In C++, a nested function refers to a function declared within the body of another function, although it's important to note that C++ does not support nested functions in the same way as some other languages; instead, you can achieve similar functionality using lambda expressions or by defining helper functions within a class.
Here's a simple example using a lambda expression:
#include <iostream>
int main() {
auto square = [](int x) { return x * x; }; // Nested function using lambda
std::cout << "Square of 5: " << square(5) << std::endl;
return 0;
}
What Are Nested Functions?
In C++, a nested function refers to a function that is defined within another function. This structure allows the inner function to access variables and parameters of the outer function, which can simplify code organization and enhance readability. Understanding nested functions is particularly important for programmers who seek to structure their code efficiently and intuitively.
Nested functions are somewhat rare in C++, as they are not a standard feature like in languages such as Python or JavaScript. However, they can still be used in C++ via function objects or lambdas. Recognizing the nuances of using such inner functions can give you a deeper understanding of C++ nested functions and their applications in programming.
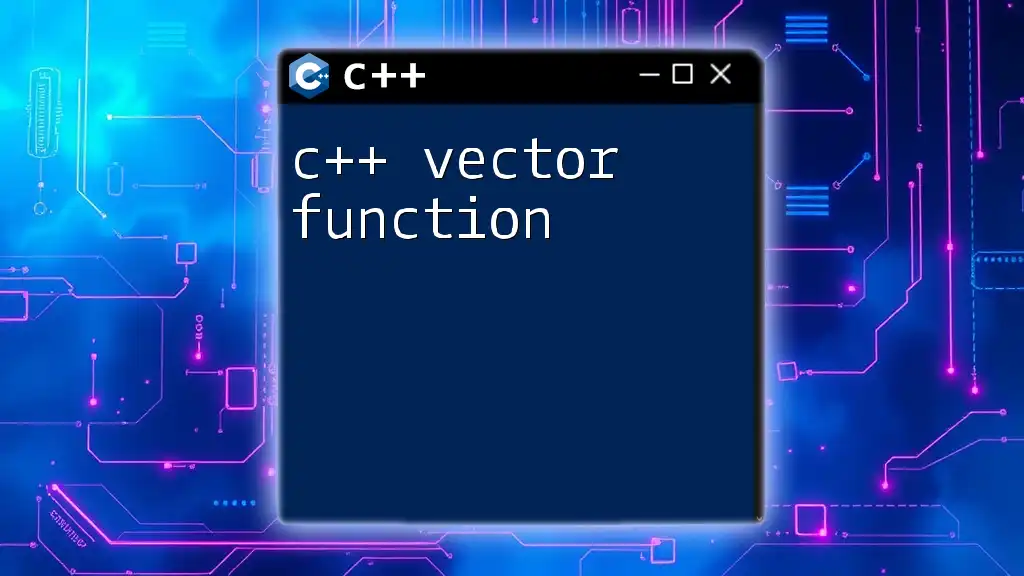
How to Define Nested Functions in C++
When defining a nested function, the syntax resembles that of a standard function, but it is placed inside another function. This nesting allows for more robust encapsulation of functionality. Here's the basic syntax for creating a nested function:
void outerFunction() {
void innerFunction() {
// Inner function logic
}
innerFunction(); // Calling the inner function
}
Example: Simple Nested Function
Let’s look at a basic example that illustrates defining and calling a nested function.
#include <iostream>
using namespace std;
void outerFunction() {
void innerFunction() {
cout << "This is a nested function." << endl;
}
innerFunction();
}
int main() {
outerFunction();
return 0;
}
In this example, the innerFunction is defined within outerFunction, and it simply outputs a message when called.
