In C++, you can check if a string contains a substring using the `find` method, which returns the position of the substring if found or `std::string::npos` if not found.
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
std::string substr = "world";
if (str.find(substr) != std::string::npos) {
std::cout << "Substring found!" << std::endl;
} else {
std::cout << "Substring not found." << std::endl;
}
return 0;
}
Understanding Strings and Substrings
What is a String?
In C++, a string is a sequence of characters that represents text. You can use either `std::string` or C-style strings (character arrays) to manage strings. The `std::string`, part of the C++ Standard Library, provides a more intuitive way to manipulate texts due to its ability to resize dynamically and come with numerous built-in methods.
What is a Substring?
A substring is essentially a smaller sequence derived from a larger string, contained within that original string. For example, in the string "Programming", "Prog" and "ming" are both valid substrings. Understanding how to identify substrings within a string is crucial for various programming scenarios, such as searching, parsing, and data validation.
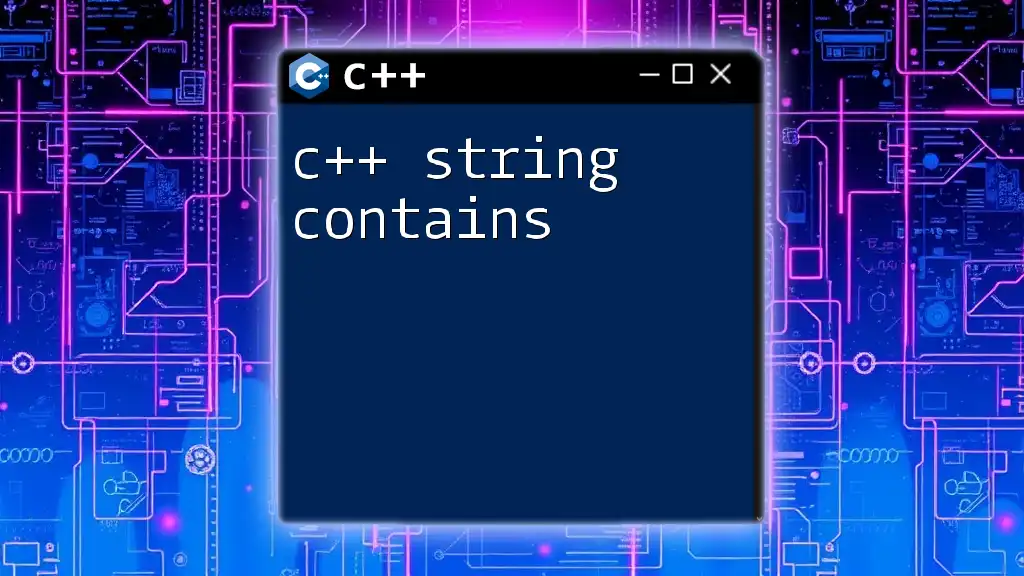
Methods to Check if a String Contains a Substring
Using std::string::find()
Explanation of the find() Method
The `find()` method is a robust function offered by the `std::string` class, which allows you to search for a specified substring. The method's syntax is straightforward, and it can return various values:
- The index of the first occurrence of the substring.
- std::string::npos if the substring is not found.
std::string str = "Hello, world!";
size_t found = str.find("world");
if (found != std::string::npos) {
// Substring found
}
Example Code Snippet
Consider the following example where we check if a specific substring is present in a string. The program will print a message based on the search result.
#include <iostream>
#include <string>
int main() {
std::string str = "Learning C++ is fun!";
std::string substr = "C++";
if (str.find(substr) != std::string::npos) {
std::cout << "Substring found!" << std::endl;
} else {
std::cout << "Substring not found!" << std::endl;
}
return 0;
}
Using std::string::find_first_of()
Explanation of find_first_of() Method
The `find_first_of()` method differs from `find()` in that it searches for any of the characters within a set of specified characters. This can be particularly useful when you're looking for the position of the first occurrence of any character from a list.
size_t found = str.find_first_of("aeiou");
Example Code Snippet
Here’s a code snippet that demonstrates how to use `find_first_of()` to locate the position of the first vowel in a string.
