The `find_first_of` function in C++ is used to locate the position of the first occurrence of any character from a specified set in a string.
#include <iostream>
#include <string>
int main() {
std::string str = "hello world";
std::string chars = "aeiou"; // vowels to search for
size_t position = str.find_first_of(chars);
if (position != std::string::npos) {
std::cout << "First vowel found at position: " << position << std::endl;
} else {
std::cout << "No vowels found." << std::endl;
}
return 0;
}
Understanding the Basics of `find_first_of`
What is `find_first_of`?
`find_first_of` is a string manipulation function in C++ that allows you to search for the first occurrence of any character from a specified set within a string. This function is a member of the `std::string` class and is useful for locating individual characters in strings. It stands out compared to similar functions like `find()` and `find_last_of()`, which serve different purposes (like finding a substring or the last occurrence of a character, respectively).
Syntax of `find_first_of`
The syntax for `find_first_of` is as follows:
size_t find_first_of(const std::string& str, size_t pos = 0) const noexcept;
Breaking down the parameters:
- `str`: This parameter refers to the string containing a set of characters that you want to search for.
- `pos`: This optional parameter indicates the starting position from which to search. By default, it is set to 0, meaning the search will begin from the start of the string.
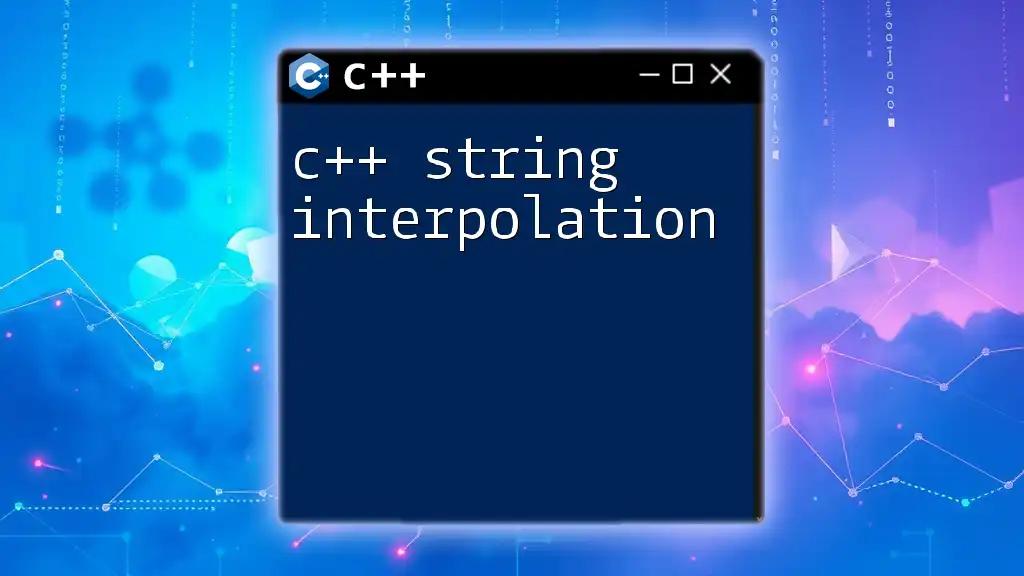
How `find_first_of` Works
Explanation of Functionality
When invoking `find_first_of`, the function scans the string from the specified position for any character that exists in the provided character set (`str`). If a character from the set is found, the function returns the index (position) of its first occurrence. If no characters from the specified string are found in the target string, `find_first_of` returns `std::string::npos`, a constant that indicates the absence of a match.
Example of Using `find_first_of`
Here’s a simple example demonstrating a basic use case of `find_first_of`:
#include <iostream>
#include <string>
int main() {
std::string sample = "Hello, World!";
size_t position = sample.find_first_of("aeiou");
if (position != std::string::npos) {
std::cout << "First vowel found at position: " << position << std::endl;
} else {
std::cout << "No vowels found." << std::endl;
}
return 0;
}
In this example, the program looks for the first vowel in the string "Hello, World!". The output would indicate the index of the first vowel found, providing a clear and practical demonstration of how `find_first_of` operates.

In-Depth Examples
Example with Dynamic Input
To further illustrate `find_first_of`, consider this interactive example where user input is incorporated:
#include <iostream>
#include <string>
int main() {
std::string input;
std::cout << "Enter a string: ";
std::getline(std::cin, input);
size_t position = input.find_first_of("xyz");
if (position != std::string::npos) {
std::cout << "'x', 'y', or 'z' found at position: " << position << std::endl;
} else {
std::cout << "None of 'x', 'y', or 'z' found." << std::endl;
}
return 0;
}
In this case, the program prompts the user to input any string and searches for the characters ‘x’, ‘y’, or ‘z’. This adds a level of interactivity while demonstrating the function’s capability to handle various input scenarios.