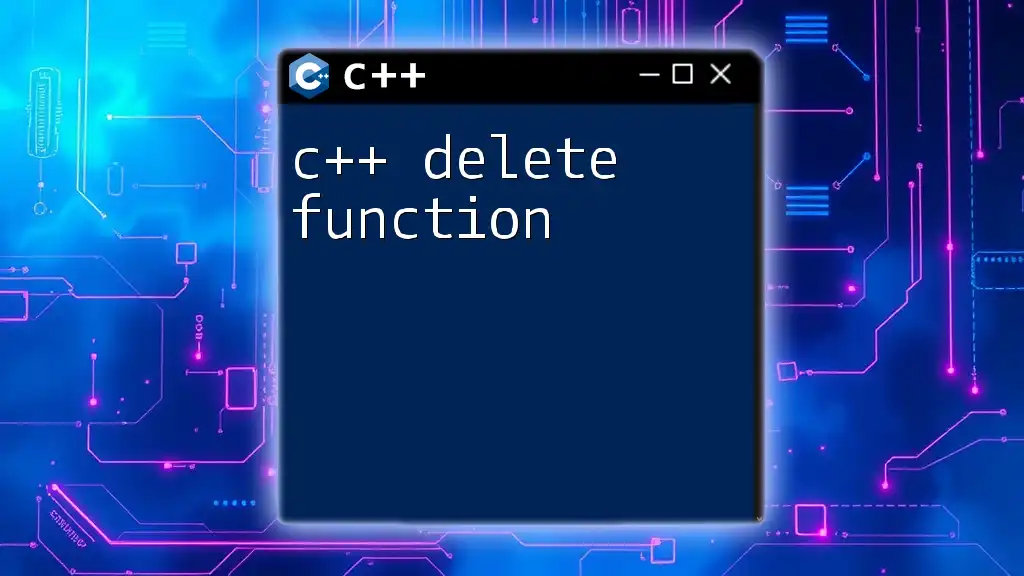
Scope and Lifetime of Nested Functions
Understanding Scope
One of the critical aspects of nested functions is scope. In C++, a nested function has access to the variables declared within the outer function's scope. This means that you can effectively use local variables from the outer function in the inner function without needing additional parameters.
Lifetime of Variables in Nested Functions
The lifetime of the variables in nested functions is determined by their scope. Variables defined in the outer function remain alive as long as the outer function is executing. Here’s an example demonstrating this:
void outerFunction() {
int x = 10; // Variable in outer function
void innerFunction() {
cout << "Value of x is: " << x << endl; // Accessing outer variable
}
innerFunction(); // Calls the inner function which uses x
}
int main() {
outerFunction();
return 0;
}
In this example, the inner function innerFunction can access the variable x defined in outerFunction, demonstrating effective use of scope.
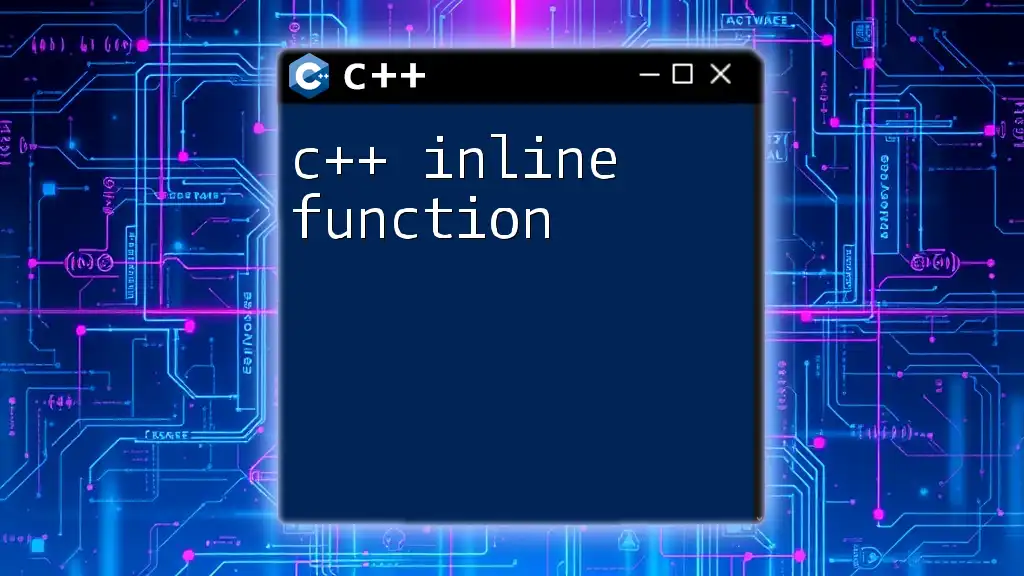
Benefits of Using Nested Functions
Code Organization
Organizing related functions together is one of the chief benefits of using nested functions. This helps to establish a clear hierarchy and flow in your code, making it easier to follow. Keeping inner logic encapsulated within the outer function can improve readability and maintainability.
