In C++, a static function is a member function that belongs to the class rather than any particular object, allowing it to be called without creating an instance of the class.
Here's a code snippet illustrating its use:
#include <iostream>
class MyClass {
public:
static void staticFunction() {
std::cout << "This is a static function." << std::endl;
}
};
int main() {
MyClass::staticFunction(); // Calling the static function without an object
return 0;
}
What is a Static Function?
A C++ static function is a special type of function that possesses a unique characteristic regarding its linkage and visibility within the code. Unlike traditional functions, which can be accessed from various files or modules within a program, a static function is only accessible within the file it is declared. This encapsulation helps to prevent name clashes and improves modularity in coding.
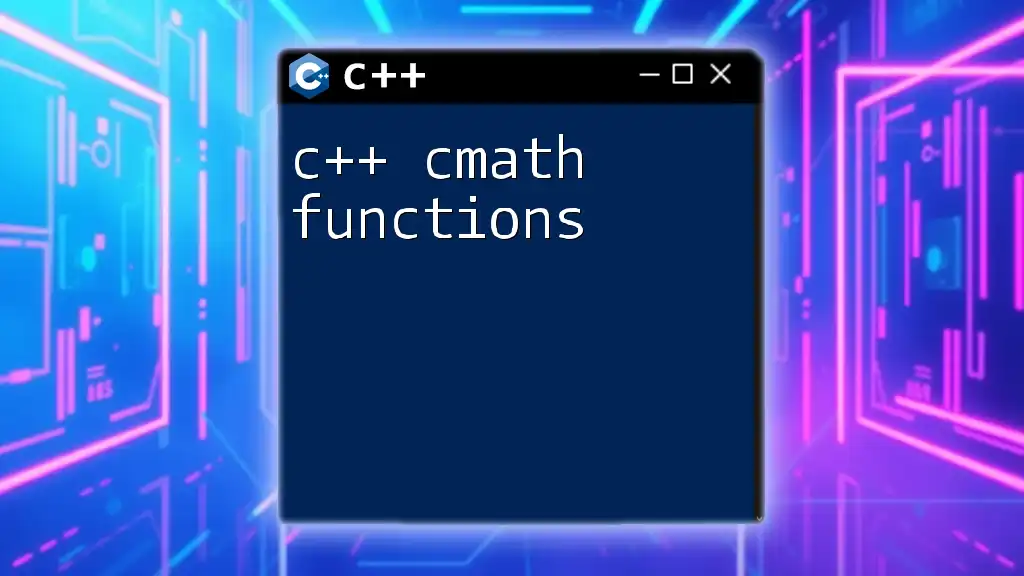
Importance of Static Functions in C++ Programming
Static functions play a crucial role in programming, particularly in the modular design of applications. By limiting the visibility of functions to just one file, developers can avoid conflicts in larger codebases where functions might have the same name but serve different purposes. This is particularly beneficial in team environments where multiple developers work on different parts of the code.
Comparison to Non-Static Functions
When compared to non-static functions, which are universally accessible, static functions allow for tighter control over function accessibility and can lead to cleaner, more maintainable code. This distinction is essential, particularly in complex applications.
