In C++, you can determine the length of a char array using the `strlen` function from the `<cstring>` library, which counts the number of characters until it reaches the null terminator `'\0'`.
#include <iostream>
#include <cstring>
int main() {
char myArray[] = "Hello, World!";
std::cout << "Length of array: " << strlen(myArray) << std::endl; // Output: 13
return 0;
}
Understanding Char Arrays in C++
What is a Char Array?
In C++, a char array is a type of array specifically designed to hold characters. It is generally used to represent strings in a manner that is both efficient and flexible within the constraints of C++'s syntax.
A fundamental difference between char arrays and C++ strings is that char arrays are essentially a block of memory containing characters, typically terminated by a null character (`'\0'`). This null character signifies the end of the string, allowing functions to determine where the string finishes.
The memory layout for a char array typically consists of:
- A sequence of characters
- A null terminator at the end
Why Measure Char Array Length?
Knowing the length of a char array is crucial for various reasons:
- Memory Management: Understanding how much memory is utilized helps avoid overflow and other memory-related issues.
- String Manipulation: Many algorithms, such as searching and sorting, rely on the specific length of the string for their operations.
- Data Processing: When reading data from inputs or files, knowing the length assists in parsing and managing the data efficiently.
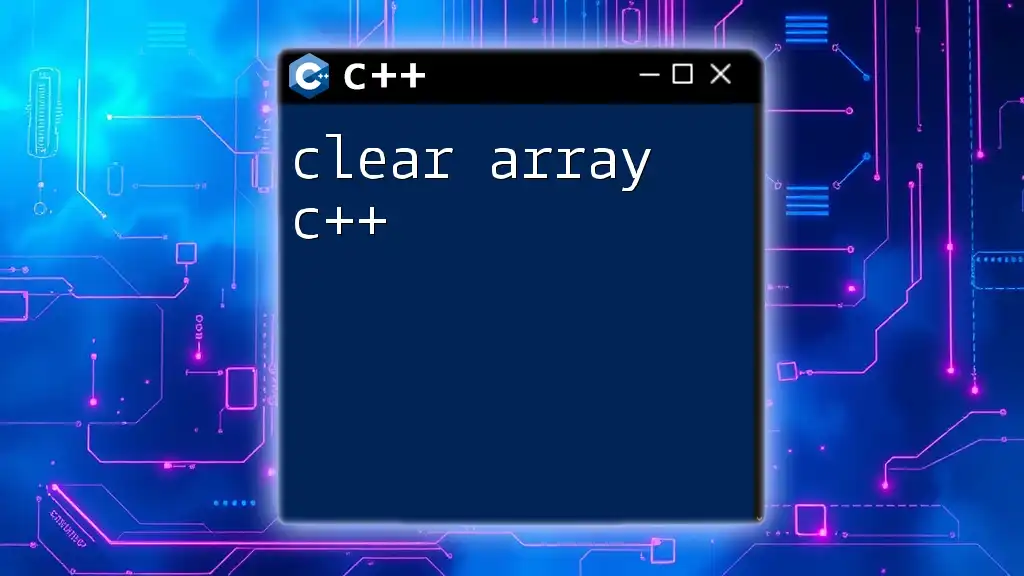
Measuring the Length of a Char Array in C++
Using `strlen()` Function
One of the simplest and most commonly used methods to measure a char array's length is through the `strlen()` function provided by the C++ Standard Library.
The basic syntax is:
size_t strlen(const char* str);
Here's how to use it in practice:
#include <iostream>
#include <cstring>
int main() {
const char* str = "Hello, World!";
size_t length = strlen(str);
std::cout << "Length of char array: " << length << std::endl; // Output: 13
return 0;
}
In this example, `strlen()` calculates the length of the string by counting all characters until it encounters the null terminator.
Keep in mind that `strlen()` only works on null-terminated strings. If the string lacks a null terminator, it can lead to undefined behavior since it will continue counting beyond the allocated memory until it randomly hits a null character.
