The `fout` command in C++ is typically used to output data to a file using an instance of `ofstream`, which allows you to write text or binary data to files in a clear and efficient manner.
Here's a simple code snippet demonstrating how to use `fout` to write to a file:
#include <iostream>
#include <fstream>
int main() {
std::ofstream fout("output.txt");
if (fout.is_open()) {
fout << "Hello, World!" << std::endl;
fout.close();
} else {
std::cerr << "Unable to open file" << std::endl;
}
return 0;
}
What is C++ Output Stream?
In C++, an output stream is a sequence of characters that are sent to a destination, usually the console or a file. This mechanism allows programmers to communicate with users and capture the results of their operations in a structured format. Output streams are a fundamental aspect of C++ programming, enabling effective data presentation and logging.
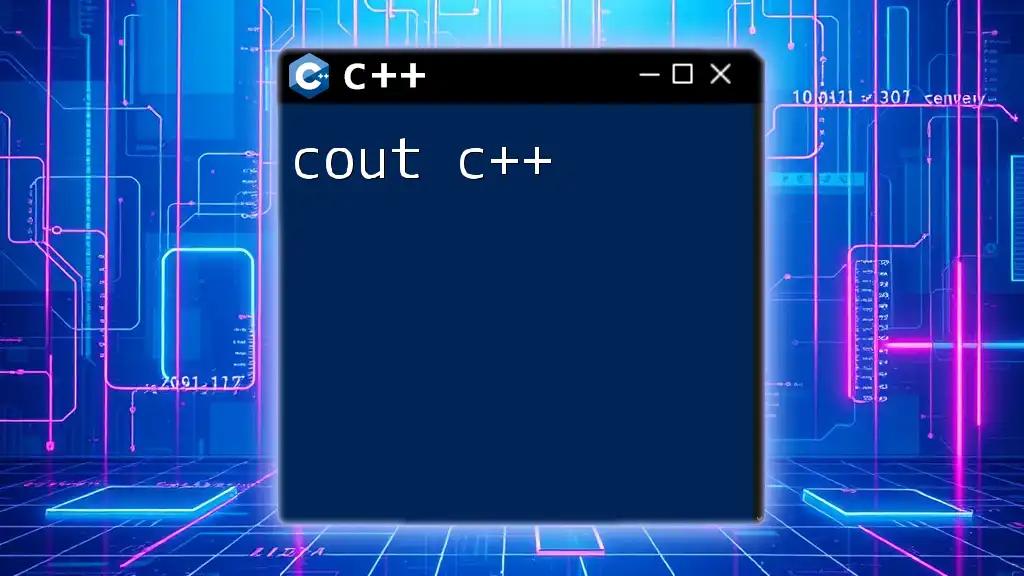
The Concept of `fout`
The term `fout` is commonly used as shorthand for output file streams in C++. It represents a convenient way to direct output to files instead of the console. Handling files allows developers to store data persistently, share output easily, and manage large volumes of information without overwhelming the output interface.
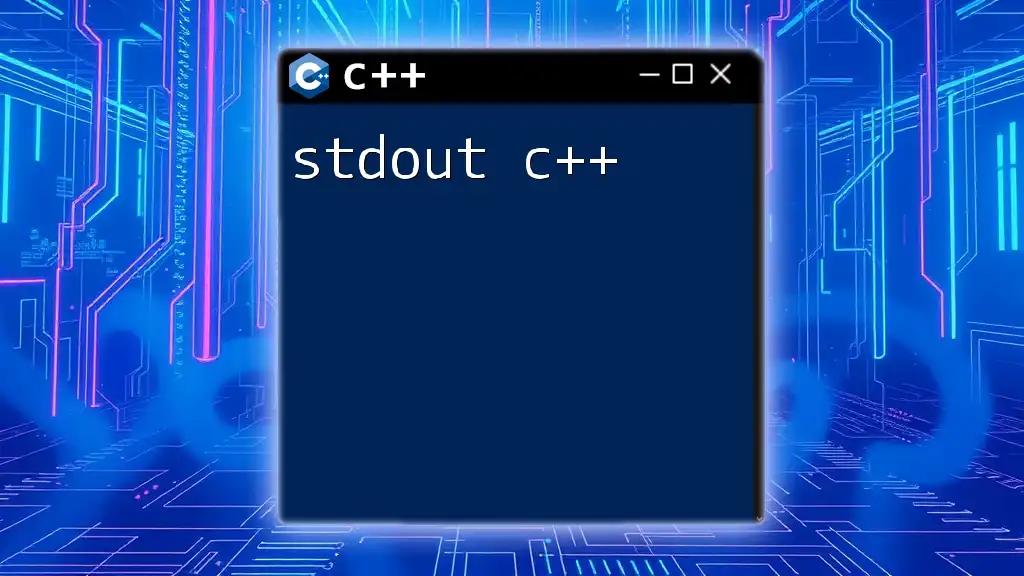
Setting Up `fout` in C++
Including Necessary Headers
To utilize file operations in C++, you need to include specific headers. The most crucial header for file management is `<fstream>`. Here's how to include the relevant headers:
#include <iostream>
#include <fstream> // for file handling
Creating an Output File Stream
Once the required headers are included, you can create an instance of `fout`. This is typically done using the `std::ofstream` class, which represents an output file stream.