Searching Multiple Characters
`find_first_of` can also be used to search for a broader range of characters. Below is an example that identifies the first digit from a sentence:
std::string text = "Find the first occurrence of any digit: 13245.";
size_t pos = text.find_first_of("0123456789");
if (pos != std::string::npos) {
std::cout << "First digit found at position: " << pos << std::endl;
}
This example reveals how `find_first_of` can be effectively utilized to locate numeric characters within a string that contains both letters and numbers.
Working with Character Arrays
You can also use `find_first_of` with C-style strings. Here’s how:
const char* text = "C++ programming is fun!";
size_t pos = std::string(text).find_first_of("aeiou");
if (pos != std::string::npos) {
std::cout << "First vowel found at position: " << pos << std::endl;
}
Here, the C-style string is converted to a `std::string` object so that `find_first_of` can be applied. This conversion is important as `find_first_of` is a member of the `std::string` class.
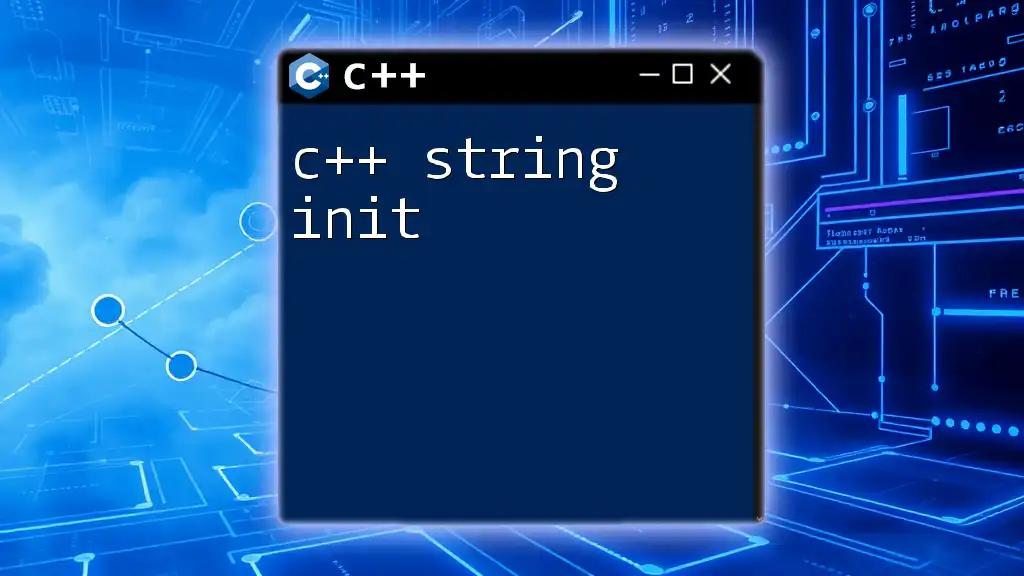
Common Pitfalls and Best Practices
When to Use `find_first_of`
`find_first_of` is particularly useful when trying to determine the first occurrence of a character (or characters) from a specified set in complex strings. For instance, it is beneficial in text processing tasks such as parsing user input, searching in files, or data validation scenarios.
Potential Issues to Watch Out For
While `find_first_of` is powerful, certain edge cases need to be considered. For example:
- Searching in an empty string will always result in `std::string::npos`.
- If you provide an empty character set, the function will also return `std::string::npos`.
Here’s a code snippet that highlights such pitfalls:
std::string test_string = "";
size_t pos = test_string.find_first_of("abc");
if (pos == std::string::npos) {
std::cout << "Empty string: No match found." << std::endl;
}
Performance Optimization
In terms of performance, `find_first_of` is generally faster than using multiple calls to `find()` because it scans directly for the first match among multiple characters in one pass. To maintain efficiency, especially in large strings, avoid unnecessary repeated calls.

Conclusion
In summary, the C++ string function `find_first_of` is a powerful tool for performing character-based searches within strings. Its ability to quickly locate the first instance of characters from a specified set makes it invaluable for string manipulation tasks. To become proficient, it's essential to practice with various examples, thereby solidifying your understanding of this function’s utility and intricacies.
Engaging with practical use cases will help cement your grasp on this function, preparing you for more advanced string-handling techniques in C++.