The `main` function in C++ serves as the entry point of a program and can accept command-line arguments through its parameters, enabling users to pass data when executing the program.
#include <iostream>
int main(int argc, char* argv[]) {
std::cout << "Number of arguments: " << argc << std::endl;
for(int i = 0; i < argc; ++i) {
std::cout << "Argument " << i << ": " << argv[i] << std::endl;
}
return 0;
}
Understanding the `main` Function in C++
The `main` function is the entry point for every C++ program. It is where the execution begins, making it essential for the program’s life cycle. The structure typically looks like this:
int main() {
// code goes here
return 0;
}
In this standard format, `main` returns an integer value, usually `0` to indicate successful execution.
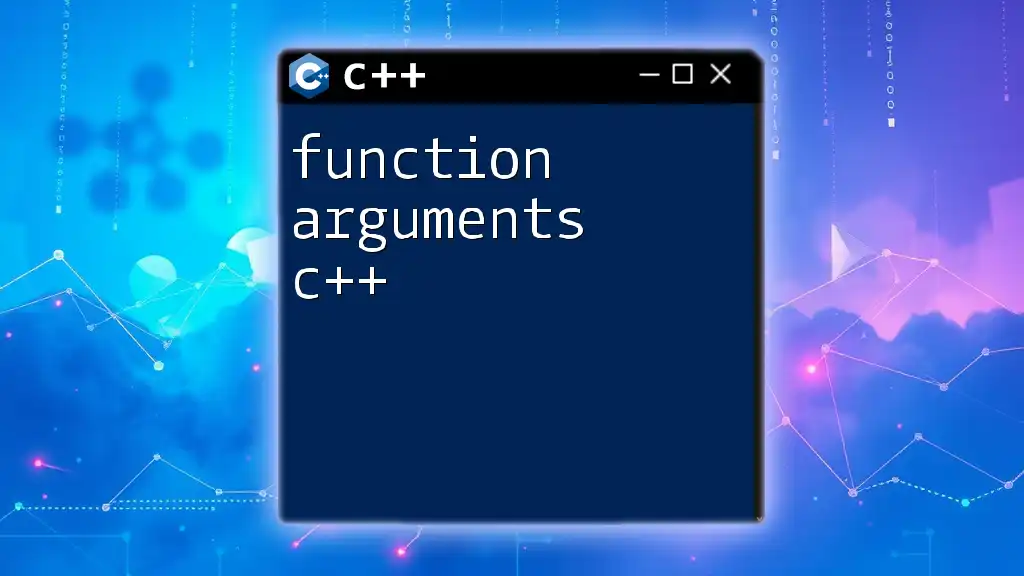
Main Arguments in C++
What Are Main Arguments?
In the context of C++, main arguments refer to the ability to pass parameters to the program via the command line when it's executed. These runtime parameters allow a program to behave differently based on user input or configuration settings given at startup.
Syntax of `main` with Arguments
To utilize command-line arguments, the signature of the `main` function expands to incorporate these parameters:
int main(int argc, char* argv[]) {
// code goes here
return 0;
}
Here, `argc` (argument count) is an integer representing the number of command-line arguments (including the program name), while `argv` (argument vector) is an array of C-style strings that hold the arguments themselves.
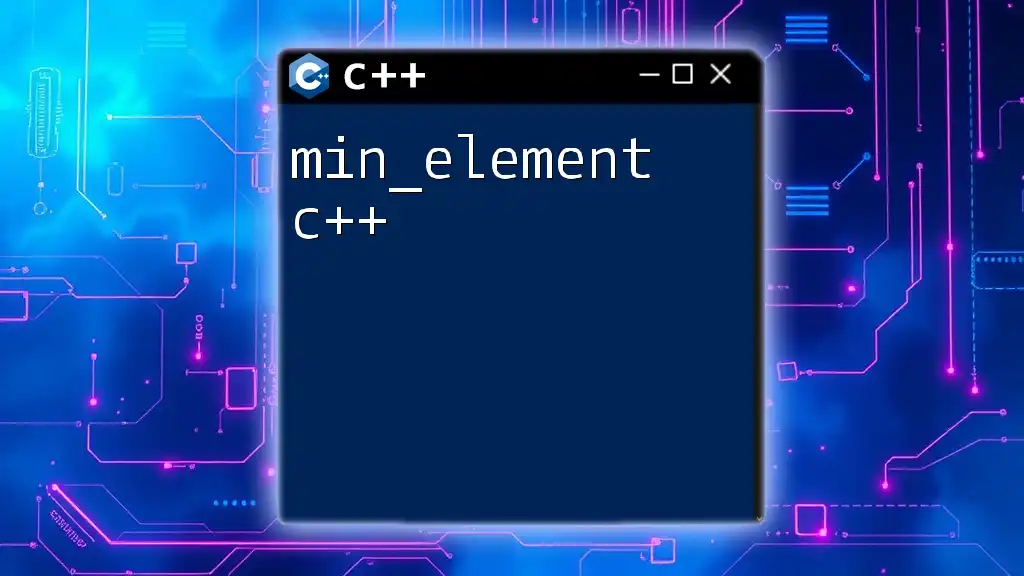
Working of `argc` and `argv`
Understanding `argc`
The `argc` parameter is crucial because it signifies how many arguments are passed when the program is executed. It’s important to note that this count always includes the name of the program itself, which is the first argument. Thus, `argc` will be at least `1`.
