In C++, a const member function is a function that cannot modify the object's state and is declared with the `const` keyword after the parameter list, ensuring that the member variables cannot be altered within that function.
class Example {
public:
int getValue() const { return value; }
private:
int value = 42;
};
What is a Const Function?
A C++ const function is a member function that is declared with the `const` qualifier at the end of its declaration. This indicates that the function is not allowed to modify any member variables of the class it belongs to. In essence, const functions promise not to change the object's state, making them safer to use in contexts where immutability is desired.
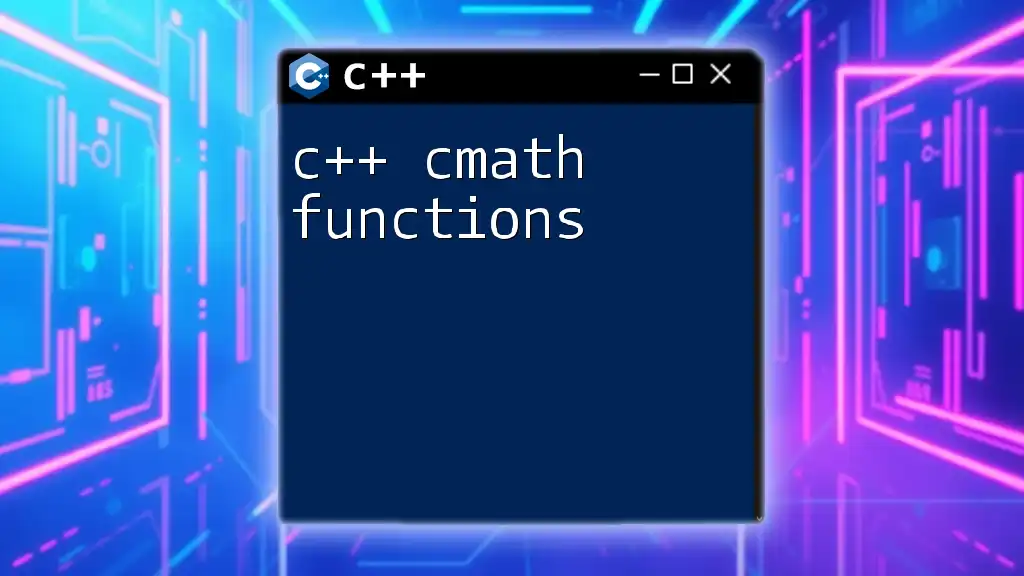
Importance of Const Functions in C++
Understanding and using const functions is essential for several reasons:
- Code Readability: Declaring functions as const clarifies the programmer's intent. Other developers (and your future self) will immediately understand that this function will not alter the object's state.
- Maintainability: When a function is marked as const, it simplifies the debugging process, as you can be certain of the state of the object when that function is called.
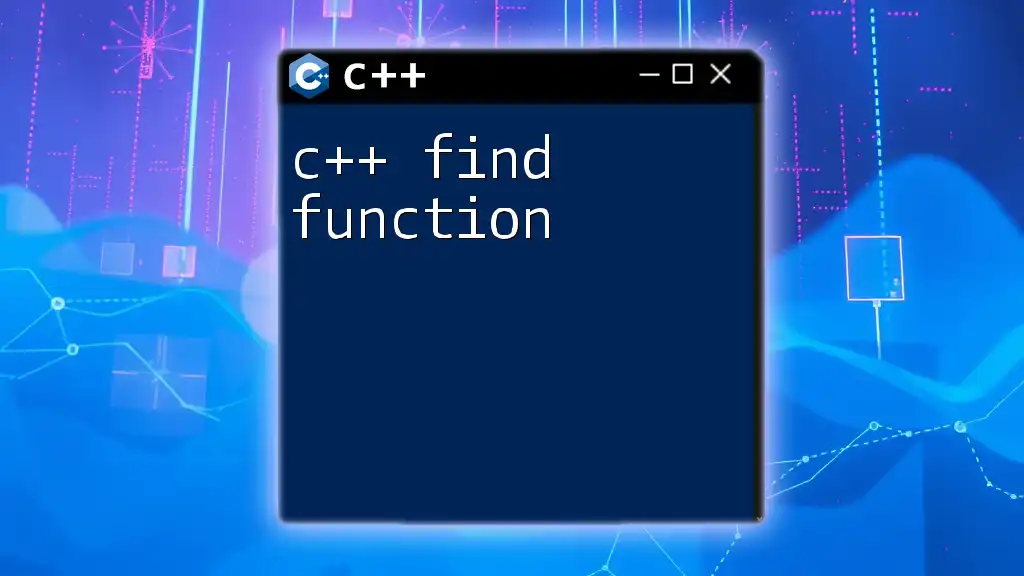
Understanding the Const Keyword in C++
Const in C++: A Fundamental Concept
The `const` keyword in C++ serves as a vital tool for enforcing immutability. This keyword can be applied in various contexts:
- Const Variables: Variables declared with `const` cannot be modified after initialization.
