A C++ virtual function table (vtable) is a mechanism used to support dynamic (runtime) polymorphism, allowing derived classes to override base class virtual functions, enabling function calls to be resolved at runtime based on the object type.
Here's a simple code snippet illustrating the concept:
#include <iostream>
class Base {
public:
virtual void show() { std::cout << "Base class show function called." << std::endl; }
};
class Derived : public Base {
public:
void show() override { std::cout << "Derived class show function called." << std::endl; }
};
int main() {
Base* b = new Derived(); // Base pointer points to Derived object
b->show(); // Calls Derived's show function due to vtable
delete b;
return 0;
}
What is a Virtual Function Table?
The C++ virtual function table (vtable) is a fundamental concept that plays a crucial role in achieving polymorphism in the language. When we define a method as virtual, we instruct the compiler to use dynamic method resolution instead of static, allowing the program to determine at runtime which method to invoke based on the derived class of the object being accessed.
The vtable is essentially a lookup table used by the compiler to store pointers to the virtual functions of a class. Whenever a class contains virtual functions, the compiler creates a vtable for that class, which serves as a reference point for all objects of that class and its derived classes. This is what enables the behavior of invoking the correct method during runtime, making it possible to use derived class methods even when they are accessed through a base class pointer.
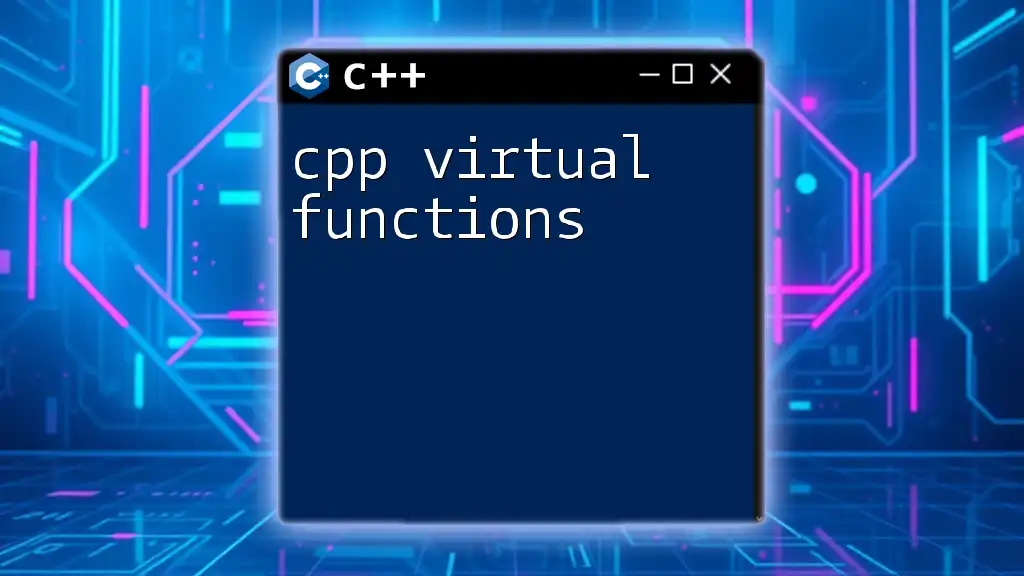
Understanding Virtual Methods
What are Virtual Methods?
In C++, a virtual method is a member function declared within a base class that can be overridden in a derived class. By marking a function as virtual, we inform the compiler to support dynamic dispatch, which means that the most derived version of the function will be called at runtime, depending on the type of the object, not the type of the reference or pointer.
For instance, consider a simple base class with a virtual function:
class Base {
public:
virtual void show() {
std::cout << "Base Class Show" << std::endl;
}
virtual ~Base() {} // Virtual destructor to prevent memory leaks
};
Syntax of Virtual Functions
The syntax for declaring a virtual function is straightforward. You use the `virtual` keyword in the function declaration within the class definition, as illustrated above. This tells the compiler to set up the vtable for the class, enabling dynamic binding of methods.
