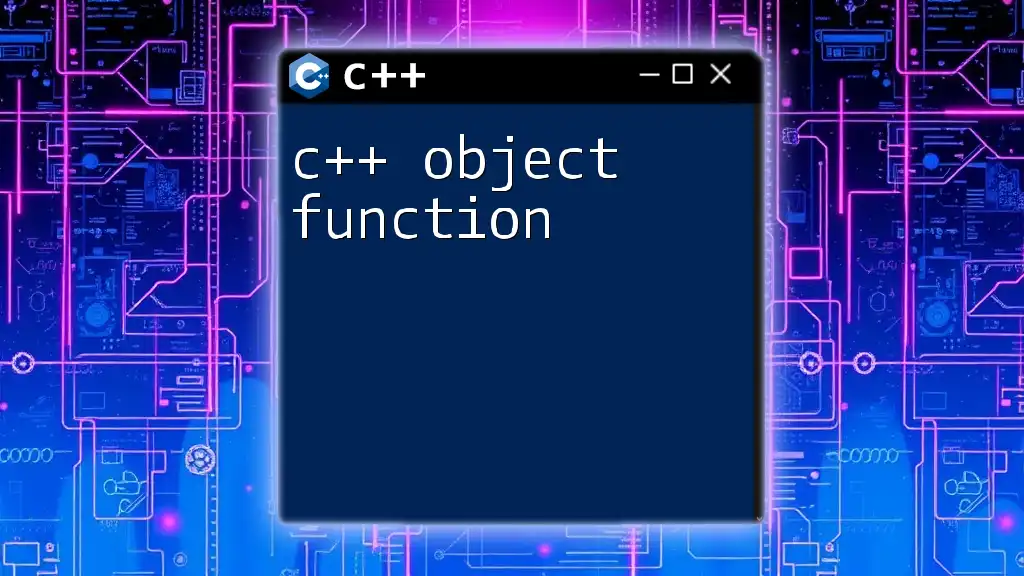
Key Concepts of Object Composition in C++
Components of Object Composition
In C++, objects often serve as components within a larger object. There are two main types of relationships to consider:
-
Aggregation represents a relationship where the contained objects can exist independently of the parent (e.g., a Classroom can contain Students, but those Students can exist independently).
-
Composition depicts a stronger relationship where the contained objects cannot exist without the parent (e.g., a Car has an Engine; without the Car, the Engine doesn’t function in this context).
Real-life examples illustrate these concepts well. Consider a Computer and its CPU, RAM, and Hard Drive. A Computer cannot function without these components, so we see a composition relationship.
Benefits of Using Object Composition
Employing object composition leads to various advantages:
- Code Reusability: By creating classes that encapsulate specific behaviors, these classes can be easily reused in different contexts.
- Improved Modularity: Each class is responsible for a specific piece of functionality, which promotes more manageable and scalable code.
- Enhanced Flexibility: Composition allows you to change or swap components without affecting the entire system, which can result in easier maintenance.
Real-world applications often embrace object composition to promote a clean separation of concerns, especially in larger software systems.
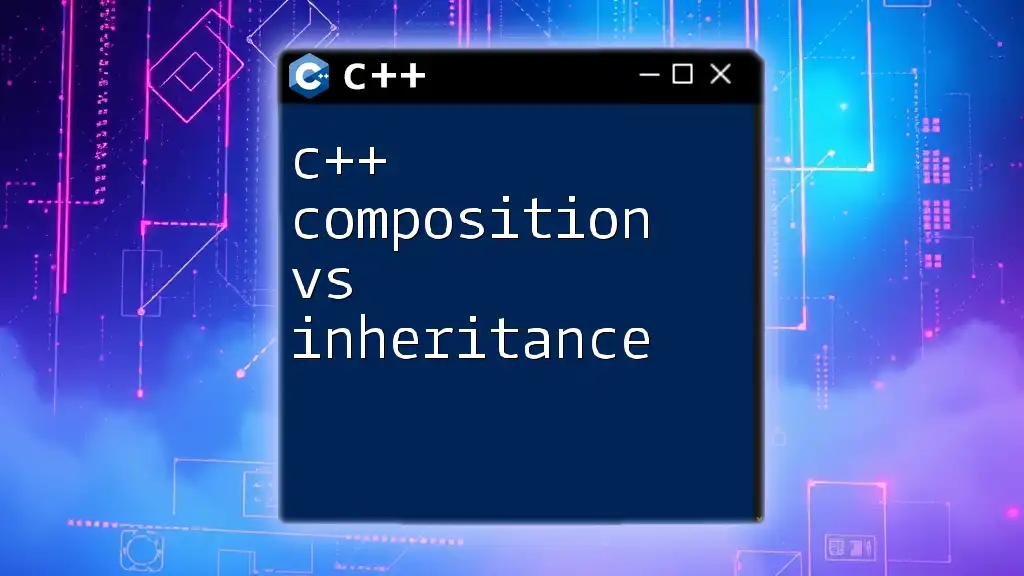
Implementing Object Composition in C++
Basic Syntax of Composition
To implement object composition, you first have to understand the basic class syntax in C++. When declaring classes for composition, you typically declare member variables as instances of other classes.
