C++ abstraction is a programming principle that allows you to hide complex implementation details and expose only the necessary parts of an object, improving code readability and maintainability.
#include <iostream>
using namespace std;
class Shape {
public:
virtual void draw() = 0; // Pure virtual function
};
class Circle : public Shape {
public:
void draw() override {
cout << "Drawing Circle" << endl;
}
};
class Square : public Shape {
public:
void draw() override {
cout << "Drawing Square" << endl;
}
};
int main() {
Shape* shape1 = new Circle();
Shape* shape2 = new Square();
shape1->draw(); // Output: Drawing Circle
shape2->draw(); // Output: Drawing Square
delete shape1;
delete shape2;
return 0;
}
Understanding C++ Abstraction
What is Abstraction in C++?
C++ abstraction is a fundamental concept in object-oriented programming that focuses on hiding the complex implementation details of a system while exposing only the necessary and relevant parts to the user. By emphasizing only the essential characteristics of an object, abstraction allows developers to manage complexity and enhance code usability.
The primary goal of abstraction is to simplify interactions with the software by promoting a cleaner interface for users and other developers. As a result, abstraction leads to code that is easier to understand, maintain, and extend.
Why Use Abstraction?
There are several benefits to incorporating abstraction into your C++ programming practices:
-
Hiding Complexity: By abstracting complex functionality, you allow users to interact with simpler interfaces. This separation of concerns allows them to utilize high-level commands without needing to understand the underlying implementations.
-
Reducing Code Duplication: Abstraction encourages the reuse of code. When you define common functionality in abstract forms (like classes and interfaces), you can implement it in various concrete classes, reducing redundancy.
-
Enhancing Usability: Clear abstractions improve usability. Well-designed abstract classes and interfaces lead to systems that are intuitive and easier to navigate.
Consider a real-world analogy: when you drive a car, you use an abstract interface—the steering wheel, pedals, and dashboard—to interact with a complex system of engines, brakes, and electronics. You don’t need to understand every detail of these systems to successfully operate the vehicle.
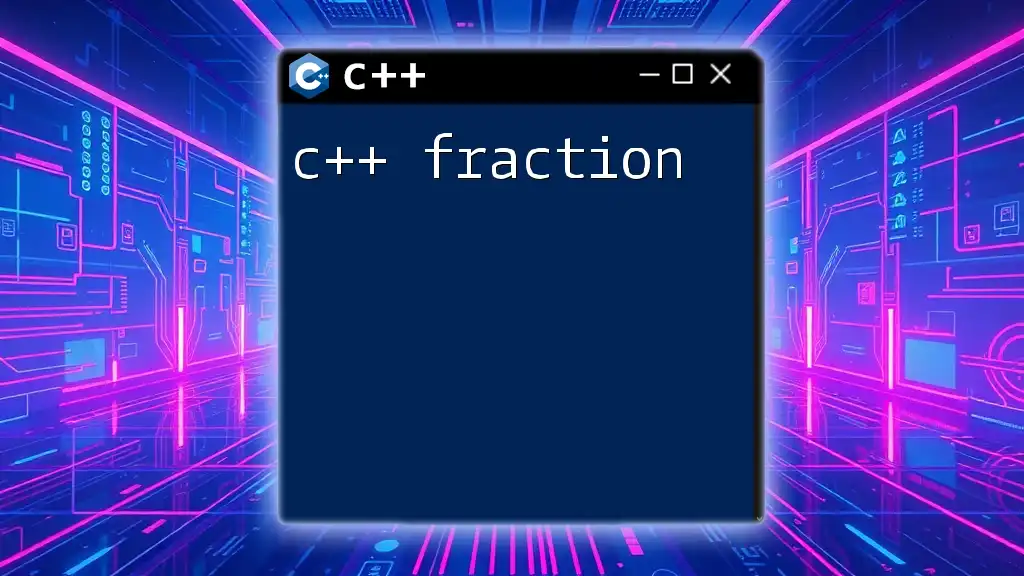
Key Concepts of Abstraction in C++
Abstract Classes
An abstract class serves as a blueprint for other classes and defines a common interface with at least one pure virtual function. This means it cannot be instantiated directly.
Here’s an illustration of how to declare an abstract class in C++:
class AbstractClass {
public:
virtual void pureVirtualFunction() = 0; // Pure virtual function
};
When you define an abstract class, you typically outline the methods that must be implemented by any derived class, allowing different implementations while maintaining a consistent interface.
Abstract classes are particularly beneficial in defining fundamentals that various entities share. For example, you might create an abstract class `Animal` with methods like `speak()` that are defined differently for `Dog` and `Cat`.
Interfaces
While not formally defined in C++, C++ utilizes a similar concept known as an interface. An interface is simply a class that contains only pure virtual functions and no data members.