Exploring `argv`
The `argv` array is where the actual command-line arguments are stored. Each index of `argv` corresponds to an argument, with `argv[0]` holding the name of the program. The passing of arguments works with null-termination, typical of C-style strings, which helps identify the end of string data.
Example Code Snippet:
#include <iostream>
int main(int argc, char* argv[]) {
std::cout << "Number of arguments: " << argc << std::endl;
for (int i = 0; i < argc; ++i) {
std::cout << "Argument " << i << ": " << argv[i] << std::endl;
}
return 0;
}
In this example, the program outputs the total number of arguments received, along with the value of each argument. This demonstrates how `argc` and `argv` can be used to retrieve command-line input effectively.
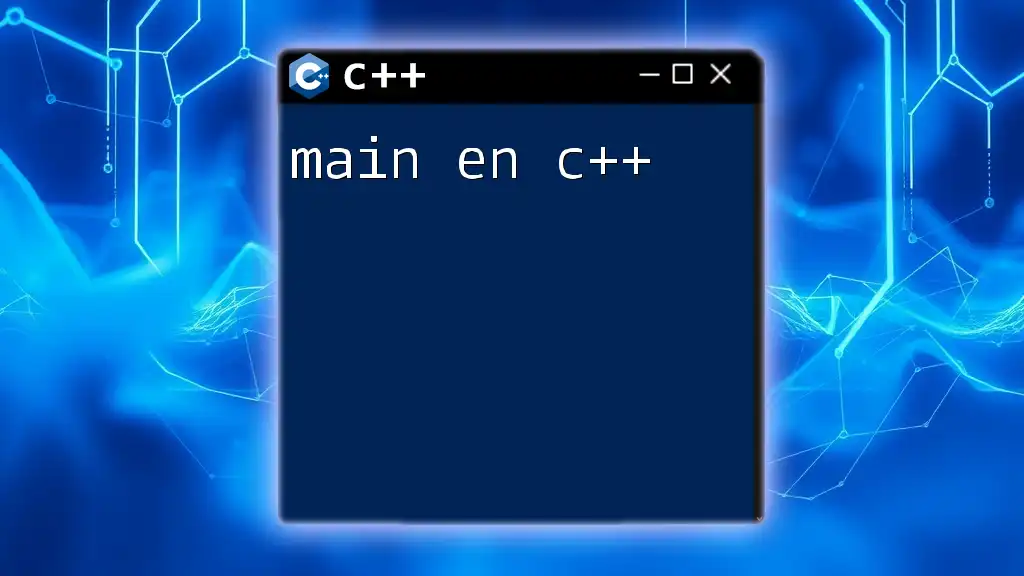
Types of Command-Line Arguments
Positional Arguments
Positional arguments are the most straightforward type of command-line arguments. They are provided in a specific order, and their sequence matters. For instance, if a program requires a file to process and an operation mode, those should be passed in the correct respective order.
Optional Arguments
Conversely, optional arguments are those that can enhance the program's functionality without being mandatory. They often allow users to modify behavior or provide additional preferences.
Example Code Snippet:
int main(int argc, char* argv[]) {
if (argc > 1) {
std::cout << "First argument: " << argv[1] << std::endl;
} else {
std::cout << "No additional arguments provided!" << std::endl;
}
return 0;
}
This code checks if any additional arguments were submitted and proceeds to display the first one if present. This illustrates the handling of optional inputs.
Flags and Options Format
Additionally, flags (such as `-h` or `--help`) can be implemented to offer options that modify application behavior. Parsing these flags requires careful inspection of the `argv` data to determine which parameters have been provided to the program.