Example Code Snippet:
class Engine {
public:
void start() { /* Logic to start the engine */ }
};
class Car {
private:
Engine engine; // Car 'has-a' Engine
public:
void startCar() { engine.start(); }
};
In this example, the `Car` class contains an instance of the `Engine` class. The `startCar()` method demonstrates how the `Car` can delegate tasks to its composed `Engine`.
Step-by-Step Example of Object Composition
Defining Classes
Let’s delve deeper into a practical example by defining a `Book` and a `Library` class.
Example #1:
Defining `Book` and `Library` classes:
class Book {
private:
std::string title;
std::string author;
public:
Book(std::string t, std::string a) : title(t), author(a) {}
void displayInfo() {
std::cout << "Title: " << title << ", Author: " << author << std::endl;
}
};
class Library {
private:
std::vector<Book> books; // Library 'has-a' Collection of Books
public:
void addBook(const Book& book) {
books.push_back(book);
}
void displayBooks() {
for (auto& book : books) {
book.displayInfo();
}
}
};
In this code, the `Library` class contains a collection of `Book` objects, demonstrating the composition relationship clearly.
Utilizing Composition
Now, we can build functionalities to manage and display our books.
Functionality: Example code for adding and displaying books:
void Library::addBook(const Book& book) {
books.push_back(book);
}
void Library::displayBooks() {
for (auto& book : books) {
book.displayInfo();
}
}
This code allows the library to manage its collection of books effectively. You can easily add new books and display the entire collection.
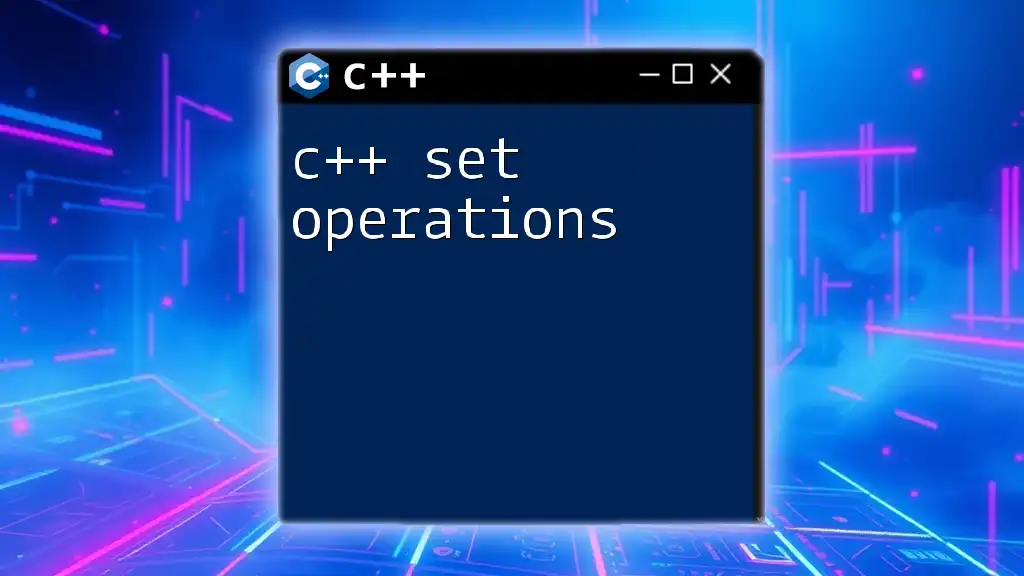
Best Practices for Object Composition
Keeping Classes Focused and Cohesive
One of the best practices when implementing object composition is adhering to the Single Responsibility Principle. Each class should have one reason to change, which means its functionality should be as focused and cohesive as possible. This results in improved maintainability.
When to Choose Object Composition
It's crucial to recognize scenarios where object composition is more beneficial than other design principles. For instance, if you're designing a complex system like a role-playing game, composition allows you to create reusable and interchangeable components (like different weapons, characters, and abilities).
Analyze your use cases carefully. Real test cases where composition reigns supreme often involve defining relationships that mirror real-world interactions between entities.
