The `qsort` function in C++ is a standard library function that sorts an array of elements using the quicksort algorithm, which is implemented in the `<cstdlib>` header.
Here’s a simple example of using `qsort`:
#include <iostream>
#include <cstdlib>
int compare(const void* a, const void* b) {
return (*(int*)a - *(int*)b);
}
int main() {
int arr[] = {5, 2, 9, 1, 5, 6};
size_t arrSize = sizeof(arr) / sizeof(arr[0]);
qsort(arr, arrSize, sizeof(int), compare);
for (size_t i = 0; i < arrSize; i++) {
std::cout << arr[i] << " ";
}
return 0;
}
Understanding the qsort Function
What is qsort?
`qsort` is a standard library function in C and C++ that provides a quick and efficient way to sort arrays. Originating from the C Standard Library, its name comes from "quick sort," the underlying algorithm it implements. This function is part of the `cstdlib` header file in C++, which allows developers to easily sort different types of data with minimal effort.
Key Features of qsort
One of the most significant features of `qsort` is its generic functionality. It can sort arrays containing various data types, such as integers, doubles, or even user-defined structures. This versatility is what makes `qsort` an essential function in any C++ programmer's toolkit.
Another prominent attribute is its reliance on function pointers. This allows you to define custom comparison logic, thus enabling the sorting of complex data types just as easily as primitive types. It provides flexibility and modularity by allowing you to plug in any comparison function as needed.
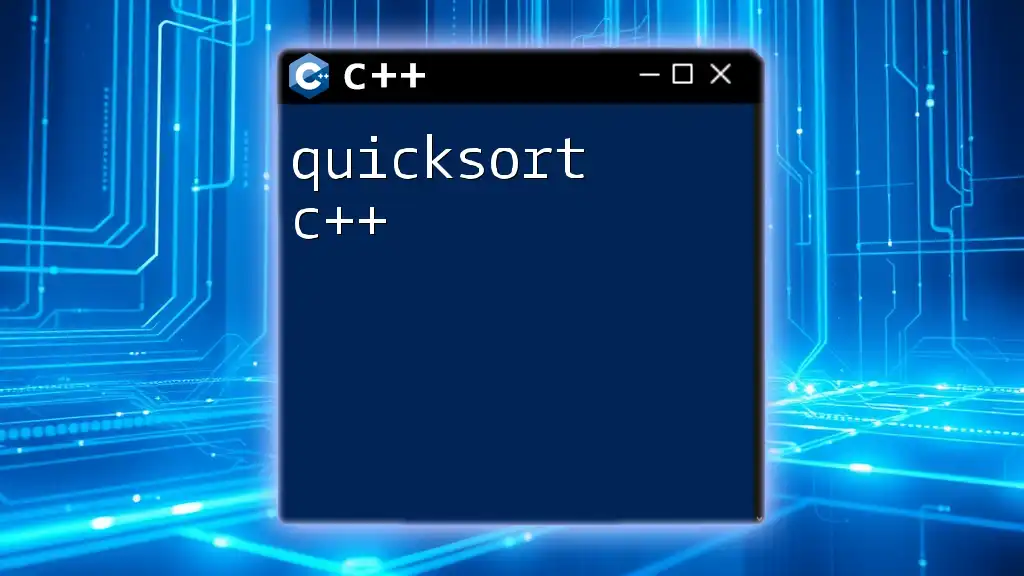
How qsort Works
Function Prototype
The prototype of the `qsort` function is as follows:
void qsort(void *base, size_t num, size_t size, int (*compar)(const void *, const void *));
Let’s break down the parameters:
- Base Pointer: This is a pointer to the array you wish to sort. As it is a void pointer, it can point to any data type.
- Number of Elements: This parameter indicates how many elements are in the array. It must be accurate for `qsort` to function correctly.
- Size of Each Element: This tells the function the size of each individual element in bytes. This is crucial for `qsort` to correctly read and manipulate the data.
- Comparison Function: A function pointer, which is defined by the user, that tells `qsort` how to compare two elements. The function is expected to return a positive value if the first element is greater than the second, a negative value if it is lesser, or zero if they are equal.
