The `find` function in C++ is used to locate the position of a substring within a string, returning the index of its first occurrence or `string::npos` if the substring is not found.
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
size_t position = str.find("world");
if (position != std::string::npos) {
std::cout << "'world' found at index: " << position << std::endl;
} else {
std::cout << "'world' not found." << std::endl;
}
return 0;
}
Understanding C++ Strings
What is a C++ String?
In C++, a string is a sequence of characters that is represented using the `std::string` class. Unlike C-style strings, which are simply arrays of characters terminated by a null character, `std::string` provides a more flexible and user-friendly way to work with text. Strings in C++ can expand and contract in size, making them easier to manipulate without worrying about memory allocation issues.
Why Use `std::string`?
The advantages of using `std::string` over C-style strings include:
- Automatic Memory Management: `std::string` handles memory allocations internally, thus minimizing the risk of memory leaks and buffer overflows.
- Safety Features: It provides built-in functions for common operations, allowing for safer string manipulation.
- Convenience: `std::string` includes member functions for common tasks such as concatenation, comparison, and searching, streamlining programming tasks.
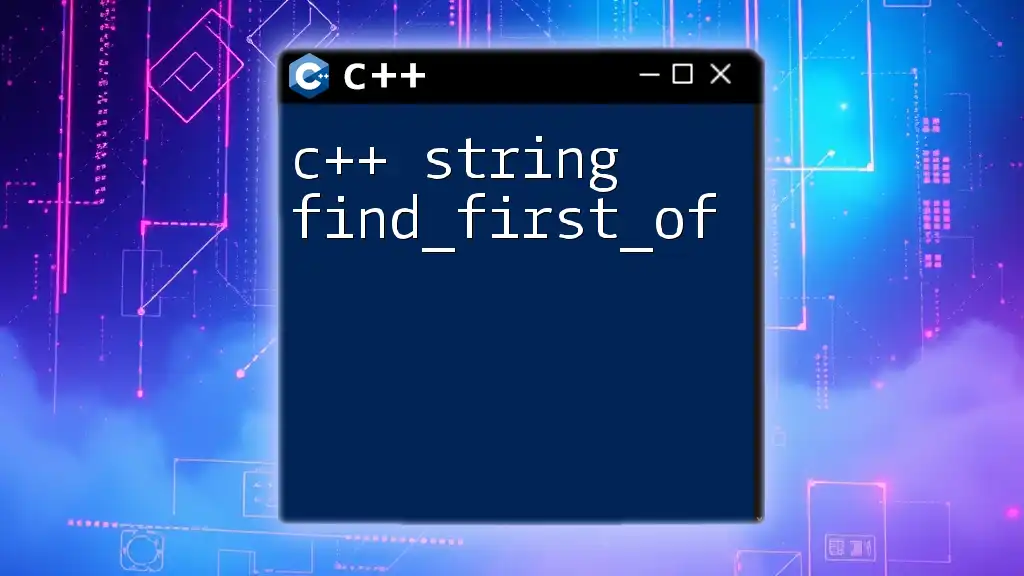
Exploring the `find` Method
What is the `find` Method?
The `find` method is a member function of the `std::string` class, used to locate a substring or a character within a string. Its primary purpose is to search for specified characters or sequences of characters and return their position within the string.
Syntax of the `find` Method
The general syntax for the `find` method is as follows:
size_t find(const string& str, size_t pos = 0) const noexcept;
In this syntax:
- `str`: This parameter specifies the substring that you want to find.
- `pos`: This optional parameter indicates the starting index for the search. By default, it is set to `0`, which means the search starts from the beginning of the string.
Return Value
The return value of the `find` method is the index position of the first occurrence of the substring. If the substring is not found, it returns `std::string::npos`, which is a constant value representing an invalid position.