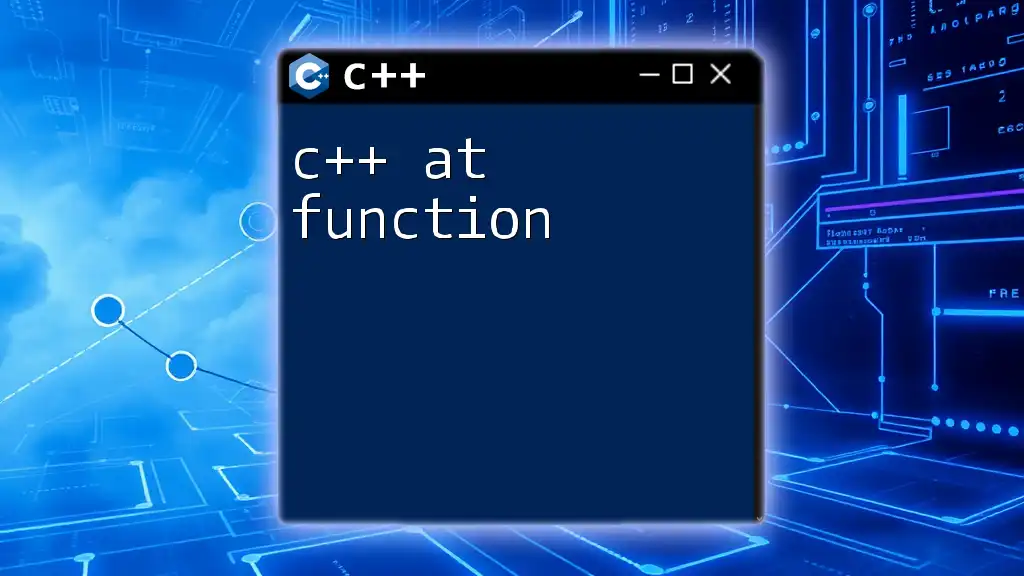
How Static Functions Work
Concept of Static Storage Duration
In C++, a static function maintains a static storage duration, which means its lifetime lasts for the entire duration of the program. When a static function is called, it retains its state and variables between calls, allowing developers to manipulate internal data without exposing it outside.
Linkage of Static Functions
Static functions have internal linkage, which means that they cannot be linked or referenced outside the file where they are defined. This internal linkage protects the function from being accessed or modified by other components of the program.
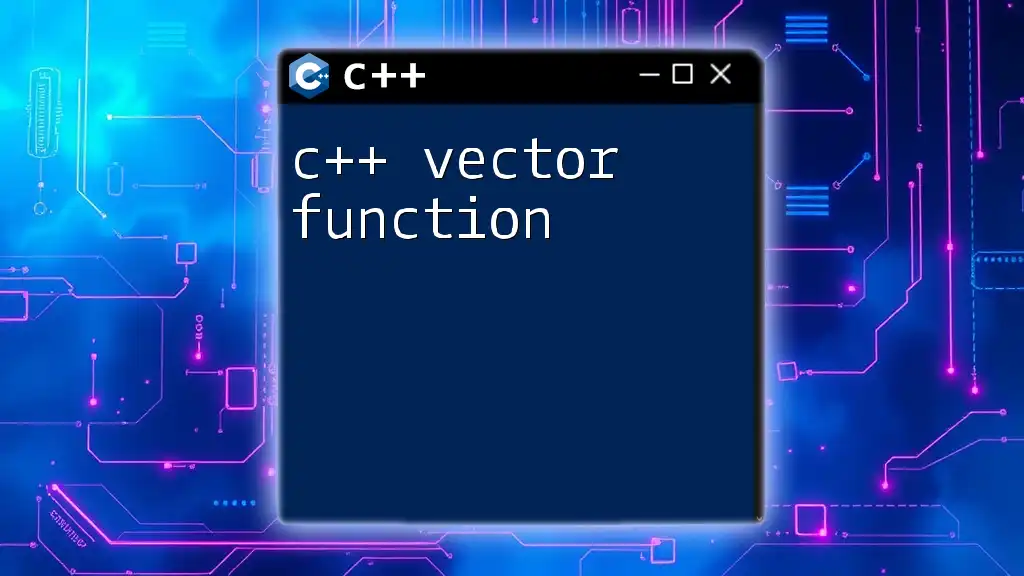
Key Characteristics of C++ Static Functions
Locality
A static function is local to the translation unit (source file) in which it resides. This locality restricts its use, ensuring that it doesn't interfere with functions defined in other files.
Lifetime
The lifetime of static functions is equal to that of the program itself. Once defined, they remain in memory throughout the execution of the program, making them efficient for repeated calls.
