In C++, to perform a case-insensitive comparison of strings, you can convert both strings to the same case (either lower or upper) before comparing them, as shown in the following example:
#include <iostream>
#include <algorithm>
#include <cctype>
#include <string>
bool caseInsensitiveCompare(const std::string& str1, const std::string& str2) {
return std::equal(str1.begin(), str1.end(), str2.begin(), str2.end(),
[](char a, char b) { return tolower(a) == tolower(b); });
}
int main() {
std::string str1 = "Hello";
std::string str2 = "hello";
std::cout << std::boolalpha << caseInsensitiveCompare(str1, str2) << std::endl; // Outputs: true
return 0;
}
Importance of Case Insensitivity
User Input and Data Consistency
When developing applications that involve user interaction, it's crucial to consider how users input data. Many individuals can input the same string in various cases, such as "Hello", "HELLO", or "hello". If string comparisons are case-sensitive, these variations would be treated as different strings, leading to potential inconsistencies or confusion. As a developer, ensuring that your application recognizes all of these as equivalent strings enhances user experience and data integrity.
Applications in Search Functionality
Case insensitivity plays a vital role in search functionalities, especially in databases or search engines. Users may not always remember the correct casing for keywords or phrases. An effective case insensitive search enhances usability, allowing users to find the results they seek without being hindered by the case of the text they input.
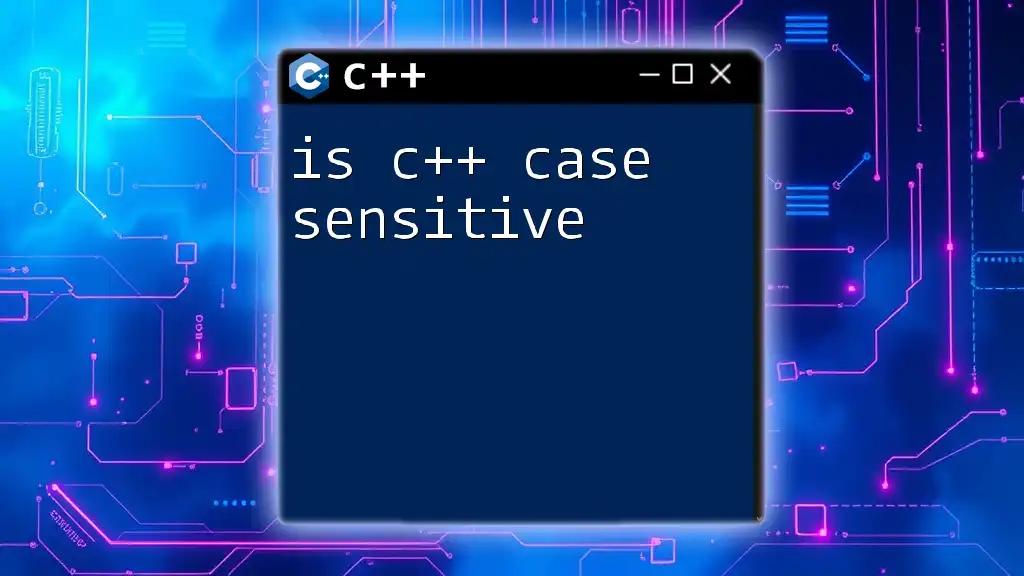
Overview of String Comparison in C++
Basic String Comparison
C++ provides a straightforward syntax for comparing strings using the `==` operator. This operator checks for equality between two strings' content, but it is case-sensitive by default.
Here is a simple example demonstrating basic string comparison:
#include <iostream>
#include <string>
int main() {
std::string str1 = "example";
std::string str2 = "example";
if (str1 == str2) {
std::cout << "Strings are equal." << std::endl;
}
return 0;
}
In this snippet, `str1` and `str2` are compared, and since they are identical, the output confirms that they are equal. However, if you changed `str2` to "Example", the output would indicate that these strings are not equal. This is where case insensitivity becomes essential.
