In C++, you can check if a substring exists within a string using the `find` method, which returns the position of the substring or `std::string::npos` if it is not found.
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
std::string toFind = "world";
if (str.find(toFind) != std::string::npos) {
std::cout << "Substring found!" << std::endl;
} else {
std::cout << "Substring not found." << std::endl;
}
return 0;
}
Understanding Strings in C++
What is a String in C++?
In C++, a string is a sequence of characters used to represent textual data. Strings can be handled using arrays of characters, known as C-style strings, or using the C++ Standard Library's `std::string` class, which simplifies string handling and provides a robust set of features.
C-style strings are essentially character arrays terminated by a null character (`'\0'`). They require manual memory management and string manipulation, leading to a higher chance of errors.
In contrast, C++ strings are managed automatically, providing dynamic resizing capabilities and a plethora of useful member functions, enhancing both code safety and productivity.
The C++ String Class
The `std::string` class is a powerful tool for string manipulation in C++. It abstracts away the complexities of manual memory management and provides numerous methods to modify and explore strings. Commonly used methods include:
- `length()`: Returns the length of the string.
- `empty()`: Checks if the string is empty.
- `append()`: Adds characters to the end of the string.
- `insert()`: Inserts characters at a specific position.
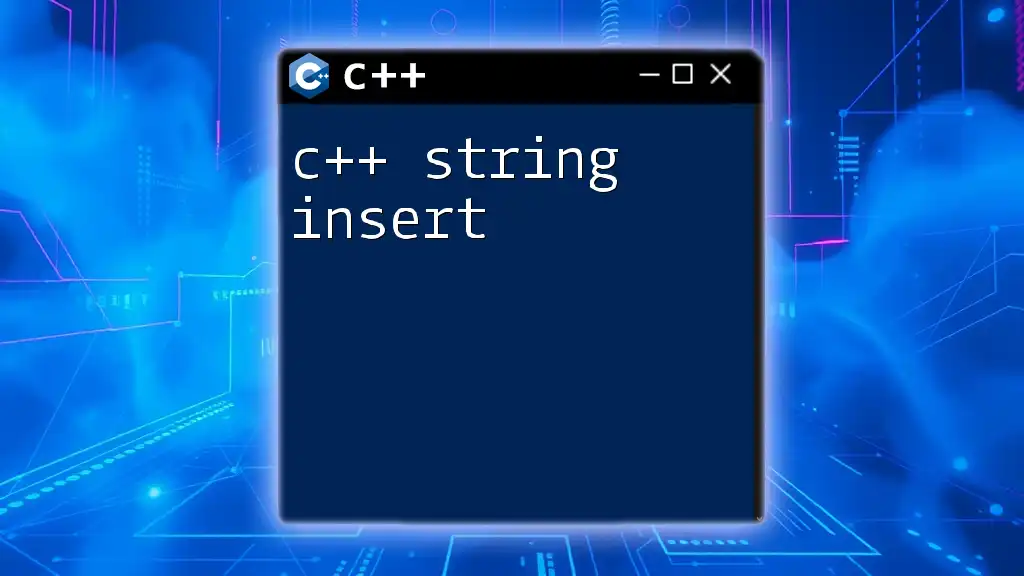
String Search Concepts
The Importance of Searching Within Strings
Searching for substrings is a fundamental operation in many applications, including text processing, data validation, and programming on user interfaces. For example, you may find a substring within a string when validating user input, parsing data files, or searching through documents.
Key Terms in String Searches
When we talk about "contains" in the context of strings, we are typically referring to the ability to determine if a certain sequence of characters (a substring) exists within another sequence (the main string). This concept is essential for implementing functionalities such as search filters, text analyzers, and other string handling applications.
