In C++, you can convert a `std::string` to a character array (C-style string) using the `c_str()` method, which provides a pointer to a null-terminated array of characters. Here's an example:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
const char* charArray = str.c_str();
std::cout << charArray << std::endl;
return 0;
}
Understanding Strings and Characters in C++
What is a String in C++?
In C++, a string is typically represented using the `std::string` class, part of the Standard Library. This class provides a flexible and convenient way to work with sequences of characters, offering various functionalities such as concatenation, substring extraction, and length checking. For example:
#include <iostream>
#include <string>
int main() {
std::string myString = "Hello, World!";
std::cout << myString << std::endl;
return 0;
}
Here, `myString` is an instance of `std::string`, showcasing its ease of use.
What is a Char in C++?
A `char` in C++ represents a single character and is denoted using single quotes. Unlike strings, which can hold multiple characters, a `char` is a basic data type for representing individual characters. Here's an example:
#include <iostream>
int main() {
char myChar = 'A';
std::cout << myChar << std::endl;
return 0;
}
In the example above, `myChar` is a single character variable.
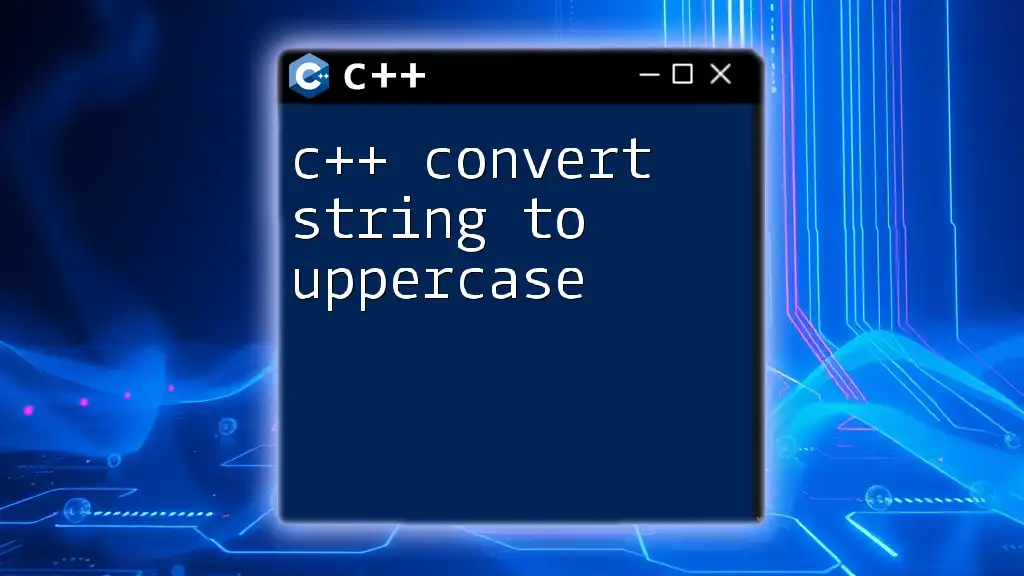
Why Convert String to Char?
Converting a string to a char is essential in various programming scenarios. Understanding when and why you need this conversion is crucial. Common use cases include:
- Input Handling: When dealing with APIs or functions that require char arrays (e.g., C-style strings).
- Compatibility: Working with legacy code where char arrays are prevalent or interfacing with lower-level libraries that expect character arrays.
Real-world examples highlight the relevance of this conversion, such as reading command-line arguments or processing user inputs.
