SDL (Simple DirectMedia Layer) is a powerful multimedia library in C++ that provides an easy way to handle graphics, audio, and input, making it ideal for game development and multimedia applications.
Here's a simple example of initializing an SDL window in C++:
#include <SDL.h>
int main(int argc, char* argv[]) {
SDL_Init(SDL_INIT_VIDEO); // Initialize SDL for video
SDL_Window* window = SDL_CreateWindow("SDL Demo",
SDL_WINDOWPOS_CENTERED, SDL_WINDOWPOS_CENTERED, 640, 480, 0); // Create a window
// Main loop
SDL_Event event;
bool running = true;
while (running) {
while (SDL_PollEvent(&event)) {
if (event.type == SDL_QUIT) running = false; // Exit on quit event
}
}
SDL_DestroyWindow(window); // Clean up the window
SDL_Quit(); // Shut down SDL
return 0;
}
What is SDL?
SDL (Simple DirectMedia Layer) is a powerful, cross-platform development library widely utilized for creating multimedia applications, including games and simulations. Originally designed to provide an abstraction layer for low-level platforms, SDL blends ease of use with performance, making it a popular choice among C++ developers.
Importance in game development and multimedia applications: SDL simplifies the complexities associated with handling graphics, audio, and user input, allowing developers to focus on their application’s core functionalities. By supporting multiple platforms, SDL enables seamless game deployment across various operating systems.
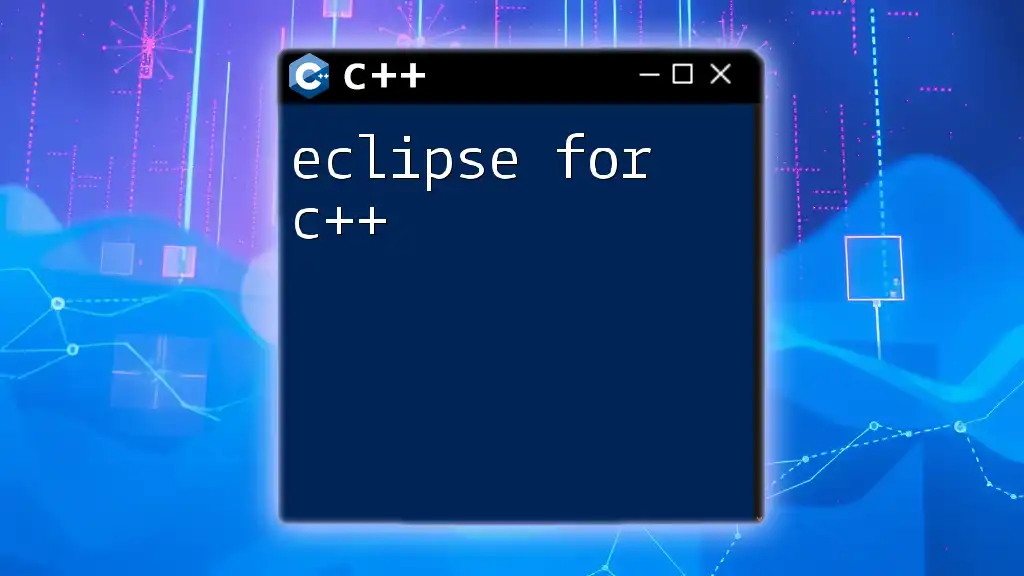
Why Choose SDL for C++?
When considering options for multimedia application development, SDL stands out primarily for its cross-platform capabilities. Developers can write their code once and compile it for Windows, macOS, Linux, and even mobile platforms, significantly reducing development time and effort.
Furthermore, SDL provides a simplified interface for handling graphics rendering, audio playback, and user input. Its comprehensive community support and extensive documentation also prove invaluable, especially for those new to C++ or game development.
