The `inserter` in C++ is a part of the Standard Template Library (STL) that allows you to insert elements into a container at a specific position using an iterator.
Here's a code snippet demonstrating how to use `inserter` with a `std::vector`:
#include <iostream>
#include <vector>
#include <iterator>
int main() {
std::vector<int> vec = {1, 2, 3, 6, 7};
std::vector<int>::iterator it = vec.begin() + 3;
std::vector<int> toInsert = {4, 5};
std::copy(toInsert.begin(), toInsert.end(), std::inserter(vec, it));
for (int num : vec) {
std::cout << num << " ";
}
return 0;
}
In this example, the values 4 and 5 are inserted into the `vec` before the element 6.
What is an Inserter?
Definition
Inserters in C++ are special types of output iterators that facilitate the addition of elements to collections. They enable the insertion of elements in a streamlined manner, thereby improving the efficiency of data manipulation in various STL containers. Understanding the conceptual role of inserters will allow developers to leverage them effectively in their applications.
Types of Inserters
There are three primary types of inserters in C++:
-
Back Insert Iterator: This iterator appends elements to the end of a container. It's widely used when you want to add items to the back of dynamic arrays or lists.
-
Front Insert Iterator: This iterator allows elements to be prepended to the front of a container. This can be useful when the order of elements needs to be prioritized, such as in stacks.
-
Insert Iterator: The insert iterator is used for inserting elements at a specific location within a container, allowing for more flexible placement of items.
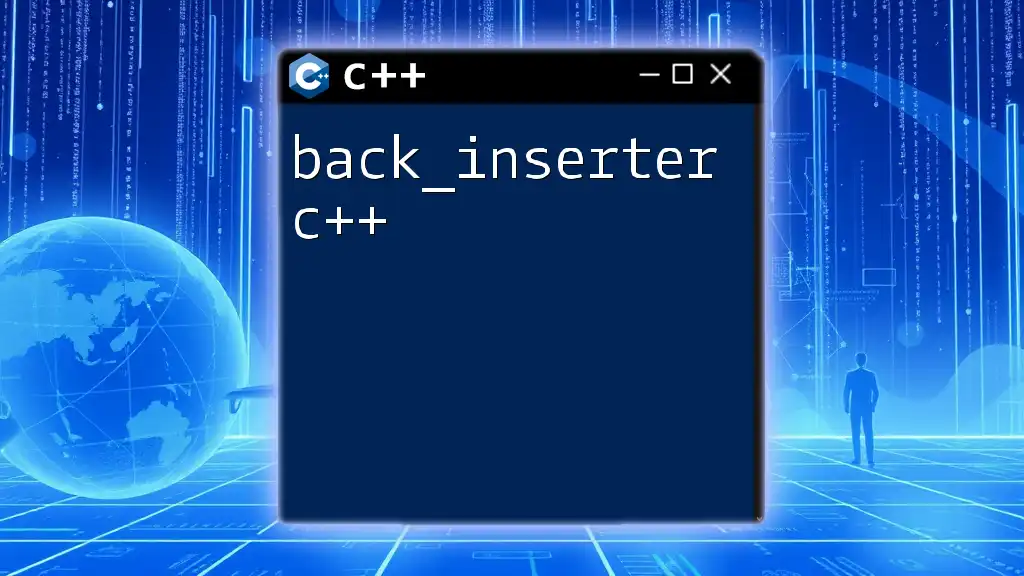
Working with Inserters
How Inserters Function
Inserters essentially wrap the functionality of iterators, allowing them to perform insertion operations. This means that they modify the content of the container they are associated with, as opposed to simply reading values. Inserters interact primarily with STL collections, which take advantage of their inherent capabilities to handle dynamic memory efficiently.
In using inserters, one must consider certain limitations. For example, certain iterators are specialized for certain kinds of containers. Using a back insert iterator with a container that does not support appending naturally, like a `set`, will lead to unexpected behavior or compilation errors.