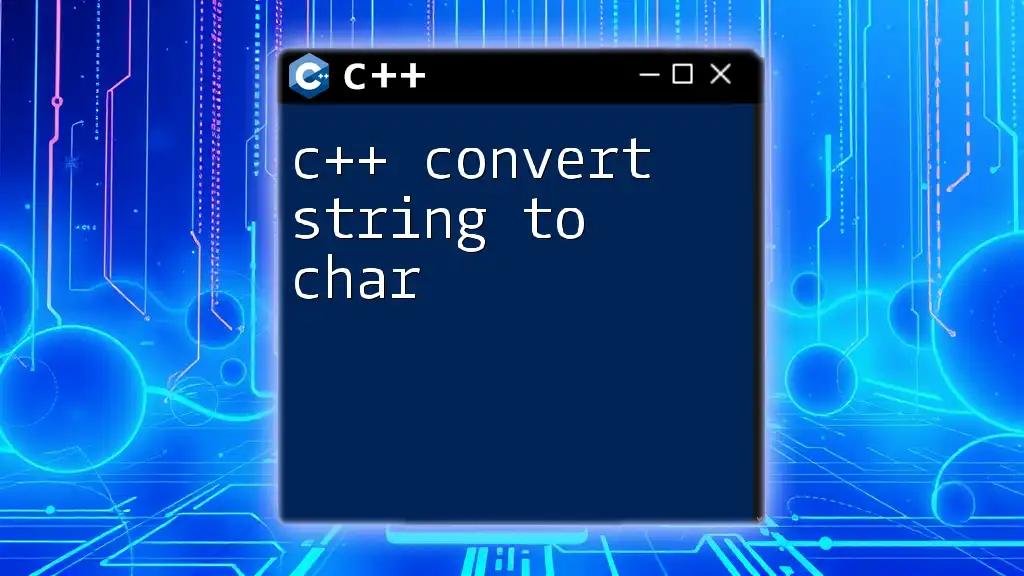
Implementing Case-Insensitive Comparison
Using Standard Library Functions
Transform Function from `<algorithm>`
One effective way to implement case insensitive comparison is to use the `std::transform` function from the `<algorithm>` library. This function can convert the entire string to a common case, typically lowercase.
#include <algorithm>
#include <string>
std::string toLower(const std::string& str) {
std::string lowerStr = str;
std::transform(lowerStr.begin(), lowerStr.end(), lowerStr.begin(), ::tolower);
return lowerStr;
}
This `toLower` function takes a string, transforms each character to lowercase, and returns the modified string. This sets the stage for a case insensitive comparison.
Example of Case-Insensitive Comparison
Using the `toLower` function, you can create a function that compares two strings regardless of their case:
#include <iostream>
#include <string>
#include <algorithm>
bool caseInsensitiveCompare(const std::string& str1, const std::string& str2) {
return toLower(str1) == toLower(str2);
}
int main() {
std::string str1 = "Hello";
std::string str2 = "hello";
if (caseInsensitiveCompare(str1, str2)) {
std::cout << "The strings are equal (case insensitive)." << std::endl;
} else {
std::cout << "The strings are not equal." << std::endl;
}
return 0;
}
In this code, regardless of the casing of `str1` and `str2`, they will be treated as equal because both are transformed to lowercase before comparison.
Custom Comparison Function
Implementing a More Efficient Approach
Transforming both strings might not be efficient, especially for larger strings. An alternative approach is to compare characters directly while considering their case. This method skips the overhead of creating additional strings:
bool caseInsensitiveCompareDirect(const std::string& str1, const std::string& str2) {
if (str1.length() != str2.length()) return false;
for (size_t i = 0; i < str1.length(); ++i) {
if (tolower(str1[i]) != tolower(str2[i])) return false;
}
return true;
}
This function starts by checking the lengths of the two strings. If they are not equal, it immediately returns false. It then iterates through each character, comparing them in a case insensitive manner.
