A C++ template function allows you to create a function that works with any data type, enabling code reusability and type safety.
template <typename T>
T add(T a, T b) {
return a + b;
}
What are Template Functions?
C++ template functions are a powerful feature of the language allowing you to write generic and reusable code. They enable you to create a single function definition that can work seamlessly with different data types. This not only saves time and effort but also enhances the maintainability of your code.
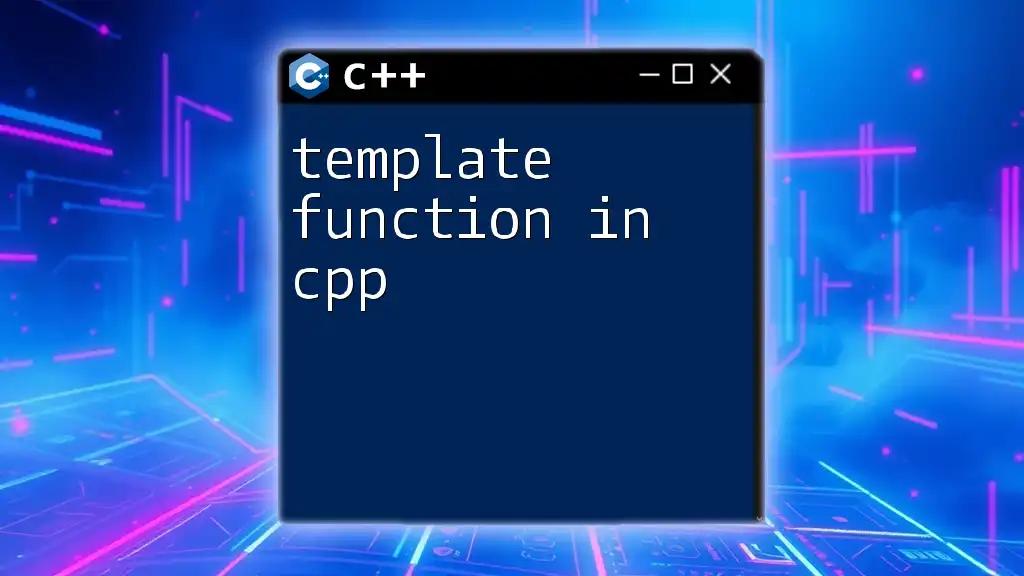
Understanding Templates in C++
What Are C++ Templates?
Templates can be seen as blueprints for functions or classes. They are instrumental in generic programming, allowing you to define operations that can be performed on various data types without rewriting the same function for each one.
There are two main types of templates:
- Function templates: Functions that operate on generic types.
- Class templates: Classes designed to handle a variety of data types.
Syntax of Templates
The basic syntax for defining a template function involves the `template` keyword followed by angle brackets containing a type placeholder. This can be either `typename` or `class`, both of which are interchangeable in the context of function templates.
template <typename T>
T functionName(T param1, T param2) {
// Function body
}
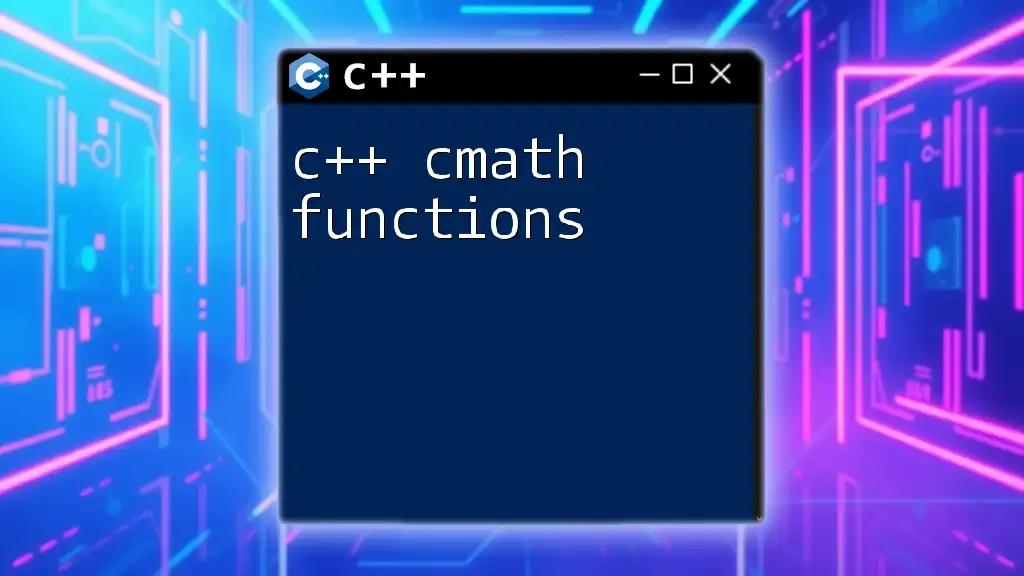
Creating a Template Function
Step-by-Step Guide
Creating a simple template function is straightforward. Let’s consider a function that adds two values:
template <typename T>
T add(T a, T b) {
return a + b;
}
In this example, `T` serves as a placeholder for any data type. The function `add` takes two parameters of type `T` and returns their sum. The versatility of this function allows it to handle different data types like integers, floats, and even custom objects, provided they support the addition operation.