Accessibility
The accessibility of a static function is confined to the file where it is declared. This feature is useful for implementing helper functions that shouldn't be accessible globally, thereby encapsulating their functionality.
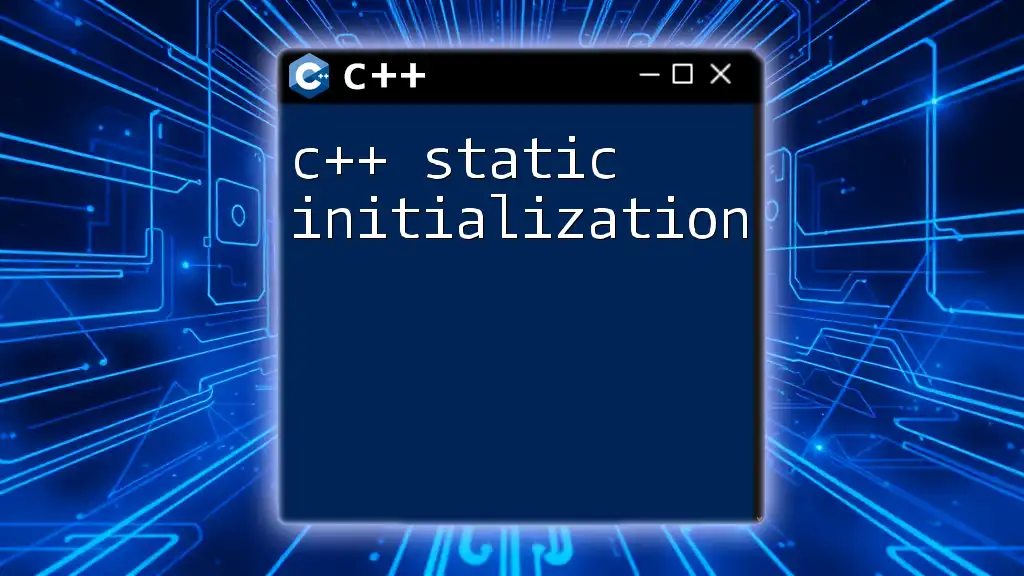
Syntax of a Static Function in C++
The syntax for declaring a static function in C++ is straightforward:
static returnType functionName(parameters) {
// Function body
}
Example of a Simple Static Function
Here's a basic example of a static function in action:
#include <iostream>
static void greet() {
std::cout << "Hello, Static Function!" << std::endl;
}
int main() {
greet(); // Calling the static function
return 0;
}
In this example, the `greet` function is declared as static, which confines its accessibility to the source file. Calling this function from `main` works seamlessly, showcasing its intended use without any external visibility.
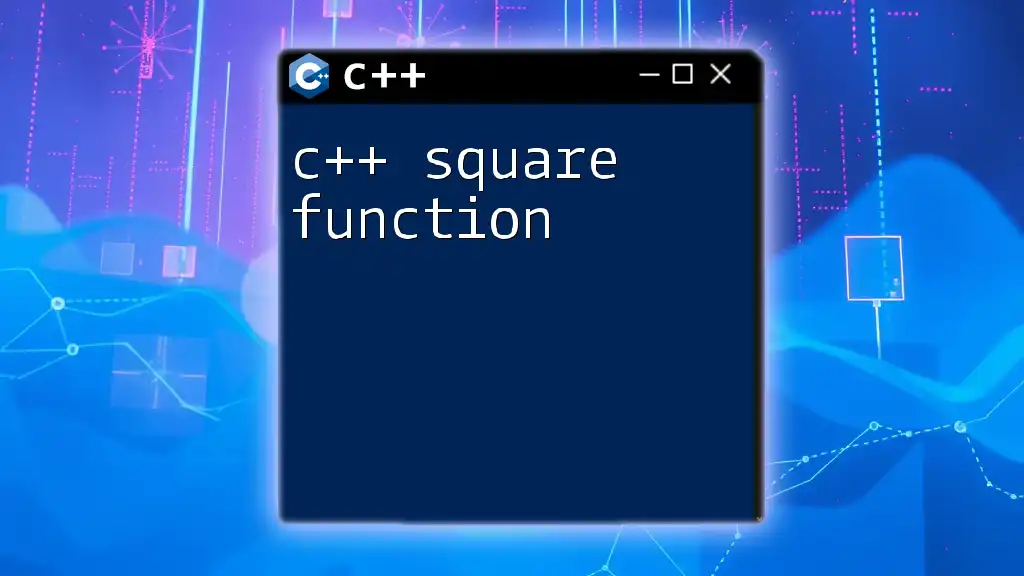
Scope and Visibility of Static Functions
File Scope vs. Global Scope
When a static function is declared, it has file scope. This means that it cannot be referenced outside its defining source file. In contrast, global functions, which aren't static, can be accessed from any file within the same program, provided that the declaration is available.