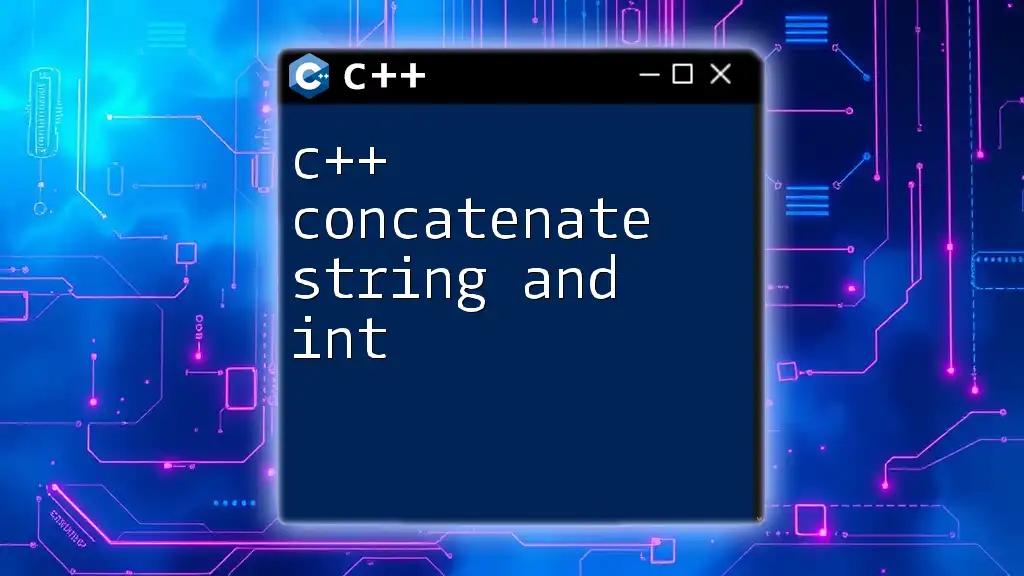
Performance Considerations
Time Complexity of String Comparison
When implementing string comparisons, especially for long strings or large datasets, it's vital to consider time complexity. Generally, string comparisons have a time complexity of O(n), where n is the length of the string. However, using the `toLower` method involves an additional pass through the string, which can add up in performance costs.
Optimizing Performance
To optimize string comparisons, you can:
- Implement early exit conditions, such as checking the lengths of strings first.
- Avoid unnecessary string transformations when possible. Utilizing a direct comparison based on character codes, as described earlier, reduces overhead drastically, making your application more efficient.
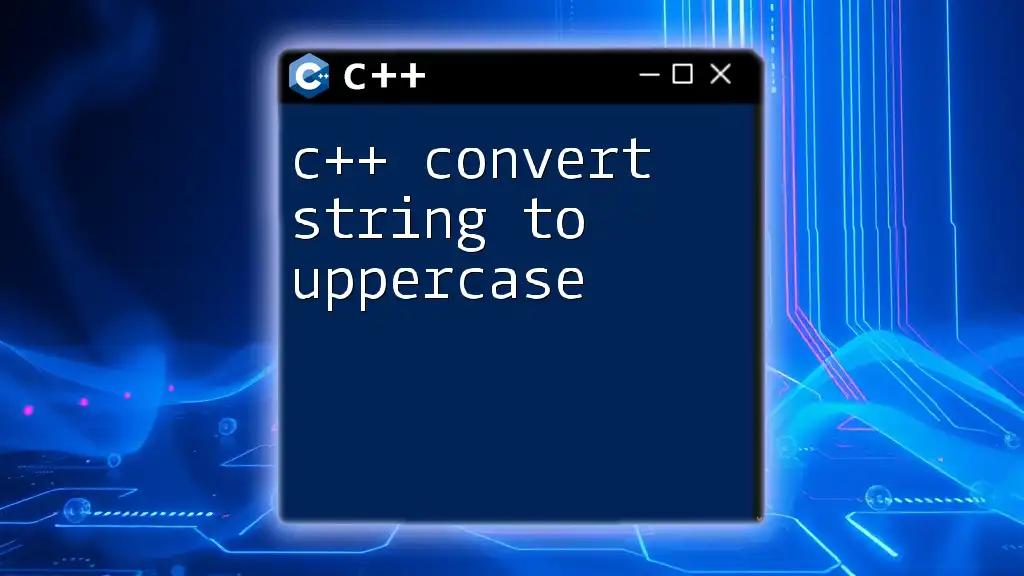
Common Use Cases
Input Validation
In user-driven applications, validating input against specific strings (like usernames or commands) is a common use case for case insensitive comparison. For example, in a login system, both "Admin" and "admin" should permit access to the same account.