Manual Calculation of Char Array Length
If you want finer control or need to avoid using library functions, you can manually compute the length of a char array. This approach is straightforward and educational, allowing you to understand how strings are structured at the memory level.
Here’s one way to do it:
#include <iostream>
size_t manual_strlen(const char* str) {
size_t length = 0;
while (*str++ != '\0') {
length++;
}
return length;
}
int main() {
const char* str = "Hello, World!";
std::cout << "Length of char array: " << manual_strlen(str) << std::endl; // Output: 13
return 0;
}
In this code snippet, the function `manual_strlen()` iteratively traverses the char array until it encounters the null terminator, incrementing a `length` variable. This approach provides insight into how strings are processed in memory.
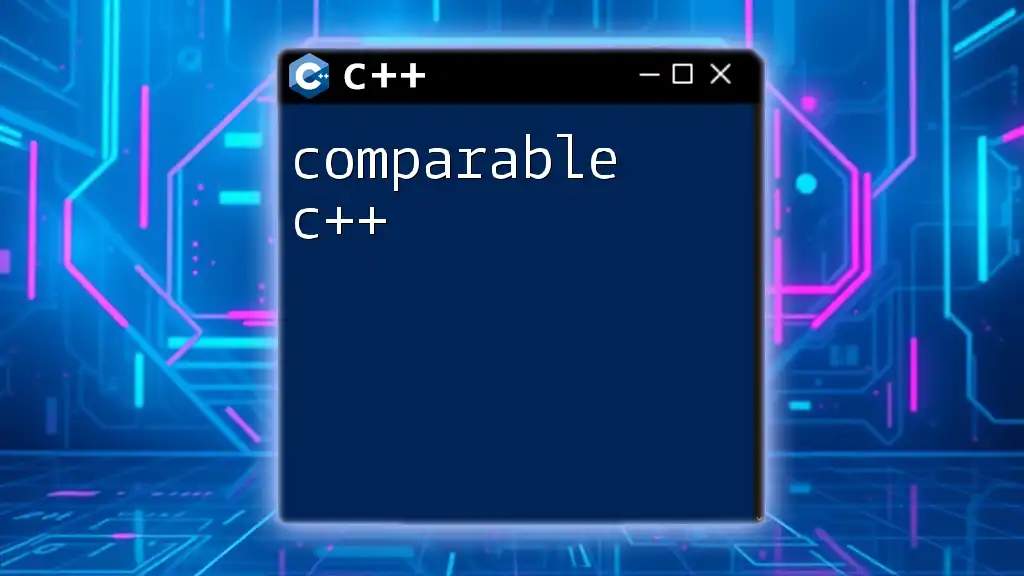
Special Cases when Measuring Char Array Length
Uninitialized Char Arrays
A common pitfall arises when dealing with uninitialized char arrays. If a character array isn't explicitly initialized, its contents are undefined, and using functions like `strlen()` may lead to consequences such as accessing random memory.
For example:
#include <iostream>
int main() {
char str[100]; // Uninitialized char array
std::cout << "Length of char array: " << strlen(str) << std::endl; // Undefined behavior
return 0;
}
In such cases, it's critical to always initialize your char arrays before usage to avoid catastrophic errors.
Arrays with Null Characters
Including null characters within a char array can significantly affect the computed length. For instance, the following example demonstrates how `strlen()` only counts characters before the first occurrence of `'\0'`:
#include <iostream>
#include <cstring>
int main() {
const char str[] = "Hello\0World"; // Includes null character in the array
std::cout << "Length counted by strlen: " << strlen(str) << std::endl; // Output: 5
std::cout << "Actual size of char array: " << sizeof(str) << std::endl; // Output: 11
return 0;
}
It's essential to be aware that the value returned by `strlen()` differs from the actual size of the array obtained by `sizeof()`, as `sizeof()` returns the total allocated memory size.