Using Inserters with STL Containers
std::vector
To demonstrate how inserters work, consider the following code snippet utilizing a back insert iterator with `std::vector`:
#include <iostream>
#include <vector>
#include <iterator>
int main() {
std::vector<int> vec;
std::back_insert_iterator<std::vector<int>> back_it(vec);
*back_it = 10;
*back_it = 20;
for (const auto &val : vec) {
std::cout << val << " "; // Outputs: 10 20
}
return 0;
}
In this example, the `back_insert_iterator` enables us to easily append values `10` and `20` to the vector `vec`. After the insertions, when iterating over `vec`, we can see the newly added elements. The advantage of using a back inserter lies in its simplicity and readability, allowing for a clean way to build up a sequence dynamically.
std::list
Now, let us look at the `std::list` and see how a front insert iterator works:
#include <iostream>
#include <list>
#include <iterator>
int main() {
std::list<int> lst;
std::front_insert_iterator<std::list<int>> front_it(lst);
*front_it = 30;
*front_it = 40;
for (const auto &val : lst) {
std::cout << val << " "; // Outputs: 40 30
}
return 0;
}
In this example, we employ a `front_insert_iterator` to add elements `30` and `40` to the front of the list `lst`. Upon iterating through the list, the output reflects the LIFO (Last In, First Out) nature of lists using this iterator. The use case for a front inserter is particularly beneficial when the order of insertion is critical and allows you to add elements without needing to rearrange existing items.
std::set
Finally, let’s discuss how to use an insert iterator with a `std::set`:
#include <iostream>
#include <set>
#include <iterator>
int main() {
std::set<int> myset;
std::insert_iterator<std::set<int>> ins_it(myset, myset.begin());
*ins_it = 15;
*ins_it = 5;
for (const auto &val : myset) {
std::cout << val << " "; // Outputs: 5 15
}
return 0;
}
The above code shows how we can insert the numbers `15` and `5` at the beginning of the set `myset`. Because a `set` inherently disallows duplicates and maintains order, we see the values automatically sorted during output. Using an insert iterator is particularly advantageous in this scenario, allowing for easy indexing while maintaining the integrity of set rules.
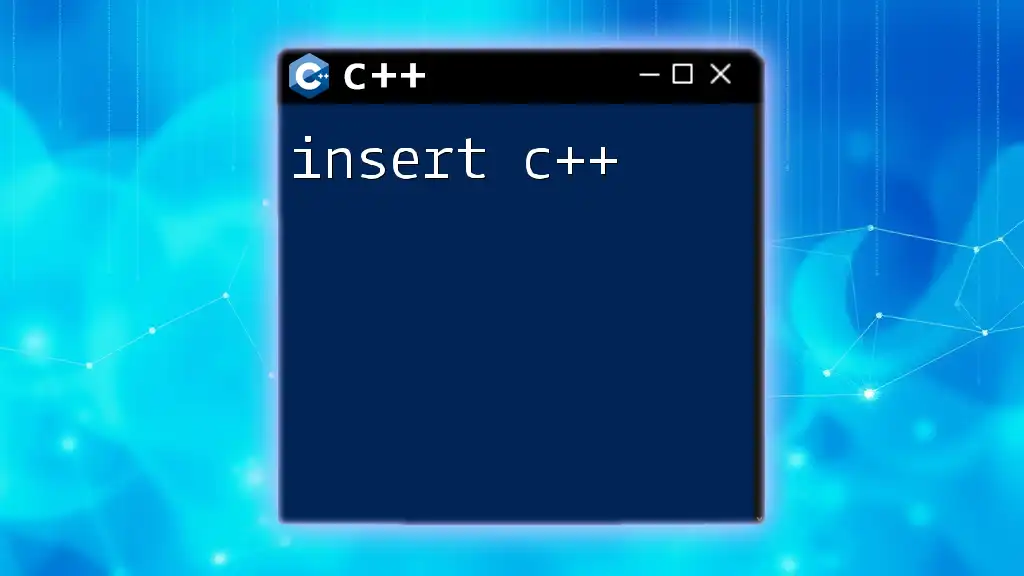
Comparisons and Performance
Performance Analysis of Different Inserters
Inserters, by design, are streamlined for usage with their respective containers. For instance, back insert iterators generally work with `O(1)` complexity for `std::vector`, while using a front insert iterator in a `std::list` maintains constant time complexity as well. However, keep in mind that the efficiency of these operations hinges on the underlying container's attributes; for instance, inserting into a `std::deque` might result in different performance characteristics.
Common Pitfalls
While working with inserters, developers might encounter some pitfalls, such as:
- Dereferencing Iterators on Empty Containers: Attempting to insert into an empty container without proper initialization can lead to dereferencing errors.
- Confusion between Insert and Back/Front Insert Iterators: Misusing an insert iterator on a container that only supports back or front inserting can lead to exceptions and complications in code.
