In C++, you can convert a char array to a std::string by using the string constructor that takes a char pointer as an argument.
Here's a code snippet demonstrating this:
#include <iostream>
#include <string>
int main() {
const char* charArray = "Hello, World!";
std::string str(charArray);
std::cout << str << std::endl;
return 0;
}
Understanding Char Arrays and Strings in C++
Definition of Char Arrays
A char array is essentially a collection of characters stored in contiguous memory locations. It is characterized by its need for a null terminator (`'\0'`) to signify the end of the string. This is critical for functions that operate on C-strings to know where the string ends.
For instance, you can declare a char array like this:
char message[] = "Hello, World!";
In this example, `message` is an array of characters that gets automatically null-terminated by the compiler. Understanding this structure is essential when you aim to convert a char array to a string in C++.
Definition of Strings in C++
On the other hand, in modern C++, we use the `std::string` class from the Standard Library. This class provides dynamic memory management and many convenient methods for manipulation. Unlike char arrays, `std::string` can automatically resize itself as needed, offering greater flexibility.
Here's a simple example of a `std::string`:
std::string strMessage = "Hello, World!";
The advantages of using `std::string` over char arrays include:
- Automatic memory management
- Enhanced functionality for string manipulation
- Easier concatenation and formatting
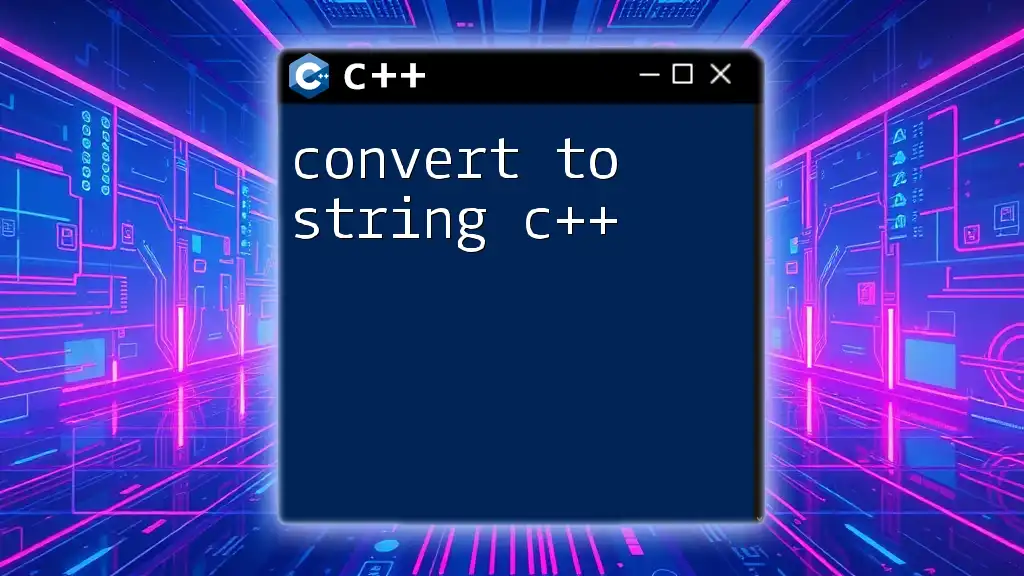
Why Convert Char Array to String?
The purpose of converting a char array to a string often arises in various scenarios such as string manipulation, data processing, or user input. Whenever you receive a C-style string (char array), you may wish to utilize the superior functionality of `std::string`. This conversion can also streamline code and improve readability.