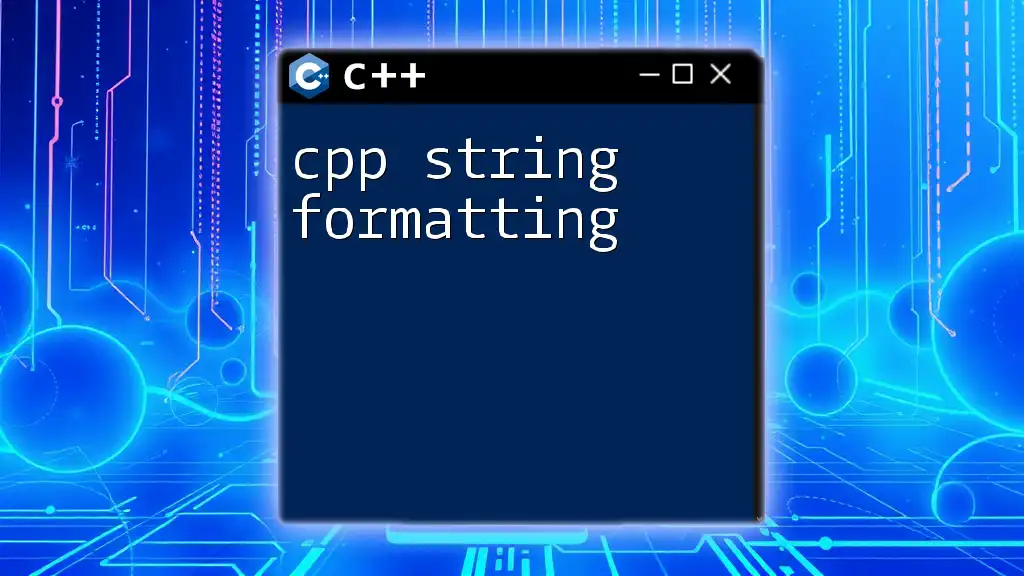
How to Use the `find` Method
Basic Example of `find`
To illustrate the use of the `find` method, consider the following simple example:
#include <iostream>
#include <string>
int main() {
std::string text = "Hello, World!";
size_t position = text.find("World");
if (position != std::string::npos) {
std::cout << "'World' found at position: " << position << std::endl;
}
return 0;
}
In this snippet, the program searches for the substring "World" in the string "Hello, World!" and outputs its position if it is found.
Using Different Start Positions
Searching from Specific Index
The `pos` parameter allows you to specify where to start searching in the string. For instance:
#include <iostream>
#include <string>
int main() {
std::string text = "Hello, World! Hello again!";
size_t position = text.find("Hello", 5);
if (position != std::string::npos) {
std::cout << "'Hello' found at position: " << position << std::endl;
}
return 0;
}
In this example, the search for "Hello" begins at index 5, which will return the position of the second occurrence of "Hello".
Finding Characters
Searching for Individual Characters
You can also locate individual characters in a string using the `find` method:
#include <iostream>
#include <string>
int main() {
std::string text = "Hello, World!";
size_t position = text.find('o');
if (position != std::string::npos) {
std::cout << "'o' found at position: " << position << std::endl;
}
return 0;
}
In this case, the program looks for the character 'o'. It recognizes the first occurrence and returns its index.
Case Sensitivity
The `find` method is case-sensitive, meaning that "hello" and "Hello" would be treated as different strings. Here's an example:
#include <iostream>
#include <string>
int main() {
std::string text = "Hello, World!";
size_t position = text.find("hello");
if (position == std::string::npos) {
std::cout << "'hello' not found (case sensitive)." << std::endl;
}
return 0;
}
In this example, the search for "hello" fails because the case does not match, showcasing the method's case-sensitivity.