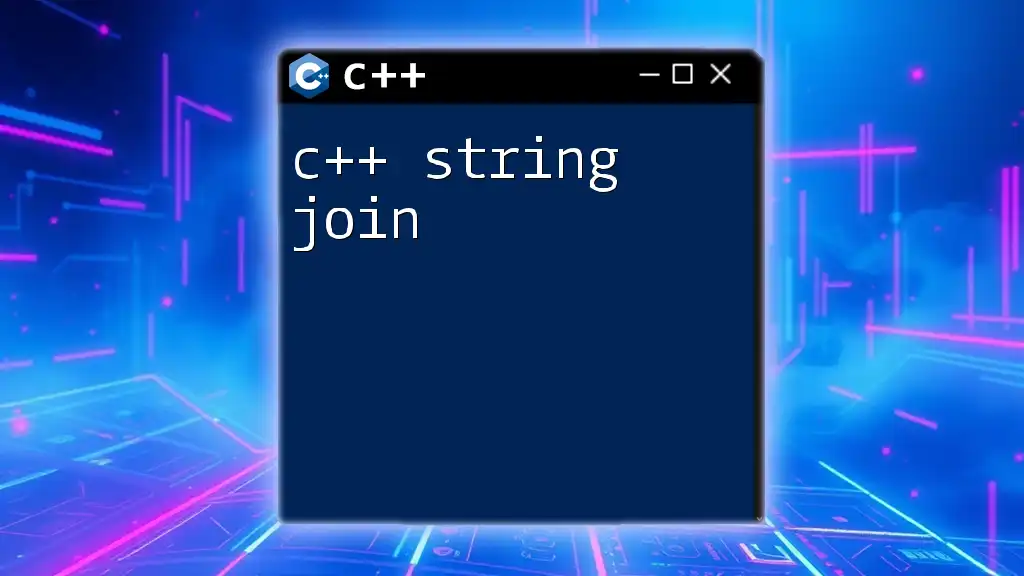
How to Check if a String Contains a Substring
Using the `.find()` Method
One of the most straightforward ways to check if a string contains a substring in C++ is by using the `.find()` method. This method returns the position of the first occurrence of the specified substring. If the substring is not found, it returns `std::string::npos`.
Syntax:
size_t find(const string& str, size_t pos = 0) const noexcept;
Example:
#include <iostream>
#include <string>
int main() {
std::string text = "Hello, World!";
std::string searchTerm = "World";
if (text.find(searchTerm) != std::string::npos) {
std::cout << "The text contains the substring!" << std::endl;
} else {
std::cout << "Substring not found." << std::endl;
}
return 0;
}
In this example, the program searches for "World" within "Hello, World!" and successfully finds it, printing the corresponding message.
Using the `.rfind()` Method
The `.rfind()` method operates similarly to `.find()`, but it searches for the last occurrence of a substring within a string. This is particularly useful when dealing with repeated substrings.
Syntax:
size_t rfind(const string& str, size_t pos = npos) const noexcept;
Example:
#include <iostream>
#include <string>
int main() {
std::string text = "banana";
std::string searchTerm = "an";
size_t position = text.rfind(searchTerm);
if (position != std::string::npos) {
std::cout << "Found 'an' at index: " << position << std::endl;
} else {
std::cout << "Substring not found." << std::endl;
}
return 0;
}
Here, the program checks for the substring "an" and finds its last occurrence at the specified index.
Using the `.find_first_of()` and `.find_last_of()` Methods
These methods help locate the first or last occurrence of any character from a set within the string. They can be particularly effective when searching for multiple characters.
Syntax:
size_t find_first_of(const string& str, size_t pos = 0) const noexcept;
size_t find_last_of(const string& str, size_t pos = npos) const noexcept;
Example:
#include <iostream>
#include <string>
int main() {
std::string text = "Hello, World!";
std::string searchSet = "aeiou";
size_t index = text.find_first_of(searchSet);
if (index != std::string::npos) {
std::cout << "First vowel found at index: " << index << std::endl;
}
return 0;
}
In this instance, the program identifies the first vowel in the string, illustrating how to effectively search for multiple characters.
