In C++, the `int` range typically refers to the set of integer values that can be stored within the `int` data type, which is generally from -2,147,483,648 to 2,147,483,647 on a 32-bit system.
Here's a code snippet demonstrating how to display the minimum and maximum values of the `int` type using the `<limits>` header:
#include <iostream>
#include <limits>
int main() {
std::cout << "Int Min: " << std::numeric_limits<int>::min() << std::endl;
std::cout << "Int Max: " << std::numeric_limits<int>::max() << std::endl;
return 0;
}
Understanding the Range of Int in C++
What is an Int in C++?
In C++, the `int` data type represents an integer, which is a whole number that can be positive, negative, or zero. It is one of the most commonly used data types in programming, particularly for storing numeric data that doesn't require fractional components. Understanding the int range in C++ is essential because it directly affects how your program handles numerical values—particularly regarding memory usage and potential overflow issues.
Default Size and Range of Int
Standard Sizes of Int Data Type
The size of the `int` data type can vary depending on the platform and compiler. However, it is generally defined as:
- A standard `int` is typically 4 bytes (32 bits) on most systems.
- It may vary from 2 bytes (16 bits) to 8 bytes (64 bits) on different systems.
Range of Int in C++
The range of the `int` data type refers to the minimum and maximum values that an `int` can hold. In C++, you can easily find this range using the `<limits>` header.
To clarify, the range of int in C++ can be obtained as follows:
- Minimum value (`INT_MIN`): This is the smallest value an `int` can represent.
- Maximum value (`INT_MAX`): This is the largest value an `int` can represent.
Here’s a simple code snippet to display the int range:
#include <iostream>
#include <limits>
int main() {
std::cout << "The range of int:" << std::endl;
std::cout << "Minimum: " << std::numeric_limits<int>::min() << std::endl;
std::cout << "Maximum: " << std::numeric_limits<int>::max() << std::endl;
return 0;
}
When you run this code, you'll typically see the output:
The range of int:
Minimum: -2147483648
Maximum: 2147483647
This indicates that, for a standard 32-bit system, the int range is from -2,147,483,648 to 2,147,483,647.
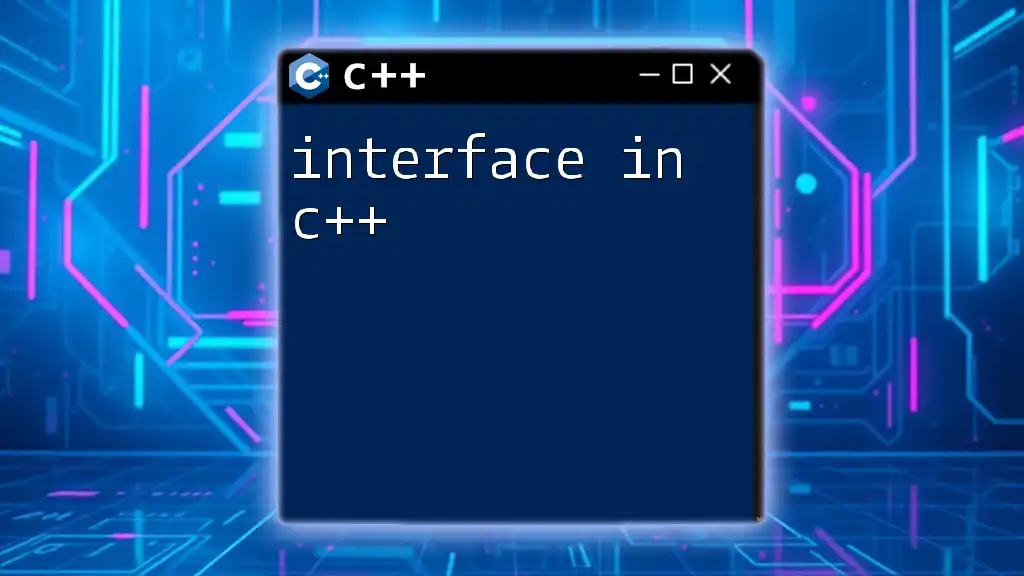
Variants of Integer Types in C++
Different Integer Types in C++
Beyond the standard `int`, C++ includes several variants of integer types to suit different needs. These include `short`, `long`, and `long long`, each with its own range and memory requirements. Knowing their ranges can help optimize your code for performance and memory usage.
