Creating a simple game in C++ can be accomplished by setting up a basic structure that initializes game elements and handles user input for gameplay.
Here's a code snippet to illustrate a simple text-based guessing game:
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
std::srand(static_cast<unsigned>(std::time(0)); // Seed for random number generation
int randomNumber = std::rand() % 100 + 1; // Random number between 1 and 100
int guess;
std::cout << "Guess a number between 1 and 100: ";
while (std::cin >> guess) {
if (guess < randomNumber) {
std::cout << "Too low! Try again: ";
} else if (guess > randomNumber) {
std::cout << "Too high! Try again: ";
} else {
std::cout << "Congratulations! You guessed the number!\n";
break;
}
}
return 0;
}
Getting Started with C++ for Game Development
Setting Up Your Development Environment
To begin making a game in C++, you will first need to set up your development environment. This involves selecting an IDE (Integrated Development Environment) that suits your needs. Popular choices include Visual Studio and Code::Blocks, both of which provide user-friendly interfaces and powerful debugging tools.
Next, installing a C++ compiler is crucial. For Windows users, MinGW is a widely-used option that allows you to compile C++ code effortlessly. Follow the instructions to install MinGW, ensuring it’s properly added to your system’s PATH.
You will also need to incorporate essential libraries and frameworks that can significantly ease game development. Two notable libraries are SFML (Simple and Fast Multimedia Library) and SDL (Simple DirectMedia Layer). Both libraries offer robust functionalities for handling graphics, sound, and input.
Basics of C++
Before diving into game development, it’s essential to grasp the basic concepts of C++. Understanding C++ syntax and structure is a fundamental step. Here are some key points:
- Data types (int, float, char, etc.) and variables are the building blocks of storing information.
- Control structures such as if statements and loops (for, while) enable decision-making in your game logic.
Furthermore, an understanding of Object-Oriented Programming (OOP) is pivotal. OOP principles like encapsulation, inheritance, and polymorphism allow game designers to create modular and expandable systems. For instance, creating a base class for game objects can enable various types of game entities to inherit common properties and behaviors.
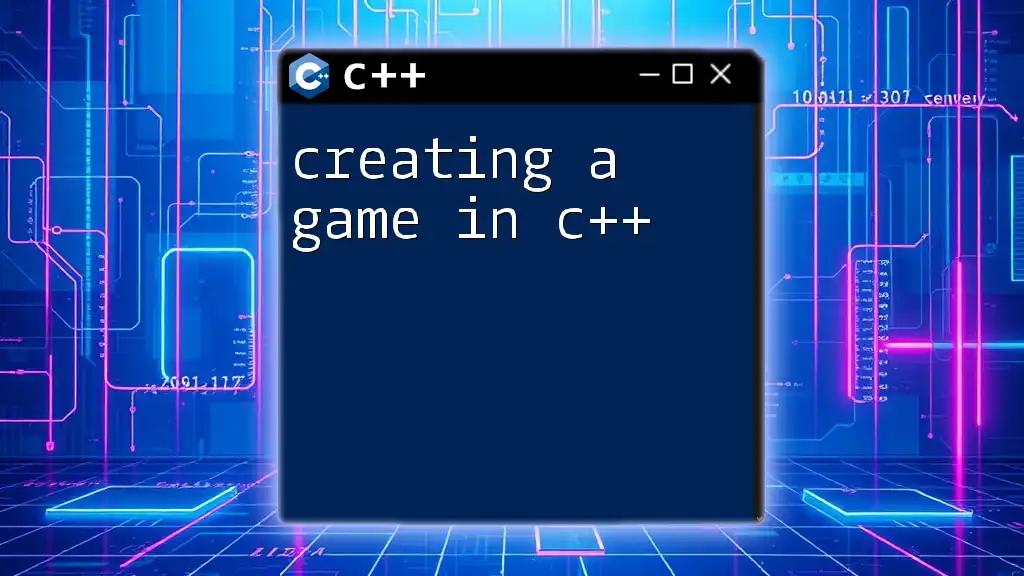
Designing Your Game
Conceptualizing Your Game Idea
The first step in making a game in C++ is to conceptualize your game idea. Consider the various game genres—action, adventure, puzzle, etc.—and brainstorm the core gameplay mechanics. What will make your game unique? Engaging storytelling and interesting characters can also enhance your game's appeal.