#include <iostream>
#include <string>
int main() {
std::string str = "C++ programming rules!";
size_t pos = str.find_first_of("aeiou");
if (pos != std::string::npos) {
std::cout << "First vowel found at position: " << pos << std::endl;
}
return 0;
}
Using std::string::find_last_of()
Explanation of find_last_of() Method
Contrastively, `find_last_of()` searches for the last occurrence of any character from a specified set. This is handy in many applications, such as parsing or processing strings from the end.
size_t pos = str.find_last_of("!.");
Example Code Snippet
Here’s an example that demonstrates the use of `find_last_of()` to find the last punctuation mark in a string.
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World. Welcome to C++!";
size_t pos = str.find_last_of("!.?");
if (pos != std::string::npos) {
std::cout << "Last punctuation found at position: " << pos << std::endl;
}
return 0;
}
Using Regular Expressions
Overview of C++ Regular Expressions
If your string searching requirements are more complex, consider using regular expressions available in the `<regex>` library of C++. Regular expressions allow for flexible pattern matching, which can accommodate complex substring criteria that simple methods cannot effectively handle.
#include <regex>
Example Code Snippet
In the following example, we utilize a regex pattern to check if a given substring matches within a main string.
#include <iostream>
#include <regex>
#include <string>
int main() {
std::string str = "Regular expressions make substring searching easy!";
std::regex expression("easy");
if (std::regex_search(str, expression)) {
std::cout << "Substring matched using regex!" << std::endl;
}
return 0;
}
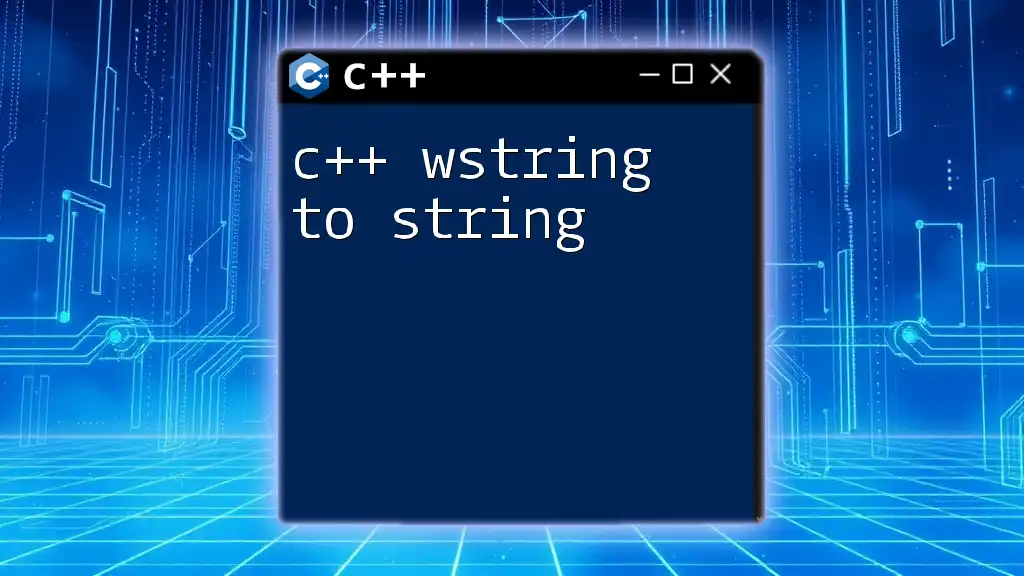
Performance Considerations
Time Complexity of Each Method
Each method comes with its own time complexity:
- `find()`: Average-case time complexity is O(n), where n is the length of the string.
- `find_first_of()`: Similar to `find()`, with a complexity based on the search character set.