- Const Pointers: Pointers can be constant themselves or can point to constant data, each with its own distinct behavior.
Const Member Functions vs. Non-Const Member Functions
When defining member functions, the addition of `const` at the end alters how the function behaves:
class MyClass {
public:
int x;
MyClass(int val) : x(val) {}
// Const member function
int getValue() const {
return x;
}
// Non-const member function
void setValue(int val) {
x = val;
}
};
In this example, the `getValue` function does not modify the object and can be called on both const and non-const instances. In contrast, the `setValue` function can only be called on non-const instances because it modifies the state.
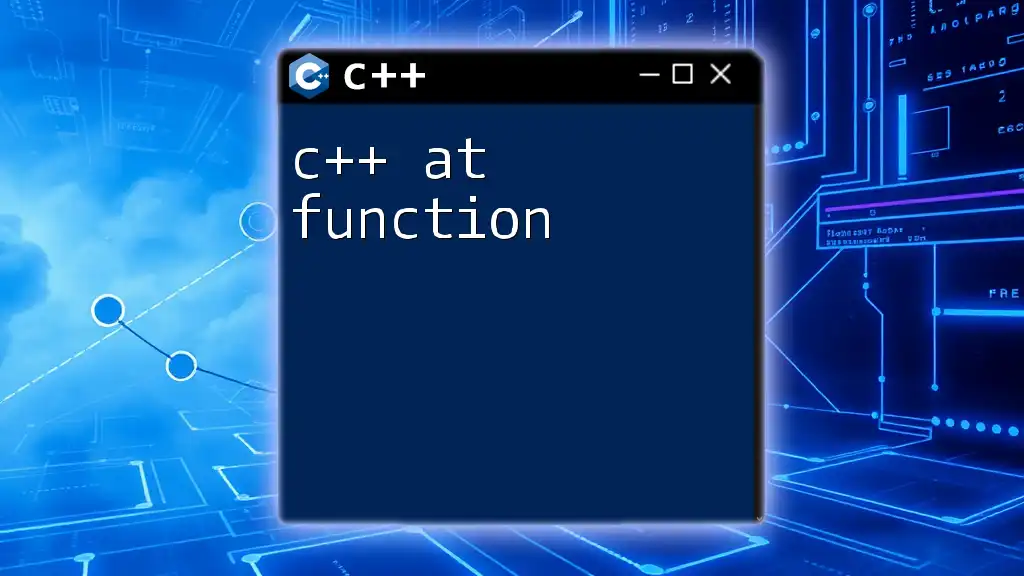
Defining Const Member Functions
How to Declare a Const Member Function
To declare a const member function, append the `const` keyword to the function signature within the class definition. This keyword is placed after the parameter list and before the function body:
class MyClass {
public:
int data;
MyClass(int n) : data(n) {}
// Const function declaration
void display() const {
std::cout << "Data: " << data << std::endl;
}
};
When `display` is called, it guarantees that it will not change the `data` member, providing a promise of immutability.
