In C++, `std::format` from the C++20 standard allows you to create formatted strings similarly to f-strings in Python, enabling concise and readable string formatting.
#include <iostream>
#include <format>
int main() {
int value = 42;
std::string message = std::format("The answer is {}", value);
std::cout << message << std::endl;
return 0;
}
Understanding C++ FStrings
What is an f-string in C++?
An f-string or formatted string in C++ is a concise way to directly embed variable values within a string. This powerful feature simplifies the task of string interpolation, which allows developers to construct dynamic strings by including variable expressions in a readable format. The key advantage of using f-strings is their ability to enhance code readability, making it easier for developers to understand the intent behind the code at a glance.
Key Characteristics of C++ FStrings
String Interpolation
String interpolation is a process through which placeholders in a string can be replaced with actual variable values. With f-strings, this interpolation can be done seamlessly. Instead of concatenating strings using the `+` operator, f-strings enable a more straightforward approach. They allow variables to be inserted directly into the string.
Simplicity and Conciseness
Consider the following traditional method of concatenating strings in C++:
#include <iostream>
#include <string>
int main() {
int age = 30;
std::string message = "I am " + std::to_string(age) + " years old.";
std::cout << message;
return 0;
}
The above code is functional but can be cumbersome when dealing with multiple variables. F-strings can greatly simplify this process and minimize errors due to misplaced operators.
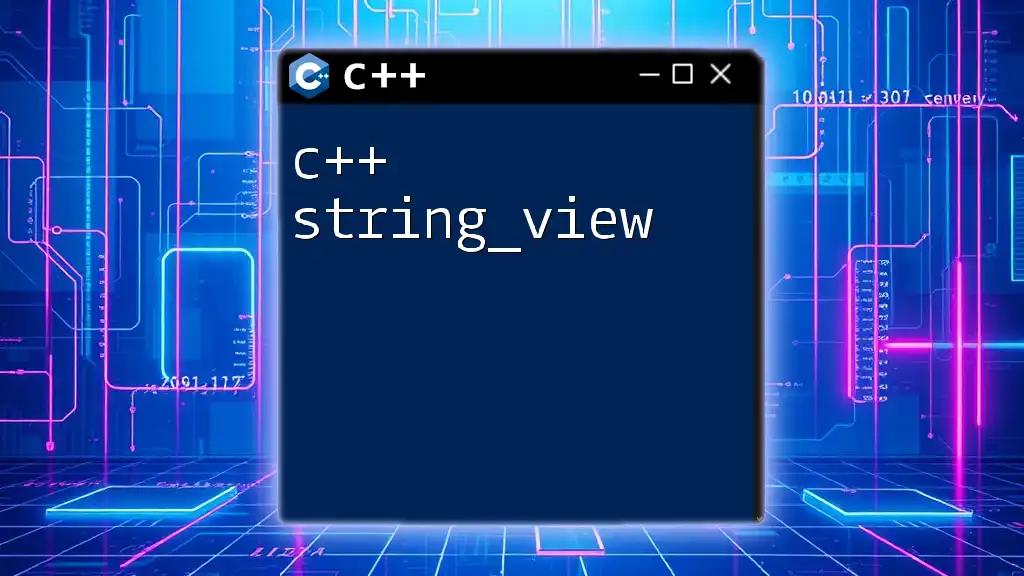
Syntax and Usage of C++ FStrings
Basic Syntax of C++ FStrings
The syntax for creating f-strings in C++ is straightforward. You can simply insert a variable inside curly braces `{}` within a string. Here's an example illustrating this:
#include <iostream>
#include <string>
int main() {
std::string name = "Alice";
std::cout << "Hello, " + name + "!" << std::endl;
return 0;
}
In this case, the variable `name` is directly included in the string, significantly enhancing readability.
Advanced Usage of C++ FStrings
Formatting Options
F-strings in C++ allow for various formatting options. For instance, you can specify how numeric outputs are displayed. This can be extremely useful for making numeric data more readable or presented just as you want.
Multi-line f-strings
Creating multi-line f-strings can also be done efficiently. Here’s an example:
#include <iostream>
#include <string>
int main() {
std::string multiline = R"(
Hello, World!
This is a multi-line f-string example.
)";
std::cout << multiline;
return 0;
}
This multi-line f-string approach keeps your code clean and easy to follow.