Creating Game Assets
Once you've defined your game concept, it’s time to gather your game assets. Game assets are essential components that bring your game to life and can include:
- Graphics (sprites, animations)
- Sound effects and music
- User interface elements
Utilize tools like Tiled for 2D level design and Audacity for sound editing to create or modify your assets.
Prototyping Your Game Idea
A prototype gives you a tangible representation of your game concept. It doesn’t have to be perfect; instead, it should demonstrate your core gameplay mechanics. Start simple—perhaps by creating a “Hello, World!” game that presents a text window. This approach allows you to manage the basic structure of your game without getting lost in complexity.
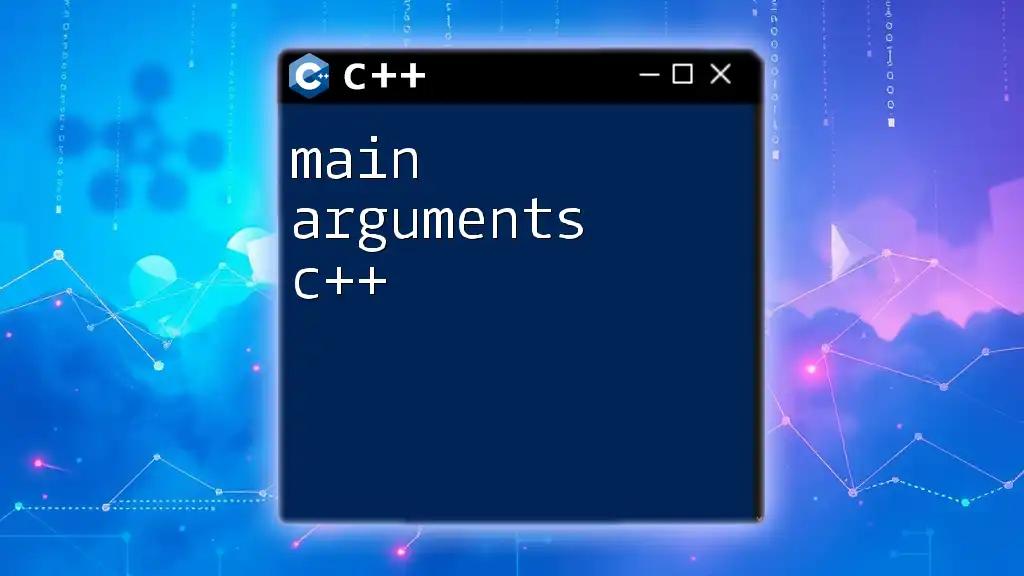
Structuring Your Game Code
Setting Up the Game Loop
Every game relies on a game loop, a fundamental structure driving the game logic and rendering process. The game loop continuously checks for user input, updates the game state, and redraws the game graphics.
A basic structure for your game loop might look like this:
while (isRunning) {
handleInput();
updateGame();
render();
}
This loop retains control over game progression, ensuring the integration of various processes in a synchronized manner.
Handling Game States
Game states help manage different phases of your game, such as menus, gameplay, pauses, and game over screens. By creating a state manager, you can easily switch between these states and manage the flow of your game effectively.
Using Classes for Game Objects
Leveraging classes allows for organized code and reusability. Define a game object class that encapsulates properties and behaviors. For instance, a class for the player might look like this:
class Player {
public:
void update();
void render();
private:
int x, y;
};
By defining properties (like position) and methods (update and render), you establish a clear framework for how each object in your game functions.