std::ofstream fout("output.txt"); // Create/output to a file
In this example, `output.txt` is the name of the file where data will be written. If the file does not exist, C++ will create it for you.
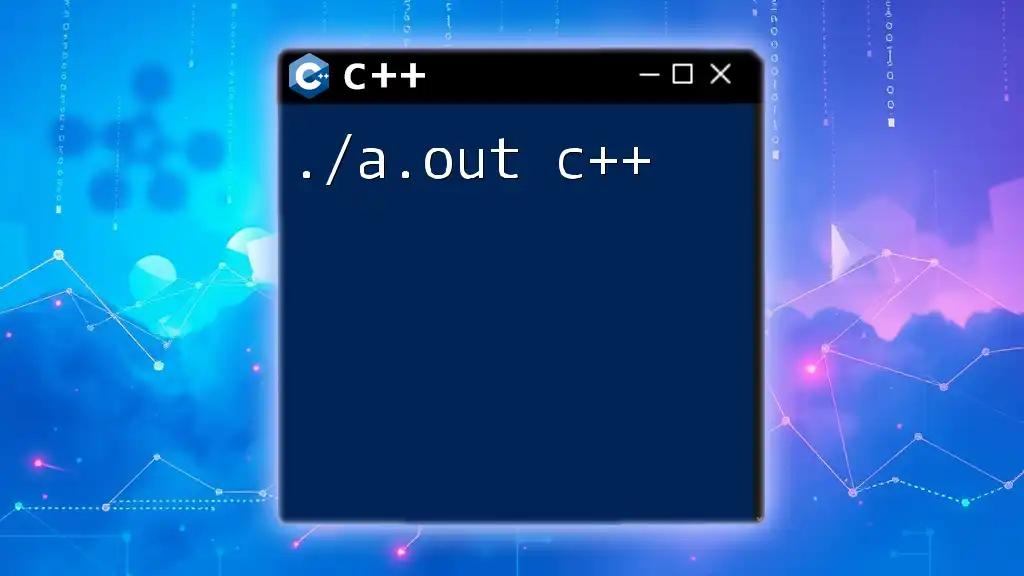
Writing Data to Files Using `fout`
Basic Writing Techniques
You can use the `<<` operator to write data into files with `fout`, just as you would output to the console. This is the basic technique employed for writing strings, numeric values, and other data types.
fout << "Hello, World!" << std::endl; // Write string data
Writing Different Data Types
`fout` is versatile and can handle various data types. You can write integers, floats, characters, and even complex objects as long as the objects can stream their content.
int num = 42;
fout << "The answer is: " << num << std::endl; // Integer
When writing data, make sure to close each output with `std::endl` or a newline character (`'\n'`) to format your output correctly.