Escaping Characters
Sometimes string literals require escape sequences, particularly when including quotes or backslashes. Using f-strings, you can manage these characters easily. For instance, to include quotes within your string, you can use the escape character (`\`):
#include <iostream>
#include <string>
int main() {
std::string text = "He said, \"Hello!\"";
std::cout << text << std::endl;
return 0;
}
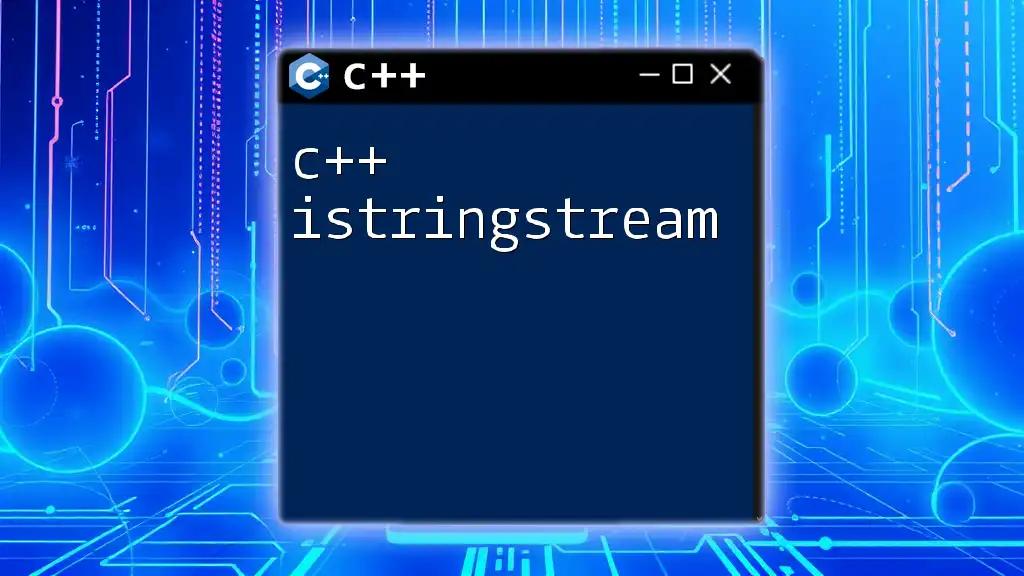
Best Practices for Using C++ FStrings
Keeping Code Readable
When utilizing f-strings, prioritize clarity. Avoid complex interpolations where possible. Keeping your f-strings simple helps others (and future you) understand what's happening at a glance. It is important not to overcomplicate lines; if a single f-string becomes too complex, consider breaking it into multiple lines or variables.
Performance Considerations
Efficiency in coding is crucial. While f-strings improve readability, developers must also be aware of performance implications. F-strings can be more memory efficient compared to traditional methods, especially when handling large strings. However, lazy initialization and clear memory practices should still be considered when working in performance-critical applications.

Common Mistakes and Troubleshooting
Common Errors when Using FStrings
As with any powerful feature, it’s easy to make mistakes. One common error is forgetting to include the right data type within the curly braces. Often, developers may overlook casting when trying to concatenate different types. Always ensure variables are explicitly cast where necessary.
Debugging FStrings
When debugging issues, proper inspection of variable values is indispensable. Use simple output statements to check the contents of interpolated variables. For instance, take a look at this snippet for debugging:
#include <iostream>
#include <string>
int main() {
std::string name = "Alice";
std::cout << "Debugging: " << name << std::endl; // Inspecting variable value
return 0;
}
Printing out variable values prior to their use in f-strings can help eliminate confusion.
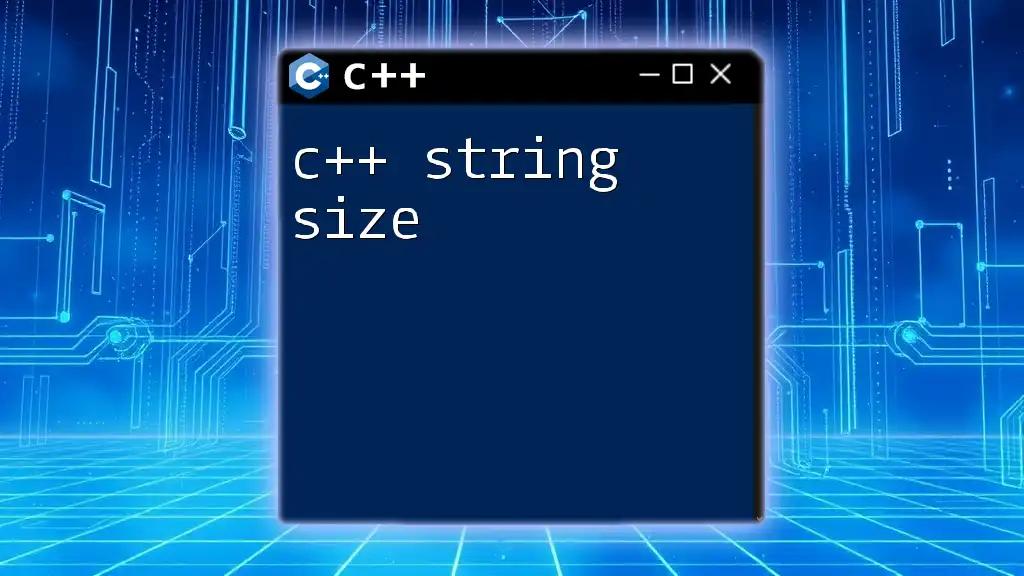
Conclusion
In conclusion, C++ fstrings are a powerful tool that significantly enhance string manipulation and interpolation in C++. They enable cleaner, more concise code, allowing developers to create strings that are both functional and easy to read. Utilizing f-strings can lead to writing more efficient and maintainable code, making them an essential part of any C++ programmer's toolkit.

Call to Action
Ready to dive deeper into the world of C++? Join our community and subscribe to stay updated on more tutorials, tips, and tricks about mastering C++ and related programming topics! Keep an eye out for our upcoming posts where we will explore advanced C++ concepts and powerful string manipulation techniques.