Here’s a brief overview of these types:
- `short`: Typically 2 bytes (16 bits), with a range of -32,768 to 32,767.
- `long`: Typically 4 bytes (32 bits) or 8 bytes (64 bits), depending on the platform.
- `long long`: Typically 8 bytes (64 bits), allowing for much larger values.
You can use the following code to print out the ranges of these types:
#include <iostream>
#include <limits>
int main() {
std::cout << "Short: " << std::numeric_limits<short>::min() << " to " << std::numeric_limits<short>::max() << std::endl;
std::cout << "Int: " << std::numeric_limits<int>::min() << " to " << std::numeric_limits<int>::max() << std::endl;
std::cout << "Long: " << std::numeric_limits<long>::min() << " to " << std::numeric_limits<long>::max() << std::endl;
std::cout << "Long Long: " << std::numeric_limits<long long>::min() << " to " << std::numeric_limits<long long>::max() << std::endl;
return 0;
}
This allows you to compare ranges explicitly, ensuring that you choose the most appropriate type for your program's needs.
Signed vs. Unsigned Int
When using integer types in C++, it's essential to understand the difference between signed and unsigned integers.
- Signed integers can store both negative and positive values.
- Unsigned integers can only store non-negative values, with a range that starts at 0 and goes to a higher maximum value.
Here's a code snippet illustrating signed and unsigned int ranges:
#include <iostream>
#include <limits>
int main() {
std::cout << "Signed int range: " << std::numeric_limits<int>::min() << " to " << std::numeric_limits<int>::max() << std::endl;
std::cout << "Unsigned int range: " << 0 << " to " << std::numeric_limits<unsigned int>::max() << std::endl;
return 0;
}
Understanding these distinctions is crucial when designing programs that require specific numeric ranges, particularly in competitive or performance-sensitive coding scenarios.
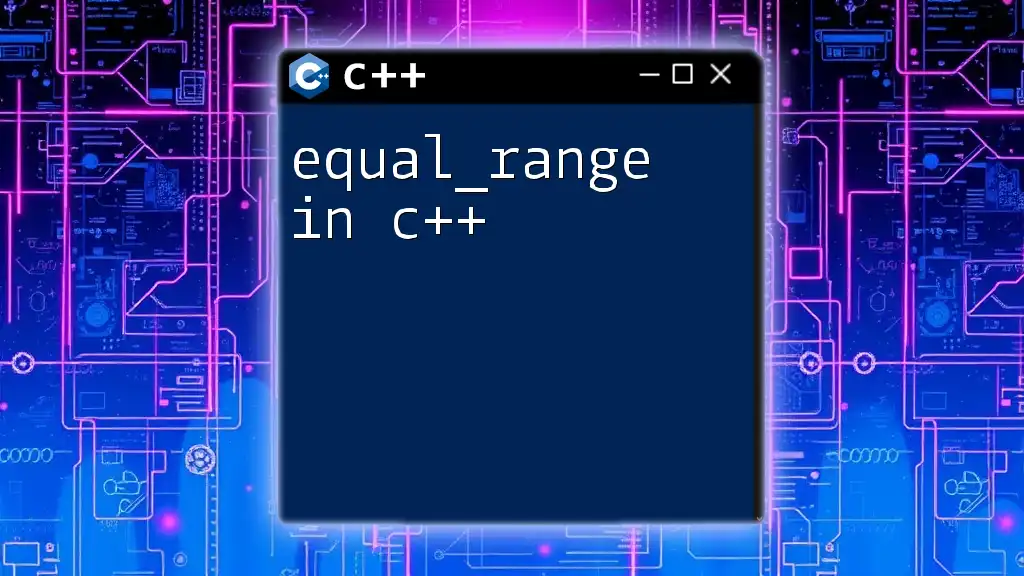
Practical Applications of Int Range
Choosing the Right Int Type
Selecting the correct integer type can significantly impact both performance and memory usage. When working on resource-constrained systems or performance-critical applications, using types like `short` or `char` for smaller values may yield better results. Conversely, if handling large numbers, consider using `long long`.
