The `std::to_string` function in C++ is used to convert various numeric types (such as `int`, `float`, and `double`) to their equivalent `std::string` representations.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <string>
int main() {
int number = 42;
std::string strNumber = std::to_string(number);
std::cout << "The string representation of the number is: " << strNumber << std::endl;
return 0;
}
What is `to_string`?
C++ `to_string` is a powerful utility that facilitates the conversion of various data types into a `std::string`. This function is particularly useful when you need to generate human-readable forms of numeric values for logging, displaying in user interfaces, or preparing data for storage in text formats. In a programming landscape where data manipulation is key, mastering the `to_string` function is essential for any C++ developer.
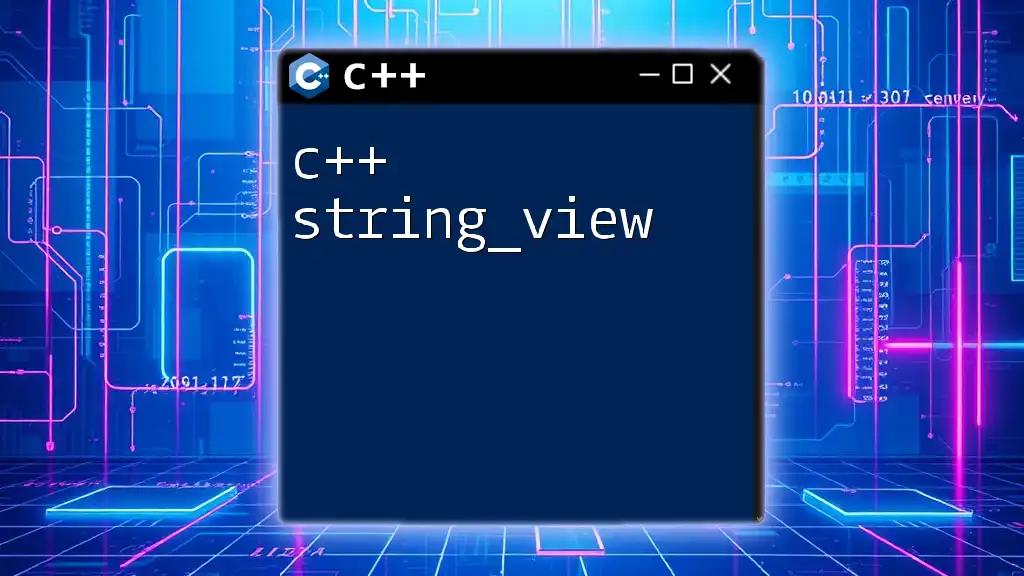
Why Use `to_string`?
One of the primary reasons to use `to_string` is its ability to quickly convert data types into strings without the complexities and boilerplate of alternative methods. You can readily format text messages or store numerical data in a string representation, making it a versatile tool to have in your C++ toolkit. For example, when combining strings and numerical values, `to_string` simplifies the process.
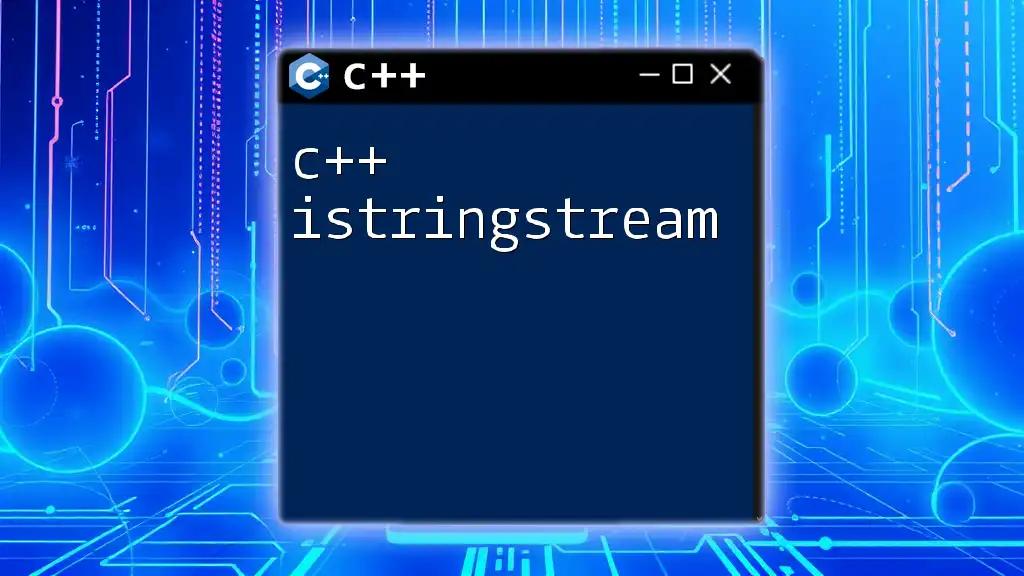
The Function Signature
The `to_string` function has a straightforward syntax that looks like this:
std::string to_string(int value);
The function accepts a parameter of various supported types and returns a `std::string`. Here's a brief overview of its function signatures for different types:
- `to_string(int)`
- `to_string(long)`
- `to_string(unsigned long)`
- `to_string(float)`
- `to_string(double)`
- `to_string(long double)`
Understanding these signatures allows you to confidently use `to_string` for type conversions across numerical values.