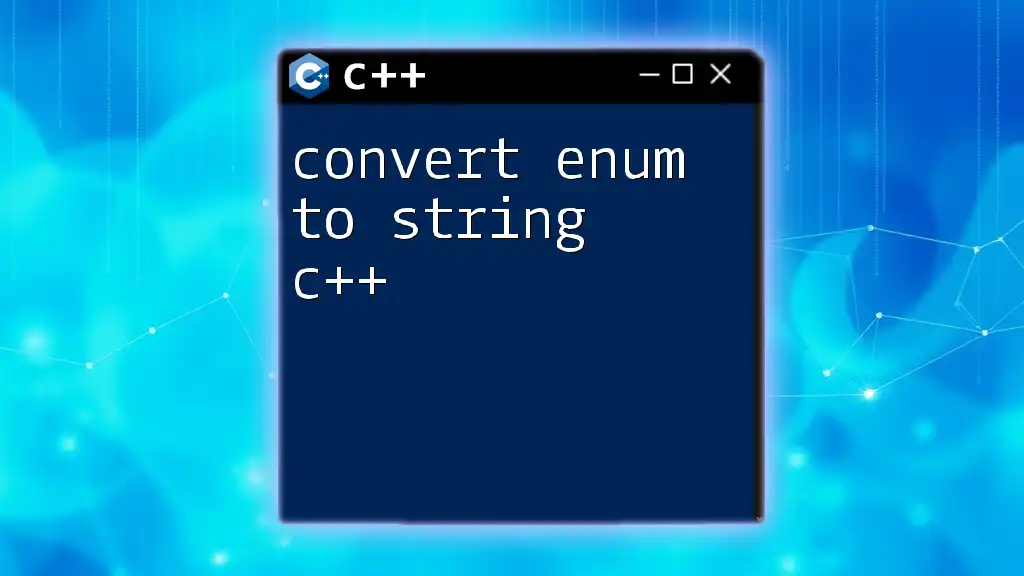
Methods to Convert Char Array to String in C++
Using the `std::string` Constructor
One of the simplest ways to convert a char array to a string is by utilizing the `std::string` constructor. The constructor can take a char array as an argument, effectively initializing a `std::string`.
Consider this example:
char message[] = "Hello, World!";
std::string strMessage(message);
This method is particularly useful due to its brevity and simplicity, instantly converting the character array to a string, with attention to null-termination handled successfully.
Using `std::string::assign()`
Another effective method for converting a char array to a string is through the `assign()` method of `std::string`. This allows for greater flexibility since the string can be assigned any char array even after its initialization.
Here’s how it works:
char message[] = "Hello, World!";
std::string strMessage;
strMessage.assign(message);
This approach can be handy if you need to convert multiple char arrays into the same string instance throughout your program.
Using String Literals Directly
For initializing a string directly from string literals, you can simply use the initialization method. This approach does not require a char array at all.
For example:
std::string strMessage = "Hello, World!";
This direct assignment is straightforward and optimal for constants. It eliminates any char array's overhead and offers compile-time efficiency.
Using `std::copy()`
For a more manual method of conversion, you can use the `std::copy()` algorithm from the `<algorithm>` header. This allows you to copy the characters from the char array to the string effectively.
Here’s how you can implement it:
#include <algorithm>
#include <iterator>
char message[] = "Hello, World!";
std::string strMessage;
std::copy(message, message + sizeof(message) - 1, std::back_inserter(strMessage));
This method gives you more control but requires additional headers and might be seen as unnecessarily verbose for simple tasks.
Using the `+` Operator
You can also concatenate a char array to a `std::string` using the `+` operator, thereby directly forming a new `std::string`.
Here's an example of this method:
char message[] = "Hello, ";
std::string strMessage = message + "World!";
While this method appears convenient, ensure you consider its context in coding practices, as it can lead to confusion for complex operations.