When a derived class overrides this method, it can use the `override` keyword for clarity:
class Derived : public Base {
public:
void show() override {
std::cout << "Derived Class Show" << std::endl;
}
};
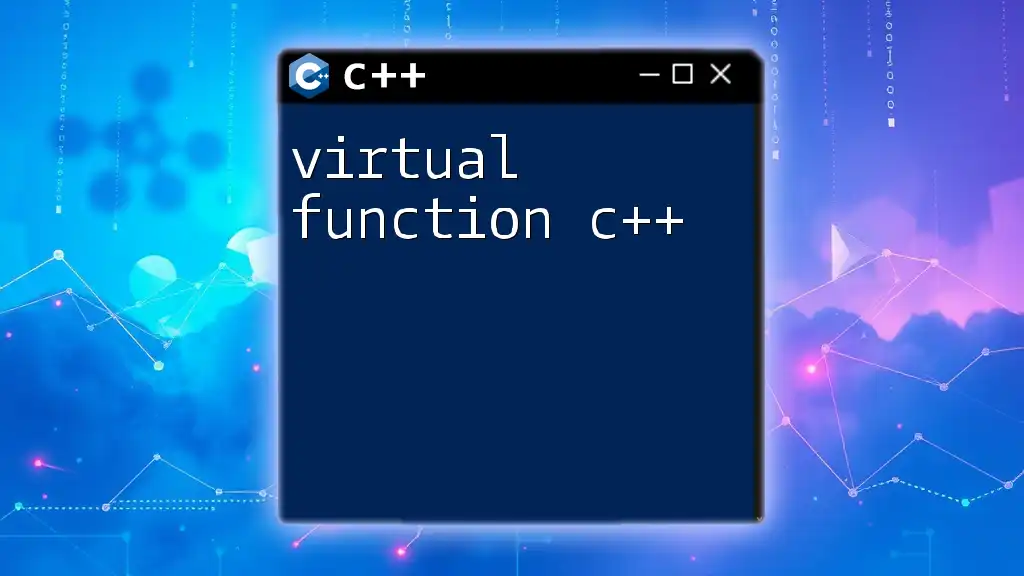
The Mechanics of The Virtual Table in C++
Creation of the vtable
The vtable is created at compile time, but its references are utilized during runtime. When you instantiate a class with virtual functions, the compiler generates a vtable for that class, containing pointers to the virtual functions defined in the class. This table is maintained for each class with virtual functions, and every object of such a class contains a hidden pointer (often termed as vptr) pointing to its class's vtable.
Structure of the vtable
Description of the vtable structure
The vtable consists of an array of pointers to virtual functions. Each class that declares or inherits a virtual function will have its own vtable.
For example, if we have a base class `Base` with one virtual function and a derived class `Derived` that overrides this function, the vtable would look something like this:
Base vtable:
+-----------------+
| Base::show() |
+-----------------+
Derived vtable:
+--------------------+
| Derived::show() |
+--------------------+
This illustrates how the vtable enables dynamic dispatch based on the actual object type rather than the pointer/reference type.