Supported Types
The `to_string` function supports several integral and floating-point types, ensuring flexibility in what you can convert to strings. Below are the common types that can be converted:
-
Integers: Converts integral types directly into string format.
std::string str = std::to_string(42); // "42"
-
Floating-point numbers: Converts floats and doubles to string, it is important to note the potential loss of precision.
std::string pi = std::to_string(3.14); // "3.140000"
-
Other types: You can also convert types like `long`, `unsigned long`, and `long double`.
Practical Examples of `to_string`
Example with Integers: One of the simplest yet effective uses of `to_string` involves concatenating variables with strings. Imagine you want to create a message for a user. Here’s how it might look:
int age = 25;
std::string message = "I am " + std::to_string(age) + " years old.";
std::cout << message; // Outputs: I am 25 years old.
In this example, we combined the string "I am " with the string representation of the integer `age`, producing a complete and understandable message.
Example with Floating-point Numbers: When working with floating-point values, `to_string` helps maintain readability. Here’s how you might use it:
double pi = 3.14159;
std::string piString = std::to_string(pi);
std::cout << "Pi is approximately " << piString; // Outputs: Pi is approximately 3.141590
Notice how all six decimal digits are retained. However, you may want a more concise representation. Be mindful of how you format floating-point values.

Common Pitfalls and Issues
Precision Loss in Floating-point Conversion: One common issue with using `to_string` is that it may not always retain the precision you expect, especially when dealing with floating-point numbers. For instance, converting `3.14` might give you `3.140000`, which may not align with your anticipated format. Understanding this limitation is crucial to avoid unexpected outcomes in your applications.
Locale Sensitivity: The output of `to_string` can also change depending on the current locale settings of the program. This can impact how decimal points and commas are represented. If your application is designed for international use, testing across different locales is a good practice to ensure consistent results.
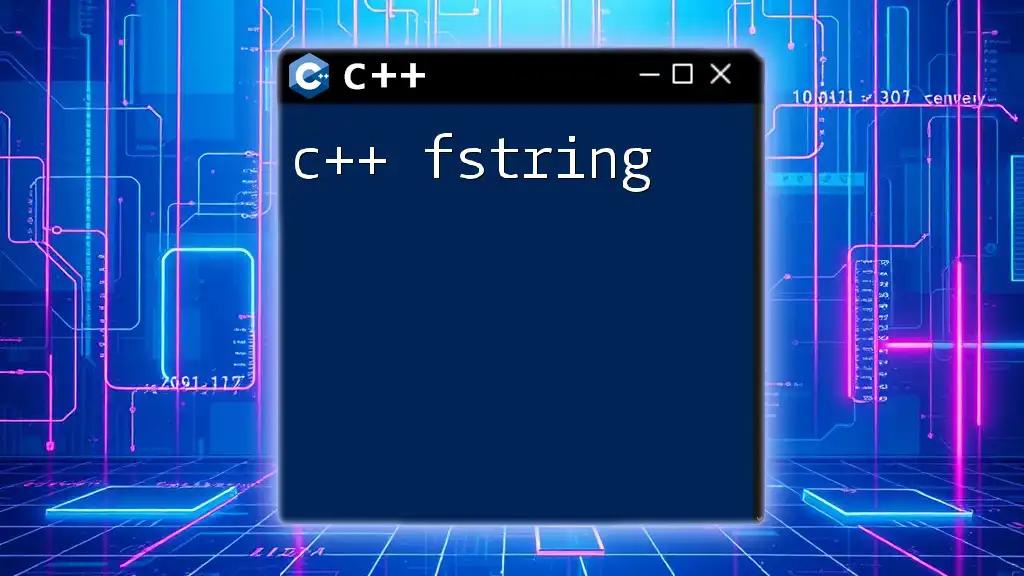
Alternatives to `to_string`
While `to_string` is convenient, there are scenarios where alternatives might be more suitable.
Using String Streams: Another commonly used method is `std::ostringstream`, which provides more formatting flexibility compared to `to_string`. Here's how it looks:
#include <sstream>
#include <iostream>
std::ostringstream oss;
oss << 42;
std::string str = oss.str();
std::cout << str; // Outputs: 42
Using `ostringstream` allows you to format output with specific decorations or precision, making it advantageous in scenarios where custom formatting is needed.
Using `sprintf`: The `sprintf` function from the C standard library can also be employed for formatting strings. Here’s a quick example:
#include <cstdio>
char buffer[50];
sprintf(buffer, "Value: %.2f", 3.14);
std::cout << buffer; // Outputs: Value: 3.14
However, `sprintf` is not type-safe and can lead to buffer overruns if not carefully managed, making it less ideal in modern C++.