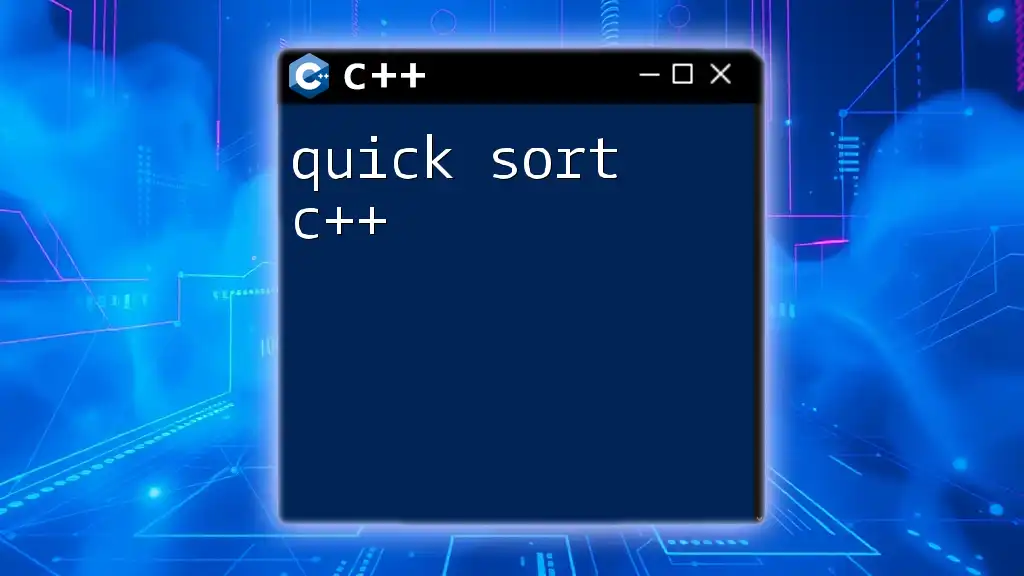
Implementing qsort in C++
Setting Up Your C++ Development Environment
Before diving into coding, ensure you’re working in the right C++ environment. Choose an IDE or editor that you’re comfortable with. Popular choices include Visual Studio, Code::Blocks, and Eclipse. Make sure your compiler supports C++ standards to avoid any compatibility issues.
Example Code: Sorting an Array of Integers
Here's a practical example demonstrating how to sort an array of integers using `qsort`:
#include <iostream>
#include <cstdlib> // For qsort
int compare(const void *a, const void *b) {
return (*(int*)a - *(int*)b);
}
int main() {
int arr[] = {5, 3, 2, 8, 6};
int n = sizeof(arr) / sizeof(arr[0]);
qsort(arr, n, sizeof(int), compare);
for (int i = 0; i < n; i++) {
std::cout << arr[i] << " ";
}
return 0;
}
Let's break down the code:
- Header Files: The `cstdlib` header is included for using the `qsort` function.
- Comparison Function: The `compare` function takes two `void` pointers, casts them to `int` pointers, and returns the difference between the two. This logic sorts integers in ascending order.
- Main Function: An integer array is defined and populated. The number of elements is calculated, then `qsort` is called, passing the array, the number of elements, the size of an integer, and the comparison function.
- Output: Finally, a loop iterates through the sorted array to print its contents.
Example Code: Sorting an Array of Strings
Next, let’s see how to sort an array of strings:
#include <iostream>
#include <cstdlib> // For qsort
#include <cstring> // For strcmp
int compareStrings(const void *a, const void *b) {
return strcmp(*(const char**)a, *(const char**)b);
}
int main() {
const char *arr[] = {"apple", "orange", "banana", "grape"};
int n = sizeof(arr) / sizeof(arr[0]);
qsort(arr, n, sizeof(const char*), compareStrings);
for (int i = 0; i < n; i++) {
std::cout << arr[i] << " ";
}
return 0;
}
The breakdown of this code is as follows:
- Header Files: Besides `cstdlib`, we include `cstring` for string comparison.
- Comparison Function: `compareStrings` uses `strcmp` to compare two strings, allowing `qsort` to sort them lexicographically.
- Main Function: We define an array of string literals, calculate its size, and call `qsort` similarly to the previous example, passing the string array and the string comparison function.
- Output: The sorted strings are printed to the console.