Encapsulation
Nested functions offer a great means of encapsulation. By defining a function inside another, you can hide its implementation details from the rest of the program. This helps in reducing the potential for name conflicts, as the inner function is scoped within the outer function.
Improved Functionality
Another benefit of nested functions is their ability to create closures. Closures can capture the outer function's variables and use them later in the nested function, which enhances functionality and code flexibility.
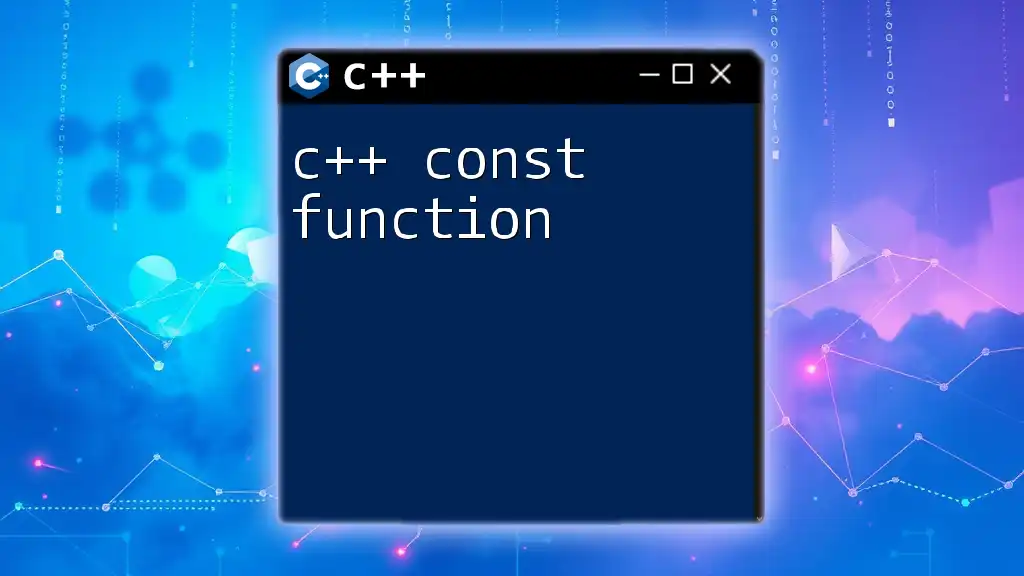
Potential Drawbacks of Nested Functions
Readability Concerns
While nested functions have numerous advantages, they can also lead to readability concerns. Overusing nested functions may make code complex and difficult to follow. It can also introduce cognitive overload for someone unfamiliar with your code.
Performance Overheads
Nested functions might impose some performance overhead due to the additional layers of function calls. Thus, in performance-critical applications, it's essential to analyze whether their use will meet efficiency requirements.