Static Functions and Header Files
It's important to maintain best practices when using static functions with header files. Avoid declaring static functions in header files that are included in multiple source files, as this can lead to duplicate definitions.
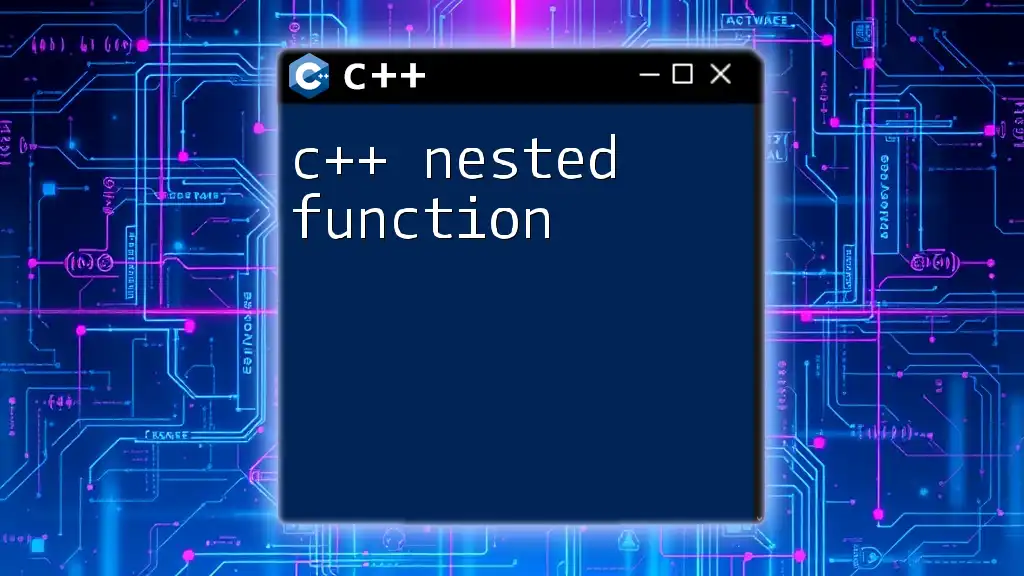
When to Use Static Functions in C++
Typical Use Cases for Static Functions
Some common scenarios where static functions prove advantageous include:
- Utility Functions: Functions that perform common tasks for a module without needing to be publicly exposed.
- Encapsulation: When implementing complex logic that doesn't need to be accessed globally, static functions can help maintain a clear interface.
- Implementation Details: Functions that are part of the internal workings of a module, which should not be exposed to consumers of the module.
Pros and Cons of Static Functions
Pros:
- Encapsulation: They help encapsulate functionality within a specific translation unit.
- Prevention of Namespace Clashes: They reduce the chances of function name collisions.
Cons:
- Limited Accessibility: If necessary, static functions can't be accessed from other files.
- Testing Limitations: They may complicate unit testing as testing frameworks usually prefer functions with broader visibility.