- `find_last_of()`: Also O(n) due to searching from the end.
- Regular expressions: These can be more complex, often resulting in higher overhead relative to simpler methods, particularly for larger strings and patterns.
Choosing the Right Method
When deciding which method to use, consider:
- Readability: Simpler methods like `find()` are usually easier to read and maintain.
- Performance: For large datasets or frequent searches, optimal performance becomes critical. Hence, prefer the most straightforward method that meets your criteria.
- Complexity of Search: If matching patterns or handling various scenarios is necessary, regular expressions are indispensable, despite their slight overhead.
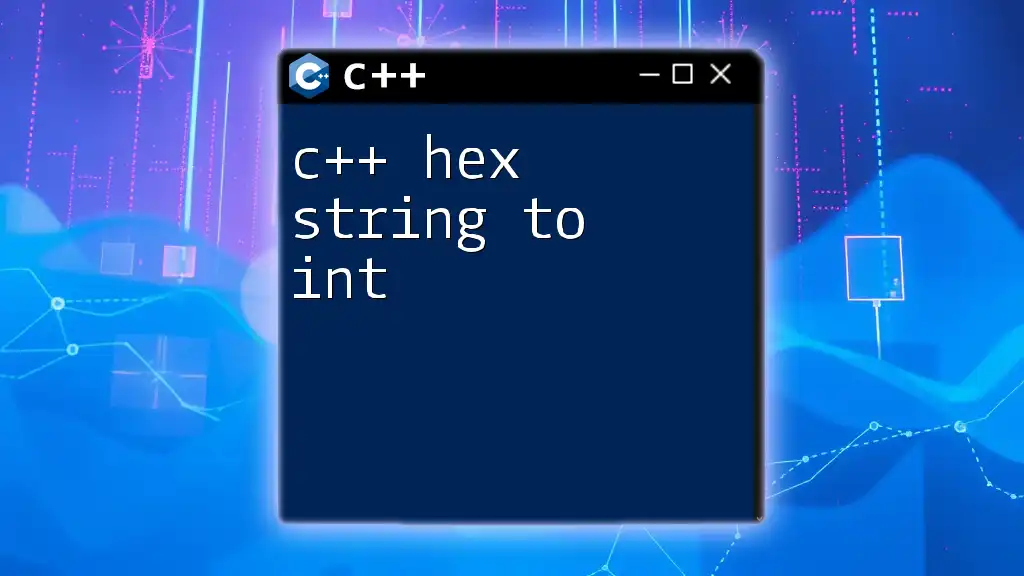
Common Errors and Troubleshooting
Common Mistakes in Substring Search
One prevalent mistake during substring searching is misunderstanding return values. Always remember that if a substring is not found, methods return `std::string::npos`, not -1, which can lead to incorrect assumptions and errors in further logic.
Debugging Tips
When debugging substring search issues, it’s crucial to validate your input strings. Ensure that the strings contain the expected characters and that the search criteria are correctly specified. Print intermediate values to keep track of your function's logic flow.