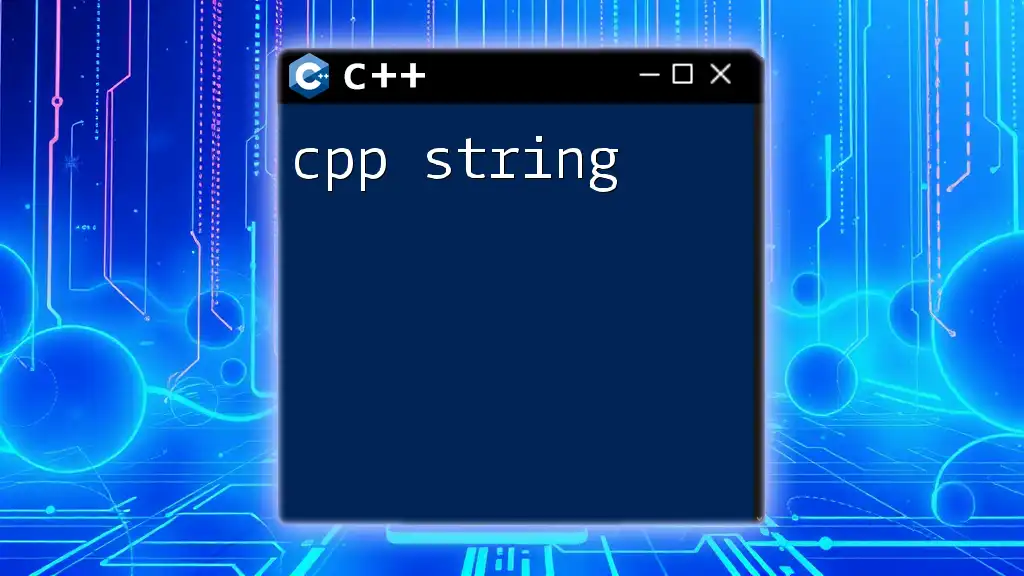
Advanced Use Cases of `find`
Finding Substrings Not Present
Handling Not Found Cases
The `find` method can return `std::string::npos`, indicating that a substring was not found. This is important to handle gracefully in your program:
#include <iostream>
#include <string>
int main() {
std::string text = "Hello, World!";
size_t notFound = text.find("C++");
if (notFound == std::string::npos) {
std::cout << "Substring not found." << std::endl;
}
return 0;
}
In this example, the attempt to find "C++" results in a message indicating that the substring was not found.
Using `find` in Loops
Searching for Multiple Occurrences
You can leverage the `find` method within a loop to locate all occurrences of a substring:
#include <iostream>
#include <string>
int main() {
std::string text = "repetition is key, repetition leads to mastery";
size_t position = 0;
while ((position = text.find("repetition", position)) != std::string::npos) {
std::cout << "Found at: " << position << std::endl;
position++; // Move past the current found substring
}
return 0;
}
This code snippet searches and prints the positions of all occurrences of "repetition" in the given text.
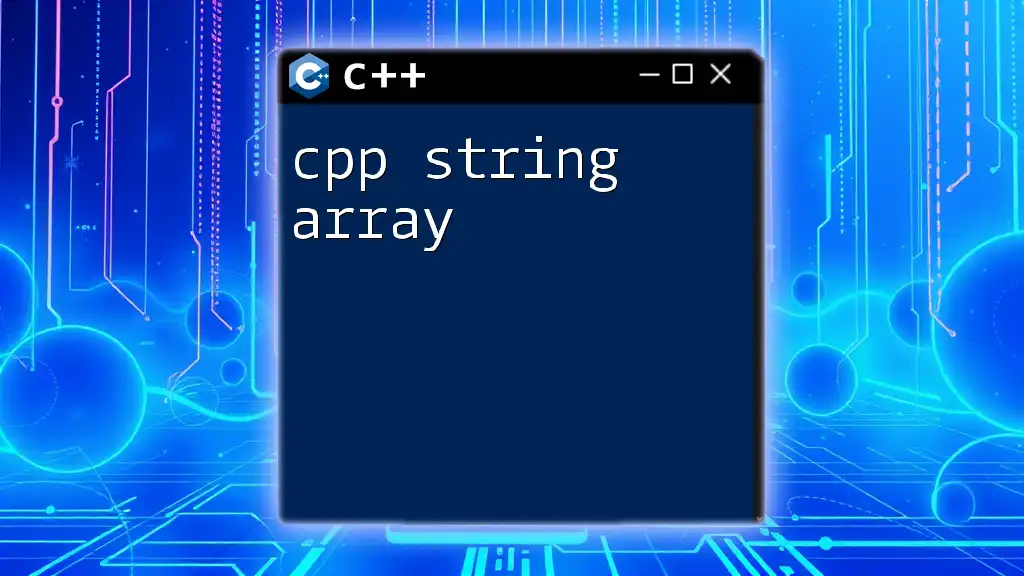
Performance Considerations
Time Complexity of `find`
The average time complexity for the `find` method is O(n), where n is the length of the string. The worst-case performance may approach this if repetitively checked for overlapping characters or strings.