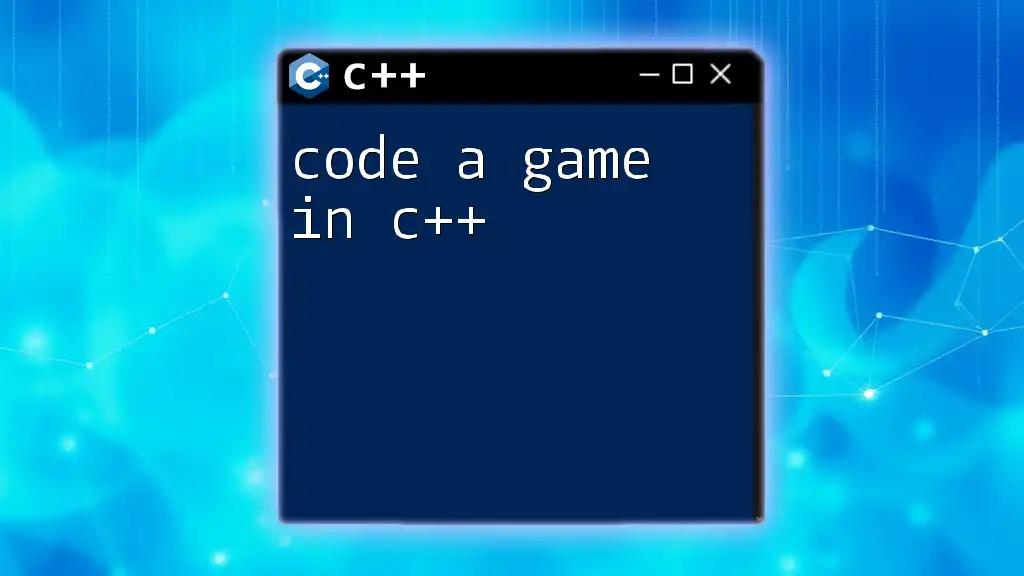
Putting It All Together
Integrating Graphics with SFML
To create an engaging visual experience, integrate SFML into your project. Start by initializing the game window and loading images.
Here's a simple example demonstrating how to display a sprite:
sf::Texture texture;
texture.loadFromFile("player.png");
sf::Sprite sprite(texture);
window.draw(sprite);
This code snippet shows how to load a texture from a file and draw it to the window, enabling a visual representation of your game objects.
Adding Sound Effects and Music
Incorporating sound effects and music can significantly enhance player immersion. SFML simplifies audio management. Load your sound files and utilize the `sf::Sound` and `sf::Music` classes to add auditory elements to your game:
sf::SoundBuffer buffer;
buffer.loadFromFile("sound.wav");
sf::Sound sound(buffer);
sound.play();
Implementing User Input
Responsive user input is critical for a smooth gameplay experience. Handle keyboard and mouse input seamlessly. For example, to move the player character using keyboard controls, you can implement the following code:
if (sf::Keyboard::isKeyPressed(sf::Keyboard::Left)) {
player.move(-1, 0);
}
This snippet detects if the left arrow key is pressed, moving the player character accordingly.

Testing and Debugging Your Game
Playtesting Techniques
Playtesting is an essential component of game development. It ensures that your game is enjoyable and functions as intended. Engage friends or fellow developers to collect constructive feedback that can guide adjustments and improvements to gameplay mechanics.
Common Bugs and Fixes
Debugging is part of the development process. While working on your game, you may encounter issues such as crashes or unexpected behaviors. Utilize tools like the Visual Studio Debugger or GDB to identify and resolve bugs effectively.
