A C++ virtual template function allows for the definition of a template that can be overridden in derived classes, enabling dynamic polymorphism while providing type flexibility.
Here's a simple code snippet demonstrating the concept:
#include <iostream>
template<typename T>
class Base {
public:
virtual void display(T value) {
std::cout << "Base display: " << value << std::endl;
}
};
template<typename T>
class Derived : public Base<T> {
public:
void display(T value) override {
std::cout << "Derived display: " << value << std::endl;
}
};
int main() {
Base<int>* obj = new Derived<int>();
obj->display(5); // Calls Derived's display
delete obj;
return 0;
}
Understanding Virtual Functions in C++
What is a Virtual Function?
A virtual function in C++ is a member function in a base class that you expect to override in derived classes. When you call a virtual function through a base class pointer or reference, C++ determines which function to execute at runtime, enabling dynamic polymorphism. This is essential in achieving flexible and manageable code in scenarios involving inheritance.
Here’s a simple example to illustrate a basic virtual function:
class Base {
public:
virtual void show() {
std::cout << "Base class show function called." << std::endl;
}
};
class Derived : public Base {
public:
void show() override {
std::cout << "Derived class show function called." << std::endl;
}
};
When you create an instance of `Derived` and call the `show` function using a pointer of type `Base`, the derived version of the function gets executed:
Base* b = new Derived();
b->show(); // Output: Derived class show function called.
How Virtual Functions Work
The underlying mechanism that allows virtual functions to behave as they do is the vtable, which stands for virtual table. Each class with virtual functions has its own vtable that holds pointers to the virtual functions available for that class. When a virtual function is invoked, the program looks up the actual function to call using this vtable.
Example of how the vtable operates:
int main() {
Base* b = new Derived();
b->show(); // Calls Derived's show() due to the vtable lookup.
delete b;
}
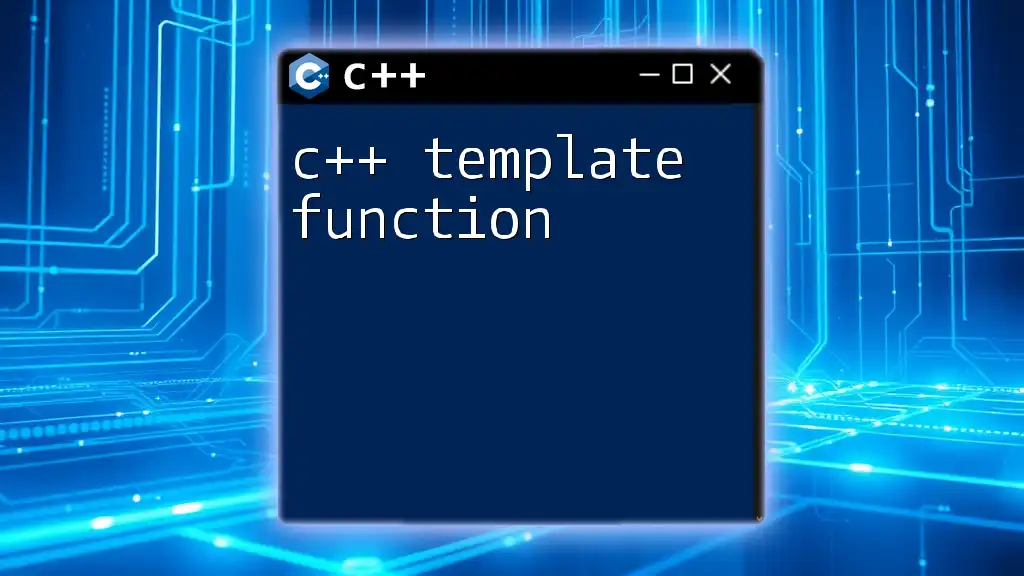
Understanding Templates in C++
What is a Template?
A template enables you to write generic and reusable code. In C++, you can have function templates and class templates, which allow you to define functions or classes with a placeholder type. This functionality helps you write versatile code that can work with any data type.
