In C++, you can convert an enum to a string by implementing a function that maps the enum values to their respective string representations using a switch statement or a lookup table. Here's an example code snippet:
#include <iostream>
#include <string>
enum Color { Red, Green, Blue };
std::string enumToString(Color color) {
switch (color) {
case Red: return "Red";
case Green: return "Green";
case Blue: return "Blue";
default: return "Unknown Color";
}
}
int main() {
Color myColor = Green;
std::cout << "The color is: " << enumToString(myColor) << std::endl;
return 0;
}
What is an Enum?
Definition of Enum
An enum is a user-defined type in C++ that enables you to define a variable that can hold a set of predefined constant values. They enhance code clarity and can make programs easier to maintain. For example, you might create an enum for different colors, states, or error codes.
Benefits of Using Enums
Enums contribute significantly to code quality and safety by:
- Improving code readability: Instead of using arbitrary numbers or strings, enums provide meaningful names.
- Providing type safety: Enums restrict the values a variable can hold, reducing potential coding errors.
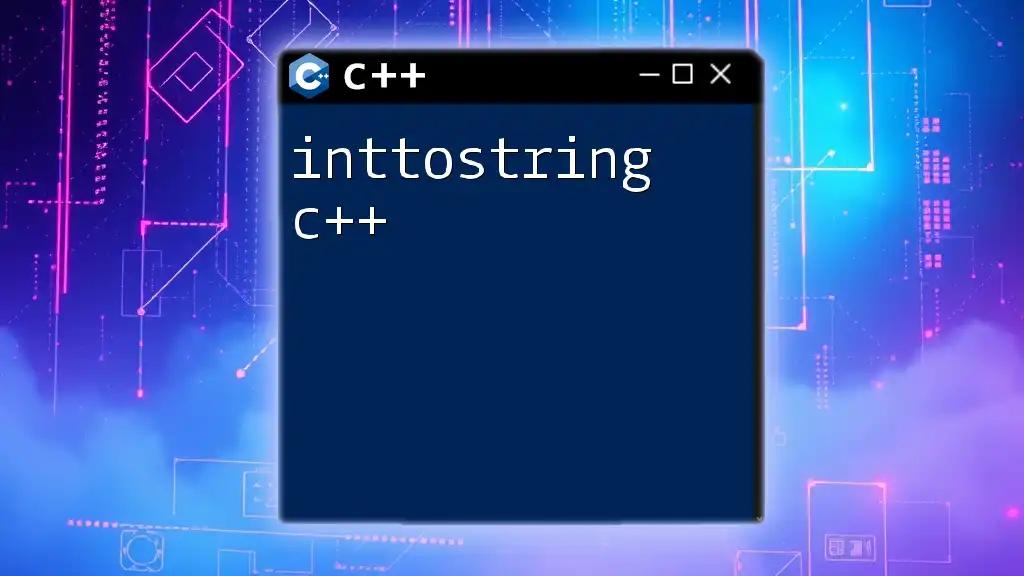
Why Convert Enum to String?
Converting enums to strings is often necessary in various situations. Common use cases include:
- Logging and debugging: When an application runs into an issue, printing out meaningful string representations of enum values can provide insight into what went wrong.
- Storing enum values in a more readable format: When sending data over a network or saving to a database, using human-readable text is usually more viable.
- Displaying user-friendly messages in GUIs: Providing a graphical user interface that displays enum values as strings enhances user experience.

Basic Enum to String Conversion Techniques
Using a Switch Statement
One straightforward way to convert enums to strings is utilizing a switch statement. This method involves defining a function that matches the enum values to their corresponding string representations.
Here’s a simple implementation:
enum Color { RED, GREEN, BLUE };
std::string enumToString(Color color) {
switch (color) {
case RED: return "Red";
case GREEN: return "Green";
case BLUE: return "Blue";
default: return "Unknown color";
}
}
In this example, the function checks the passed enum value and returns the appropriate string. It's a clear and effective approach, but it can become cumbersome with numerous entries.
Using an Array of Strings
Another efficient way to convert an enum to a string is to use an array of strings. This approach requires you to maintain a parallel array with string representations corresponding directly to the enum values.
Here’s how this can be implemented:
enum Color { RED, GREEN, BLUE };
const std::string ColorNames[] = { "Red", "Green", "Blue" };
std::string enumToString(Color color) {
return ColorNames[color];
}
In this example, the index of the string in the `ColorNames` array corresponds to the enum value. This method is sleek and makes it easy to add new enum values.

C++ Enum Class to String Conversion
What is an Enum Class?
An enum class is a scoped enumeration introduced in C++11 that provides better type safety and scoping, making enums more robust and manageable. Unlike traditional enums, enums under an enum class do not implicitly convert to integers, hence minimizing accidental value assignments.
Strategies for Enum Class to String Conversion
Using a Switch Statement
You can use a switch statement for enum classes just as you would with traditional enums. However, you need to specify the scope of the enum when referencing its values.
Here’s an example:
enum class Color { RED, GREEN, BLUE };
std::string enumClassToString(Color color) {
switch (color) {
case Color::RED: return "Red";
case Color::GREEN: return "Green";
case Color::BLUE: return "Blue";
default: return "Unknown color";
}
}
This method is similar to its non-class counterpart, but it provides the added advantage of type safety.
Using a Map
For larger enums or when adding/removing values frequently, using a map can be more effective. This approach can be cleaner and less prone to error.
Here's how to implement it:
#include <map>
enum class Color { RED, GREEN, BLUE };
std::map<Color, std::string> ColorMap = {
{ Color::RED, "Red" },
{ Color::GREEN, "Green" },
{ Color::BLUE, "Blue" }
};
std::string enumClassToString(Color color) {
return ColorMap[color];
}
Using a map allows for easy modifications; adding new colors only involves updating the map, rather than rewriting larger code blocks.