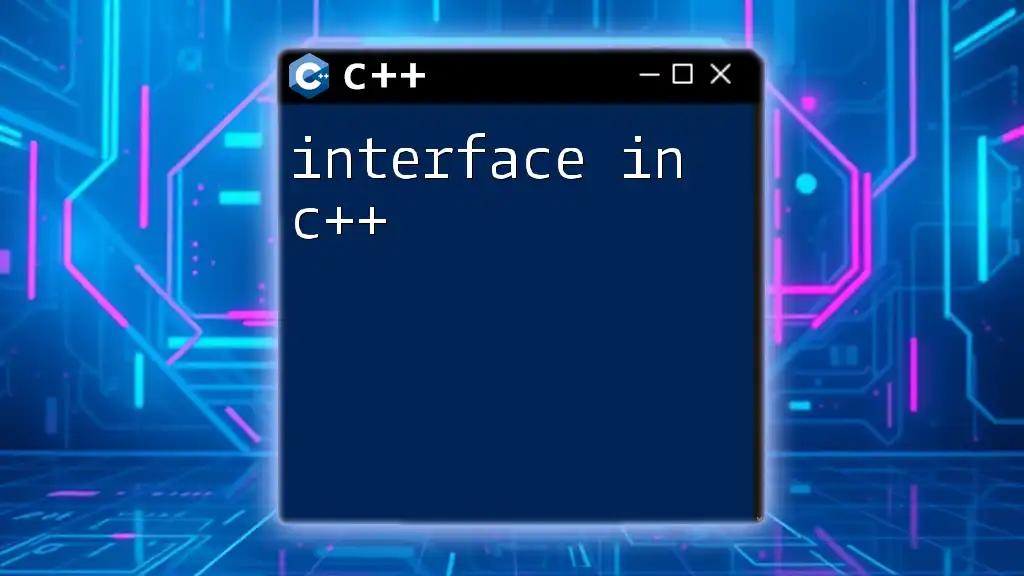
Finalizing Your Game
Optimizing Game Performance
When making a game in C++, performance optimization is crucial, especially if your game involves complex graphics or physics. Techniques such as reducing draw calls, optimizing memory usage, and using efficient algorithms can enhance your game’s performance while ensuring a smooth user experience.
Preparing for Release
Once your game reaches completion, prepare for release by building it for different platforms (PC, console, mobile). You may also want to implement basic marketing strategies, such as creating promotional materials and leveraging social media to attract potential players.
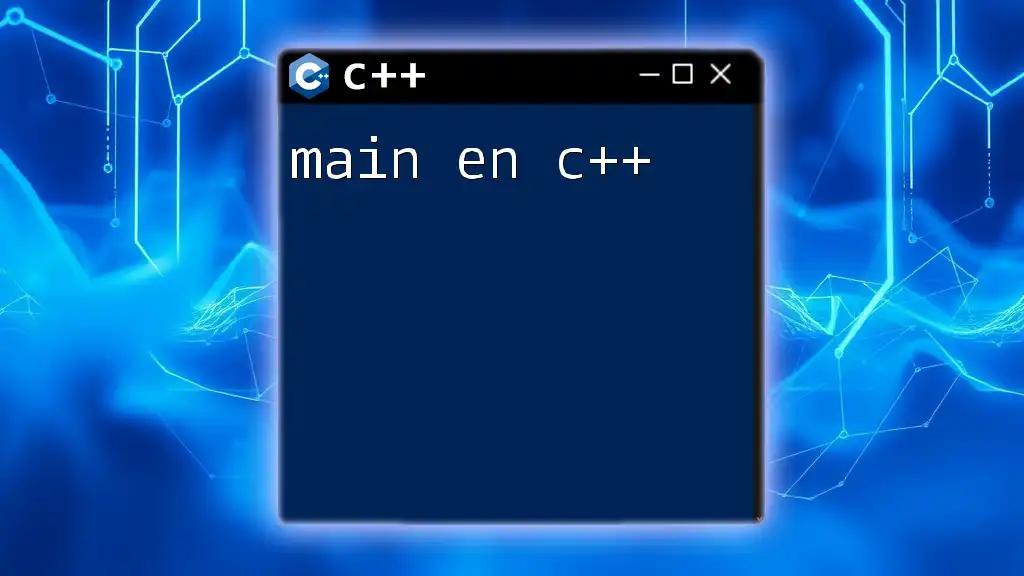
Conclusion
Recapping the journey of making a game in C++, it’s clear that the process involves distinct steps from setting up your development environment to finalizing your game for release. Continue to hone your skills and explore various resources to expand your knowledge in C++ game development. Remember, the key to success lies in creativity, practice, and community engagement to refine and perfect your craft.