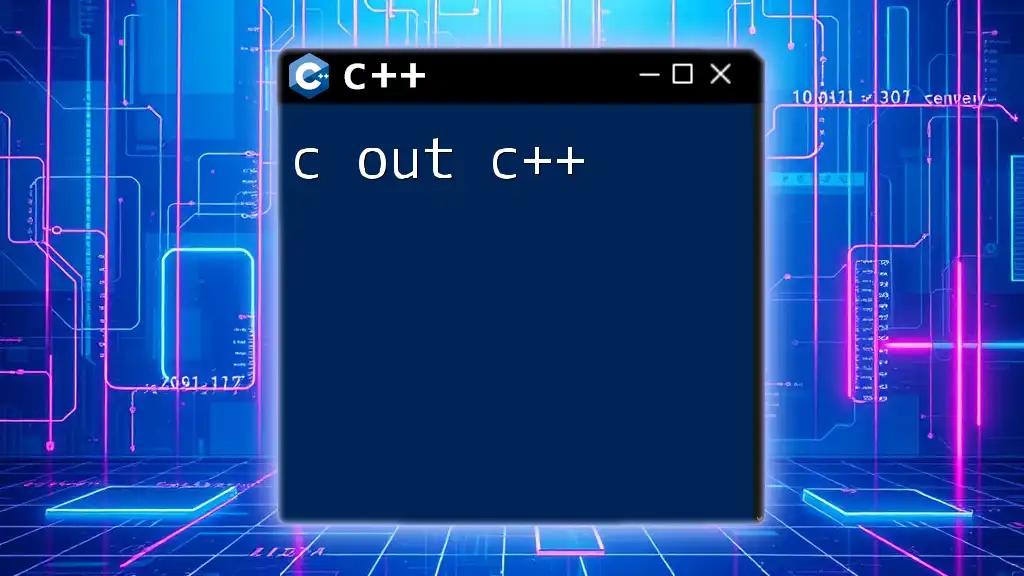
Closing the Output Stream
Importance of Closing Files
It's critical to close any file streams you open. Closing streams properly ensures that all data is flushed to the file and resources are freed. Not doing so may result in data loss or file corruption.
Closing the `fout`
To close your output stream, simply call the `close()` method on your `fout` object:
fout.close(); // Properly close the output file
This line should come after you have finished writing data to your file.

Error Handling in File Output Operations
Checking if File Opened Successfully
Before writing data with `fout`, always check that the output file has been opened correctly. This is an essential step in error handling and ensures that your program can handle potential file I/O issues gracefully.
if (!fout) {
std::cerr << "Error opening file!" << std::endl;
}
Handling Write Errors
While writing to a file, various issues may arise, such as running out of disk space or attempting to write to a read-only file. Implementing proper error handling practices, such as using exception handling or checking file states, can mitigate these problems.

Example Application of `fout`
Creating a Simple Log File
One practical example of using `fout` in C++ is to create a logging application. Here’s how to build a simple program that logs user input into a file:
#include <iostream>
#include <fstream>
int main() {
std::ofstream fout("log.txt");
if (!fout) {
std::cerr << "Error opening file!" << std::endl;
return 1; // Exit if the opening fails
}
for (int i = 0; i < 5; i++) {
fout << "Log entry " << i + 1 << std::endl;
}
fout.close();
return 0;
}
This example opens a file named `log.txt`, checks for successful opening, and writes five log entries into the file.

Advanced Techniques Using `fout`
Using `fout` with File Buffers
In C++, file streams are buffered. This means that data is collected in memory before being written to disk. By managing buffers, you can optimize file I/O operations. For example, you can use the `flush()` method to manually flush the buffer to the file whenever necessary:
fout.flush(); // Forces any buffered output to be written to the file
Different File Modes
When opening a file with `fout`, you can specify several modes that dictate how the file should be used. For example, you might want to append to an existing file instead of overwriting it. This can be done using file mode flags.
std::ofstream fout("output.txt", std::ios::app); // Open file in append mode
By using `std::ios::app`, all new data will be appended to the end of the file, preserving existing content.

Common Pitfalls with `fout`
Forgetting to Close Streams
One of the most common mistakes in file handling is forgetting to close streams after use. Failing to do so can lead to data loss, corrupted files, or memory leaks. Always ensure that your file streams are properly closed, either manually or by using RAII principles.
Mismanagement of File Paths
When dealing with files, it's crucial to manage file paths properly. Using relative paths can often lead to errors if the working directory changes. It's advisable to use absolute paths or ensure that your relative paths are correct.