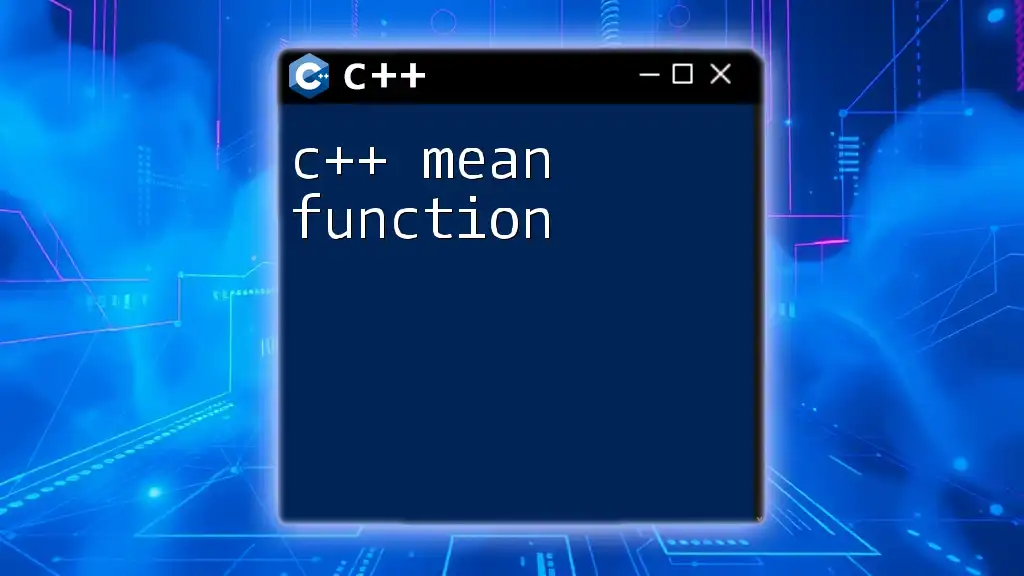
Best Practices for Using Static Functions
Naming Conventions
To maintain clarity, static functions should follow consistent naming conventions that clearly indicate their scope and usage, such as prefixing them with "static_" to denote restricted visibility.
Documentation and Code Clarity
Well-documented static functions make the codebase easier to maintain and understand. Even though they are local, comments should be included to explain their purpose and functionality.
Maintaining Code Quality
Static functions should be kept concise and focused on a single task to promote readability and maintainability. Ensure that any changes to static functions are carefully considered, as they can impact all uses within their specific translation unit.
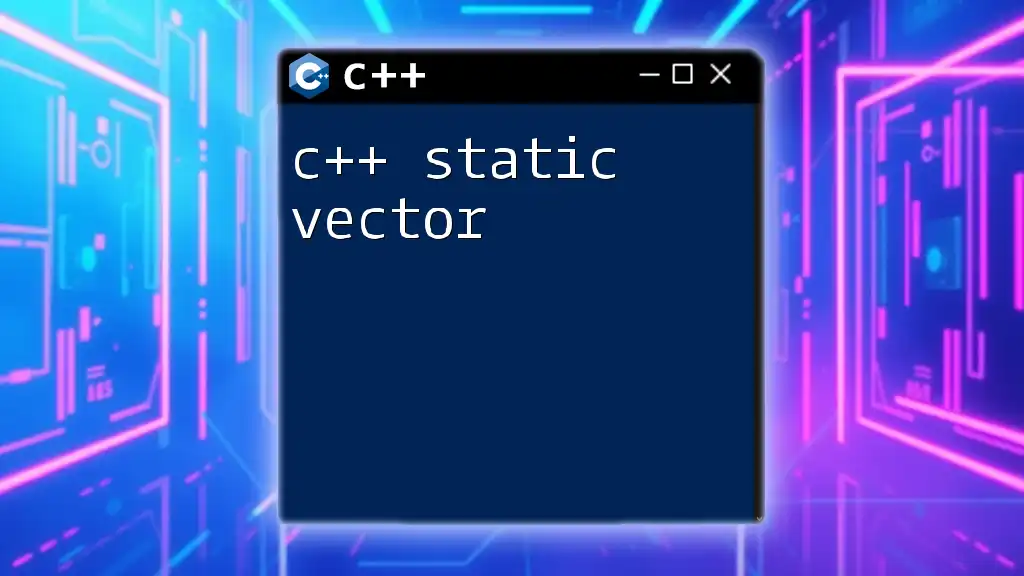
Advanced Concepts in Static Functions
Static Member Functions in Classes
Static member functions in classes are a different category altogether. While they operate as part of a class, they still maintain the characteristics of static functions in that they do not require an instance of the class to be called. Here’s an example:
class Example {
public:
static void display() {
std::cout << "Static Member Function!" << std::endl;
}
};
int main() {
Example::display(); // Calling a static member function
return 0;
}
In this example, the static member function `display` can be called without creating an instance of the class `Example`, demonstrating yet another powerful use of static functions.