A simple function template example is shown below:
template <typename T>
T add(T a, T b) {
return a + b;
}
This function can now accept parameters of any type, as long as the `+` operator is defined for that type.
The Power of Templates
Templates promote type-generic programming, which allows you to write code without needing to specify the exact data type beforehand. This can lead to cleaner code and can significantly reduce duplication.
Here’s an example illustrating a class template:
template <typename T>
class Pair {
public:
T first;
T second;
Pair(T a, T b) : first(a), second(b) {}
};
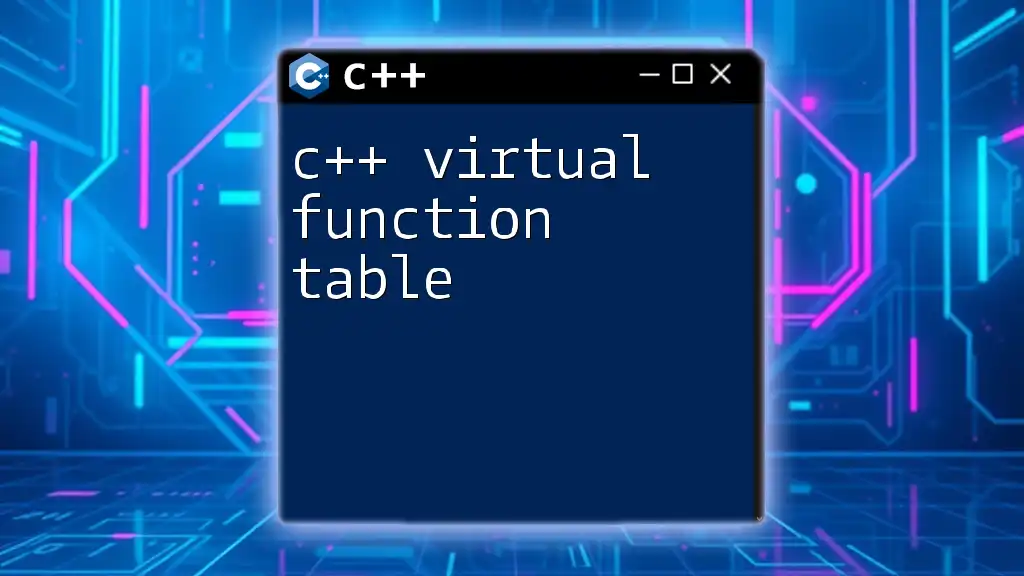
Combining Virtual Functions and Templates
What is a Virtual Template Function?
A virtual template function combines the principles of virtual functions and templates. It allows you to define a virtual function within a template class, enabling polymorphic behavior while maintaining type flexibility. This is particularly useful when working with a class hierarchy where each derived type may exhibit different behaviors based on its template parameter.
How to Declare Virtual Template Functions
To declare a virtual template function, the syntax is straightforward. You define the template above your class definition and then specify that the function is virtual.
