In C++, an interface is typically defined using an abstract class that contains pure virtual functions, which must be implemented by derived classes.
Here’s a simple example:
class MyInterface {
public:
virtual void functionA() = 0; // Pure virtual function
virtual void functionB() = 0; // Pure virtual function
};
class MyClass : public MyInterface {
public:
void functionA() override {
// Implementation of functionA
}
void functionB() override {
// Implementation of functionB
}
};
Understanding Interfaces in C++
What is an Interface?
An interface in programming defines a contract that classes can implement, specifying which methods must be provided without defining how they should be executed. This concept is integral to object-oriented programming and serves to promote encapsulation and loose coupling.
Interfaces differ from abstract classes primarily in that all methods in an interface are pure virtual functions, which means they do not have any implementation. In contrast, abstract classes can contain both virtual and non-virtual methods, allowing for some shared implementation.
Why Use Interfaces?
Using interfaces comes with several advantages:
-
Encapsulation: Interfaces provide a way to hide the implementation details, focusing only on the behavior.
-
Decoupling: Interfaces allow different parts of a system to communicate without being tightly coupled to each other. This is crucial for maintaining and extending applications.
-
Flexibility: By defining interfaces, developers can create multiple implementations that can be swapped out without affecting other parts of the program.
![Understanding Literals in C++ [A Quick Guide]](/_next/image?url=%2Fimages%2Fposts%2Fl%2Fliterals-in-cpp.webp&w=1080&q=75)
Creating Interfaces in C++
Defining an Interface in C++
In C++, you can define an interface by creating a class that contains only pure virtual functions. Here’s the syntax for defining an interface:
class IShape {
public:
virtual double area() const = 0; // Pure virtual function
virtual void draw() const = 0; // Another pure virtual function
virtual ~IShape() {} // Virtual destructor
};
In this code snippet, `IShape` serves as an interface with two pure virtual functions: `area()` for calculating the shape's area and `draw()` for rendering the shape.
Implementing Interfaces
When a class implements an interface, it inherits from the interface class and provides concrete definitions for all the pure virtual functions. Below is an example of how you could implement an interface called `IShape` in two classes, `Circle` and `Rectangle`.
#include <iostream>
#include <cmath>
class Circle : public IShape {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double area() const override {
return M_PI * radius * radius;
}
void draw() const override {
std::cout << "Drawing a Circle\n";
}
};
class Rectangle : public IShape {
private:
double width, height;
public:
Rectangle(double w, double h) : width(w), height(h) {}
double area() const override {
return width * height;
}
void draw() const override {
std::cout << "Drawing a Rectangle\n";
}
};
In this example, both `Circle` and `Rectangle` classes have their own implementations of the `area()` and `draw()` methods, demonstrating how different shapes can adhere to the same interface while maintaining distinct behaviors.
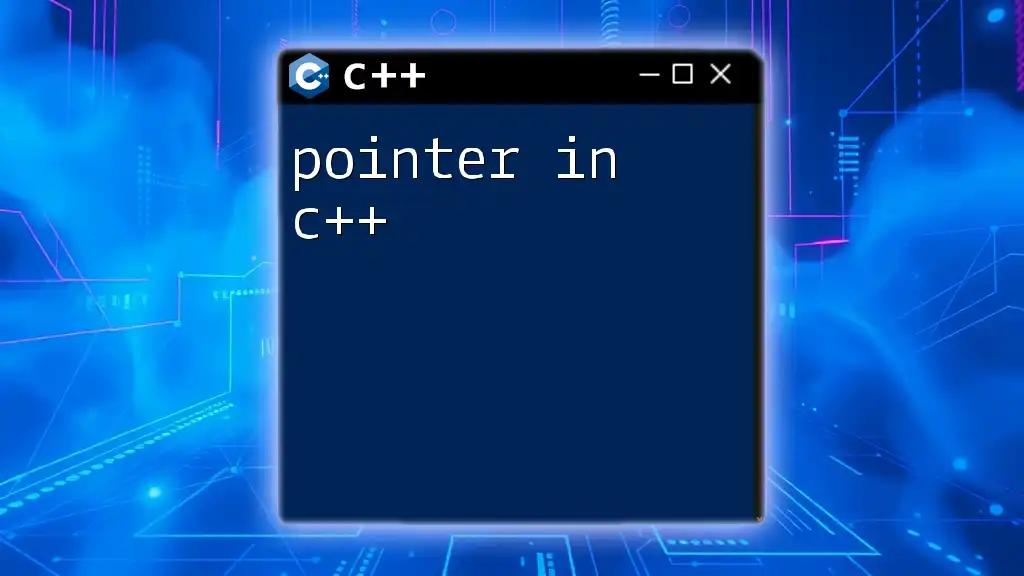
Using Interfaces in Practice
Polymorphism with Interfaces
One of the standout features of interfaces in C++ is polymorphism. You can use pointers or references to interface types to invoke the appropriate method based on the actual object type at runtime. Consider the following function that calculates and prints the area of any shape implementing the `IShape` interface:
void printShapeArea(const IShape& shape) {
std::cout << "Area: " << shape.area() << std::endl;
}
Example Use Case
Here’s how you would use the previously defined classes in a practical scenario. This code snippet demonstrates creating a `Circle` and a `Rectangle`, and then using the `printShapeArea` function to output their areas:
int main() {
Circle circle(5.0);
Rectangle rectangle(4.0, 6.0);
printShapeArea(circle);
printShapeArea(rectangle);
circle.draw();
rectangle.draw();
return 0;
}
This showcases how you can easily work with multiple implementations of the same interface, making your code scalable and flexible.