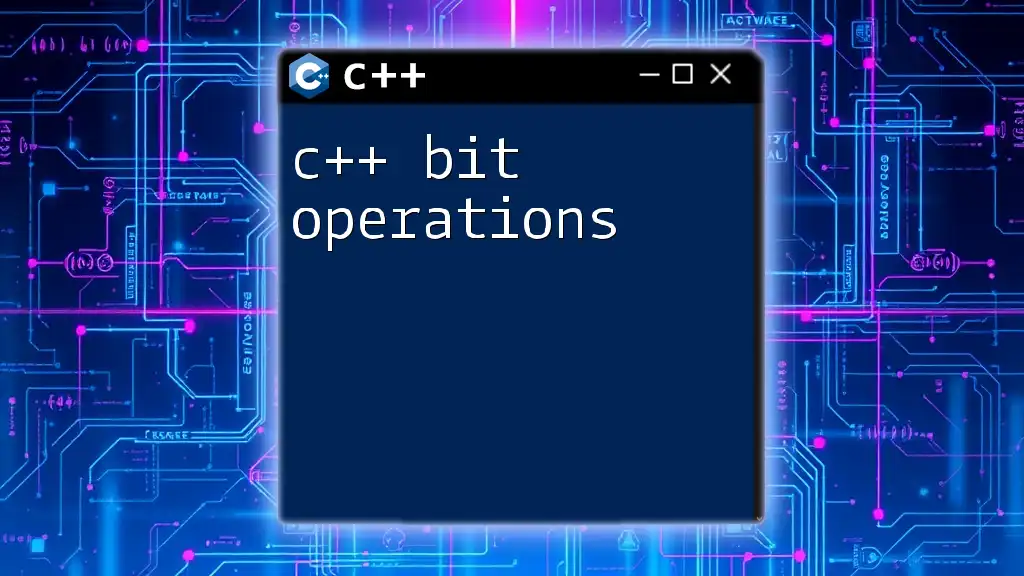
Common Pitfalls in Object Composition
Overusing Composition
While powerful, object composition can lead to extraordinary complexity if overused. It’s essential to strike a balance between components and understand when to keep things simple. Avoid creating too many small classes that increase the cognitive load on developers.
Lazy Initialization and Management
Another potential pitfall involves the lazy initialization of objects. In scenarios where a composed object might need to be created only upon first use, care must be taken to manage the life cycle of components accurately. For example, initializing an Engine inside a Car might be deferred until the Car is started; thus, handling that logic effectively is crucial.
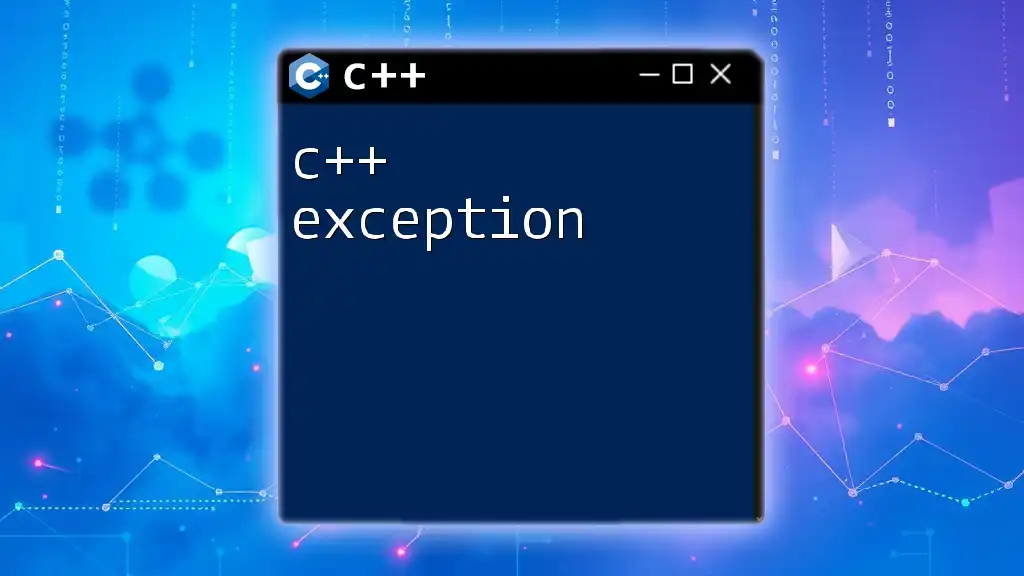
Conclusion
Recap of Object Composition in C++
C++ object composition provides a robust way to create flexible and maintainable architectures by allowing classes to contain other classes as members, leading to clearer abstractions.
Incorporating the discussed principles, benefits, and best practices will help you leverage this powerful design tool in your programming journey.
Additional Resources
To further deepen your understanding, consider exploring recommended books and online tutorials on C++ composition, as well as engaging with communities and forums where you can continue learning and asking questions pertaining to this vital technique in modern C++ programming.