Example of declaring a virtual template function:
template <typename T>
class Base {
public:
virtual void display(T data) {
std::cout << "Displaying data: " << data << std::endl;
}
};
template <typename T>
class Derived : public Base<T> {
public:
void display(T data) override {
std::cout << "Derived display: " << data << std::endl;
}
};
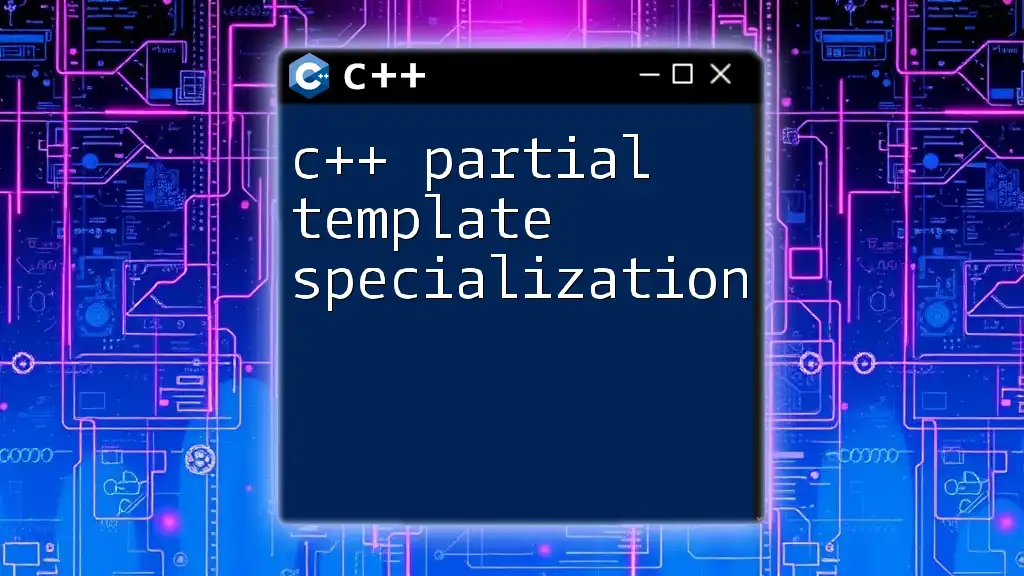
Practical Use Cases
Example 1: Polymorphic Behavior with Different Types
Let's say you want a class that can handle different types of data uniformly. By using a virtual template function, you can achieve this.
template <typename T>
class Container {
public:
virtual void hold(T value) {
std::cout << "Holding value: " << value << std::endl;
}
};
template <typename T>
class IntContainer : public Container<int> {
public:
void hold(int value) override {
std::cout << "Int container holds: " << value << std::endl;
}
};
Container<int>* container = new IntContainer<int>();
container->hold(42); // Output: Int container holds: 42
Example 2: Factory Pattern Implementation
The Factory Design Pattern benefits greatly from virtual template functions, as seen in this example:
template <typename T>
class Shape {
public:
virtual void draw() = 0; // Pure virtual function
};
template <typename T>
class Circle : public Shape<T> {
public:
void draw() override {
std::cout << "Drawing Circle" << std::endl;
}
};
template <typename T>
class Square : public Shape<T> {
public:
void draw() override {
std::cout << "Drawing Square" << std::endl;
}
};
template <typename T>
Shape<T>* createShape(const std::string& type) {
if (type == "circle")
return new Circle<T>();
else if (type == "square")
return new Square<T>();
return nullptr;
}
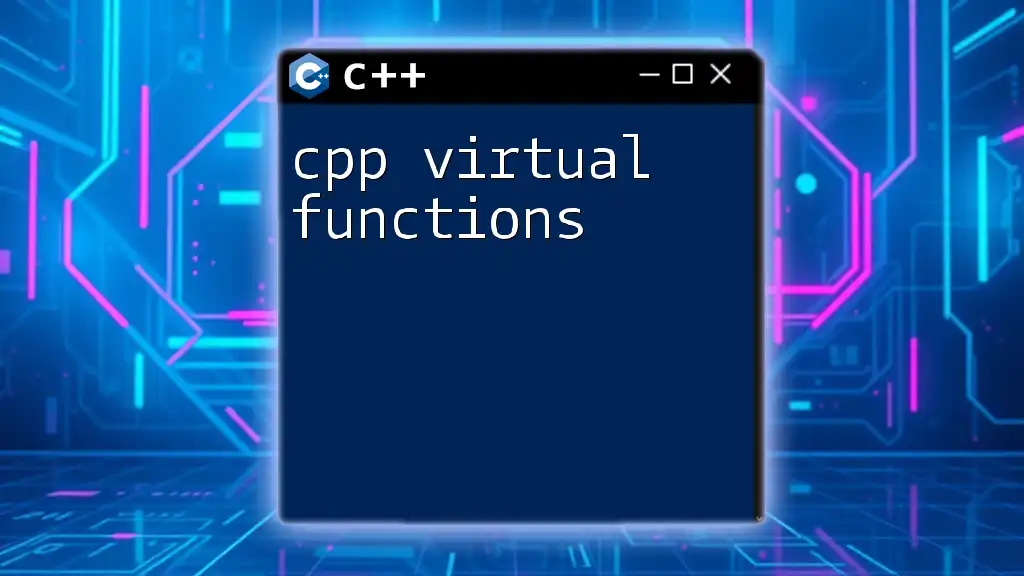
Common Challenges and Pitfalls
Understanding Limitations
While virtual template functions provide great flexibility, they might not always be the best choice. For instance, you cannot have a virtual template function that is defined outside the class declaration. Also, if you try to pass a different type to a template-defined function that expects a specific type, you will encounter compilation errors.