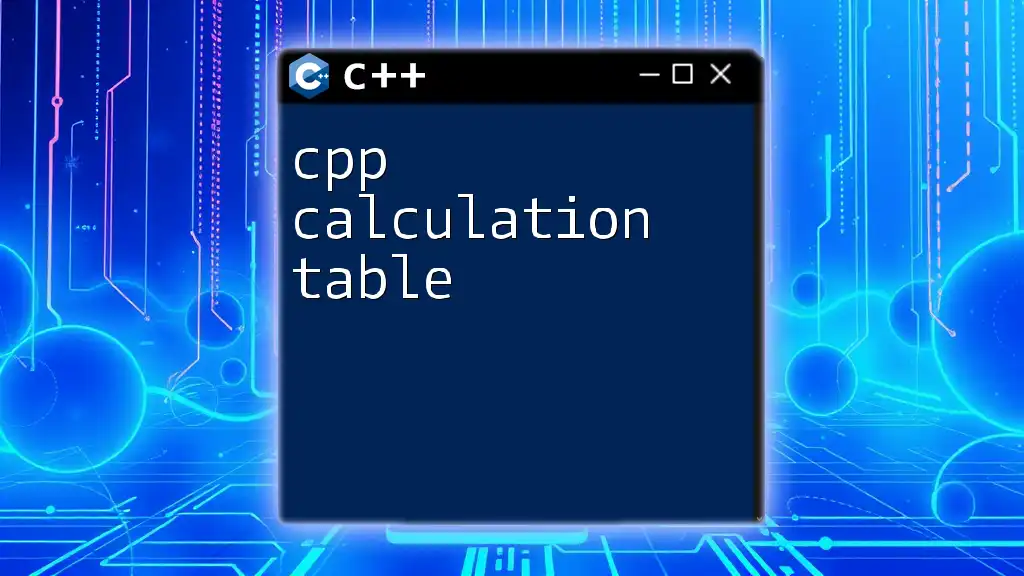
Accessing Functions Through the vtable
How vtable Lookup Works
When a virtual function is called on an object through a base class pointer or reference, the program does not know which method to call until runtime. This runtime resolution is achieved through a process known as vtable lookup. Upon invoking a virtual function, the program checks the vptr of the object to find the corresponding vtable and subsequently retrieves the address of the appropriate function to execute.
Consider the following function that takes a pointer to the base class:
void func(Base* b) {
b->show(); // Calls the appropriate show function based on object type
}
If you pass an instance of `Derived` to this function, it will call `Derived::show()` instead of `Base::show()`, thanks to the vtable mechanism.
Example: Base and Derived Classes
Here’s a detailed example demonstrating how the vtable facilitates polymorphism:
class Base {
public:
virtual void show() {
std::cout << "Base Class Show" << std::endl;
}
virtual ~Base() {} // Ensure proper cleanup
};
class Derived : public Base {
public:
void show() override {
std::cout << "Derived Class Show" << std::endl;
}
};
int main() {
Base* b = new Derived(); // Upcasting
b->show(); // Outputs: Derived Class Show
delete b; // Clean up
}
In this scenario, the `show()` function of `Derived` is invoked even though we are using a `Base*` pointer, demonstrating the power of the C++ vtable in enabling polymorphism.
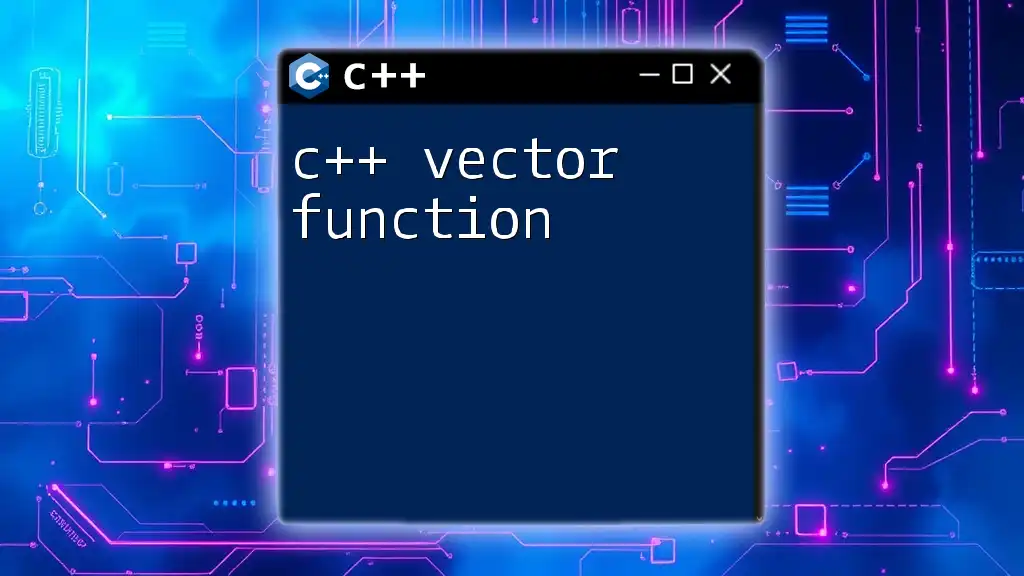
Common Pitfalls and Best Practices
Common Mistakes When Using Virtual Functions
One of the most common mistakes in C++ when working with virtual functions is neglecting to declare the destructor of a base class as virtual. This can lead to resource leaks and undefined behavior when derived class objects are deleted through base class pointers.