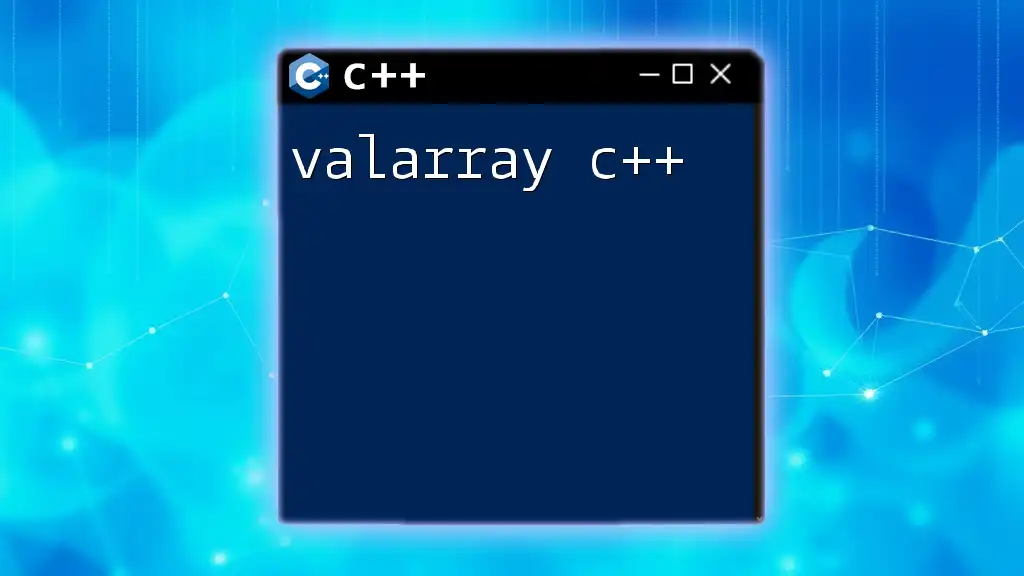
Best Practices for Working with Char Arrays
Avoiding Buffer Overflows
Buffer overflows occur when data exceeds the bounds of an allocated array, leading to memory corruption and potential security vulnerabilities. To mitigate these risks, always validate the size of the input before copying data into a char array. Functions like `strncpy()` allow you to set a limit for how many characters to copy, which can be incredibly useful.
When to Use `std::string` Instead
While char arrays are useful, C++ provides the `std::string` class, which offers several advantages:
- Automatic memory management, reducing the risk of overflow
- Built-in functions for manipulation and comparison
- Easier to read and maintain code
Here's a brief comparison:
#include <iostream>
#include <string>
int main() {
const char charArray[] = "Hello, World!"; // Using char array
std::string str = "Hello, World!"; // Using std::string
std::cout << "Char Array Length: " << strlen(charArray) << std::endl; // Output: 13
std::cout << "std::string Length: " << str.length() << std::endl; // Output: 13
return 0;
}
The `std::string` class simplifies string manipulation while handling memory allocation automatically, making it a preferred option for most applications in modern C++.