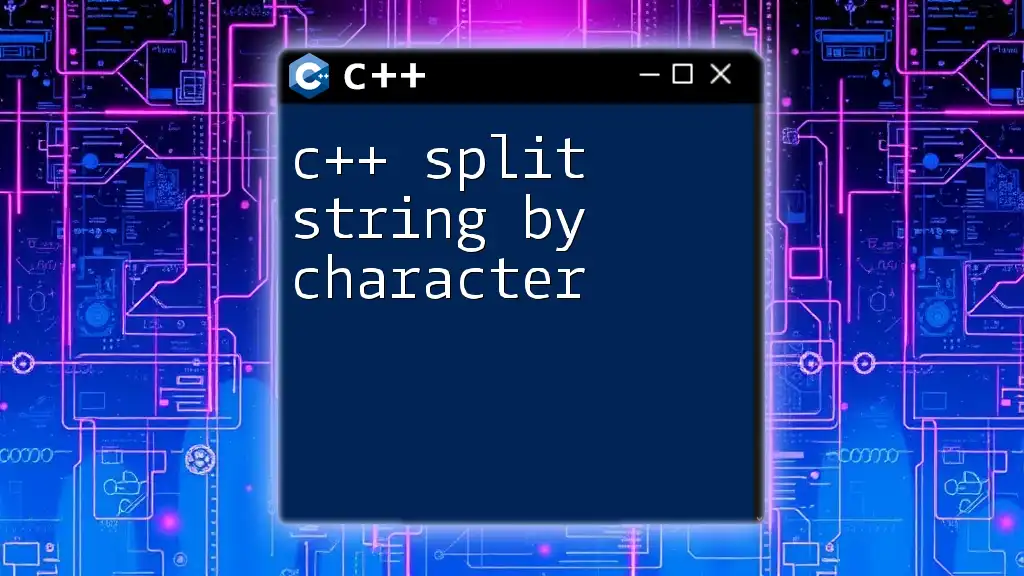
Methods to Convert String to Char in C++
Using `c_str()` Method
One of the most straightforward ways to convert a string to a char is by using the `c_str()` method. This method returns a pointer to a null-terminated character array representation of the string. Here's how to use it:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello";
const char* cstr = str.c_str();
std::cout << cstr << std::endl; // Outputs: Hello
return 0;
}
Manual Conversion Using Array Indexing
Another method involves manually copying each character from the string to a char array. While this uses more lines of code, it grants full control over the conversion process. Below is an example:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello";
char charArray[6]; // Allocate space for null terminator
for (size_t i = 0; i < str.length(); ++i) {
charArray[i] = str[i];
}
charArray[str.length()] = '\0'; // Null-terminate
std::cout << charArray << std::endl; // Outputs: Hello
return 0;
}
Using STL `std::copy`
Another efficient approach is to use `std::copy` from the Standard Template Library. This method simplifies the copying process and can help improve readability. Here’s an example:
#include <iostream>
#include <string>
#include <algorithm>
int main() {
std::string str = "Hello";
char charArray[6]; // Include space for null terminator
std::copy(str.begin(), str.end(), charArray);
charArray[str.size()] = '\0'; // Null-terminate
std::cout << charArray << std::endl; // Outputs: Hello
return 0;
}
Using `std::vector<char>` for Dynamic Conversion
For dynamic strings or cases where the size isn't predetermined, using `std::vector<char>` offers a robust solution. This approach provides built-in memory management and flexibility. Here's how to implement it:
#include <iostream>
#include <string>
#include <vector>
int main() {
std::string str = "Hello";
std::vector<char> charVector(str.begin(), str.end());
charVector.push_back('\0'); // Null-terminate
std::cout << charVector.data() << std::endl; // Outputs: Hello
return 0;
}
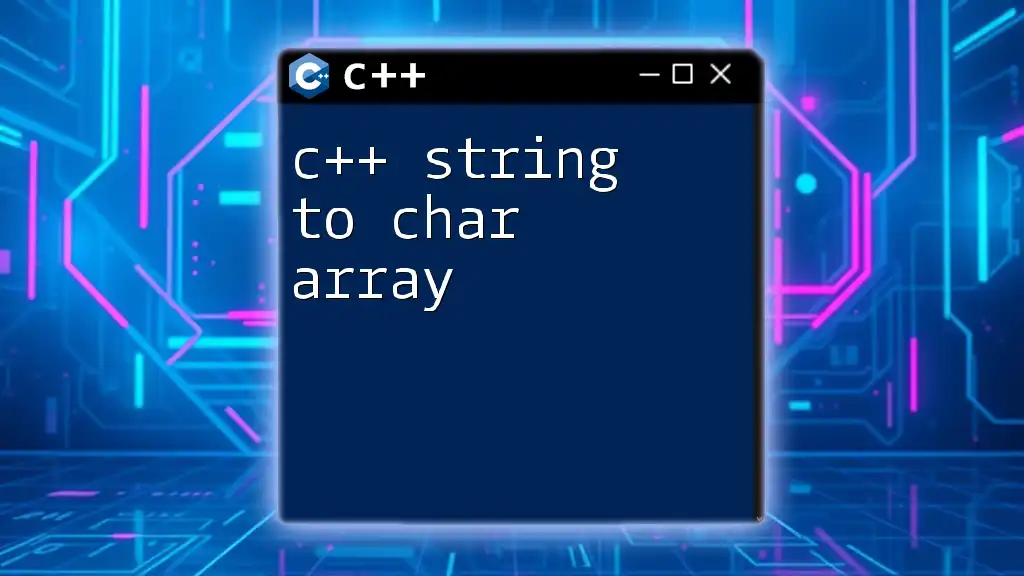
Common Pitfalls When Converting String to Char
Memory Management Issues
When converting a string to a char array, memory management is paramount. Failing to handle memory properly can lead to leaks or undefined behavior. For example, if you dynamically allocate memory but forget to deallocate it, you'll create a memory leak. Always ensure the allocated space is freed appropriately.
