In C++, you can convert an integer to a string using the `std::to_string` function, which provides a simple and efficient way to achieve this.
#include <string>
#include <iostream>
int main() {
int number = 42;
std::string strNumber = std::to_string(number);
std::cout << strNumber; // Output: 42
return 0;
}
Understanding the Basics of C++ Data Types
What is an Integer in C++?
In C++, integers represent whole numbers and are typically used for counting, indexing, and performing arithmetic operations. There are several types of integer data types, including `int`, `long`, `short`, and `unsigned int`, each with its own range and memory consumption. For instance, the default `int` type in C++ usually holds values between -2,147,483,648 and 2,147,483,647.
What is a String in C++?
A string in C++ is often represented using the `std::string` class, which is part of the C++ Standard Library. This class provides a wide array of functions for manipulating sequences of characters. Unlike C-style strings (character arrays), `std::string` handles memory management automatically and provides a more flexible interface for string manipulation.
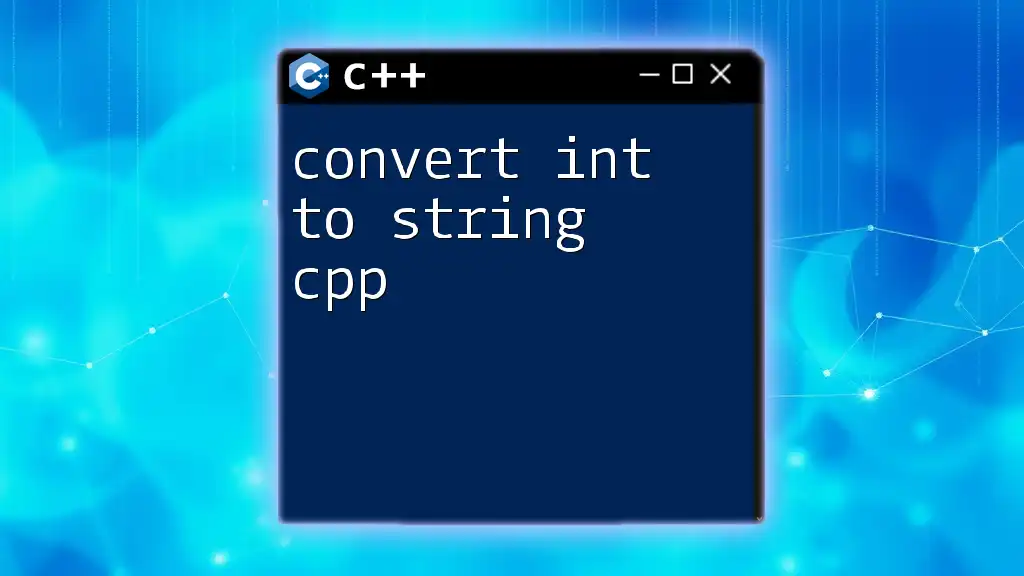
Why Convert Integer to String in C++?
Common Scenarios
Converting an integer to a string can be essential in various scenarios, such as:
- Formatting outputs for a user interface or creating user-readable files.
- String concatenation, where numerical values need to be combined with text.
- Serialization for storing or transmitting data more efficiently, such as when working with databases or APIs.
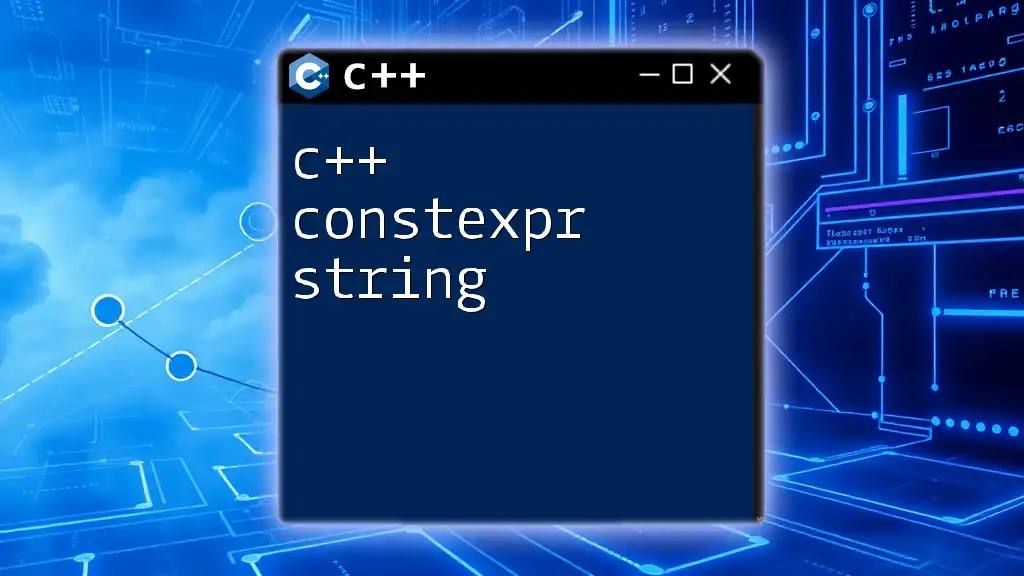
Methods to Convert Integer to String
Using `std::to_string()`
The simplest way to convert an integer to a string in C++ is by using the `std::to_string()` function. This method is straightforward and highly readable.