Alternatives to `find`
In addition to `find`, other useful methods include:
- `rfind`: Finds the last occurrence of a substring.
- `find_first_of`: Finds the first occurrence of any character from a specified set.
Depending on your requirements, you may find it more efficient to use these alternatives instead of repeatedly using `find`.
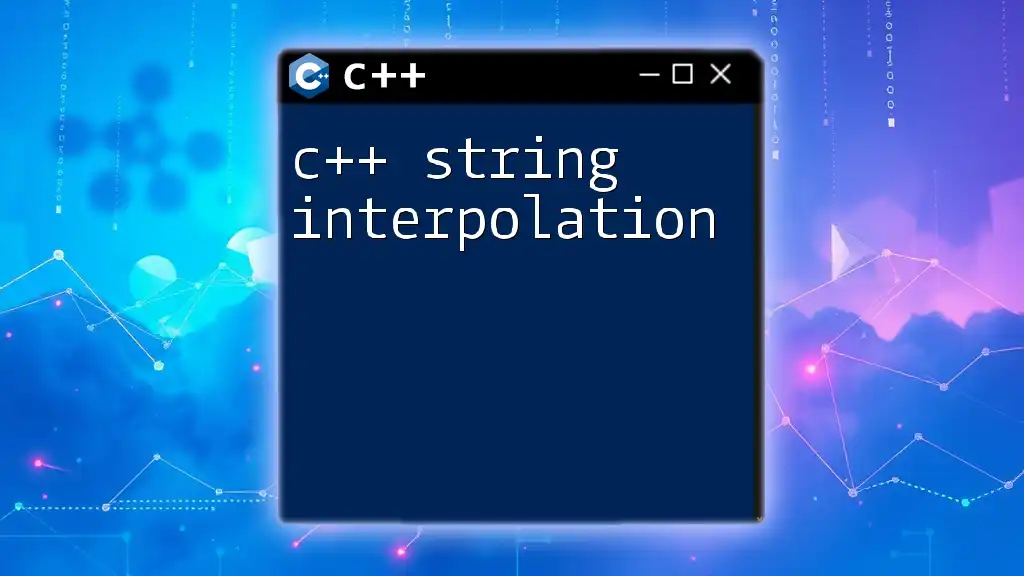
Common Pitfalls
Misunderstanding Return Values
One common mistake is failing to check for the return value of `find`. Always ensure that you compare against `std::string::npos` when determining if a substring was found.
Forgetting to Handle String Length
Before searching within a string, it’s crucial to consider its length. Relying on hard-coded positions can lead to runtime errors if the position exceeds the string's bounds.
#include <iostream>
#include <string>
int main() {
std::string text = "Hello!";
// This will lead to unexpected behavior since it's out of bounds.
size_t position = text.find("!", 10);
if (position == std::string::npos) {
std::cout << "Substring not found due to out-of-bound index." << std::endl;
}
return 0;
}
In this scenario, starting the search at an index that exceeds the string length may lead to confusion.