Misunderstanding Null Termination
Null termination is critical when working with char arrays. A missing null terminator can cause functions that expect C-style strings to read beyond allocated memory, leading to potential crashes or incorrect behavior. Always remember to include the null terminator explicitly.
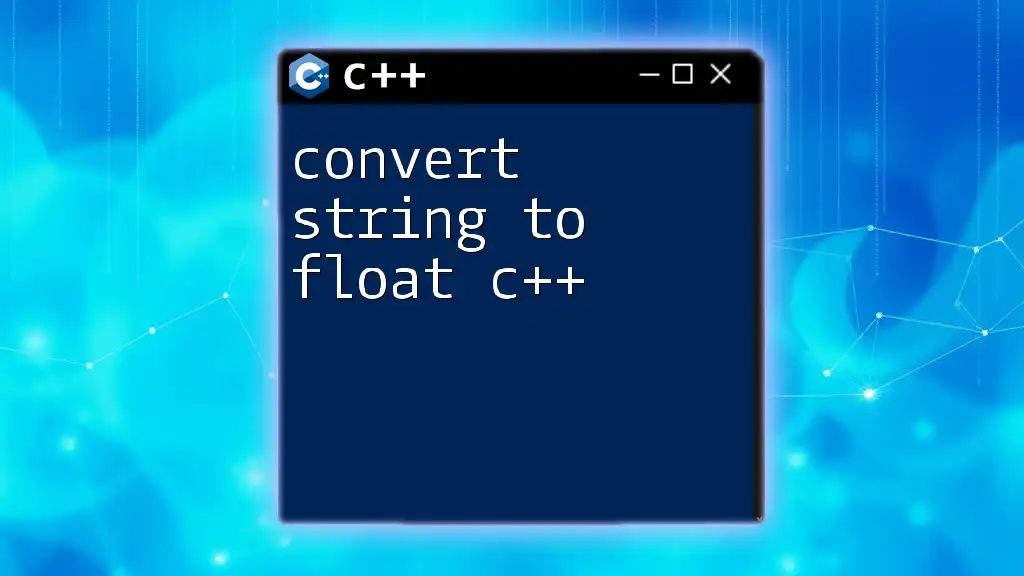
Performance Considerations
Comparing Different Methods
When it comes to performance, the method you choose can significantly impact your application's efficiency. Using `c_str()` may be faster for simple conversions, while manual copying or using `std::copy` gives you more control at the potential cost of efficiency. In performance-critical applications, always benchmark your chosen method to avoid unnecessary bottlenecks.
Best Practices for Efficient String to Char Conversion
To optimize the conversion process, consider the following best practices:
- Prefer `c_str()` when you need a read-only C-style string for interfacing with APIs.
- Use `std::vector<char>` if you require dynamic allocation or modification of the character array.
- Reduce memory allocations by reusing buffers or pre-allocating based on expected sizes.
- Utilize RAII patterns to manage resources, ensuring that necessary memory is cleaned up automatically.