Compiling and Using the Template Function
To compile a program that uses template functions, standard compilation commands apply. The template function is instantiated at the point of use based on the provided argument types. For instance:
int main() {
cout << add(5, 3) << endl; // Integer addition
cout << add(5.2, 3.3) << endl; // Double addition
}
When you run this program, it will output:
8
8.5
This demonstrates how the `add` function works with both integers and floating-point numbers, emphasizing the flexibility of C++ template functions.
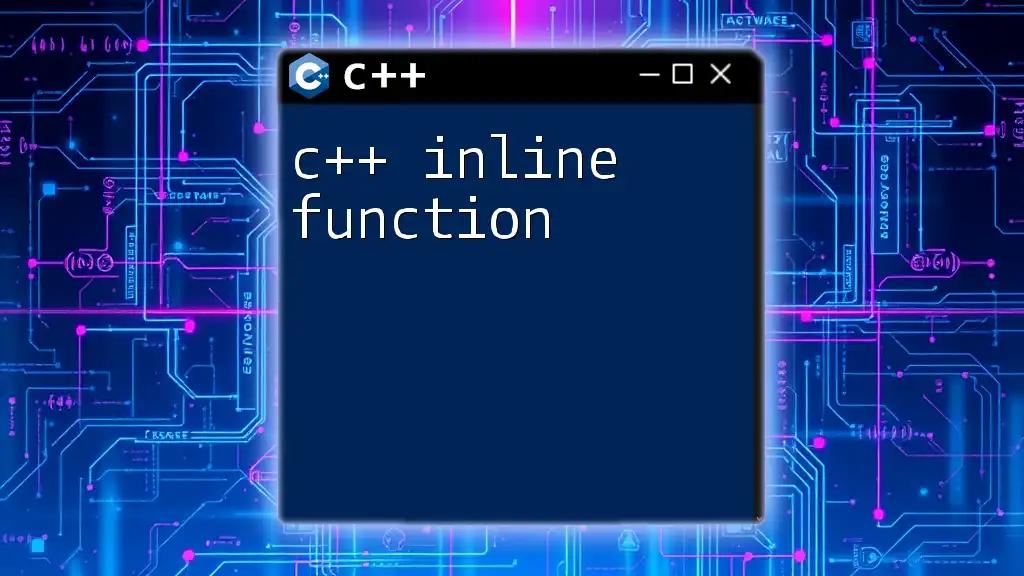
Advantages of Using Template Functions
Code Reusability
One of the primary benefits of using template functions is code reusability. With templates, the same code can handle multiple data types, reducing redundancy and improving the management of your codebase. This leads to cleaner, more organized code that is easier to maintain.
Type Safety
Templates provide type safety during compilation, ensuring that errors related to type mismatches are caught before execution. For instance, if you attempt to add two incompatible types (like an integer and a string), the compiler will raise an error, thus safeguarding against potential runtime issues.
Performance Benefits
Template functions often offer performance advantages, as they can lead to inlining and reduce the overhead associated with virtual function calls. The compiler generates a separate instance of the template for each data type used, resulting in optimized code at runtime.