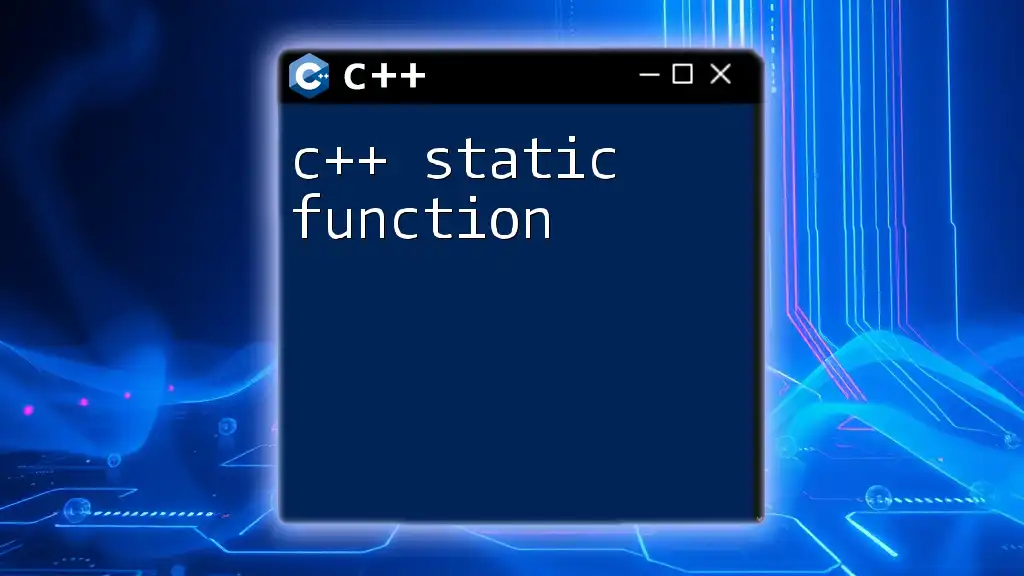
Examples of Nested Functions in Real-Life Applications
Case Study: Mathematical Computations
Nested functions can simplify complex calculations. Here’s how you might use one in a mathematical context:
double calculate(double a, double b) {
double multiply() {
return a * b; // Inner function performing multiplication
}
return multiply(); // Calling inner function to get the result
}
int main() {
cout << "The product is: " << calculate(5, 4) << endl; // Outputs "The product is: 20"
return 0;
}
In this function, the multiply inner function handles the multiplication logic, making the calculation clearer and more organized.
Case Study: Data Processing
In data processing scenarios, nested functions can help in organizing various tasks of filtering and manipulation neatly. You can encapsulate different parts of your logic while still accessing shared variables.
void processData() {
int total = 0; // Shared variable for accumulation
void addToTotal(int value) {
total += value; // Inner function manipulating outer variable
}
addToTotal(5);
addToTotal(10);
cout << "Total is: " << total << endl; // Outputs "Total is: 15"
}
int main() {
processData();
return 0;
}
In this processing example, the addToTotal inner function allows you to manipulate the total variable succinctly.
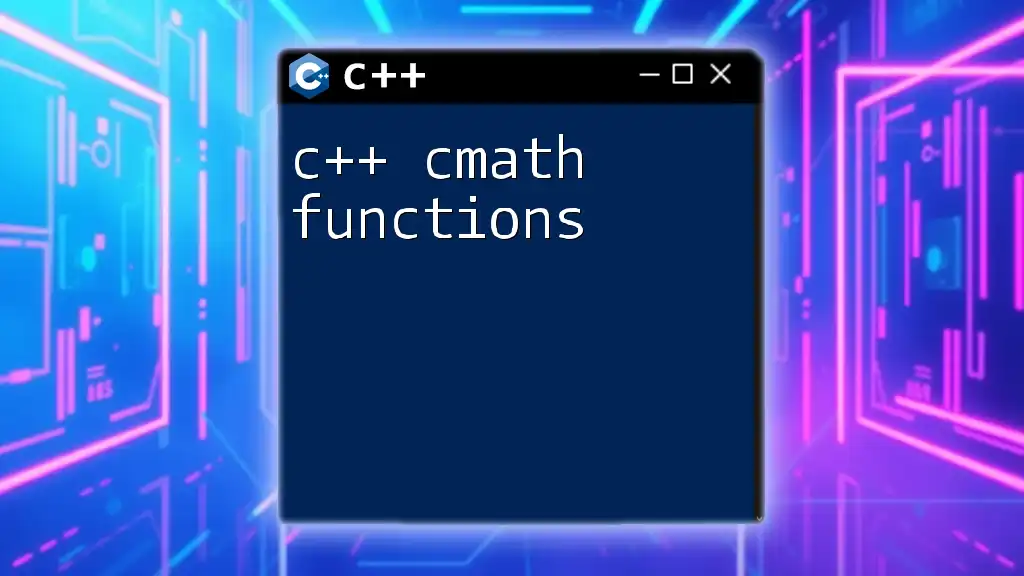
Nested Functions vs Lambda Expressions
Understanding the Differences
As C++ evolves, lambda expressions have gained popularity. While nested functions can be helpful, lambda expressions often provide a more flexible, concise solution for small tasks without needing a formal function definition.