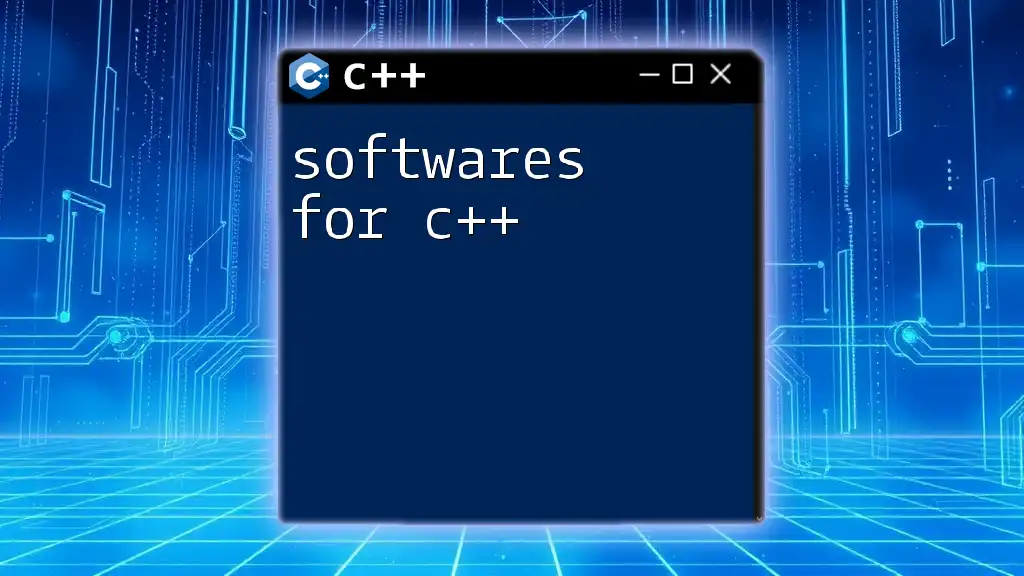
Setting Up SDL in Your C++ Environment
Installing SDL
The installation process for SDL varies by operating system but generally involves the following steps:
- Windows: You can use package managers like `vcpkg`. Open your terminal and run:
vcpkg install sdl2
- macOS: Homebrew easily gets SDL running. Execute:
brew install sdl2
- Linux: Use your package manager to install SDL. For example, on Ubuntu, you can run:
sudo apt-get install libsdl2-dev
After installation, verify that your development environment recognizes SDL by including the library in a new C++ project.
Creating Your First SDL Project
To start a basic project using SDL, consider using CMake for project management:
- Create a directory for your project.
- Write a `CMakeLists.txt` file to specify the SDL dependencies.
- Use the following example:
cmake_minimum_required(VERSION 3.10)
project(SDLExample)
find_package(SDL2 REQUIRED)
add_executable(SDLExample main.cpp)
target_link_libraries(SDLExample SDL2::SDL2)
Now you’re ready to create your `main.cpp` file and get started with SDL!
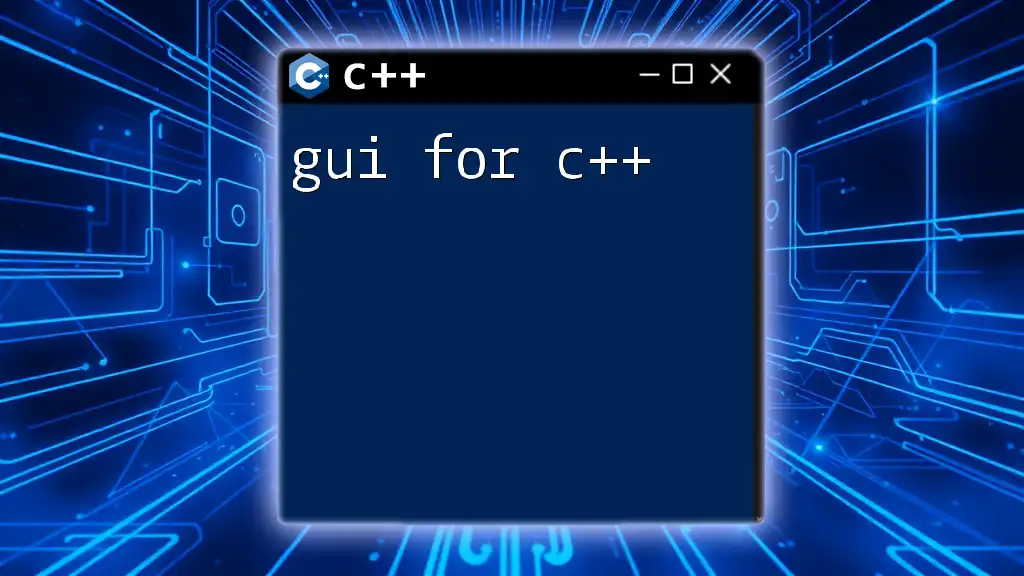
Understanding the SDL Basics
SDL Initialization
Before using SDL, it's vital to initialize it to ensure all its subsystems are set up correctly. This initialization is key for avoiding runtime errors. Use the following code snippet to initialize SDL:
if (SDL_Init(SDL_INIT_VIDEO) < 0) {
// Handle error
std::cerr << "SDL could not initialize! SDL_Error: " << SDL_GetError() << std::endl;
}
Creating an SDL Window
Creating an SDL window is straightforward. Here’s how you can create a window (make sure to include the necessary headers at the top of your code):
SDL_Window* window = SDL_CreateWindow("My SDL Window", SDL_WINDOWPOS_UNDEFINED, SDL_WINDOWPOS_UNDEFINED, 800, 600, SDL_WINDOW_SHOWN);
if (window == nullptr) {
// Handle error
std::cerr << "Window could not be created! SDL_Error: " << SDL_GetError() << std::endl;
}
Handling Renderer
To render graphics, you’ll need to create an SDL_Renderer. This is essential for drawing shapes or textures onto the window. Here’s how you create a renderer:
SDL_Renderer* renderer = SDL_CreateRenderer(window, -1, SDL_RENDERER_ACCELERATED);
if (renderer == nullptr) {
// Handle error
std::cerr << "Renderer could not be created! SDL Error: " << SDL_GetError() << std::endl;
}
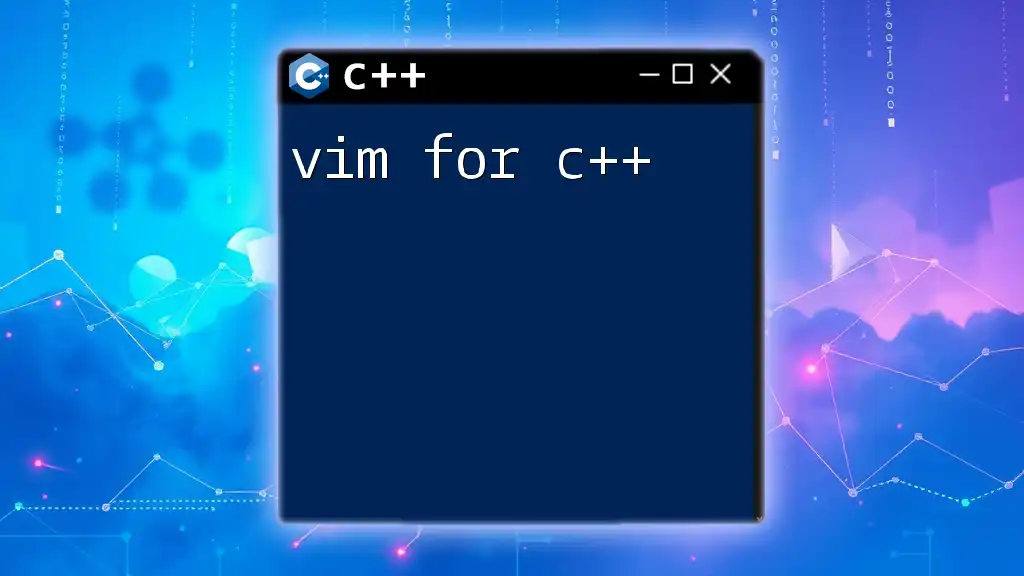
Drawing with SDL
Rendering Shapes and Textures
SDL provides several methods to draw shapes and render textures. For example, if you want to render a simple rectangle, use the following code:
SDL_Rect rect = {10, 10, 100, 100}; // x, y, width, height
SDL_SetRenderDrawColor(renderer, 255, 0, 0, 255); // Set color to red
SDL_RenderFillRect(renderer, &rect);