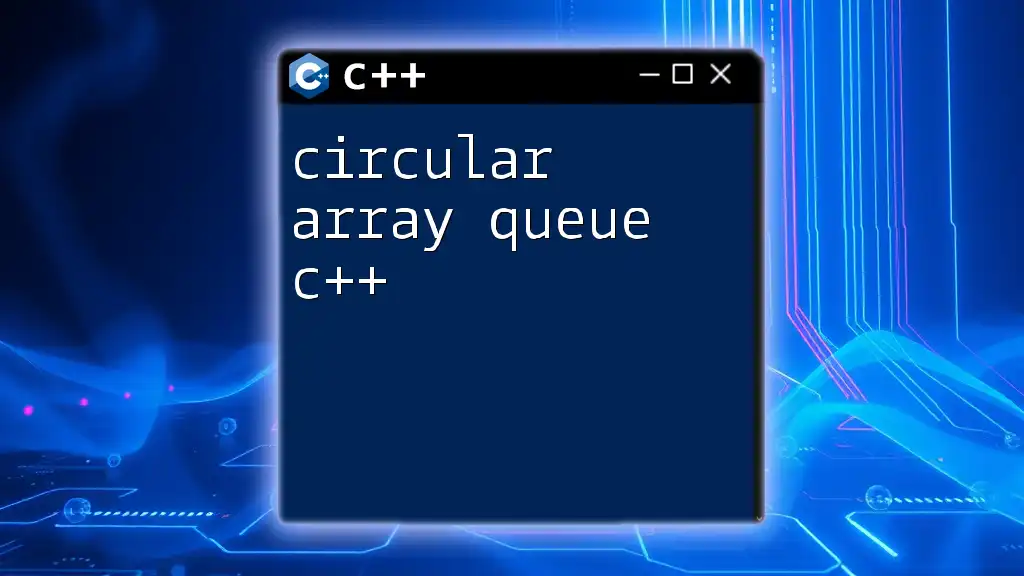
Common FAQs about Char Array Length in C++
How can I find the length of a char array without using `strlen()`?
You can manually traverse the array or use other algorithms, like using a loop, to count each character until the null terminator.
Can I store strings longer than 255 characters?
Yes, char arrays can store strings of varying lengths, limited only by the defined size of the array. However, allocating too small an array can lead to overflow, so always ensure adequate memory allocation.
What happens if I mistakenly add a character beyond the array's bounds?
Accessing memory outside the allocated space can lead to undefined behavior including program crashes, data corruption, or security vulnerabilities. Always ensure to validate your data bounds.
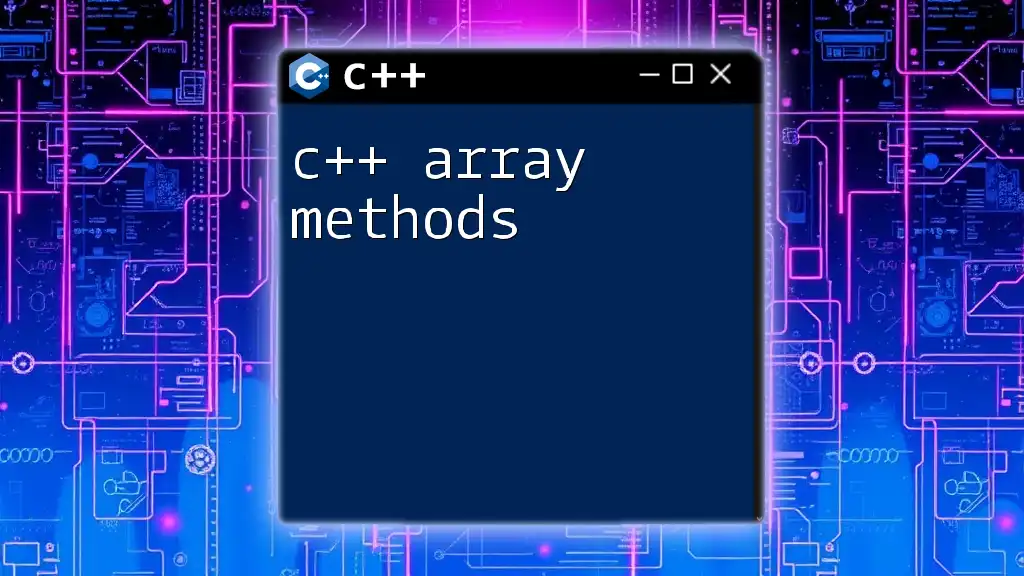
Conclusion
In summary, understanding char array length in C++ is an essential skill for any C++ developer. Mastering functions like `strlen()`, learning how to manually calculate lengths, and being cautious of common pitfalls can significantly enhance your ability to manipulate strings and handle data effectively in your applications. As best practices suggest, consider using `std::string` whenever possible to simplify your string handling while maintaining safety and readability in your code.