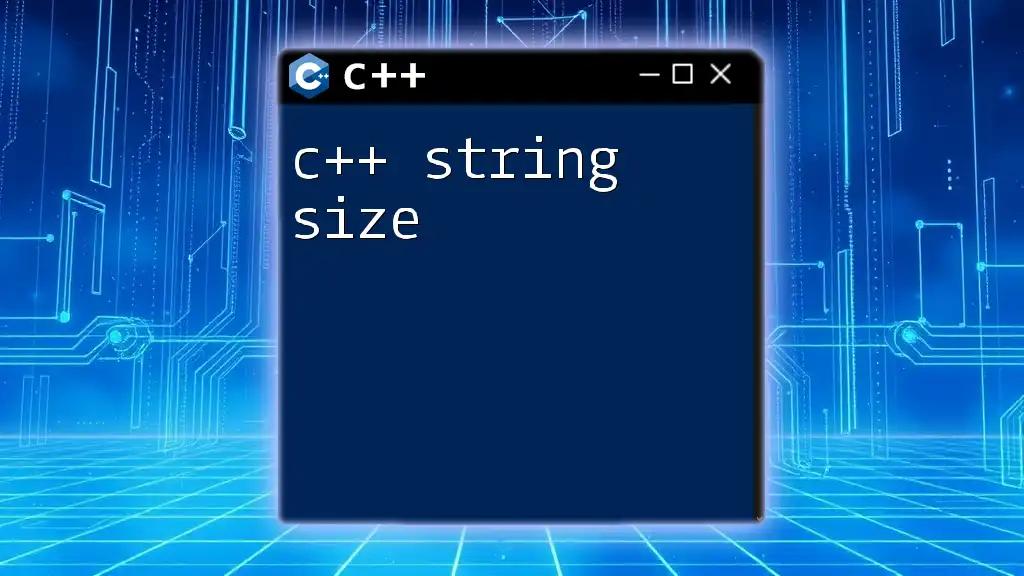
Performance Considerations
When using `to_string`, it is generally efficient for most applications. However, it’s important to consider when to optimize. If your code deals with numerous conversions in performance-critical sections, profiling your application is paramount to understand the impact and potentially seek optimizations or alternatives, such as `ostringstream`, that might perform better in specific scenarios.

Conclusion
In conclusion, the `to_string` function in C++ is a robust and convenient way to convert various data types into strings. Understanding its function signatures, supported types, practical applications, and potential issues will empower you to leverage its capabilities effectively. Practice using `to_string` in different contexts to get a solid grasp of how it can make your code cleaner and more efficient.
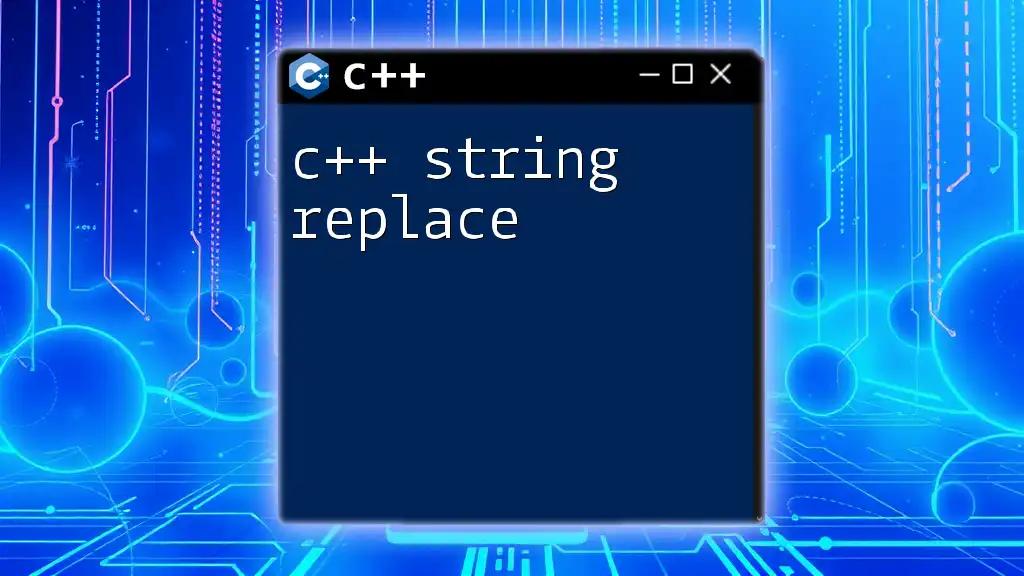
Additional Resources
For further reading, refer to the official C++ documentation, which offers detailed insights into `to_string` and related functions. Additionally, consider exploring C++ programming books or online courses to deepen your understanding and enhance your skills.

Call to Action
Finally, we invite you to share your experiences using `to_string` in your projects. Have you encountered any challenges or discovered interesting use cases? Engaging with the community can provide invaluable insights and foster collaborative learning.