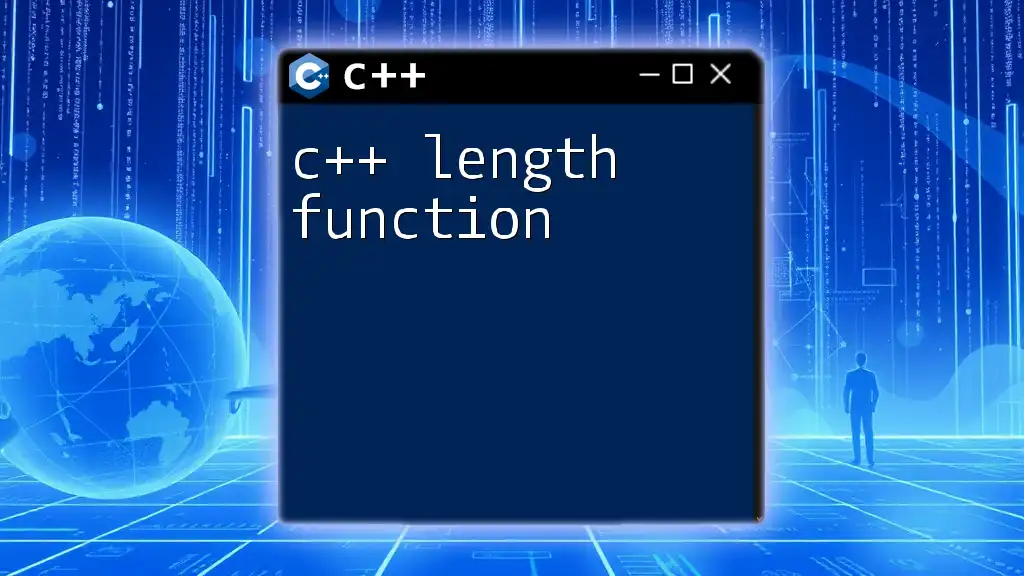
When and Why to Use Const Functions
When to Use Const Functions
Const functions should be utilized in situations where the objective is to read or compute values without altering the class's internal data. Using const functions is particularly beneficial when working with libraries, APIs, or immutable data structures where data integrity is paramount.
Benefits of Using Const Functions
By employing const functions, you achieve multiple benefits:
- Enhanced Clarity: Readers of your code can immediately see which functions are intended to create side effects and which are not.
- Compiler Optimizations: Compilers can optimize const member functions more effectively, leading to potentially better performance.
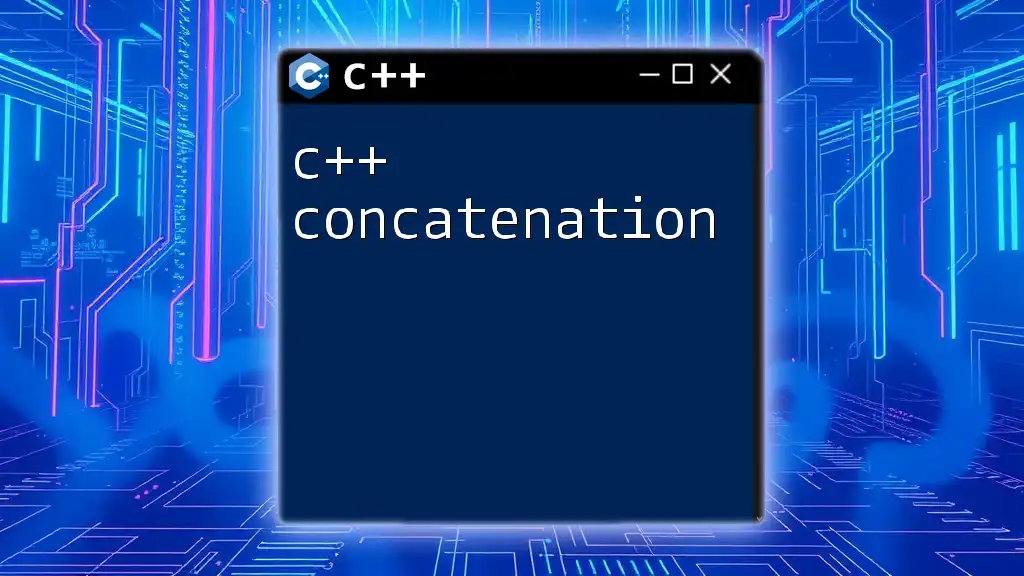
Accessing Member Variables in Const Functions
Rules for Accessing Member Variables
In const member functions, the compiler enforces rules that prevent modifications to member variables:
- No Modification: Regular member variables cannot be changed within a const member function.
- Use of Mutable: If there's a need for certain member variables to change within a const function, the `mutable` keyword allows this.
Code Example: Using Mutable Keyword
The following snippet demonstrates how to use the `mutable` keyword:
class Example {
public:
mutable int counter = 0;
void incrementCounter() const {
counter++; // allowed because counter is mutable
}
void displayCounter() const {
std::cout << "Counter: " << counter << std::endl;
}
};
In `incrementCounter`, the `counter` can be modified despite the function being const, showcasing controlled mutability.