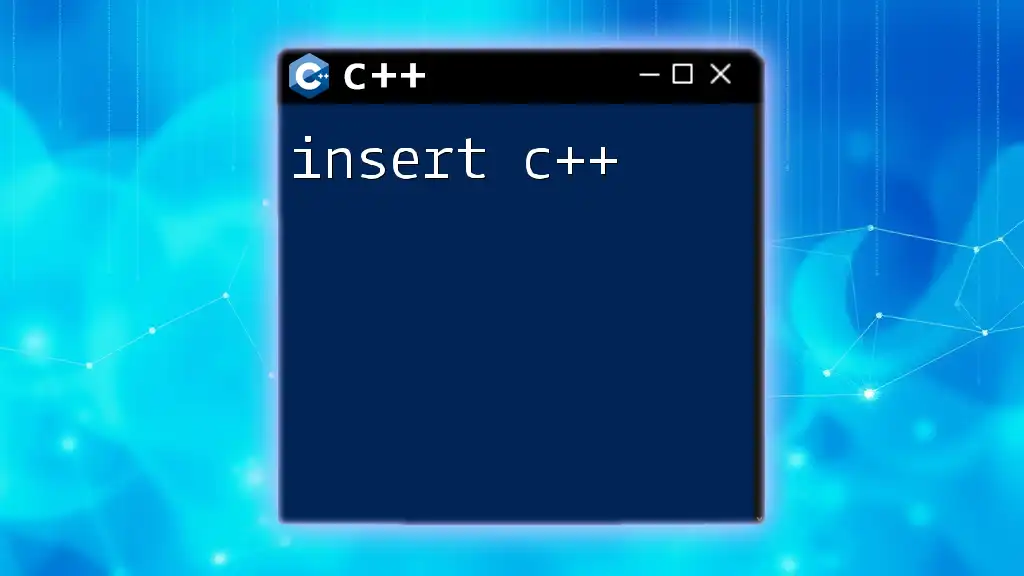
Tips and Best Practices for Using qsort
Comparison Function Guidelines
When crafting a comparison function for `qsort`, adhere to the proper return values—positive for greater, negative for lesser, and zero for equal. A well-structured comparison function ensures consistent sorting behavior.
Performance Considerations
It's crucial to understand that `qsort` has an average time complexity of O(n log n), making it efficient for most scenarios. However, its worst-case scenario can degrade to O(n²), particularly if the pivot selections are poor.
When Not to Use qsort
While `qsort` can handle various data types, it is not always the optimal choice. For instance, if you're using C++, consider using the `std::sort` function available in `<algorithm>`, which is often faster due to optimizations and typically a better choice for C++ containers like `std::vector`.
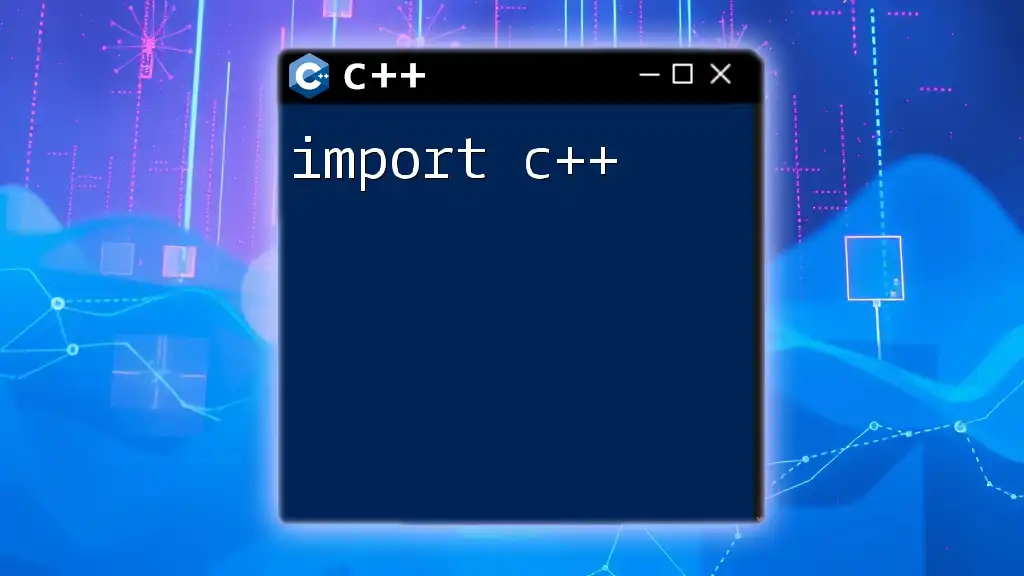
Debugging Common Issues with qsort
Typical Errors and Solutions
One common issue developers face is segmentation faults. This usually stems from incorrect size specifications or mismanagement of pointers within the comparison function. Ensure you understand the data being pointed to and the respective sizes.
Tips for Effective Debugging
Logging can significantly simplify debugging efforts. Introduce print statements in your comparison functions to track values being compared. Compiling your code in debug mode with warnings enabled can also help highlight potential issues before runtime.