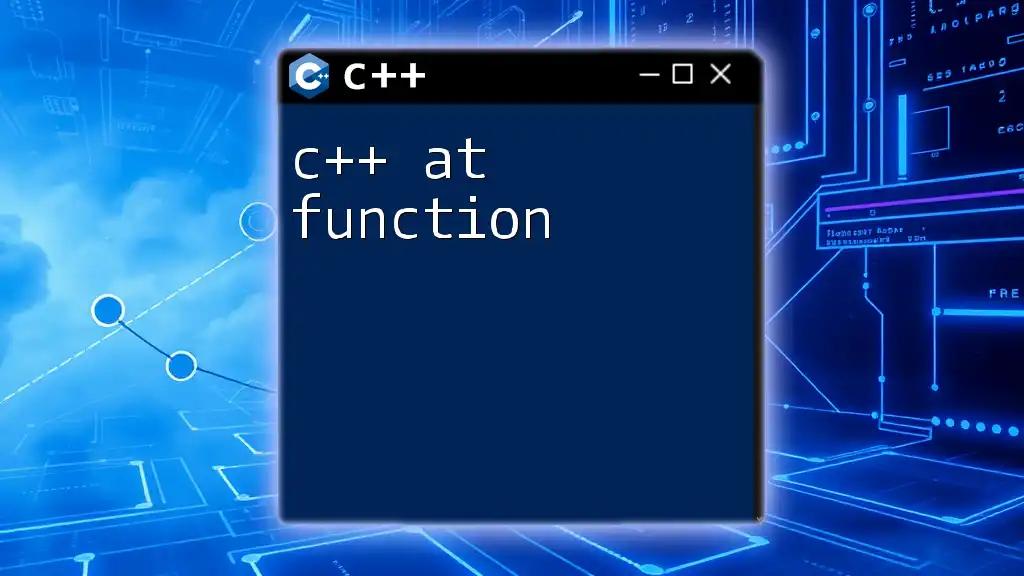
Limitations of Template Functions
Despite their advantages, template functions are not without limitations. For instance, excessive template instantiation can lead to code bloat, making the executable larger. Additionally, the complexity of templates can confuse developers, particularly with advanced features like specialization and metaprogramming.

Advanced Topics in Template Functions
Template Specialization
Template specialization is a powerful feature that allows you to define specific behavior for particular data types when using templates. There are two types of specialization: full and partial.
For example, consider a function that handles the concatenation of strings differently than numeric types:
template <>
string add<string>(string a, string b) {
return a + " " + b; // String concatenation
}
This specialization changes the behavior of the `add` function when it operates on strings, demonstrating the modularity and adaptability of template functions.
Variadic Template Functions
Variadic templates allow you to create functions that accept an arbitrary number of template arguments. This feature enhances flexibility and enables you to build functions that can handle any number of parameters.
Here’s an example of a simple variadic function:
template <typename T, typename... Args>
void print(T first, Args... args) {
cout << first << " ";
print(args...);
}
This function prints all arguments sequentially, regardless of their types, showcasing the dynamic capabilities of C++ templates.
Template Metaprogramming
Template metaprogramming is a method of performing computation at compile time using template features. It enables programmers to write code that dynamically adapts based on types or values.
For instance, you can create static assertions using templates to enforce constraints on type usage, enhancing overall code safety and performance.