Code Snippet:
#include <iostream>
#include <string>
int main() {
int num = 123;
std::string str = std::to_string(num);
std::cout << "Converted string: " << str << std::endl;
return 0;
}
Explanation: The `std::to_string()` function takes an integer as an argument and returns a string representation of that integer. This method is easy to implement and very user-friendly, making it a popular choice among developers.
Advantages:
- Readability: It clearly conveys the intention of conversion from an integer to a string.
- Ease of use: Minimal code is required, making it beginner-friendly.
Using String Streams
Introduction to `std::ostringstream`
Another efficient method to convert integers to strings is by using `std::ostringstream`, part of the `<sstream>` header. This method allows for more complex formatting if desired.
Code Snippet:
#include <iostream>
#include <sstream>
int main() {
int num = 456;
std::ostringstream ss;
ss << num;
std::string str = ss.str();
std::cout << "Converted string: " << str << std::endl;
return 0;
}
Explanation: In this example, `std::ostringstream` creates an output string stream. The integer is inserted into the stream, and then `ss.str()` is called to retrieve the string representation. This approach not only converts integers but also allows for formatting and concatenation directly in the stream.
Advantages:
- Flexibility: Supports formatting with multiple data types in a unified manner.
- Concatenation: Easily combine strings and numbers using the stream insertion operator (`<<`).
Using C-style Strings with `sprintf`
Understand C-style Strings
For those who need to involve lower-level string operations, using C-style strings with `sprintf` is another option. However, it requires careful memory management to avoid buffer overflows.
Code Snippet:
#include <iostream>
#include <cstdio>
int main() {
int num = 789;
char buffer[50];
sprintf(buffer, "%d", num);
std::string str(buffer);
std::cout << "Converted string: " << str << std::endl;
return 0;
}
Explanation: In this approach, a character array (buffer) is defined to hold the string representation. `sprintf()` formats the integer into the buffer, and then a `std::string` is constructed from the buffer contents. While powerful, this method comes with the risk of buffer overflows if not correctly managed.
Considerations:
- Buffer management: Ensure the buffer is sufficiently large to hold all possible integer values.
- Safety: Prefer safer alternatives, in most cases, unless performance is critical.