Overflow and Underflow in Integers
Overflow occurs when an operation attempts to create a numeric value that exceeds the data type's maximum limit. Conversely, underflow happens when a value falls below its minimum threshold.
For example, when you add 1 to `INT_MAX`, it loops back to `INT_MIN`:
#include <iostream>
#include <limits>
int main() {
int maxInt = std::numeric_limits<int>::max();
std::cout << "Max Int: " << maxInt << std::endl;
std::cout << "Overflow: " << (maxInt + 1) << std::endl; // Exhibit overflow behavior
return 0;
}
In most programming scenarios, overflow and underflow can lead to unexpected behavior and bugs, making it essential to understand these concepts and plan for them in your programming logic.
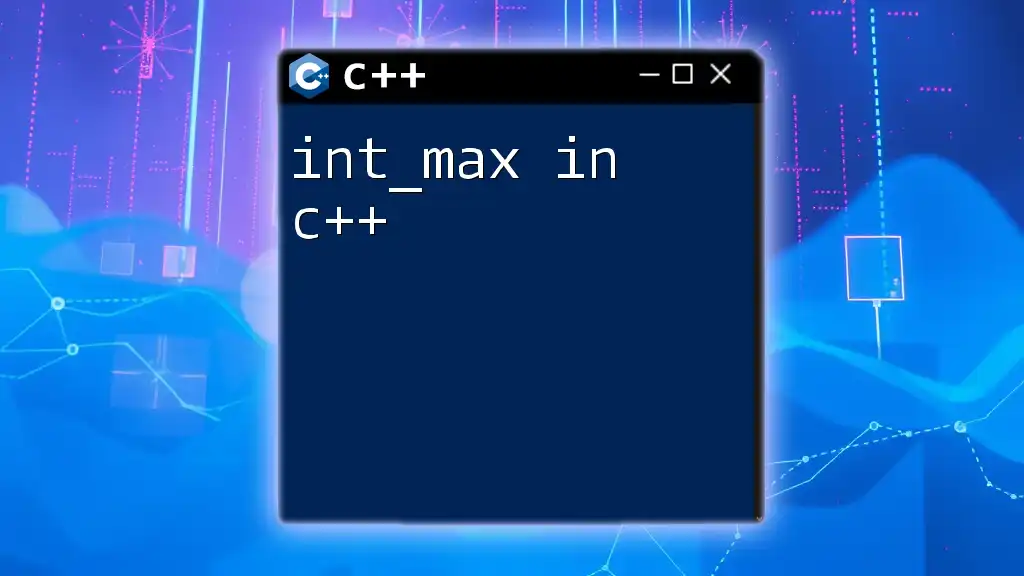
Best Practices for Working with Int in C++
Effective Variable Declaration
When declaring integer variables, follow best practices to ensure clarity in your code. Use descriptive variable names and avoid using types that are unnecessarily large. For example, use `short count` instead of `long count` if the range accommodates your needs.
Error Handling Techniques
Having robust error handling mechanisms is crucial. Use assertions to catch overflow situations where feasible, or implement exception handling in parts of your code that might lead to out-of-range errors. C++ does not inherently throw exceptions for integer overflows, so be proactive.
Performance Considerations
Finally, the size of your integer type can significantly affect your program's performance. Smaller types may consume less memory, enabling you to utilize cache more effectively and improve performance in large arrays or data sets.
![Understanding Literals in C++ [A Quick Guide]](/images/posts/l/literals-in-cpp.webp)
Conclusion
Understanding the int range in C++ is crucial for effectively utilizing this fundamental data type in your programming projects. By grasping the implications of choosing between signed and unsigned integers, exploring variants like `short` and `long`, and recognizing overflow and underflow scenarios, you position yourself to write cleaner, more efficient code.
From the nuances of the int range in C++ to practical applications and best practices, mastering these topics can elevate your programming skills and enhance your overall coding proficiency. As you continue to practice, remember that the choices you make in terms of data types significantly impact your code's functionality and performance.