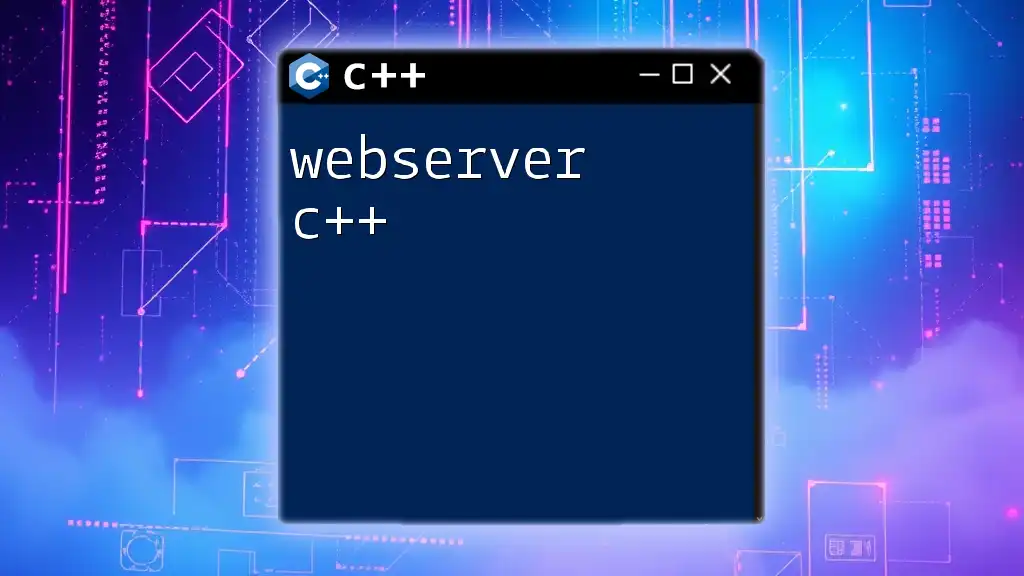
Best Practices for Using Inserters
To effectively use inserters:
- Always ensure the appropriate inserter type is matched with the container you are manipulating.
- Consider the container’s characteristics and choose the inserter that aligns with your intended operations.
- Write clear and maintainable code by encapsulating inserter operations within loops or functions.
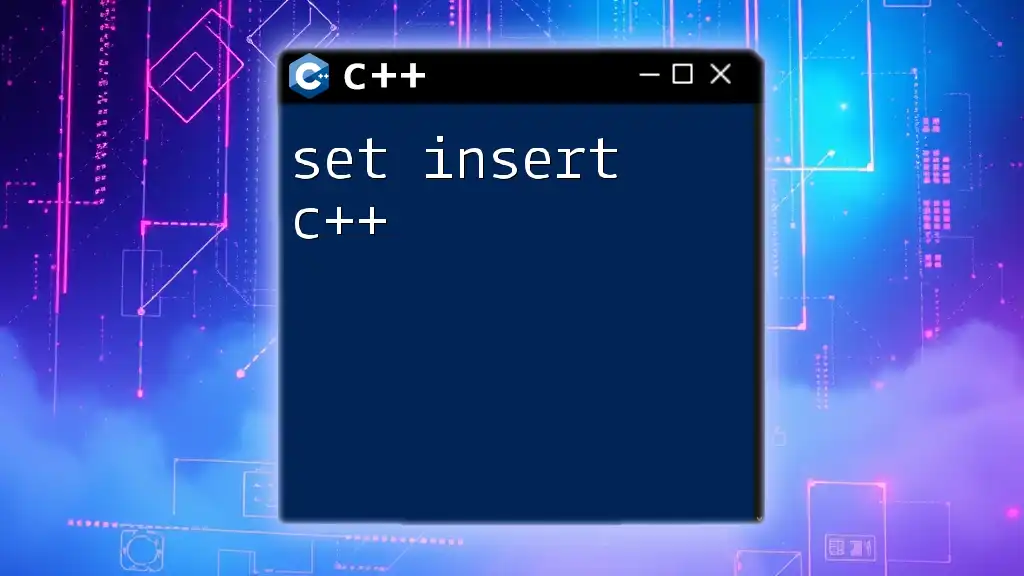
Conclusion
Understanding how to utilize inserters in C++ can greatly enhance your ability to work with collections, making data manipulation both efficient and straightforward. This guide serves to familiarize you with the capabilities of inserters across various STL containers, inspiring you to implement these practices in your future projects.