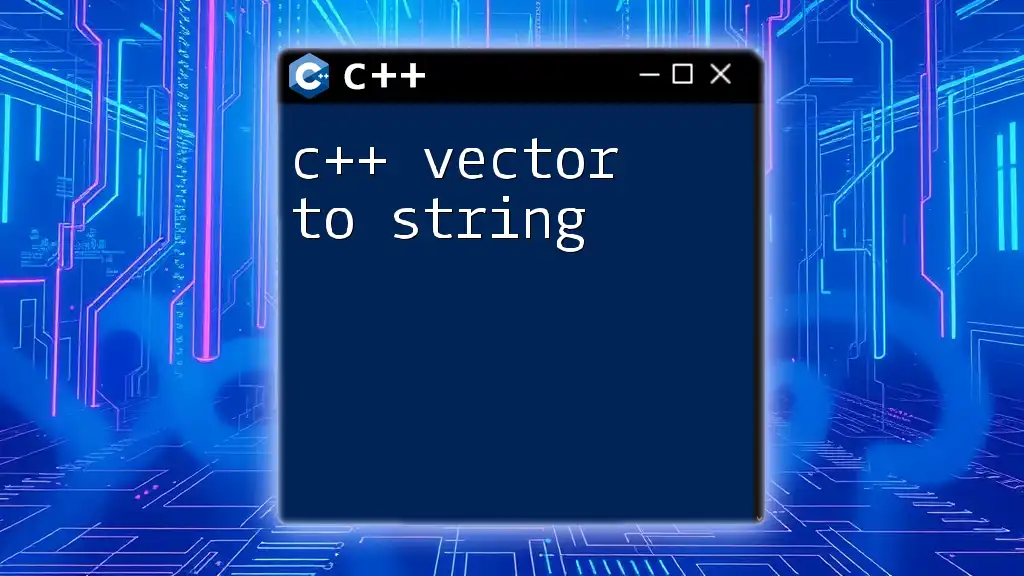
Performance Considerations
Efficiency of Different Methods
When you need to c++ convert integer to string, it's essential to consider the efficiency of each method. Generally, `std::to_string()` is the most efficient and straightforward for simple conversions. In contrast, `std::ostringstream` offers more flexibility but may come with a slight performance overhead due to stream operations. Using `sprintf()` can be very fast but lacks the safety features of the C++ Standard Library.
Memory Management
While utilizing any of these methods, understanding memory management is crucial. Automatic memory management in C++ strings eliminates many pitfalls of manual memory handling, but careful attention is still required when using C-style strings.
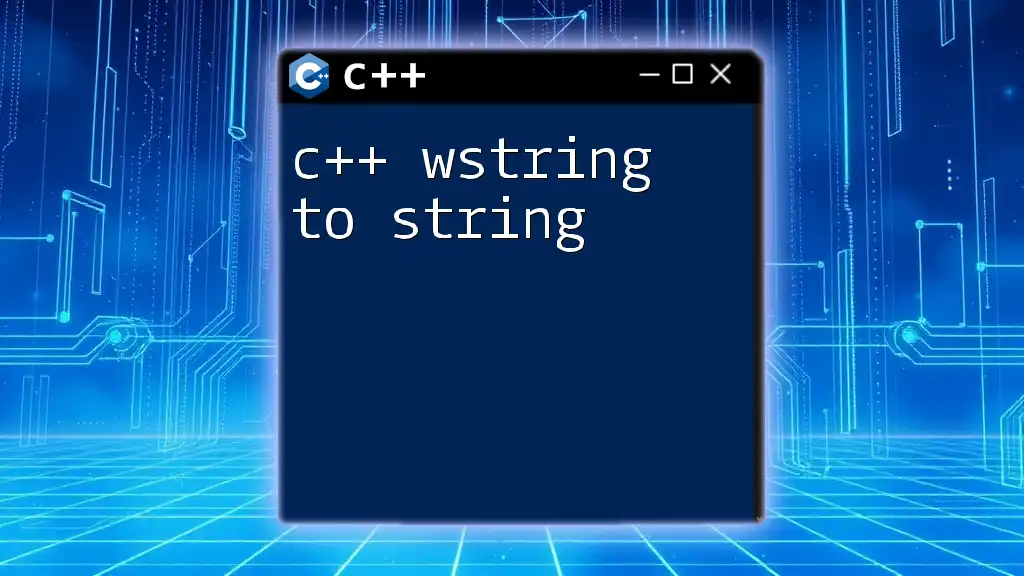
Common Errors and Troubleshooting
Type Mismatch
When converting integers to strings, a common error is passing the wrong type to conversion functions. For instance, attempting to feed a floating-point number into `std::to_string()` expecting it to directly convert could lead to unexpected behavior. Ensuring that the correct data type is used and handled properly is essential.
Performance Pitfalls
Another error is using the conversion method inefficiently. For example, unnecessarily using `sprintf` for frequent conversions can slow down the application due to the overhead of buffer creation and management.