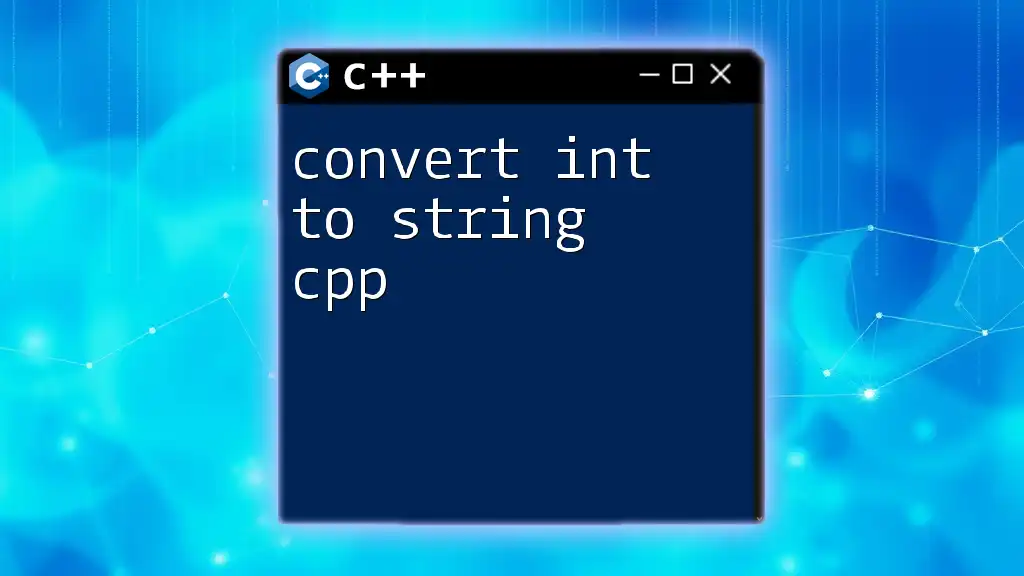
Performance Considerations
When discussing how to convert char array to string in C++, it's essential to weigh the efficiency of each method. Each method has performance implications depending on its context.
- The constructor method and the `assign()` method are generally efficient for most use cases.
- Direct initialization with string literals is often the quickest way to create a string.
- `std::copy()` is more verbose and can be less efficient due to the overhead of iterators, but it provides flexibility in more complex scenarios.
Always choose the method that fits your particular need without compromising on readability and performance.
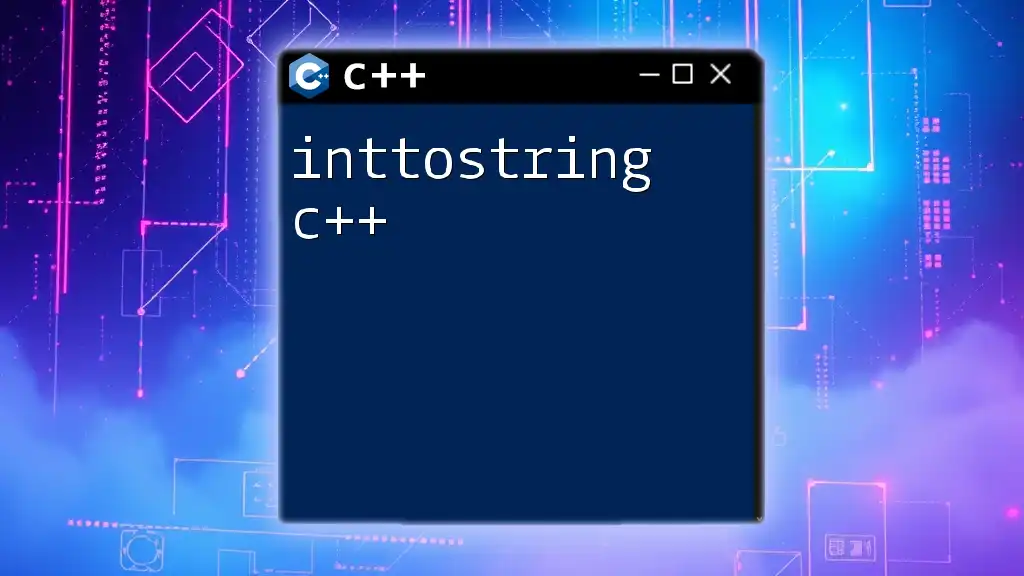
Common Pitfalls to Avoid
While converting char arrays to strings is straightforward in C++, certain pitfalls may arise:
- Forgetting Null-Termination: When manually creating char arrays, ensure they are null-terminated; failing to do so can lead to undefined behavior.
