In C++, an object function (commonly known as a member function) is a function that operates on the data members of its containing class and is invoked on an object of that class.
Here’s a simple example:
class Rectangle {
public:
int width, height;
int area() {
return width * height;
}
};
int main() {
Rectangle rect;
rect.width = 10;
rect.height = 5;
int rectArea = rect.area(); // Calls the object function
return 0;
}
Understanding the Basics of Object Functions
What Are Object Functions in C++?
C++ object functions, also known as member functions, are functions that are defined within a class and are designed to operate on the objects or instances of that class. They can access the class’s data members and can modify them as needed. This encapsulation of behavior and data is a core principle of object-oriented programming (OOP).
For example, consider the following class definition for a `Dog`:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
In this code snippet, `bark()` is an object function of the `Dog` class which outputs the string "Woof!" when called.
Characteristics of Object Functions
Understanding the characteristics of object functions is crucial for effective programming in C++.
-
Scope and Visibility: Object functions can have different access specifiers:
- Public: Accessible from outside the class.
- Protected: Accessible in the class and derived classes.
- Private: Accessible only within the class itself.
-
Static vs. Instance Methods:
- Instance Member Functions require an instance of the class to be invoked, whereas Static Member Functions belong to the class itself and can be called without an instance. Static functions cannot access non-static data members or functions.
C++ Function Objects
Introduction to Function Objects
The term "function object" or "functor" refers to an object that can be called as if it were an ordinary function. In C++, functors are implemented by overloading the `operator()` for a class or struct.
Syntax and Usage of Function Objects
Creating a function object is straightforward. You define a struct or class and overload the `operator()` method to specify the function's behavior.
struct Multiply {
int operator()(int a, int b) {
return a * b;
}
};
Multiply multiply;
std::cout << multiply(3, 4); // Outputs: 12
In this example, `Multiply` is a function object that can be called like a regular function to multiply two integers.
Advantages of Using Function Objects
Using function objects presents several advantages, including:
- Performance: In some scenarios, function objects can be more efficient than traditional function pointers due to inlining, which reduces the overhead of function calls.
- State Preservation: Unlike traditional functions, function objects can maintain state across multiple calls, allowing for more complex operations built into a single object.
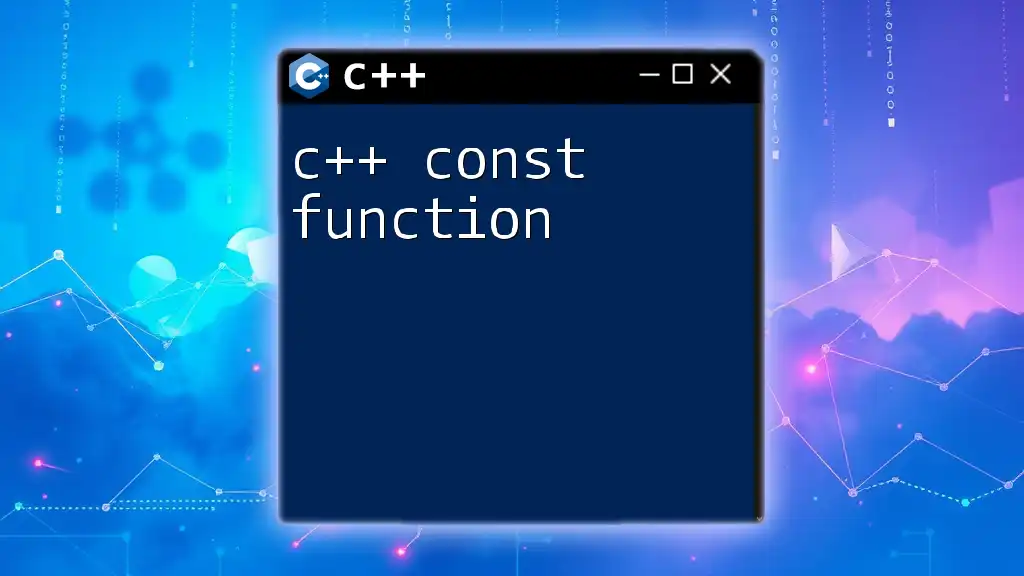
Defining and Calling Object Functions
Defining Member Functions
Defining member functions generally follows a simple syntax, as shown below:
class Cat {
public:
void meow() {
std::cout << "Meow!" << std::endl;
}
};
In this code snippet, `meow()` is defined inside the `Cat` class, and when called, it outputs "Meow!" to the console.