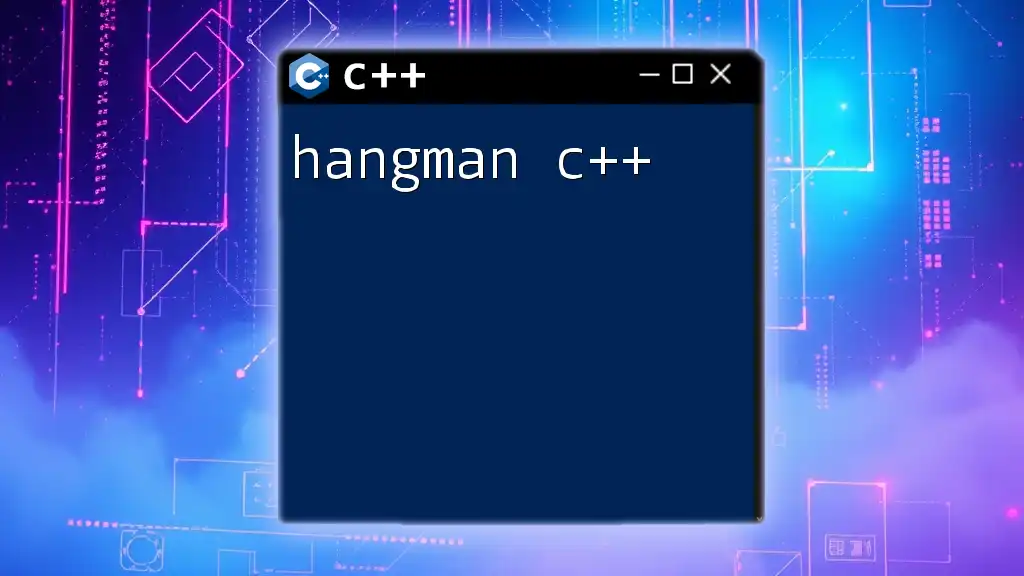
Practical Applications of `main` Arguments
Real-World Use Cases
Command-line arguments can significantly optimize functions in various applications. For example, tools for file processing can allow users to specify the file names and operational modes through command-line parameters, thus creating a flexible and efficient workflow.
Implementing Validation on Arguments
Effective C++ programming requires validating the command-line input to ensure the program executes smoothly. This involves checking that the number of arguments is correct, and that they are of the expected type (e.g., checking if a given string can be converted to an integer).
Example Code Snippet:
#include <iostream>
#include <cstdlib>
int main(int argc, char* argv[]) {
if (argc != 2) {
std::cerr << "Usage: " << argv[0] << " <number>" << std::endl;
return EXIT_FAILURE;
}
int number = std::atoi(argv[1]);
std::cout << "You entered: " << number << std::endl;
return EXIT_SUCCESS;
}
In this example, the program checks for exactly one additional argument. If the count does not match, it prints an error message and exits. Otherwise, it converts the entered argument to an integer and displays it.
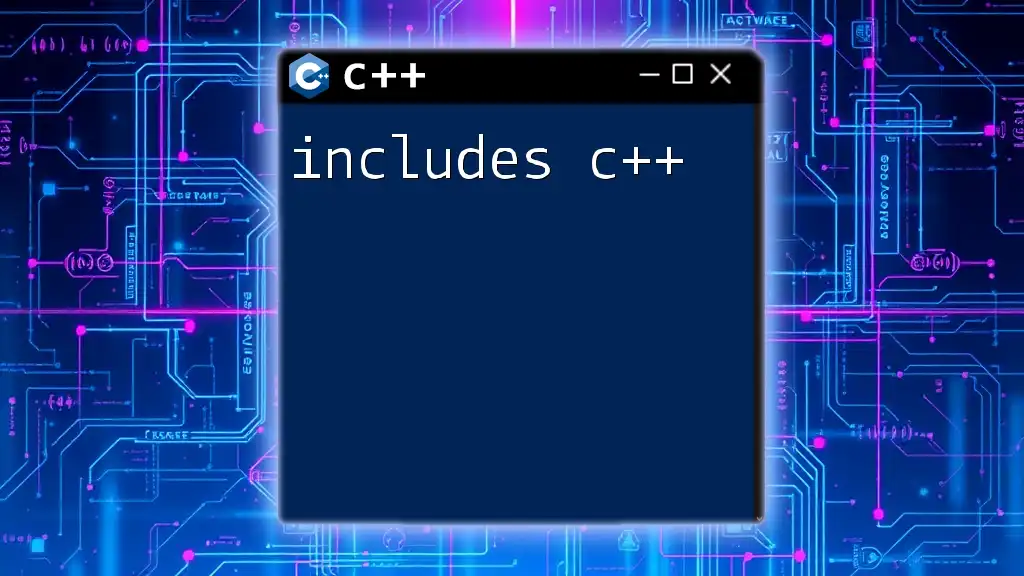
Advanced Usage of Command-Line Arguments
Parsing More Complex Arguments
As applications grow in complexity, a requirement arises for more advanced argument parsing. Numerous libraries can assist with this, such as `getopt` and `Boost.ProgramOptions`, which provide elegant ways to define and interpret command-line options.