Loading and Displaying Images
To handle images efficiently, SDL offers the SDL_image extension. Before using it, be sure to link the library. Load an image and display it using the following code:
SDL_Surface* surface = IMG_Load("image.png");
if (surface == nullptr) {
std::cerr << "Unable to load image! SDL_image Error: " << IMG_GetError() << std::endl;
}
SDL_Texture* texture = SDL_CreateTextureFromSurface(renderer, surface);
SDL_FreeSurface(surface); // Free the surface after creating the texture
// Now render the texture (assuming dimensions match the window size)
SDL_RenderCopy(renderer, texture, NULL, NULL);
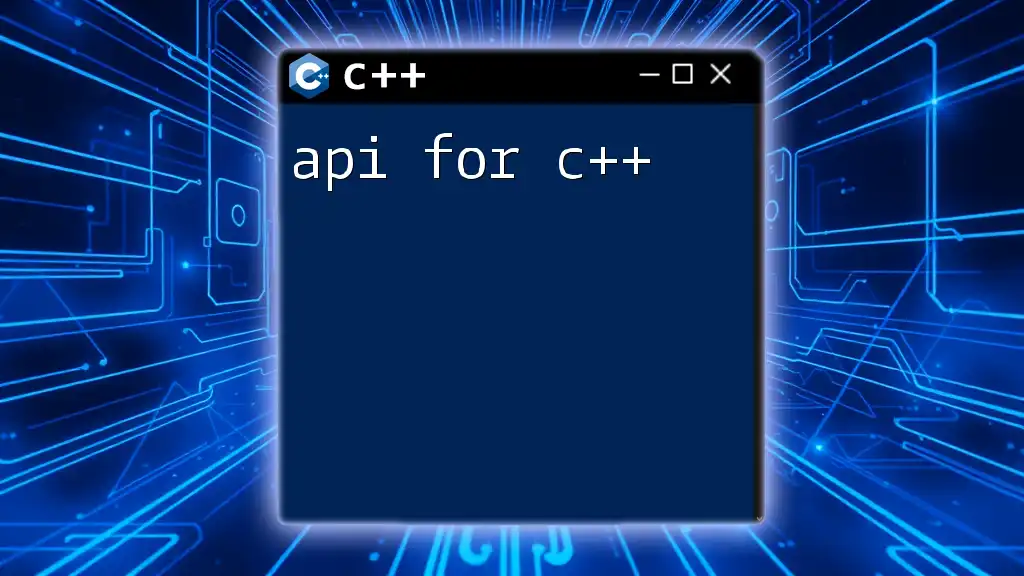
Handling Input in SDL
Keyboard Input Handling
Capturing keyboard input is crucial for interactivity in your applications. SDL_Event provides an efficient way to manage input events. An example code snippet is shown below:
SDL_Event event;
while (SDL_PollEvent(&event)) {
if (event.type == SDL_QUIT) {
// Handle close event
}
if (event.type == SDL_KEYDOWN) {
// Handle key press events
}
}
Mouse Input Handling
In addition to the keyboard, you can track mouse movement and clicks. Use the following example to capture mouse position:
int x, y;
SDL_GetMouseState(&x, &y);
// Use x and y for your logic
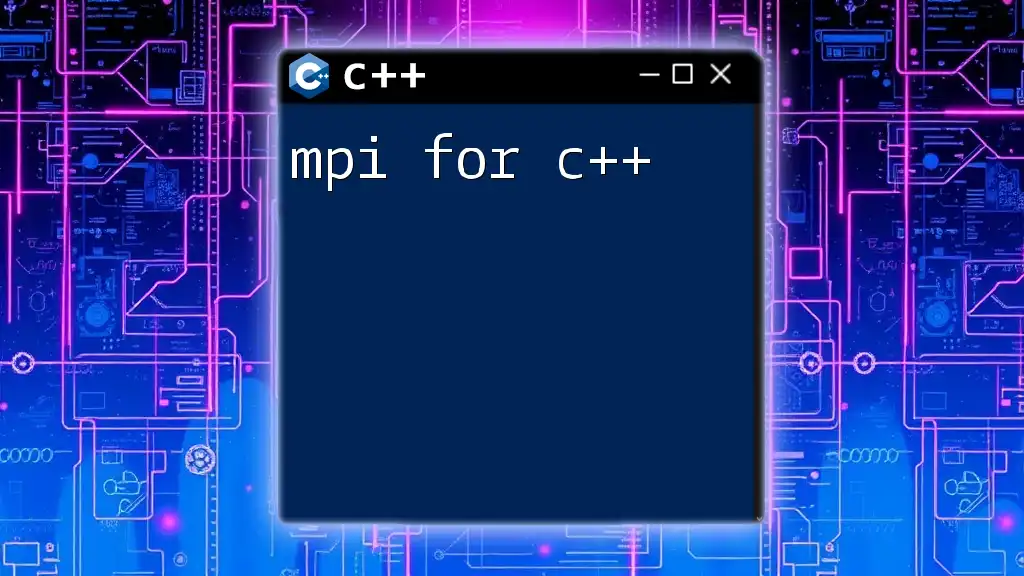
Sound and Music with SDL
Using SDL_mixer for Audio