Data Filtering in Applications
Another practical application is in filtering data. For instance, if you're allowing users to search for products in a database, make sure that searches ignore case to improve usability. If a user searches "laptop," they should see results for "Laptop," "LAPTOP," and "LapTop."
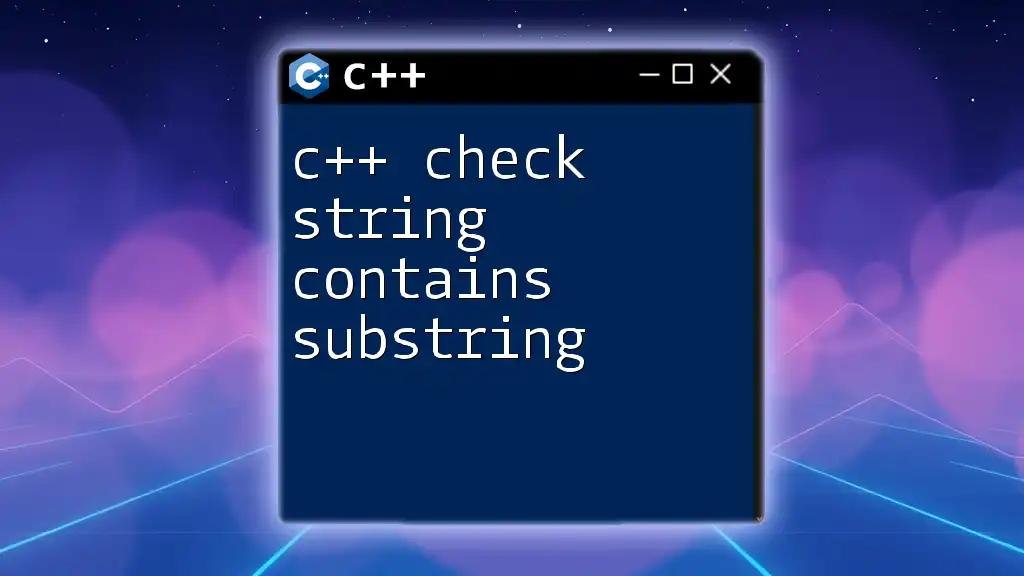
Conclusion
Recap of Key Points
Implementing a C++ case insensitive string compare is crucial for user experience and data integrity in applications. By employing functions like `std::transform` and custom comparison logic, developers can efficiently handle string comparisons that ignore case sensitivity.
Encouragement to Practice
I encourage you to implement these strategies in your own projects. Explore the different methods of comparison we've discussed, and see how they can enhance the functionality of your applications.

Additional Resources
Recommended Reading
To further your understanding of C++ string manipulation, check out resources such as:
- The C++ Standard Library documentation
- Books on C++ programming focusing on data structures and algorithms.
Code Repositories
Visit GitHub for practical examples related to string comparison, where you can find projects implementing case insensitive comparison strategies and learn from existing solutions.