Creating a Simple Argument Parser
It's also possible to create a custom argument parser for specific needs. Below is a very basic implementation:
#include <iostream>
#include <string>
void parseArguments(int argc, char* argv[]) {
for (int i = 1; i < argc; ++i) {
std::string arg = argv[i];
// Custom parsing logic
if (arg == "--help") {
std::cout << "Help message" << std::endl;
}
// Additional parsing logic could go here
}
}
int main(int argc, char* argv[]) {
parseArguments(argc, argv);
return 0;
}
This snippet provides a framework for handling custom parsing. If the `--help` flag is provided, a help message is displayed.
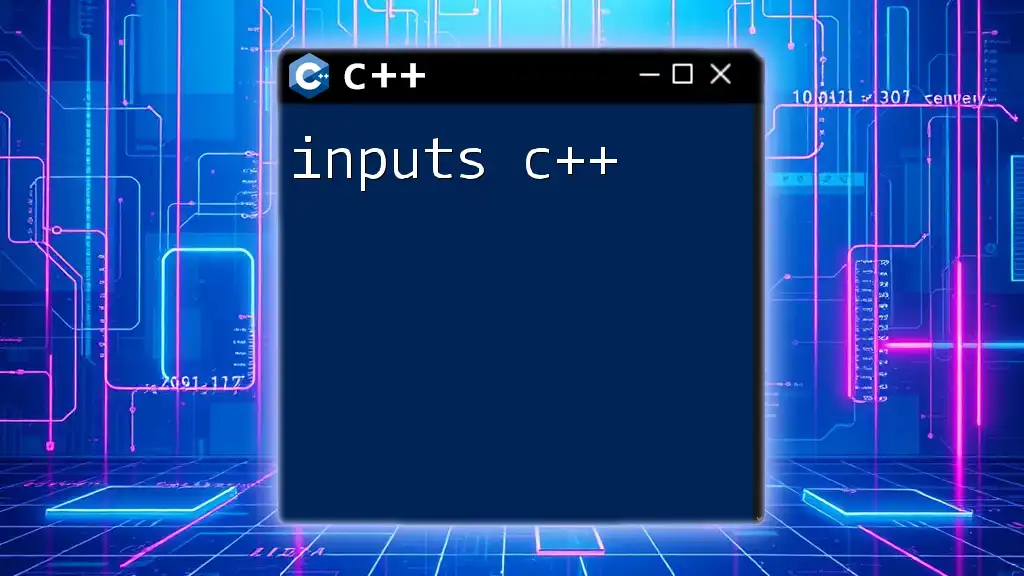
Conclusion
In summary, main arguments in C++ play a pivotal role in building flexible and robust applications. Understanding how to properly implement `argc` and `argv` can vastly enhance user interaction with your programs. By mastering these command-line argument techniques, you can create software that is more versatile, adaptive, and user-friendly. Encouragement is given for readers to practice the implementations discussed in various C++ projects to reinforce their learning.
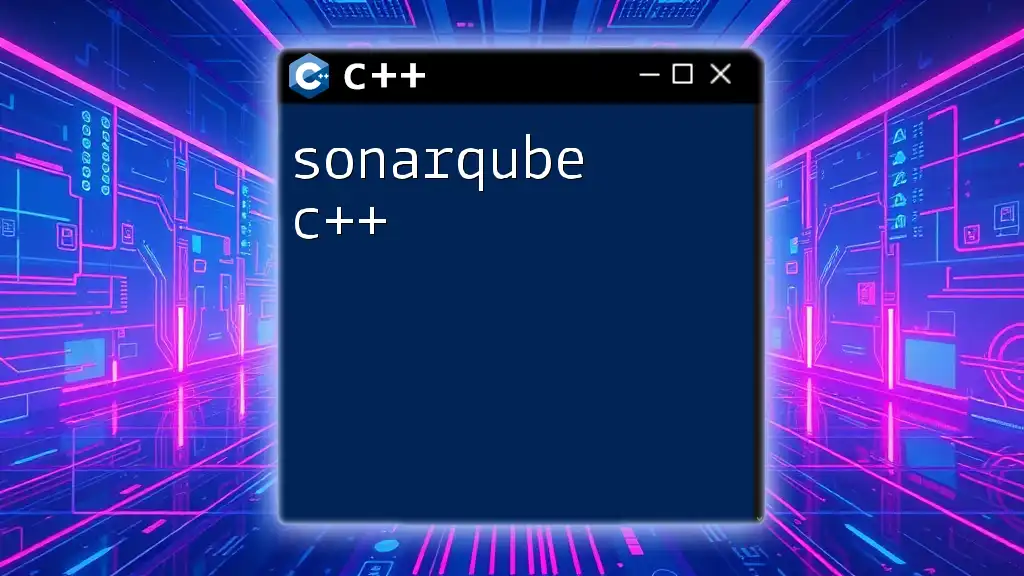
Additional Resources
For those looking to deepen their understanding, consider reviewing the official C++ documentation, exploring tutorial platforms, and engaging with community forums for support and resources.