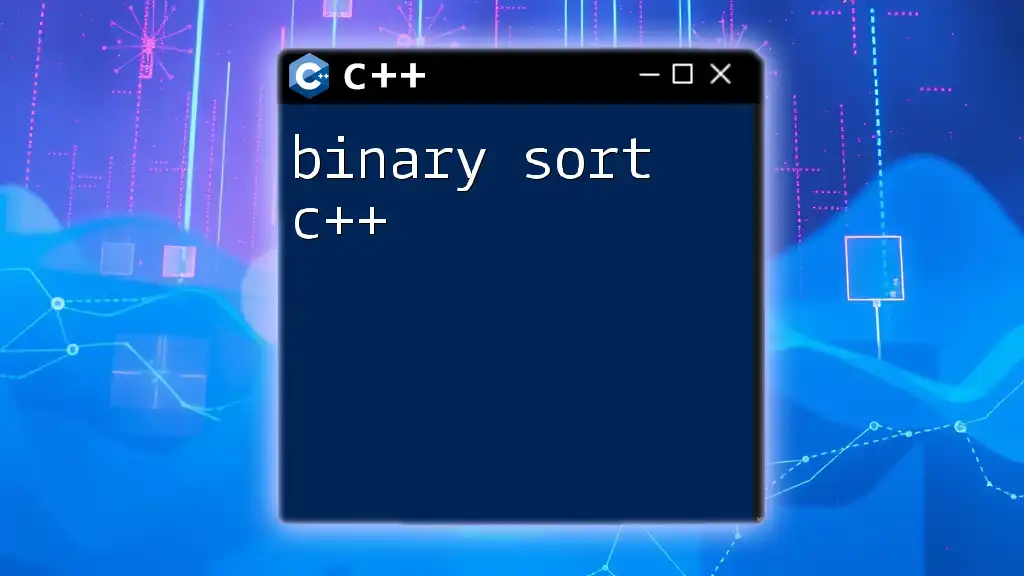
Conclusion
In summary, the `qsort` function in C++ is a powerful tool for sorting arrays of diverse data types using custom comparison logic. Understanding its parameters and proper implementation is crucial for optimizing performance and ensuring correctness. Experimenting with `qsort` provides valuable insights into sorting algorithms and can enhance your programming skills. Try out different data types and comparison strategies to fully grasp the flexibility this function offers!
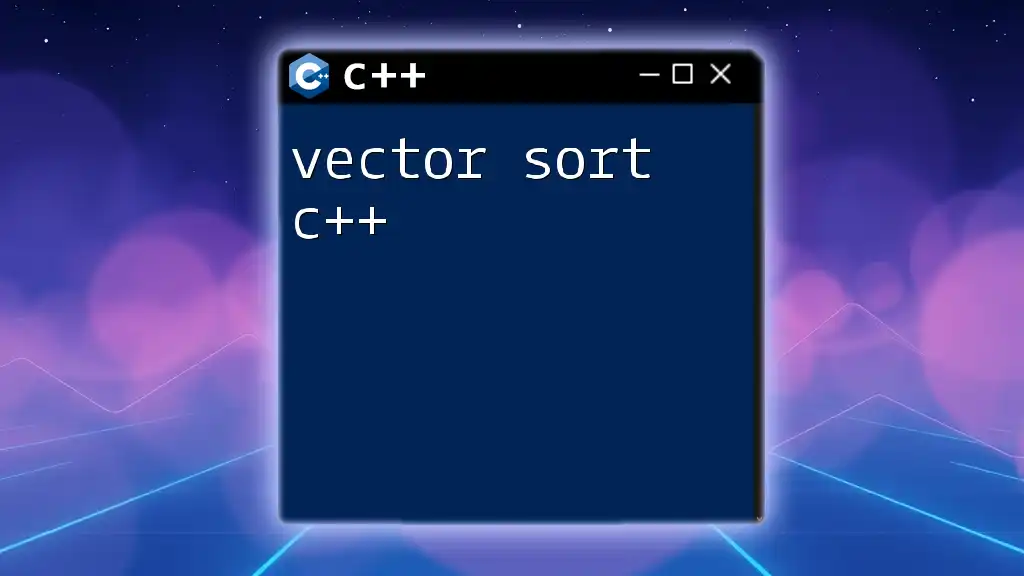
Additional Resources
For more detailed information, refer to the official documentation, explore recommended C++ programming books, and practice coding challenges on platforms such as CodeChef and LeetCode.