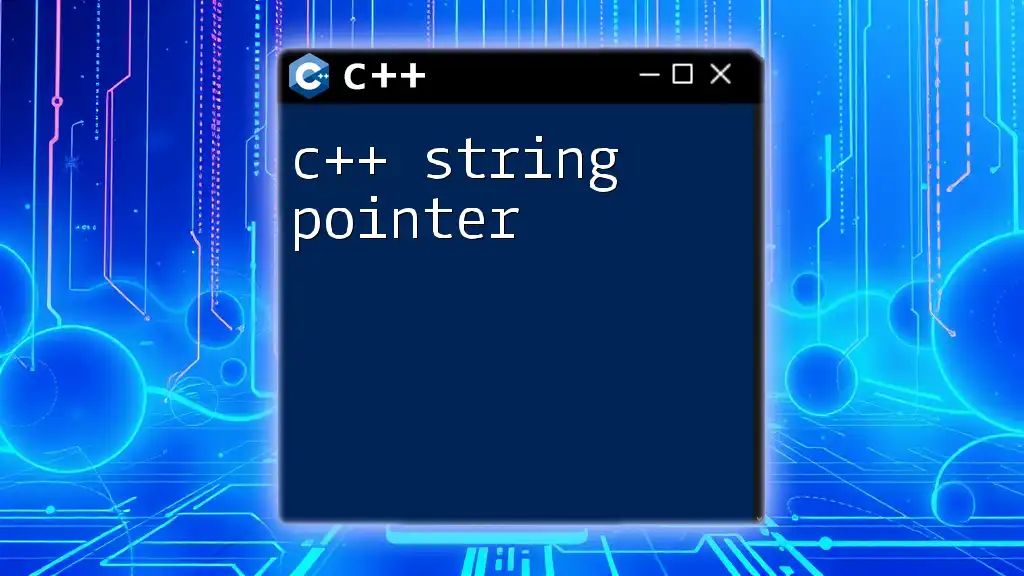
Modern C++ Approaches
Utilizing C++20 features
With the introduction of C++20, a new and more intuitive method for checking substring containment is available—namely, the `.contains()` method. This method simplifies the checking process, making it more readable and straightforward.
Syntax:
bool contains(const string& str) const noexcept;
Example:
#include <iostream>
#include <string>
int main() {
std::string text = "Hello, World!";
std::string searchTerm = "World";
if (text.contains(searchTerm)) {
std::cout << "The text contains the substring!" << std::endl;
} else {
std::cout << "Substring not found." << std::endl;
}
return 0;
}
In this example, the `contains()` method is used to determine if "World" exists in "Hello, World!", significantly enhancing code readability.
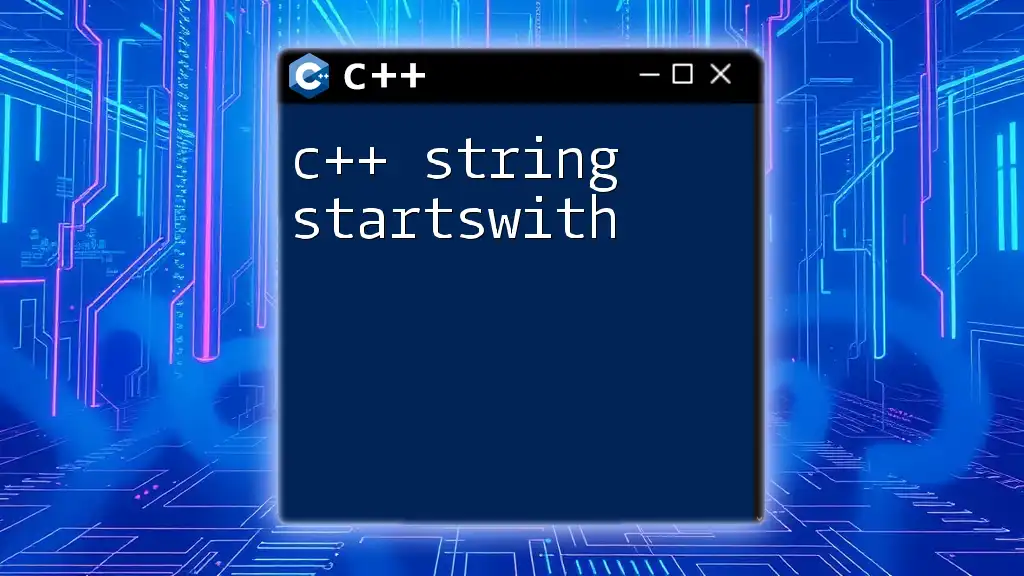
Best Practices for String Searching
Efficient String Searching
When dealing with large strings or frequent search operations, it is critical to consider performance. Algorithms like the Knuth-Morris-Pratt or Rabin-Karp can optimize searching. For scenarios where you need to check membership across multiple queries, consider using hash sets or trie structures, which can yield faster results.