For example, if the `Base` class did not have a virtual destructor, deleting a `Derived` object via a `Base` pointer would call the `Base` destructor only, causing any cleanup code in `Derived` to be skipped.
Best Practices
To effectively utilize virtual functions and vtables, consider the following best practices:
- Always declare destructors as virtual in base classes that are intended to be subclassed.
- Use `override` when overriding base class methods to catch any signature mismatches at compile time.
- Be mindful of performance. While virtual functions enable polymorphism, they come with a slight performance overhead due to the vtable lookup. If performance is critical, consider alternative designs.
- Choose virtual functions when you need polymorphic behavior. If a method does not require overriding, make it non-virtual to avoid unnecessary overhead.
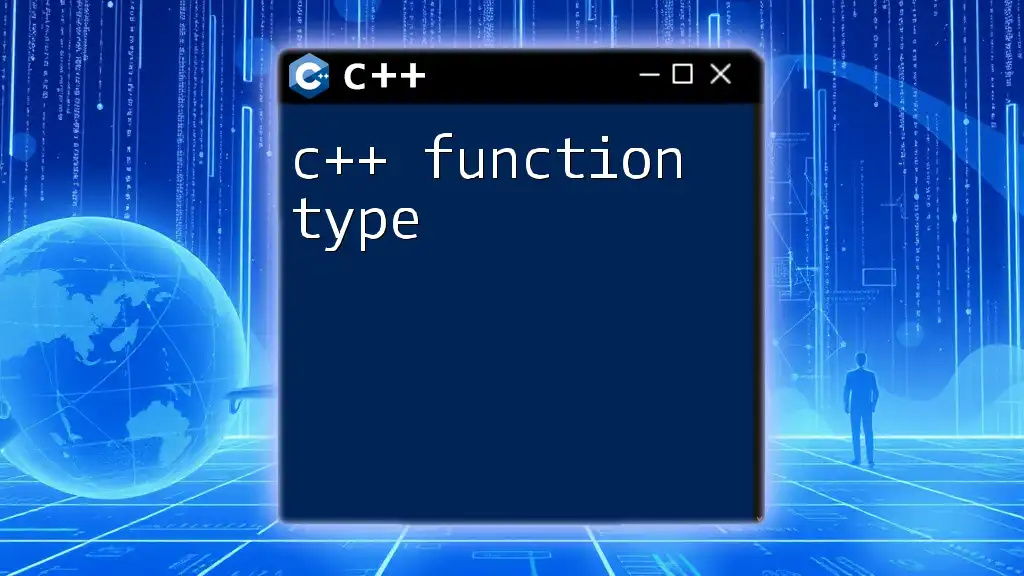
Conclusion
The C++ virtual function table is an integral part of enabling polymorphism in C++. Understanding how the vtable operates helps programmers leverage object-oriented programming effectively, fostering clear and maintainable code. By grasping the mechanics of virtual methods and the vtable, you can write more versatile and robust applications in C++. With careful consideration of best practices, you can avoid common pitfalls and optimize the usage of virtual functions in your projects.