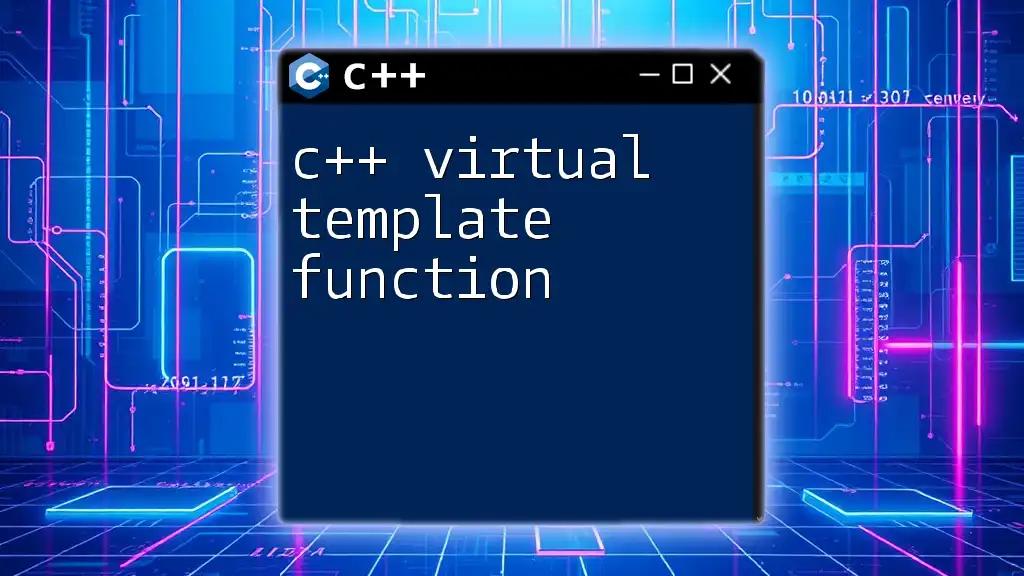
Practical Applications of Template Functions
Real-World Use Cases
Template functions have wide applications in software development, particularly in data structures and algorithms. For example, you can implement generic sort algorithms that operate on any set of elements:
template <typename T>
void sort(vector<T>& vec) {
// Sorting algorithm implementation here
}
This allows you to sort vectors of integers, floats, strings, and even user-defined types without needing to write separate sorting logic for each.
Libraries and Frameworks Utilizing Templates
The C++ Standard Template Library (STL) is a prime example of how templates can be leveraged to provide efficient, generic data structures and algorithms. Containers like `vector`, `list`, and `map` are all implemented as template classes, making them not only versatile but also memory efficient.
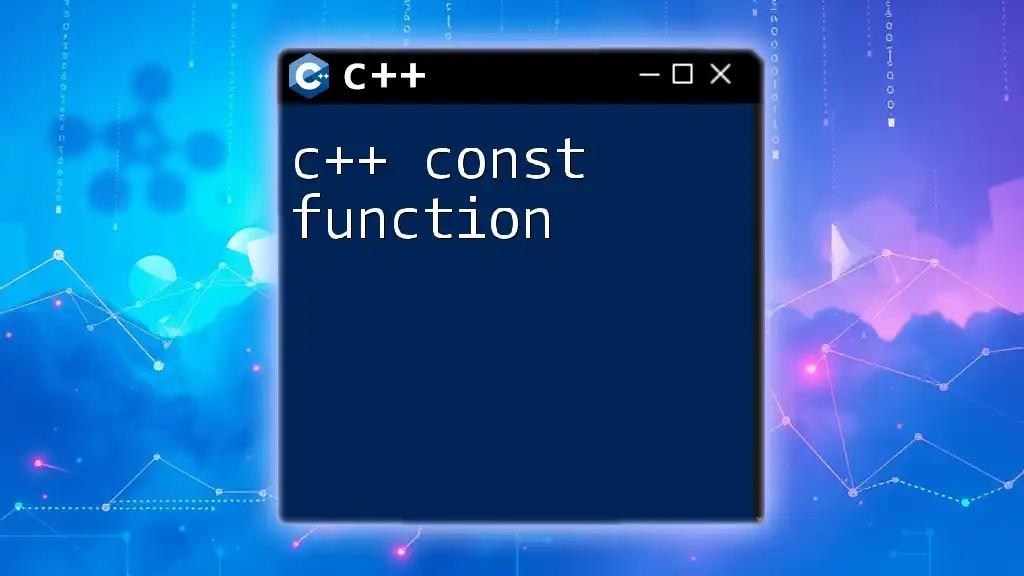
Conclusion
In conclusion, C++ template functions represent a cornerstone of modern C++ programming. Their capacity for code reusability, type safety, and performance enhancement make them an essential tool for developers. By mastering template functions and their advanced features, programmers can elevate their skills and create more efficient, maintainable codebases.