The key aspect of interfaces in C++ is their ability to promote polymorphism, allowing different classes to implement the same method in different ways. Here’s an example of how you might define an interface:
class IShape {
public:
virtual void draw() = 0; // Pure virtual function
};
In practice, you might have several shape classes, such as `Circle` and `Rectangle`, each implementing the `draw()` method according to its own logic.
Concrete Classes
Concrete classes are implementations of abstract classes and interfaces. They provide the functionality defined in the abstract parts and make the methods operational.
For instance, here's how you might implement the IShape interface for a `Circle` class:
class Circle : public IShape {
public:
void draw() override {
// Drawing code for Circle
std::cout << "Drawing Circle" << std::endl;
}
};
By defining concrete classes like `Circle` and `Rectangle`, you ensure that they provide specific behavior while adhering to the interface's design.
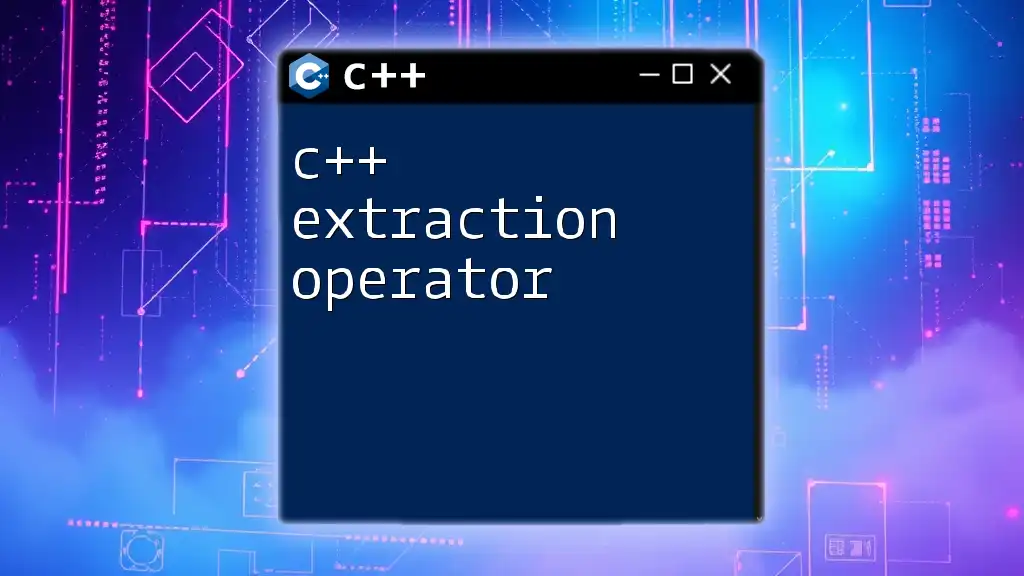
Implementing Abstraction in C++
Step-by-Step Process to Implement Abstraction
When implementing abstraction in a project, follow these steps:
-
Establish the Need for Abstraction: Analyze your project requirements and identify objects or functionalities that can benefit from abstraction.
-
Create Formal Definitions for Abstraction: Define your abstract classes and interfaces clearly. Think through what common behaviors or properties they should encapsulate.
-
Design and Implement Classes: Create concrete classes that inherit from your abstract classes/interfaces, ensuring that they correctly implement the required methods.
For example, consider designing a geometric shape library. You could define an abstract class for shapes:
class Shape {
public:
virtual double area() const = 0; // Pure virtual method
};
Then, you would implement concrete classes for different shapes like `Circle` and `Square`, where each concrete class provides its calculation for the area.
Real-World Example: Banking System
To illustrate the utility of abstraction, let’s consider a banking system. By defining an abstract class for accounts, you can implement various types of accounts, such as Savings and Current accounts with distinct behaviors.