Common Pitfalls to Avoid
While string searching may seem straightforward, several common pitfalls are worth noting:
- Case Sensitivity: The methods discussed are case-sensitive by default. To perform case-insensitive searches, consider converting both strings to the same case before performing the check.
- Substring Overlaps: Be aware that your algorithms may yield unexpected results if a search term overlaps with itself. Carefully handle such cases to avoid logical errors.
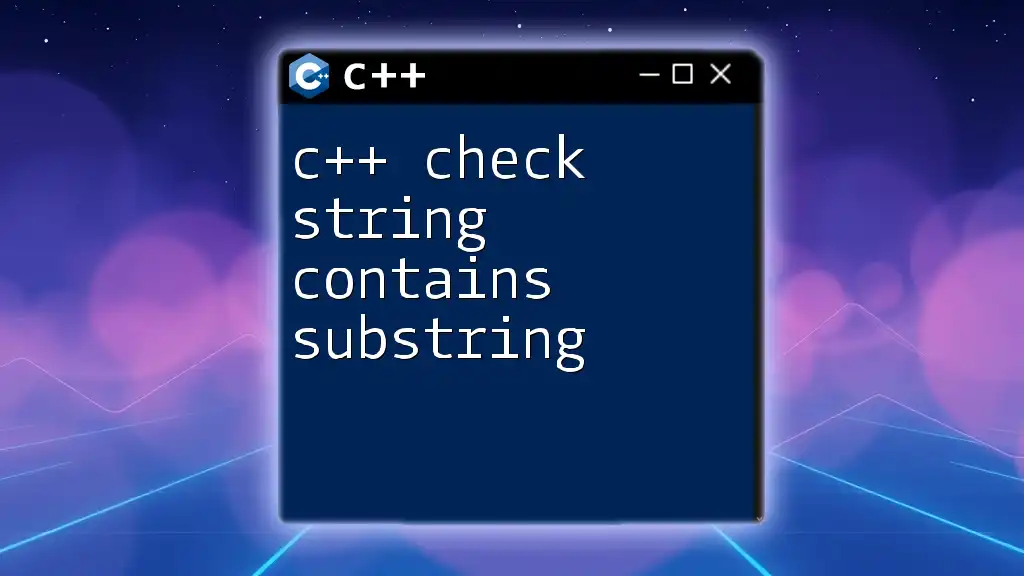
Conclusion
Summary of Key Takeaways
In this guide, we've explored various techniques for determining whether a C++ string contains a specific substring. Important methods like `.find()`, `.rfind()`, `.find_first_of()`, and the advanced `.contains()` method in C++20 offer flexible searching capabilities that cater to diverse needs.
Further Learning Resources
For those who wish to delve deeper into string manipulation and searching techniques in C++, consider exploring comprehensive books on C++ programming, online courses, and official documentation that provide deeper insights into best practices and advanced features of the C++ Standard Library.