Example of such limitation:
// This will not compile
template <typename T>
virtual void myFunction(T data) {}
Performance Considerations
Using virtual template functions comes with performance overhead due to dynamic binding. The performance might be affected when relying heavily on virtual functions in a tight loop or performance-critical applications. Profiling and measuring performance in such scenarios is essential.
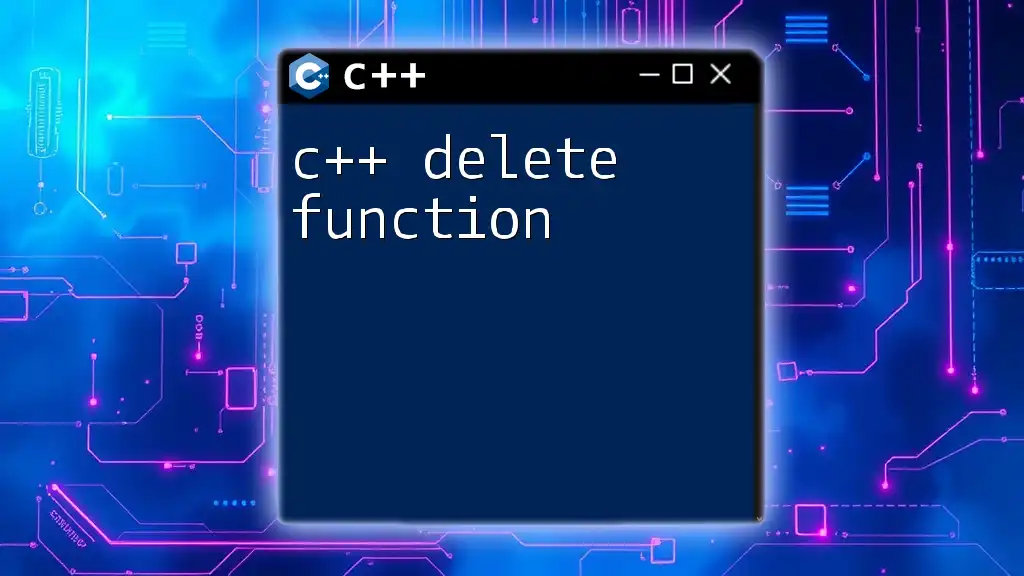
Best Practices
When to Use Virtual Template Functions
Use virtual template functions when you need different behaviors based on types in a polymorphic class hierarchy and want to retain type flexibility. Avoid using them in trivial classes that do not require polymorphic behavior, as it can complicate the design.
Tips for Writing Clear and Efficient Code
To maintain clarity:
- Use meaningful names for your template parameters.
- Ensure that your virtual function overrides are marked clearly with `override` to catch mismatches during compilation.
- Refactor large classes into smaller, well-defined components to improve maintainability.