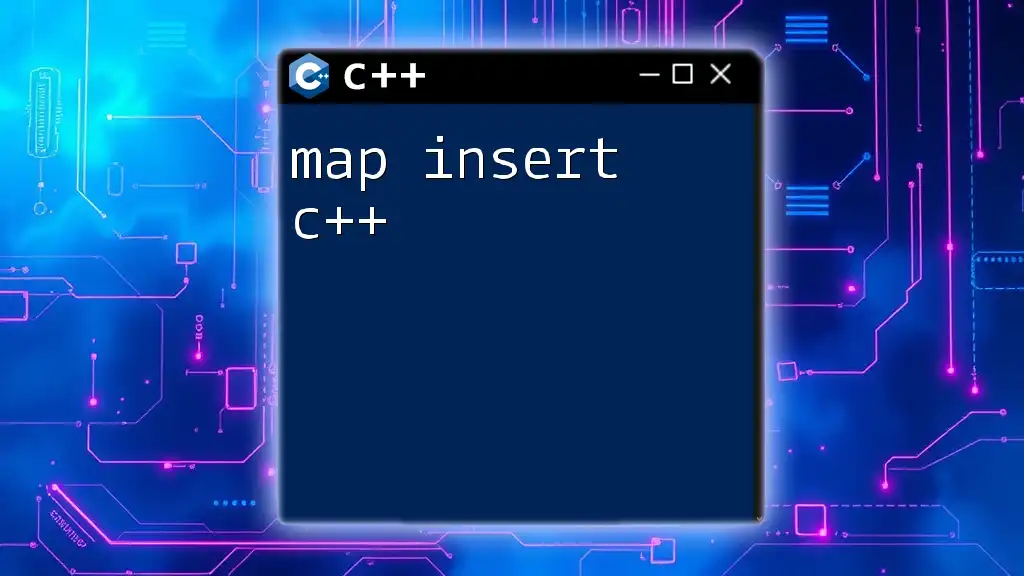
Additional Resources
For further exploration of inserters and their applications, consider consulting the C++ standard documentation, reputable coding tutorials, or engaging in community forums. These resources will provide not only theoretical underpinnings but also practical examples that expand your understanding of C++.
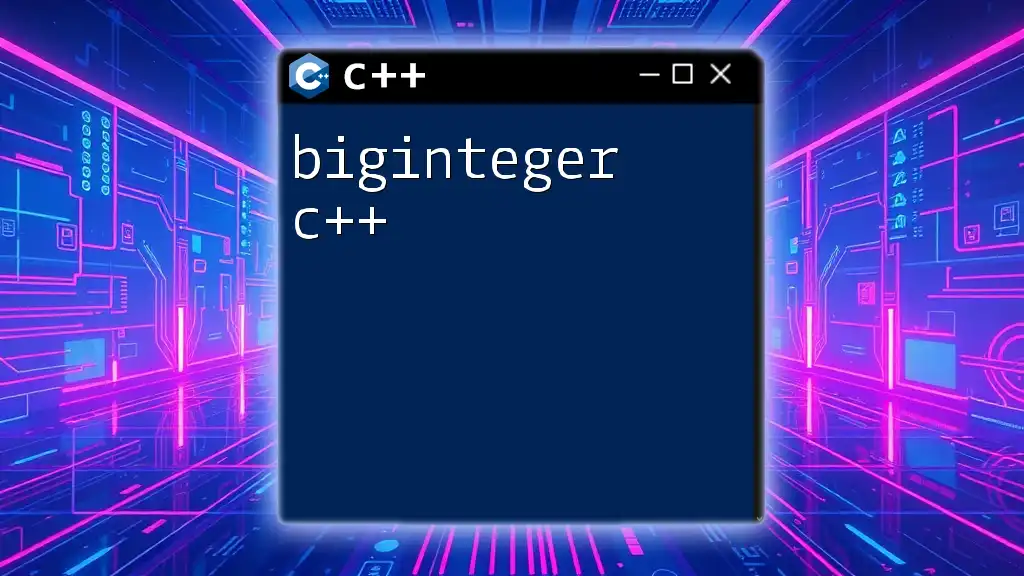
Call to Action
Have you experienced any challenges while using inserters in your coding journey? Share your thoughts, insights, or questions in the comments section below!