An example of defining an abstract class for accounts might look like this:
class Account {
public:
virtual void deposit(double amount) = 0; // Pure virtual function
virtual double getBalance() const = 0; // Pure virtual function
};
Concrete classes can then provide specific implementations of these functions:
class SavingsAccount : public Account {
private:
double balance;
public:
SavingsAccount() : balance(0.0) {}
void deposit(double amount) override {
balance += amount;
}
double getBalance() const override {
return balance;
}
};
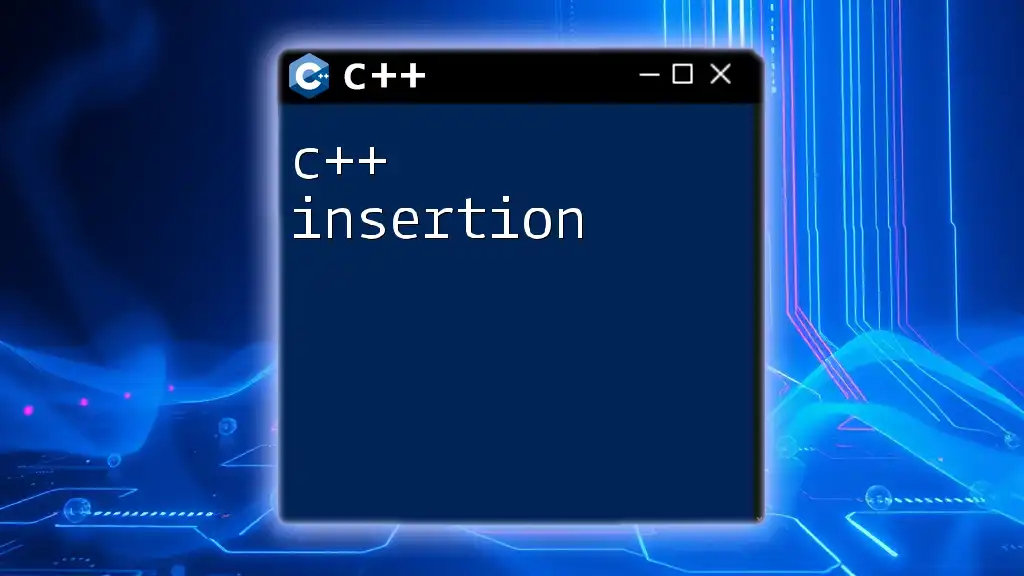
Best Practices for C++ Abstraction
Tips for Effective Use of Abstraction
-
Balance: The key is finding a balance between abstraction and complexity. While abstraction simplifies usage, too much abstraction can lead to confusion and hinder performance.
-
When to Abstract: Use abstraction when you foresee alternatives or need to implement diverse behaviors. If a feature is likely to evolve or be expanded upon, an abstract approach is often appropriate.
-
Documentation: Providing clear documentation for abstract classes and interfaces helps others understand their usage without diving into their complexities.
Common Pitfalls in Abstraction
One significant pitfall to avoid is over-abstraction. This occurs when developers create overly complex hierarchies of abstract classes and interfaces. This can result in unnecessary complexity and hinder performance.
Stay vigilant against implementing features that don’t require abstraction. Unnecessary abstraction can lead to convoluted code that becomes difficult to maintain.
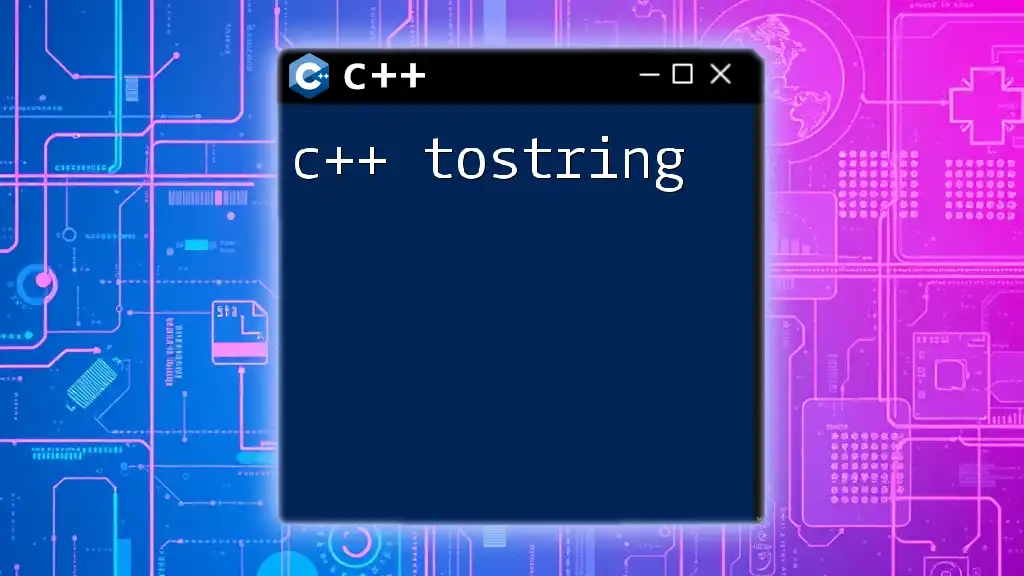
Conclusion
C++ abstraction is an indispensable tool for managing software complexity through effective design and code organization. By utilizing abstract classes and interfaces, developers can create flexible, maintainable, and reusable code. Understanding and applying these principles will undoubtedly enhance your skills in C++ programming.
As you continue your journey in learning C++, focus on recognizing the right moments to implement abstraction in your projects. Doing so will not only improve your code quality but also elevate the overall structure of your applications.