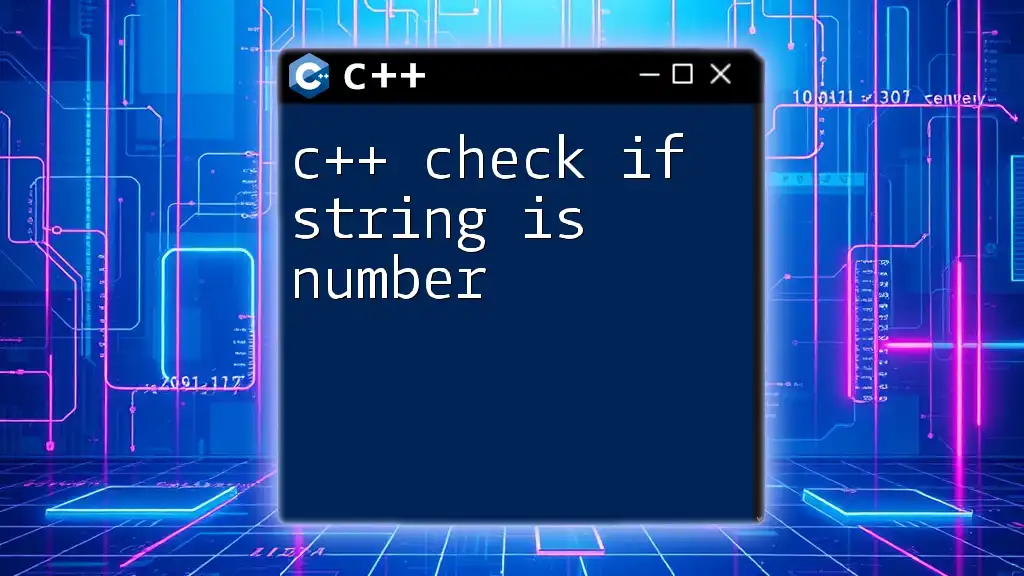
Real-World Applications
Use Cases for Substring Searches
Substring searches play vital roles in various applications, such as:
- Data parsing: Reading and interpreting structured data formats.
- User input validation: Ensuring entered data meets specific formats.
- Log analysis: Searching for specific entries or patterns within logs.
Conclusion
In C++, the ability to effectively check if a string contains a substring is a fundamental skill that can significantly enhance your programming capabilities. By understanding and utilizing various methods such as `find()`, `find_first_of()`, `find_last_of()`, and regular expressions, you can tailor your string manipulation strategies to suit your specific needs.
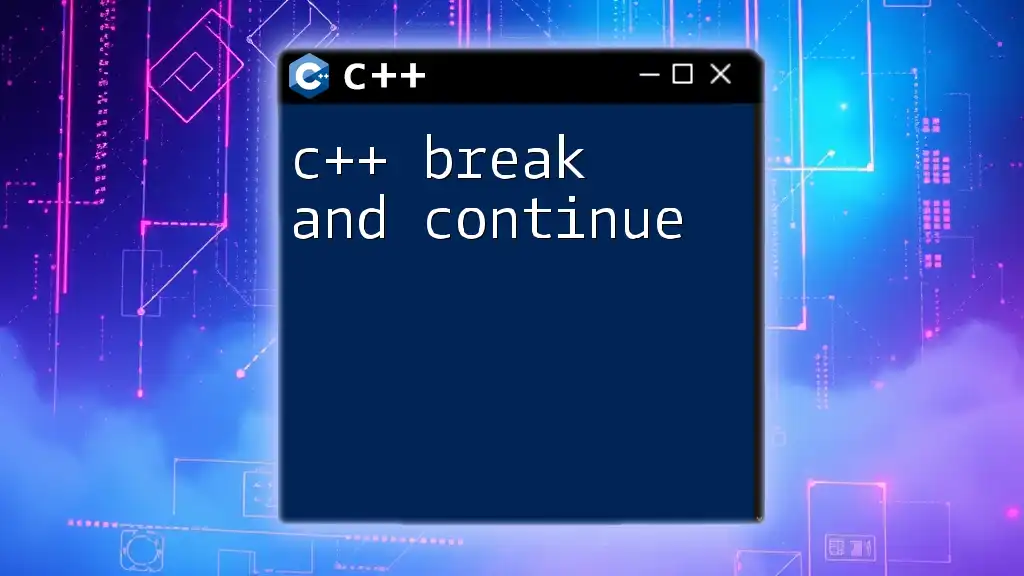
Additional Resources
Books and Online Courses
For further exploration, consider diving into recommended books on C++ programming or enrolling in online courses tailored around string manipulation and data handling.
Community and Support
Engaging with forums and communities, such as Stack Overflow or Reddit's r/cpp, can provide additional insights and help for any questions you may encounter during your journey with C++.