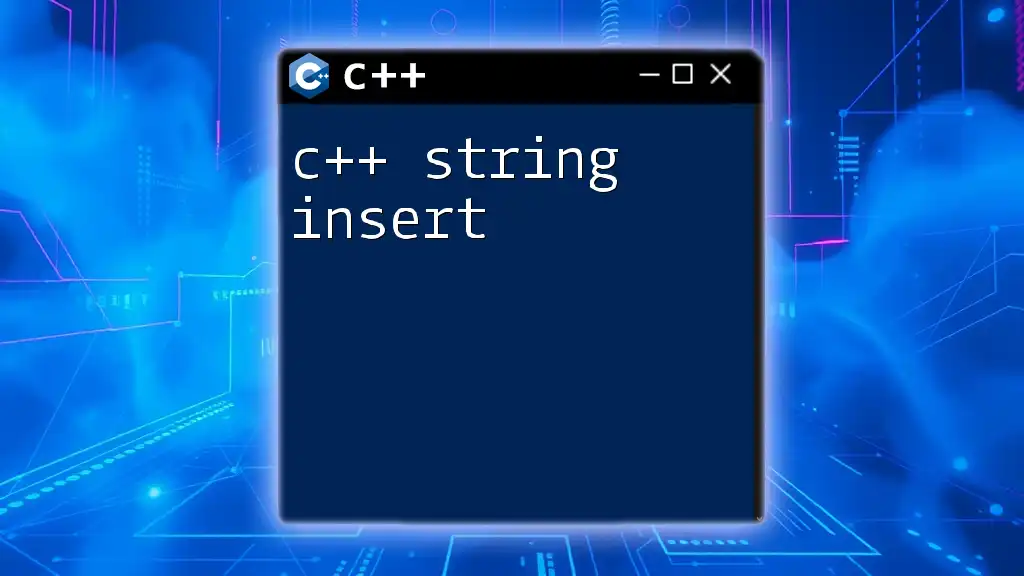
Conclusion
The `find` method in C++ is a powerful tool for locating substrings and characters within strings. Mastering its use not only enhances your ability to manipulate strings but also improves the overall robustness of your code. By practicing various examples and understanding both its strengths and limitations, you can effectively utilize `find` in your C++ programs.
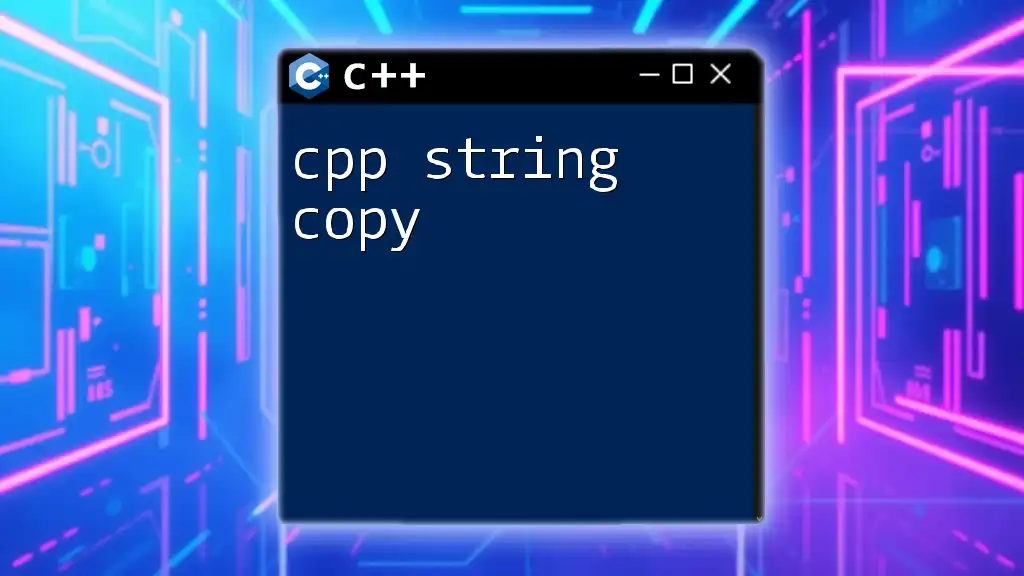
Further Reading and Resources
For more in-depth insights and examples regarding C++ strings and the `find` method, consider exploring the official C++ documentation, online tutorials, and community forums focused on C++ programming.