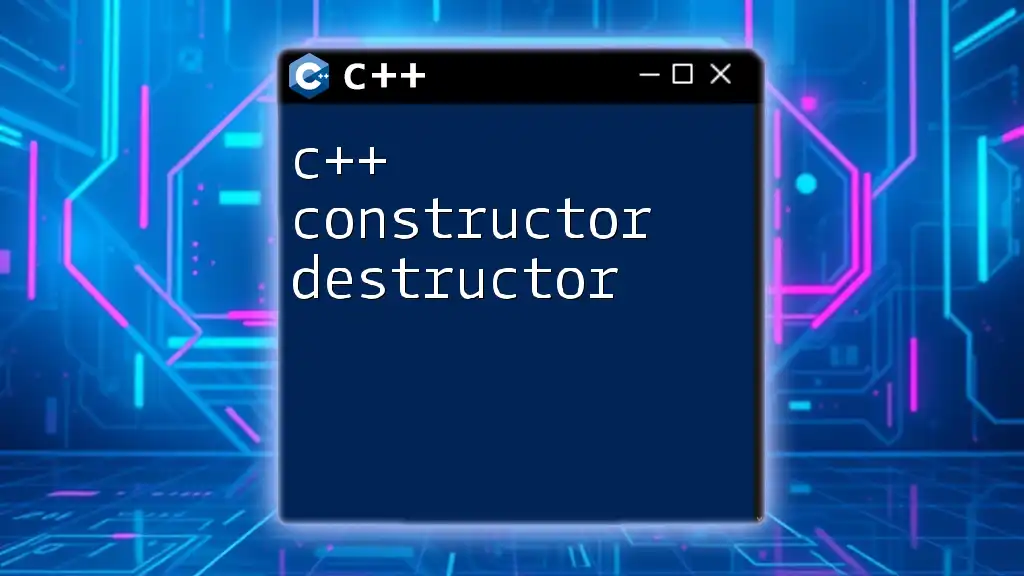
Best Practices for Using Const Functions
Consistent Use of Const
Always apply `const` wherever it is feasible, particularly when defining member functions that don’t alter the state. This practice enforces const correctness, leading to fewer bugs and clearer intentions.
Enhancing API Design with Const
Proper use of const functions can refine class interfaces. If a function doesn’t modify the object, it should be marked as const. This is not just about following conventions; it reflects thoughtful design that takes usability into account.
Testing and Debugging with Const Functions
In testing scenarios, const functions ease debugging by providing clearer expectations of behavior. You can confidently call these functions, knowing they won’t change the state of the object, leading to reliable test results.
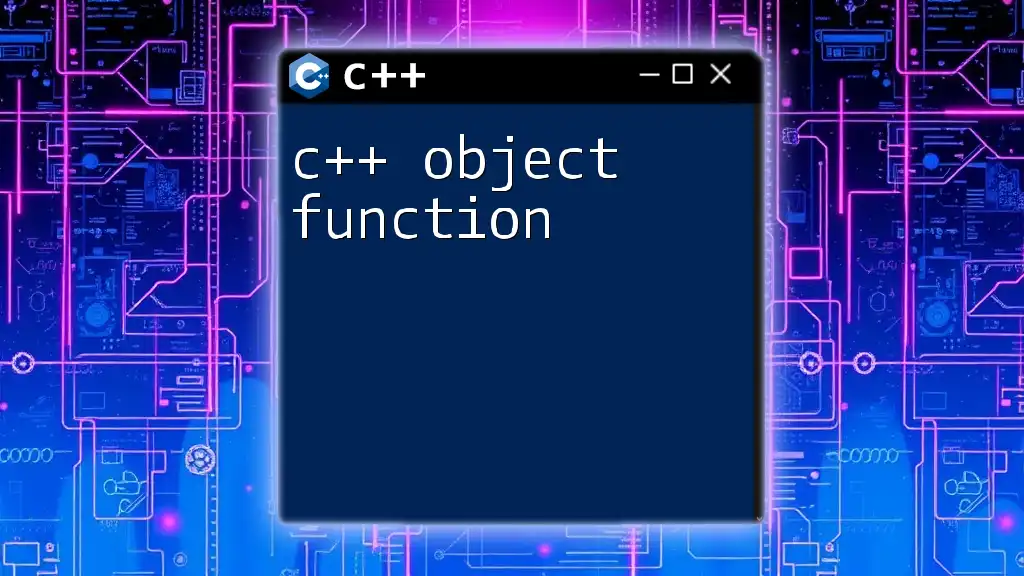
Common Mistakes and Pitfalls
Misunderstanding Const Functions
A frequent misstep among developers is misinterpreting what const functions can and cannot do. Remember, const member functions can read data but cannot change it.
Forgetting Const Correctness
Neglecting to apply const correctness can lead to hard-to-trace bugs. If functions that are intended to be const are not declared as such, it can result in unintentional modifications of object state, complicating your code's logic.