Calling Object Functions
To call an object function, you need an instance of its corresponding class. For example:
Cat cat;
cat.meow(); // Outputs: Meow!
Here, an instance of the `Cat` class named `cat` is created, and `meow()` is invoked, resulting in "Meow!" being printed.
Overloading Object Functions
C++ allows you to overload member functions, meaning you can have multiple functions with the same name but different parameter lists. This enhances flexibility and usability within your class.
class Printer {
public:
void print(int i) {
std::cout << i << std::endl;
}
void print(std::string s) {
std::cout << s << std::endl;
}
};
In the `Printer` class, the `print()` function is overloaded to handle both integers and strings, accommodating various print scenarios seamlessly.
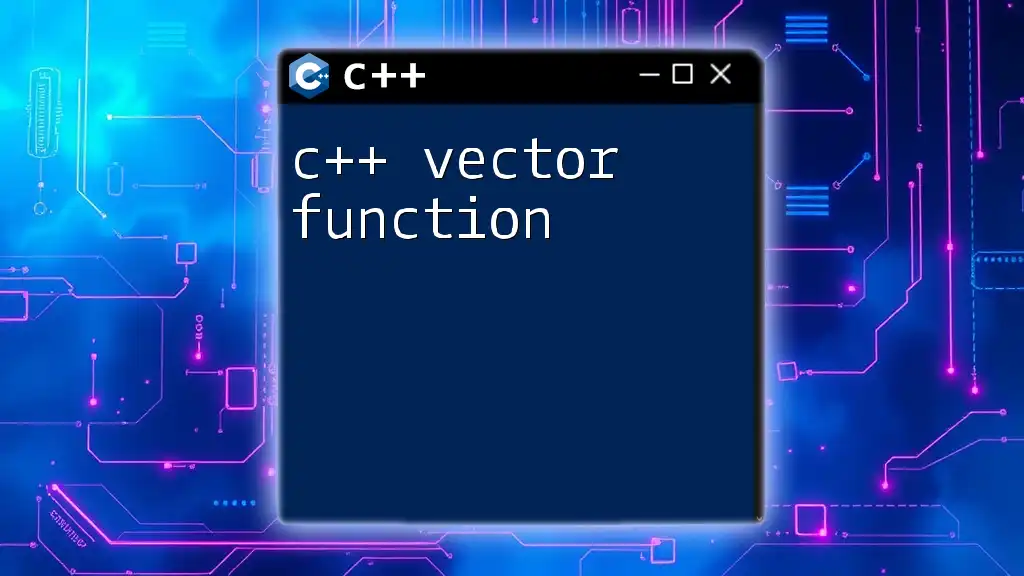
Advanced Concepts with Object Functions
Inline Member Functions
Inline member functions are defined within class definitions and are intended for performance optimization. The compiler attempts to replace function calls with the actual function code during compilation, reducing the overhead of function calls.
class Square {
public:
inline int area(int side) {
return side * side;
}
};
In this example, using the `inline` keyword conveys to the compiler that `area()` should be considered for inlining, which could result in improved efficiency, especially when the function is small and frequently called.
Virtual Functions and Polymorphism
Polymorphism is a powerful feature in object-oriented programming that allows for different implementations of the same function based on the object’s runtime type. This versatility is achieved through virtual functions.
class Base {
public:
virtual void show() {
std::cout << "Base class" << std::endl;
}
};
class Derived : public Base {
public:
void show() override {
std::cout << "Derived class" << std::endl;
}
};
In this example, `show()` is a virtual function in the `Base` class. The `Derived` class overrides `show()`, enabling the correct function to be executed based on the type of object invoking it.