Static Functions with Pointers and References
Static functions can also seamlessly interact with pointers and references, allowing them to modify data without exposing it globally. This feature is particularly beneficial in callback mechanisms or when dealing with event-driven programming.
Linking Static Functions with Multithreading
While static functions are useful, care should be taken when used in multithreaded applications. Since static functions maintain state and can be invoked by multiple threads, it’s vital to ensure that any shared data is appropriately synchronized to avoid race conditions.
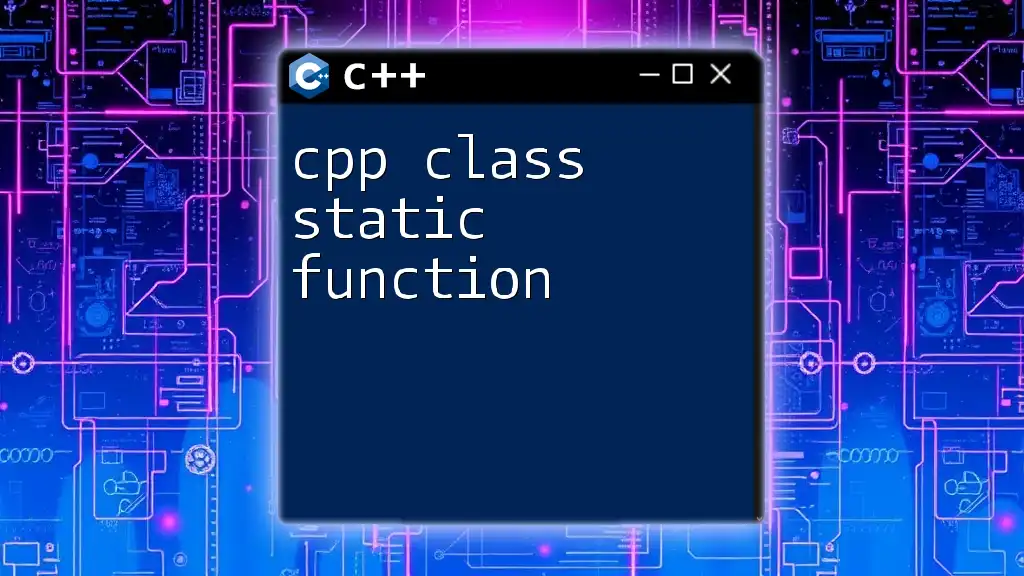
Recap of Key Points
To sum up, C++ static functions are a powerful construct, offering encapsulation and preventing namespace pollution while maintaining a static lifespan throughout the program. Understanding their behavior, especially regarding linkage and scope, can significantly enhance your coding practices.