Best Practices for Converting Enums to Strings
Avoiding Magic Numbers
Using enums avoids the introduction of magic numbers—arbitrary values in your code that can be unclear or confusing. By leveraging enums, you enhance maintainability and readability, making your intentions clear.
Considerations for Error Handling
When converting enums to strings, consider that the enum values represent a limited set of options. Implementing error handling becomes crucial, especially for unexpected values.
You might include a default case in switch statements or handle invalid keys in maps gracefully. Here’s a robust way to handle unexpected enum values:
std::string enumClassToString(Color color) {
auto it = ColorMap.find(color);
if (it != ColorMap.end()) {
return it->second;
}
return "Unknown color"; // Fallback for error handling
}
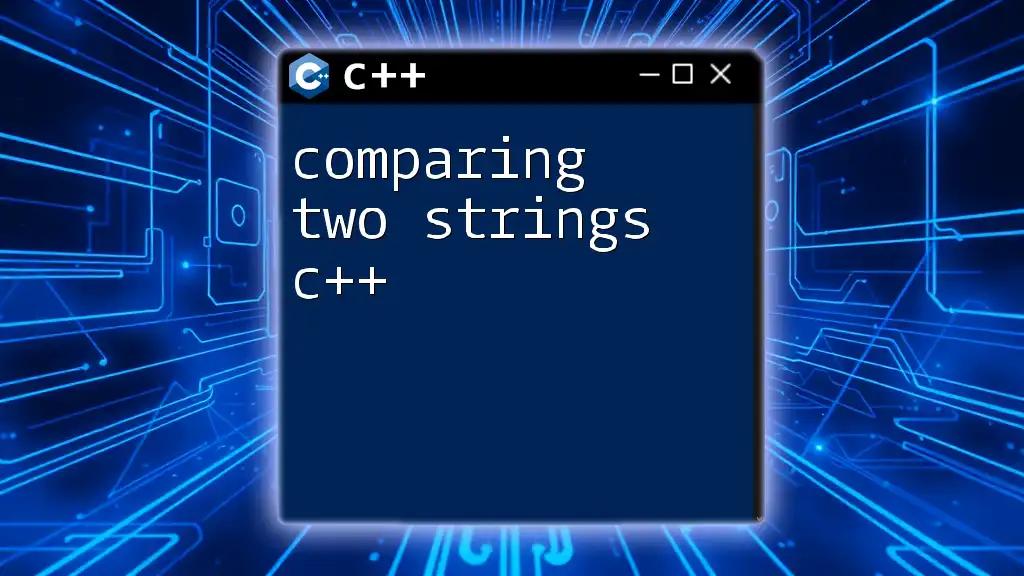
Conclusion
Converting enums to strings in C++ is a necessary skill that enhances the functionality and readability of your applications. Whether using switch statements, arrays, or maps, picking the right method based on your specific use case is fundamental. The best practices we've discussed can help ensure your enum-string conversions remain efficient and informative, paving the way for robust code. Implementing these techniques can greatly benefit your coding workflow, making your applications far more maintainable and user-friendly.
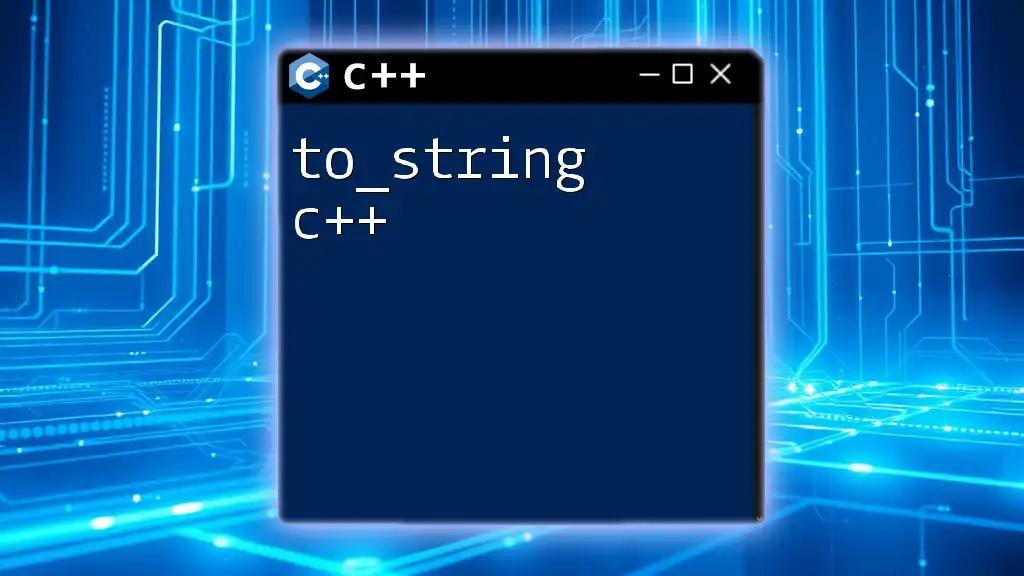
Further Reading and Resources
For deeper insights into enums and their usage, consider exploring the C++ Standard Library documentation, tutorials on advanced enum usage, or community forums that specialize in C++ programming. These resources can provide valuable learning opportunities as you continue to develop your C++ skills.