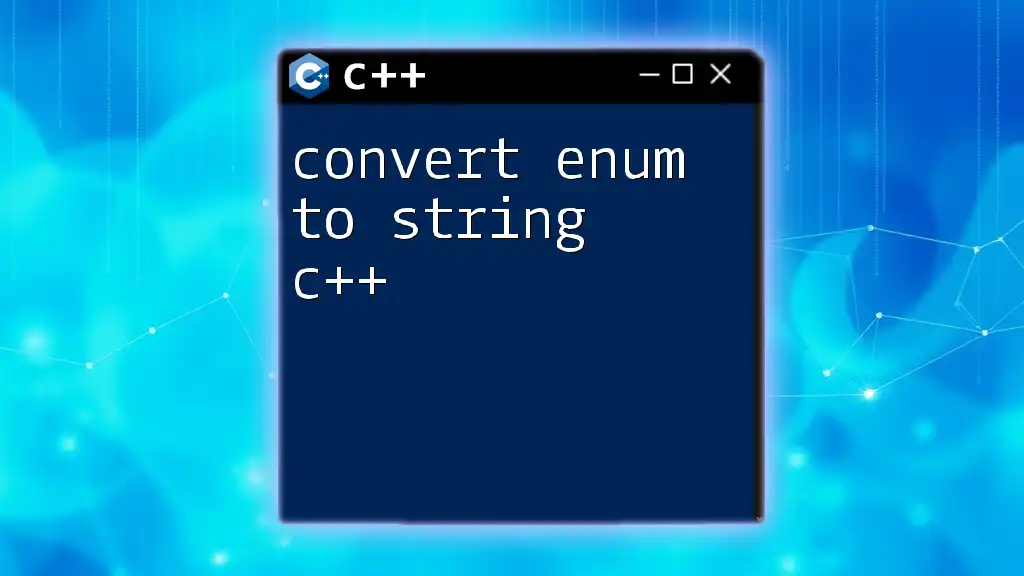
Conclusion
In summary, converting integers to strings is a vital task in C++ programming that has a range of useful applications. Understanding different methods—from `std::to_string()` for straightforward cases to `std::ostringstream` for more complex needs—empowers developers to choose the best tool for their specific requirements. By practicing these methods and being aware of potential pitfalls, you'll master the nuances of string conversion in C++.
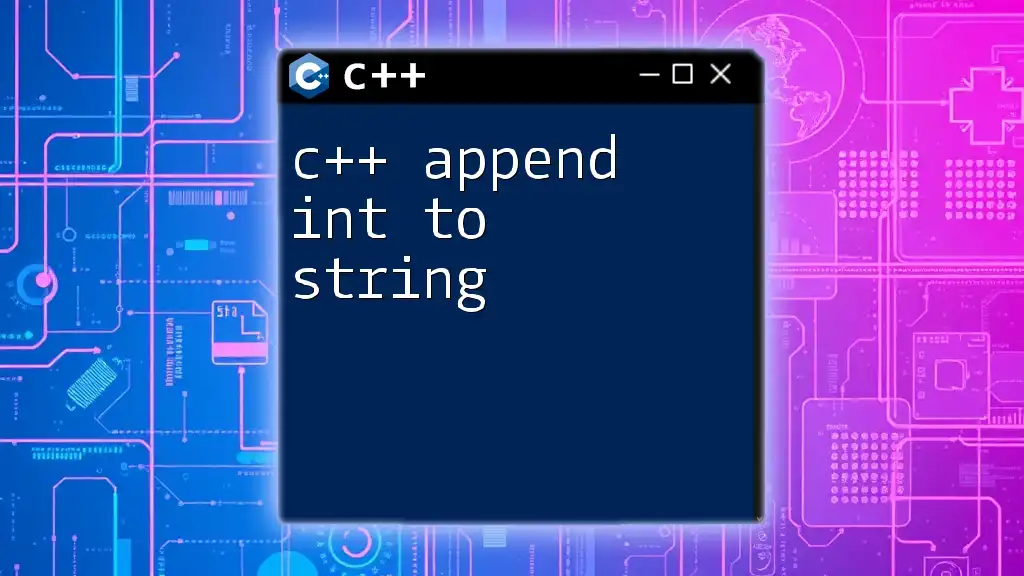
FAQ Section
How to convert an integer to a string in C++?
The best way to convert an integer to a string in C++ is by using `std::to_string()`. For more complex formatting, consider using `std::ostringstream`.
What is the difference between `std::string` and C-style strings?
`std::string` is a safer, easier-to-use string class that manages memory automatically, whereas C-style strings are character arrays that require manual memory management.
Can I convert other data types to strings in C++?
Yes, similar methods can be used to convert other types such as floats and doubles, typically leveraging `std::to_string()` or string streams.