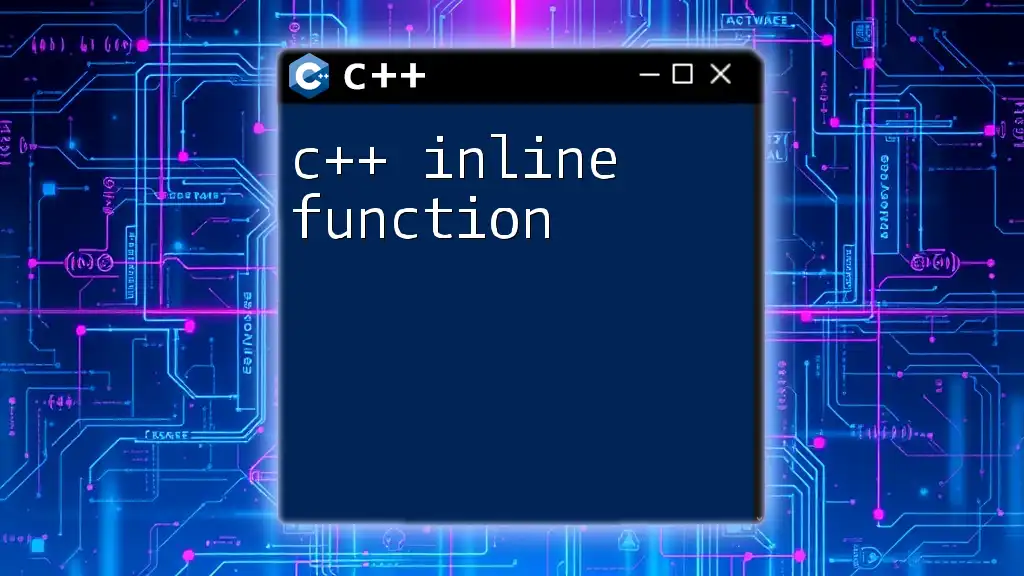
Encouragement for Practical Application
As you work on your C++ projects, consider how static functions can help encapsulate functionality and maintain clean code. Implementing them where appropriate can streamline your development process and reduce potential conflicts in larger applications.
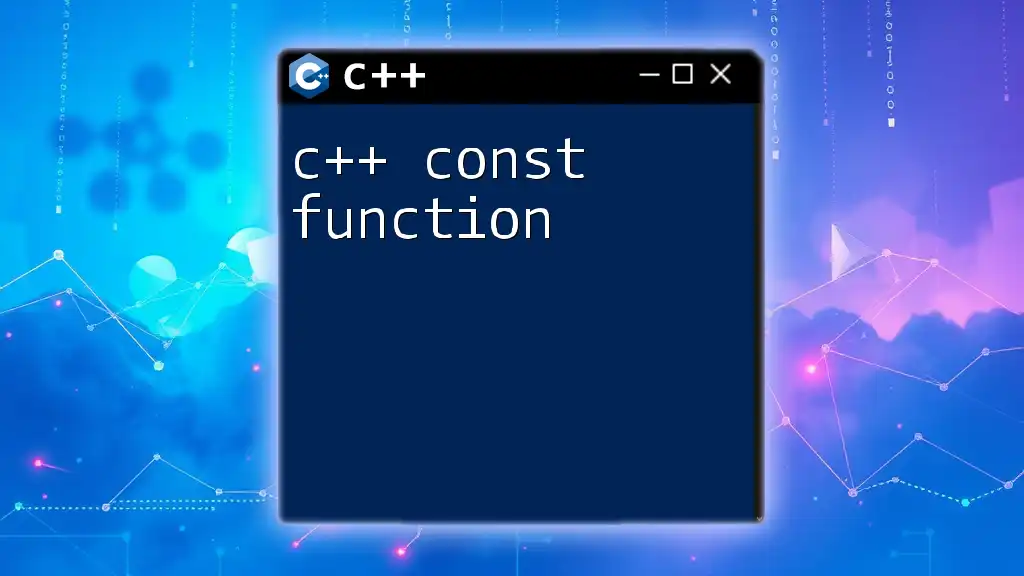
Call to Action
What has been your experience with C++ static functions? Share your insights and any questions you might have. Engaging with the coding community can provide valuable perspectives and enhance our collective knowledge!