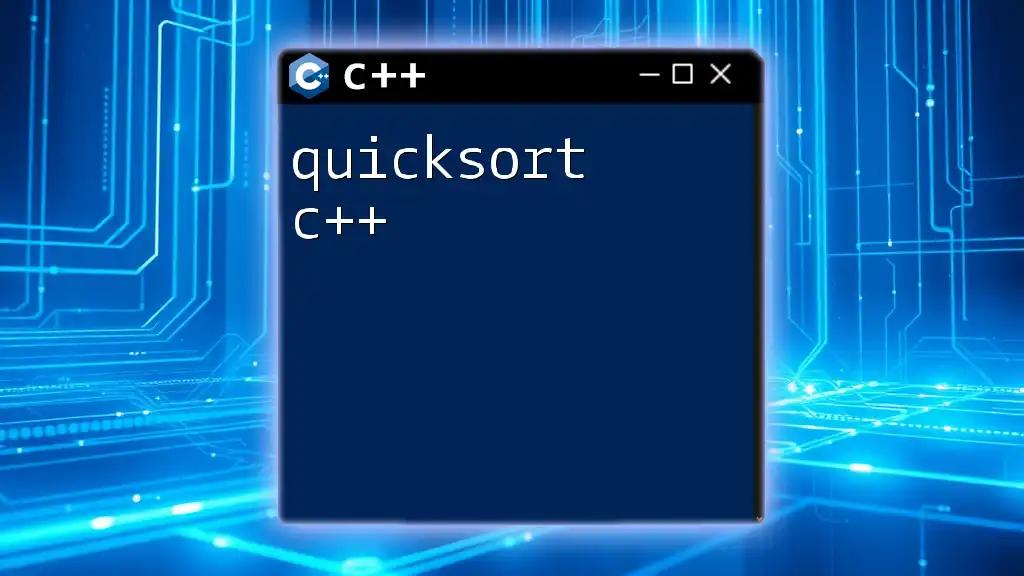
Conclusion
Understanding how to effectively use `fout c++` allows developers to manage file output efficiently and enhance their applications' interactivity. By following best practices, handling errors, and exploring advanced techniques, programmers can ensure robust file I/O operations. Embrace the power of `fout` and improve your C++ programming skills by creating applications where data storage and logging are essential components.

Additional Resources
Explore official documentation, tutorials, and reference books for further learning about C++ file handling, enhancing your understanding of how to utilize `fout` effectively. This knowledge will elevate your programming capabilities and open new avenues for project development.
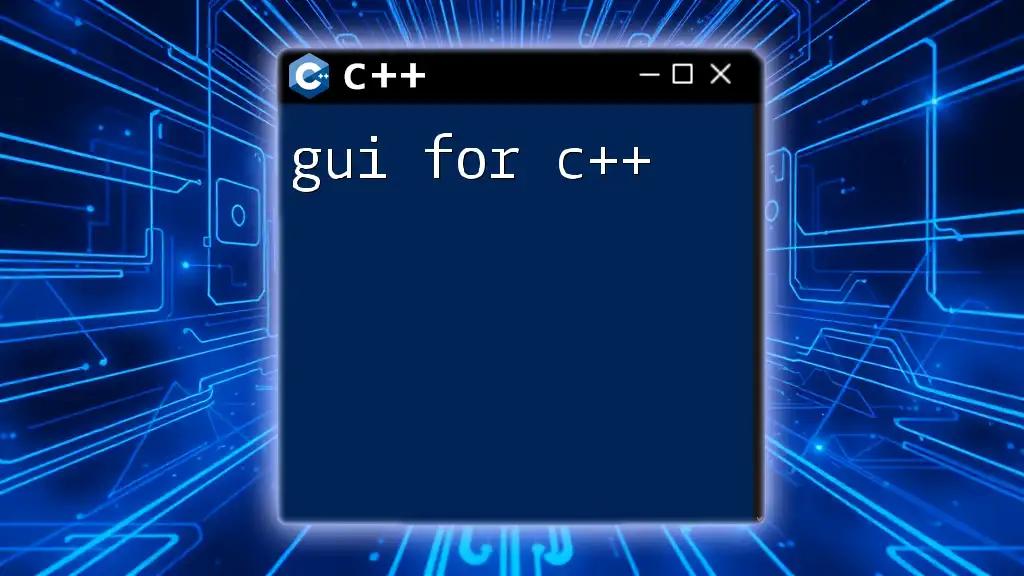
FAQs
Feel free to consider some frequently asked questions to enrich your understanding and clear any doubts about using `fout` in C++. Common queries may include file opening failures, data formatting issues, or performance-related concerns during file output operations.