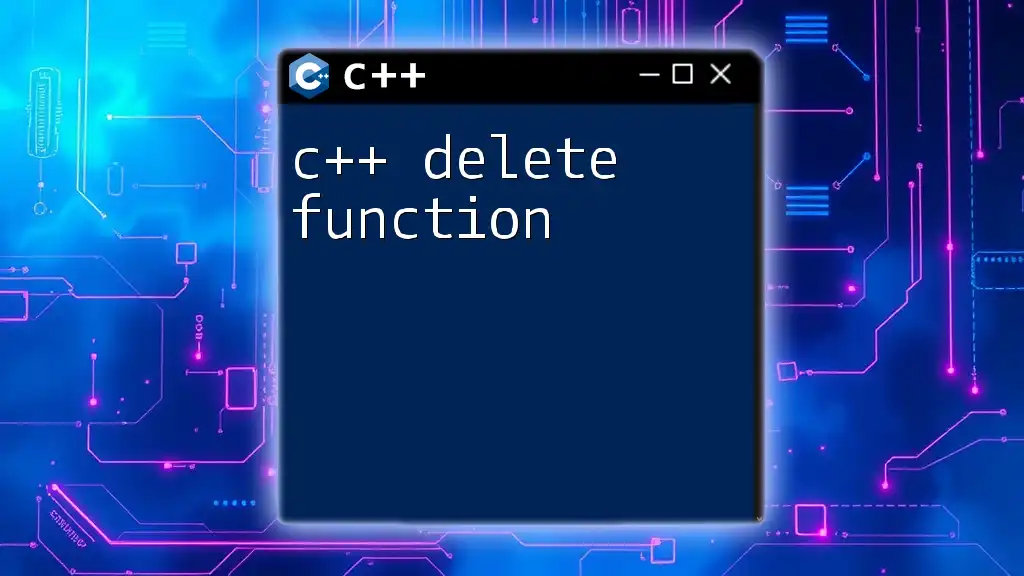
Conclusion
Carefully considering where and how to use C++ const functions can significantly improve your code's robustness and readability. Embrace the best practices discussed in this guide and incorporate const functions into your development workflow, making your code cleaner and more maintainable. As you progress, remember that understanding and applying const will not only benefit you but also anyone who collaborates with you on your coding endeavors.
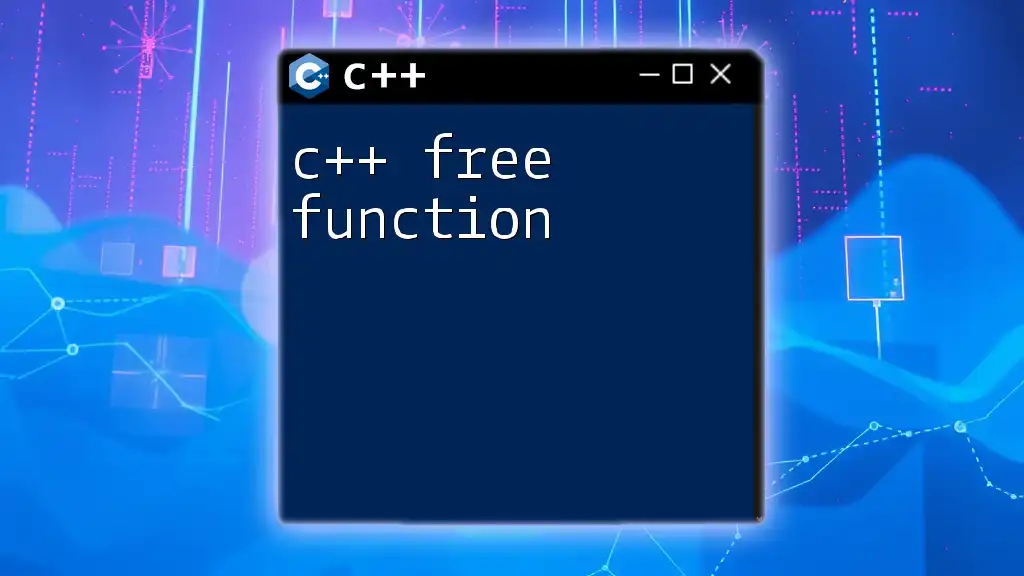
Additional Resources
To further explore the intricacies of C++ const functions and deepen your understanding, consider examining additional resources and joining C++ communities. Engaging with other developers can provide insights that enhance both your technical skills and code quality.