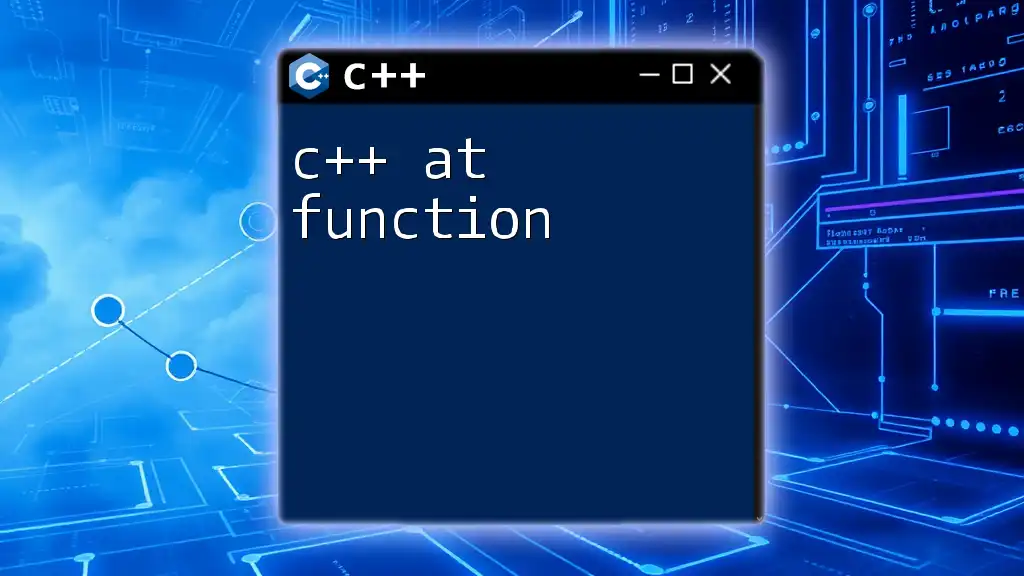
Best Practices for Using Object Functions
Code Readability and Maintenance
Writing clean, readable code is essential in software development. Encapsulating functionalities within object functions not only keeps the code organized but also aligns with the principles of OOP. Modular design allows complex tasks to be broken down into smaller, manageable functions, making debugging and maintenance easier.
Performance Considerations
When dealing with performance-sensitive code, passing objects by reference instead of by value is a recommended practice. This approach can significantly reduce unnecessary copies, especially for large objects.
void processLargeObject(const LargeObject& obj) {
// Processing obj...
}
Furthermore, it’s wise to consider the context when using inline functions and const member functions, as they can greatly impact performance and maintainability.
Recap of Key Points
To summarize the core concepts around C++ object functions:
- Object functions encapsulate behaviors within classes.
- Function objects, or functors, allow instances of classes to behave like functions.
- Proper understanding of member functions, overloading, and advanced concepts like polymorphism and inline functions is crucial for efficient C++ programming.
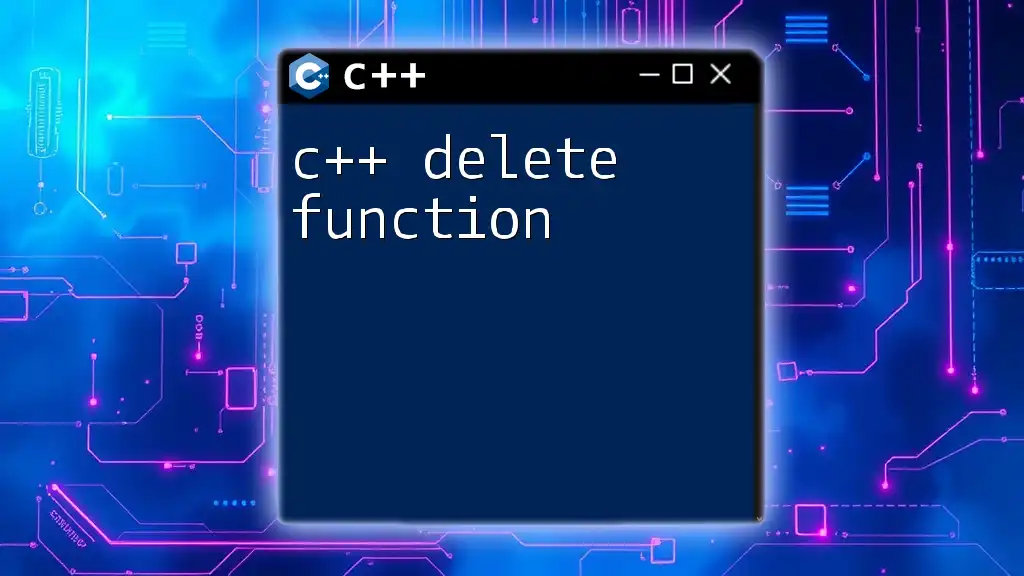
References
For further reading and deeper understanding of C++ object functions, consider exploring reputable programming books, online courses, and community-driven platforms that focus on C++ and object-oriented programming principles.