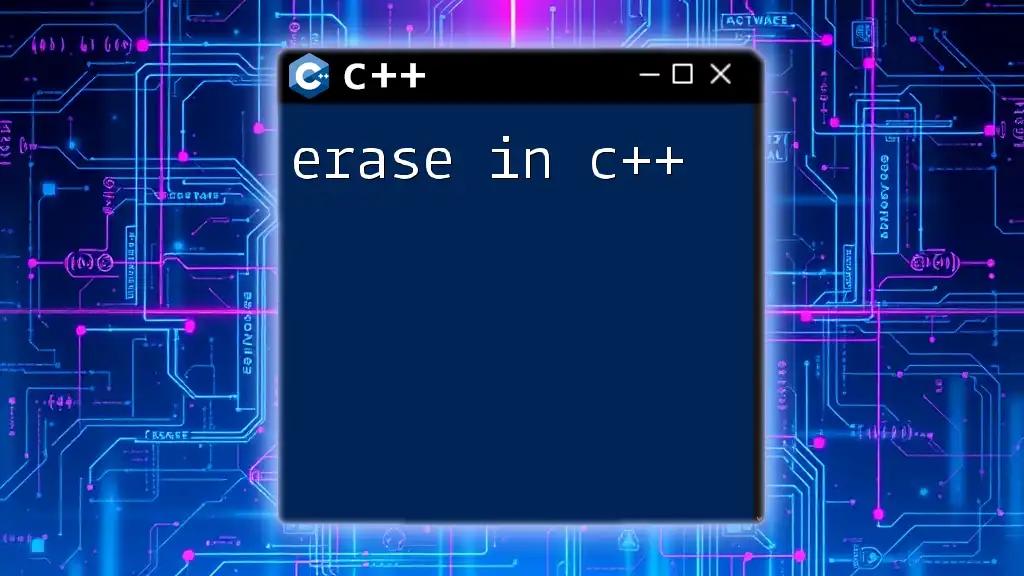
Advanced Concepts
Multiple Inheritance and Interfaces
C++ allows for multiple inheritance, meaning a class can implement multiple interfaces. This capability enhances flexibility but also places the burden of maintaining clarity in design on the developer. Here’s an example where a class `ColorfulCircle` implements both `Circle` and another interface, `IColor`:
class IColor {
public:
virtual void color() const = 0;
};
class ColorfulCircle : public Circle, public IColor {
public:
ColorfulCircle(double r) : Circle(r) {}
void color() const override {
std::cout << "Coloring Circle: Red\n";
}
};
In this instance, `ColorfulCircle` not only has the characteristics of a `Circle` but also can be painted by implementing the `color()` method.
Interface Segregation Principle
The Interface Segregation Principle (ISP) states that no client should be forced to depend on methods it does not use. This principle is particularly vital when creating interfaces. It encourages the design of smaller, more focused interfaces rather than monolithic ones, improving maintainability and usability.

Best Practices for Designing Interfaces
Defining Clear Interfaces
A clean interface is an effective one. Here are guidelines for designing interfaces:
-
Keep them simple. Each interface should encapsulate a specific behavior or aspect of functionality without spilling over into other areas.
-
Ensure that each method within the interface is necessary and provides meaningful functionality.
Documenting Interfaces
Documentation is essential when it comes to interfaces. Clear documentation can vastly improve user adoption and understanding. Consider including:
-
Usage examples of the interface and its methods.
-
Clarifications on expected behaviors and possible exceptions.
-
Information on when and how to implement various methods.
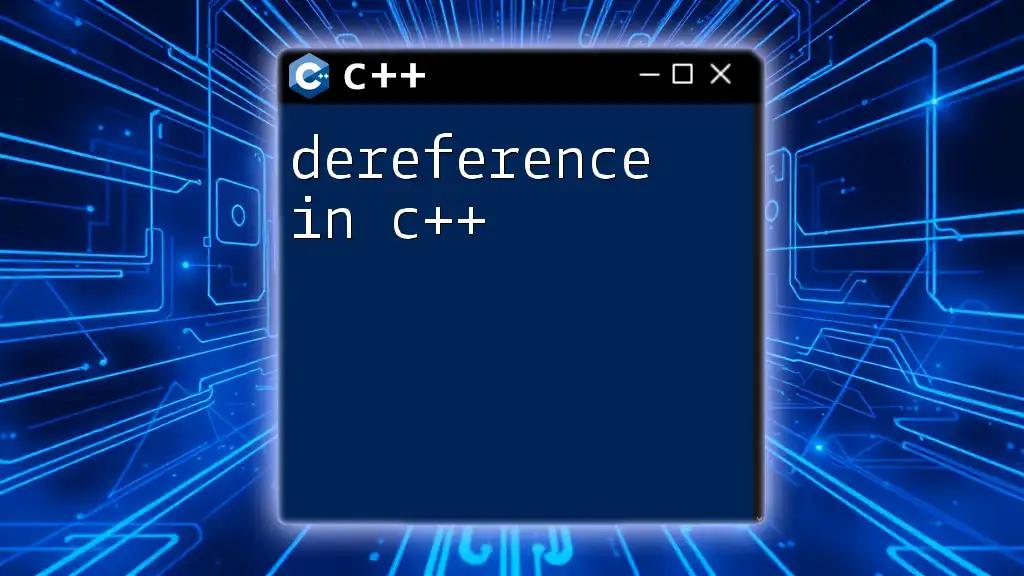
Conclusion
In this comprehensive overview of interfaces in C++, we delved into what they are, how to define and implement them, and their advantages in software design. Understanding the nuances of interfaces not only aids in writing cleaner, more maintainable code but also adheres to principles of object-oriented design that enhance collaboration and flexibility. For further learning, consider exploring resources such as books, courses, and interactive tutorials that dive deeper into the powerful capabilities of interfaces and C++.