- Memory Management: Pay attention to memory management when converting strings in larger applications. Make sure to avoid unnecessary copies that could lead to increased memory consumption.
- Using the Wrong Conversion Method: Ensure that you select the appropriate method for conversion based on your needs. Overly complex methods for simple conversions can muddle your code.
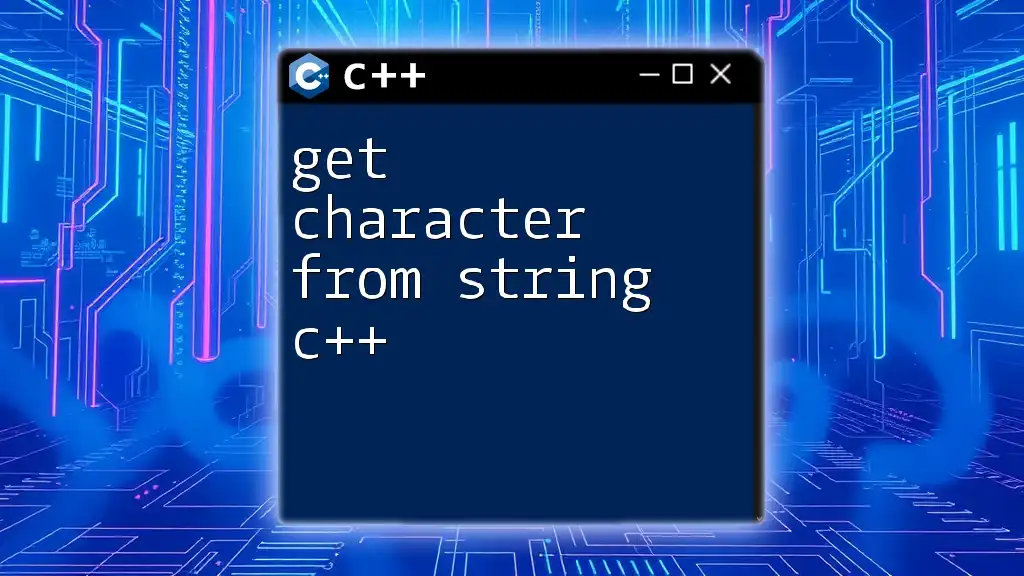
Conclusion
Converting a char array to a string in C++ is a fundamental skill that enhances your programming capabilities. With multiple methods to achieve this goal, understanding the weaknesses and strengths of each will allow for better implementation in your programs.
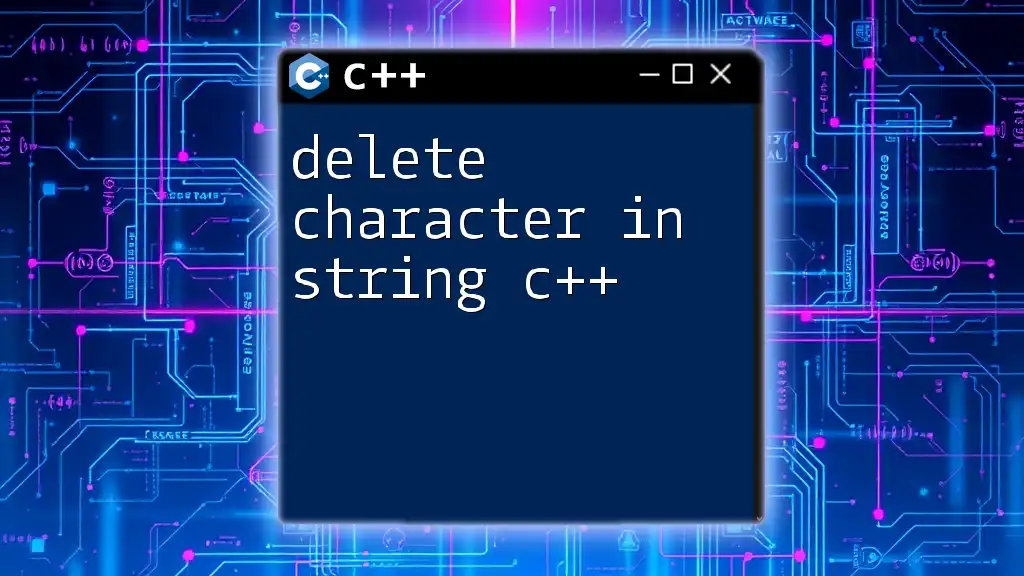
FAQs
Can I convert a `const char*` to a `std::string`?