Example Comparison
Consider the following example that demonstrates using a lambda expression instead of a nested function:
void outerFunction() {
auto lambdaFunc = [](int x) { return x * 2; }; // Lambda expression
cout << "The result is: " << lambdaFunc(5) << endl; // Outputs "The result is: 10"
}
int main() {
outerFunction();
return 0;
}
Here, using a lambda simplifies the syntax while retaining functional capabilities. It also allows for creating anonymous functions that can be passed around easily.
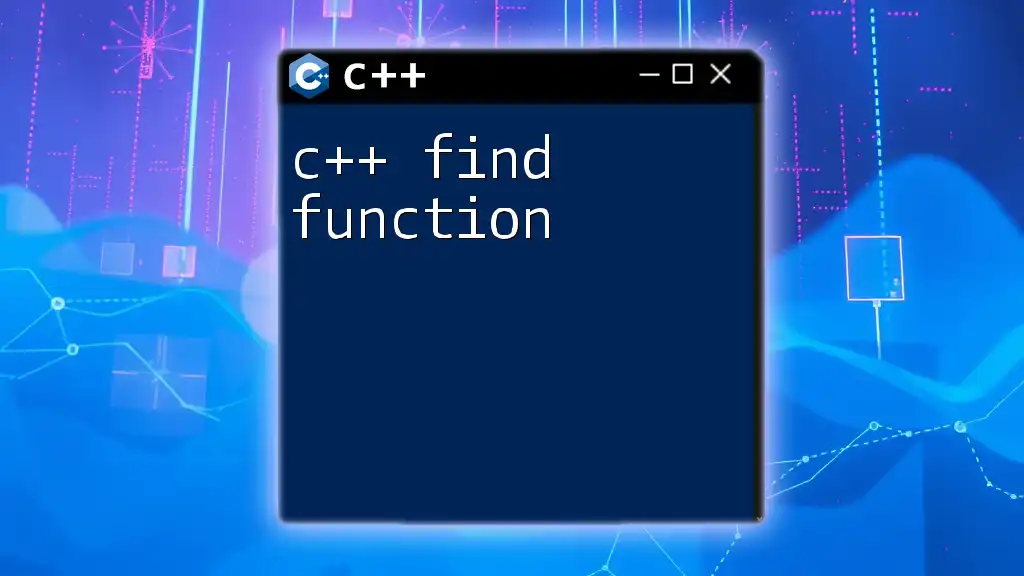
Conclusion
Over the course of this article, we’ve explored the concept of C++ nested functions, diving into their definitions, benefits, potential drawbacks, and practical applications. While nested functions can be a powerful tool for code organization and encapsulation, they require careful consideration to avoid compromising code readability and performance.
Embracing the practice of implementing nested functions can enhance your programming skills, helping you to write cleaner, more maintainable C++ code. Whether you are a novice programmer or an experienced developer, experimenting with nested functions will deepen your understanding of function scopes and closures.