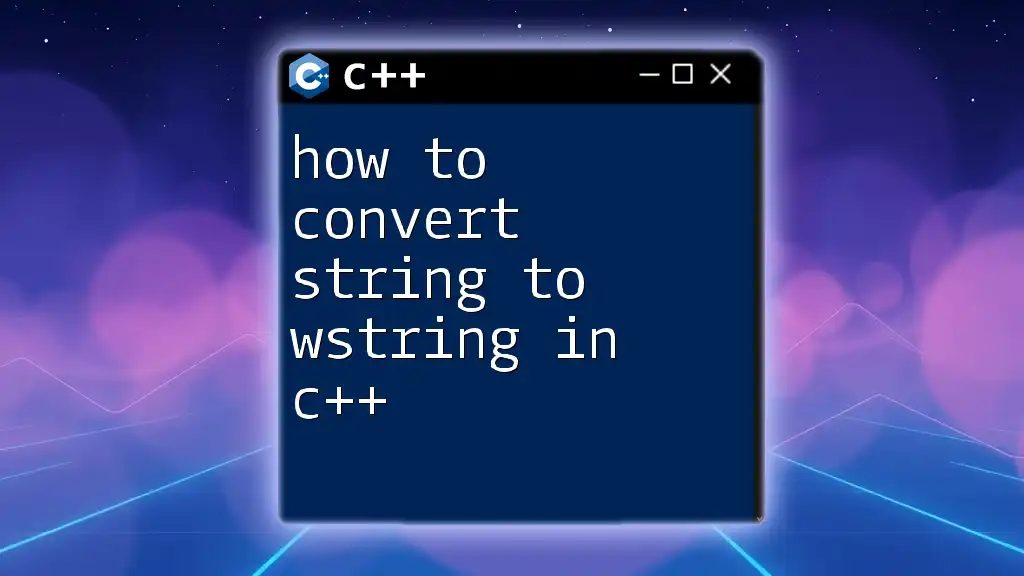
Conclusion
Understanding how to c++ convert string to char is crucial in programming, especially when interfacing with legacy systems or C-style APIs. The provided methods—from using `c_str()` to manual copying—each have their unique use cases, strengths, and weaknesses. Experiment with these approaches and find what best suits your programming needs. By mastering these techniques, you will enhance your skills and improve your C++ projects' efficiency and reliability.
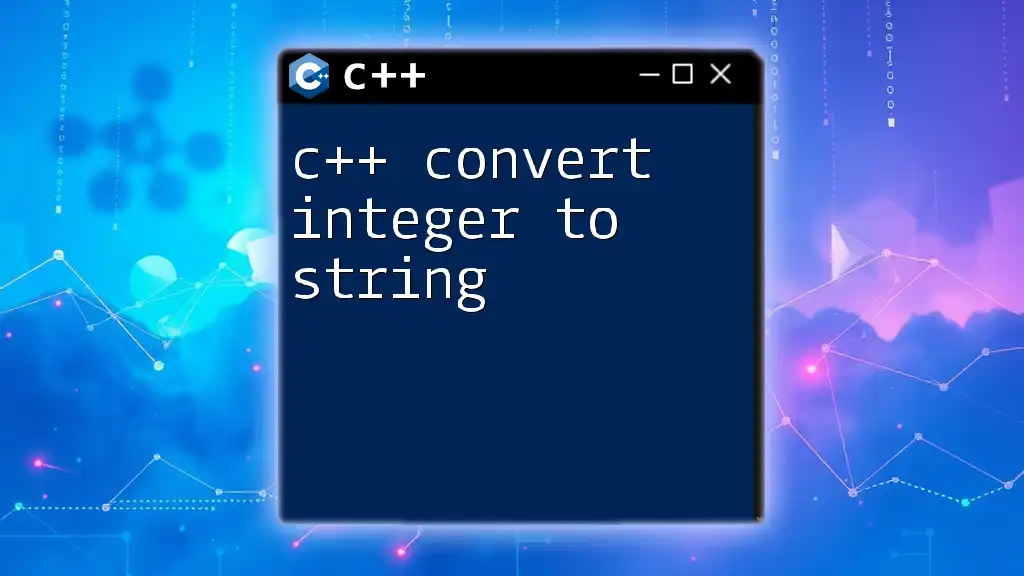
Additional Resources
For further learning, various tutorials and resources are available online that cover more advanced topics regarding C++ strings and characters. You can also refer to the official C++ documentation for in-depth explanations and examples. Connecting with coding communities or forums can also help deepen your understanding and resolve any specific queries you may have.