Yes, you can use the `std::string` constructor or `assign()` method. Both will safely handle the conversion for `const char*`.
What is the difference between `std::string` and `std::stringstream`?
While `std::string` is used for string storage and manipulation, `std::stringstream` is designed for string-based input and output, similar to how input/output streams work with files or console. `std::stringstream` allows for formatting operations and parsing strings easily.
Is there a way to convert `std::string` back to a char array?
Yes, you can obtain a char array from a `std::string` using the `c_str()` method:
std::string strMessage = "Hello, World!";
const char* charArray = strMessage.c_str();
Are there any libraries that simplify this conversion?
While the Standard Library provides robust functionality, libraries like Boost offer additional utilities for string handling. However, for most common cases, the standard methods are sufficient.
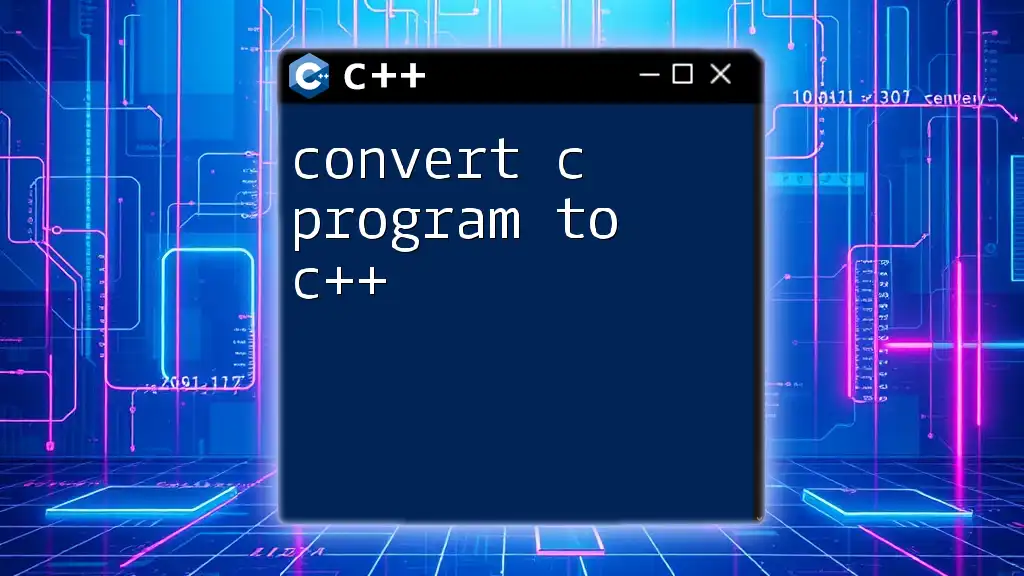
Call to Action
Now that you have a comprehensive understanding of how to convert char array to string c++, I encourage you to try out the various methods discussed today. Experiment with your own char arrays and strings. For more lessons on C++ commands and techniques, don’t forget to sign up for our courses and tutorials. Happy coding!