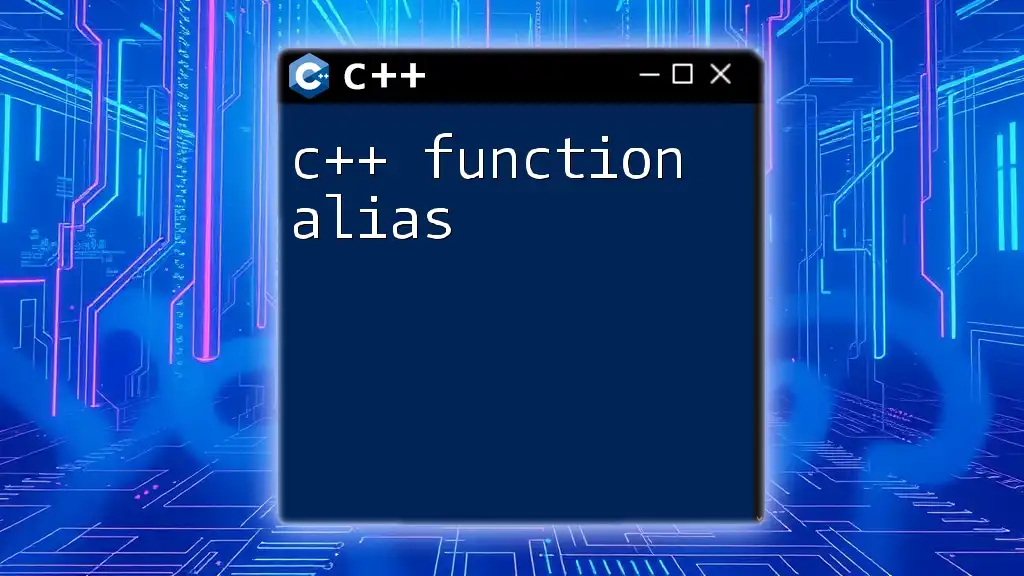
Additional Resources
For those looking to delve deeper into this topic, consider exploring more on C++ polymorphism, inheritance, and advanced object-oriented programming concepts through books and online courses.
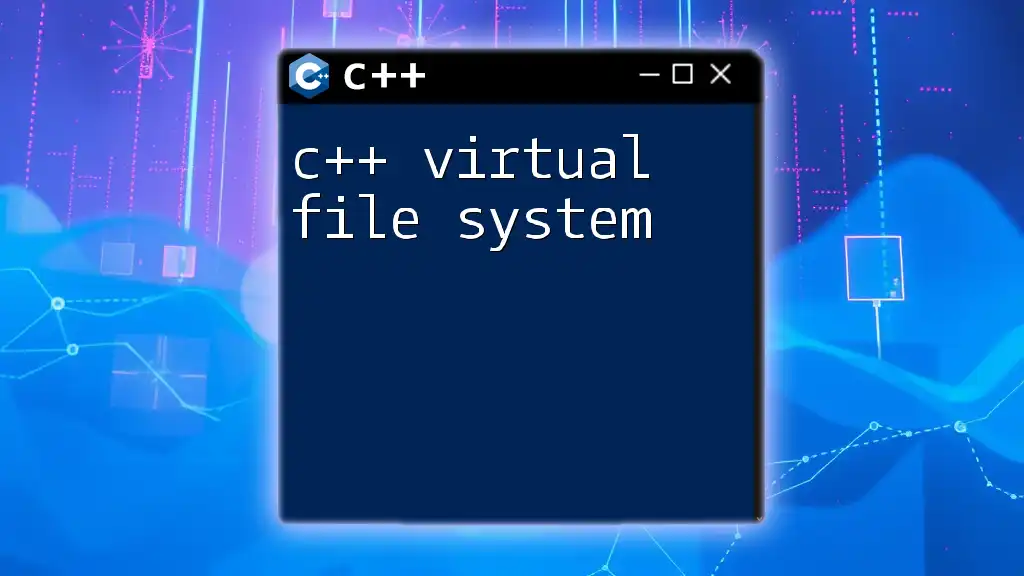
FAQs
-
What happens if I don’t declare a virtual destructor? You could face memory leaks or undefined behavior when deleting derived class objects via base class pointers.
-
Can I have static methods in classes with virtual functions? Yes, static methods do not require vtables and behave normally. They do not support polymorphism.