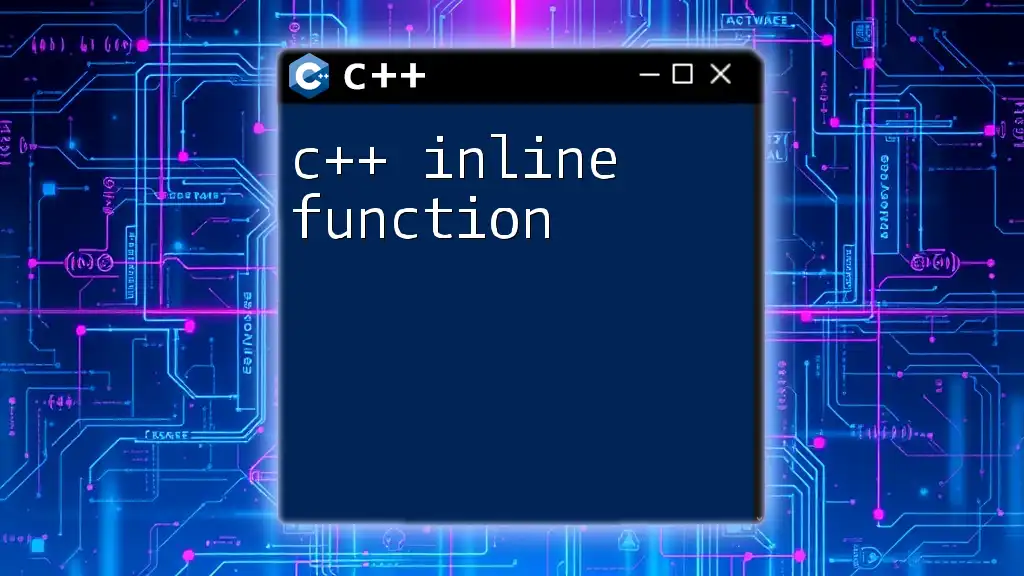
Conclusion
Throughout this article, we’ve explored the concept of C++ virtual template functions. We've covered their definitions, practical examples, and the pitfalls to watch out for. Leveraging this powerful combination allows for flexible and reusable code, critical in building robust C++ applications.
Engage with additional resources, practice your skills through hands-on projects, and don't hesitate to experiment with virtual template functions in your coding practices. Happy coding!
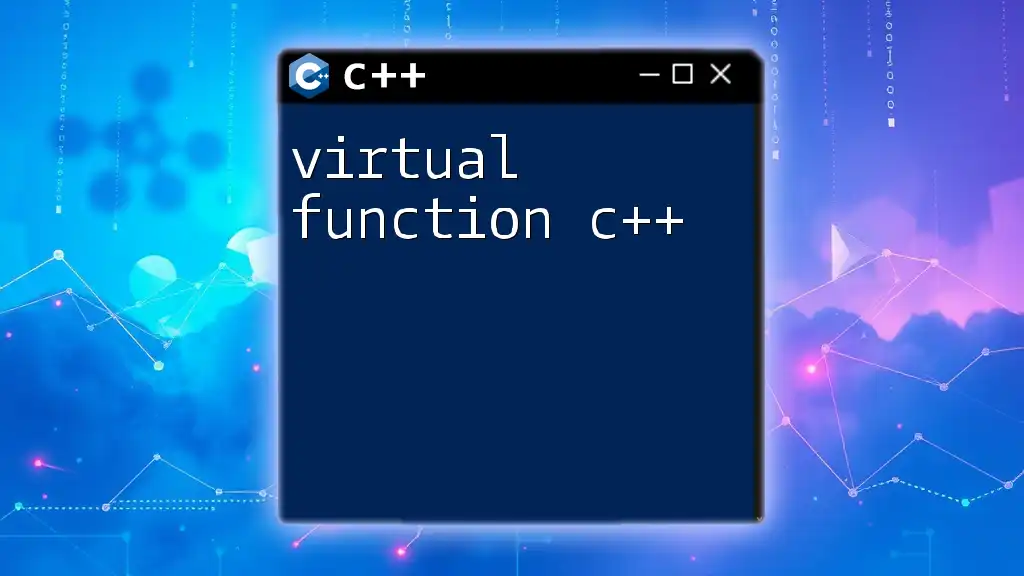
Further Reading and Resources
For deeper learning on C++ virtual functions and templates, consider the following resources:
- "The C++ Programming Language" by Bjarne Stroustrup
- C++ documentation and tutorials on virtual functions and templates
- Online forums and community support groups focused on C++ programming