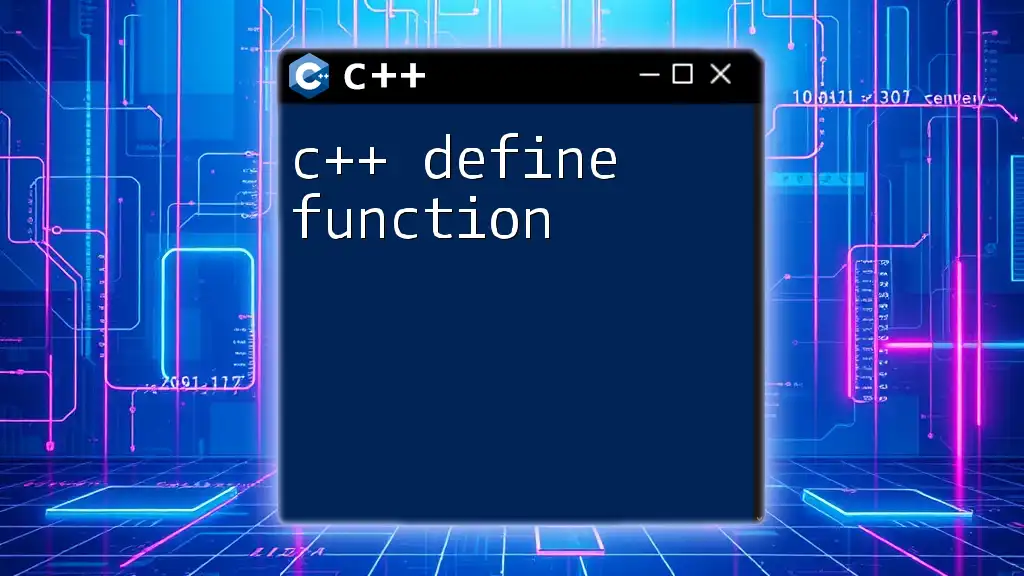
Additional Resources
For further exploration into C++ programming, consider referring to recommended books, tutorials, and documentation. Online communities and forums can also offer valuable insights and support as you continue your journey in mastering C++.
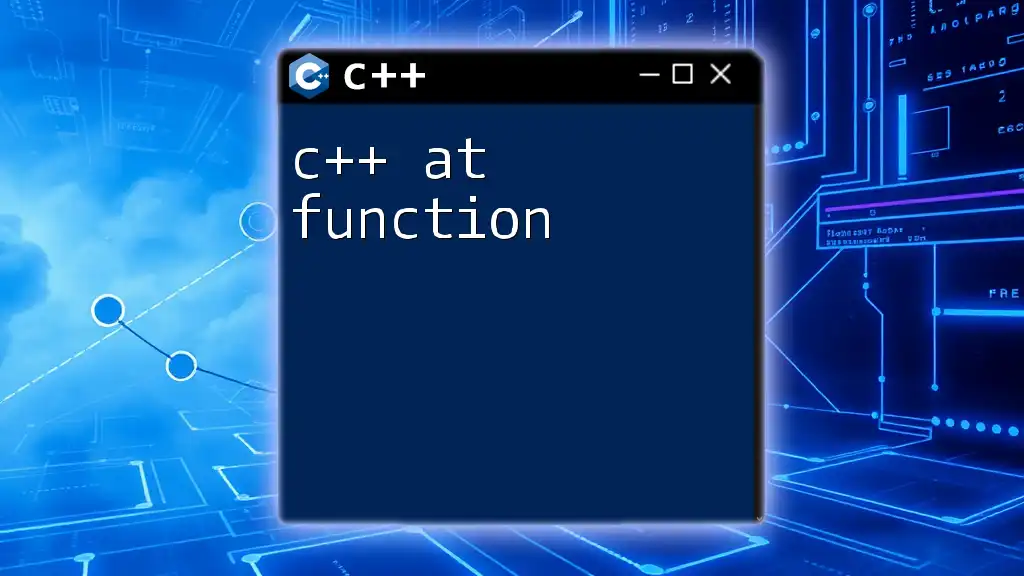
Call to Action
Stay engaged in your programming journey by subscribing for more tips and workshops focused on C++. We invite you to share your experiences, insights, and code snippets using nested functions to foster a collaborative learning environment.