To manage audio in your applications, SDL_mixer is the go-to extension. Here's how to play a sound effect:
Mix_Chunk *sound = Mix_LoadWAV("sound.wav");
if (sound == nullptr) {
std::cerr << "Failed to load sound effect! SDL_mixer Error: " << Mix_GetError() << std::endl;
}
Mix_PlayChannel(-1, sound, 0);
Playing Music with SDL_mixer
For background music, load and play it using the following code:
Mix_Music *music = Mix_LoadMUS("music.mp3");
if (music == nullptr) {
std::cerr << "Failed to load music! SDL_mixer Error: " << Mix_GetError() << std::endl;
}
Mix_PlayMusic(music, -1); // -1 means loop indefinitely
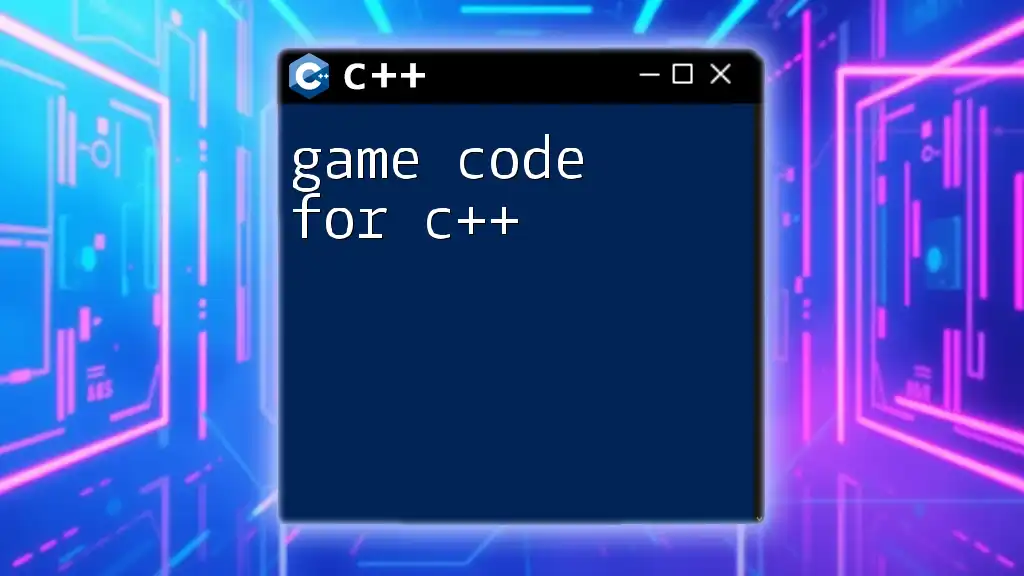
SDL for Game Development
Creating a Simple Game Loop
A game loop is the backbone of any game, conceiving the update, render, and event logic phases. A simple loop structure looks like this:
bool running = true;
while (running) {
SDL_Event event;
while (SDL_PollEvent(&event)) {
if (event.type == SDL_QUIT) {
running = false;
}
}
// Update game logic…
// Render updates…
SDL_RenderPresent(renderer);
}
Managing Game States
Your game may include various states such as menus, playing, or paused. An effective way to organize this would be to use an enumeration or a state machine. Here’s a simple structure using an enum:
enum GameState { MENU, PLAYING, PAUSED };
GameState currentState = MENU;
// Switch between states based on user input...
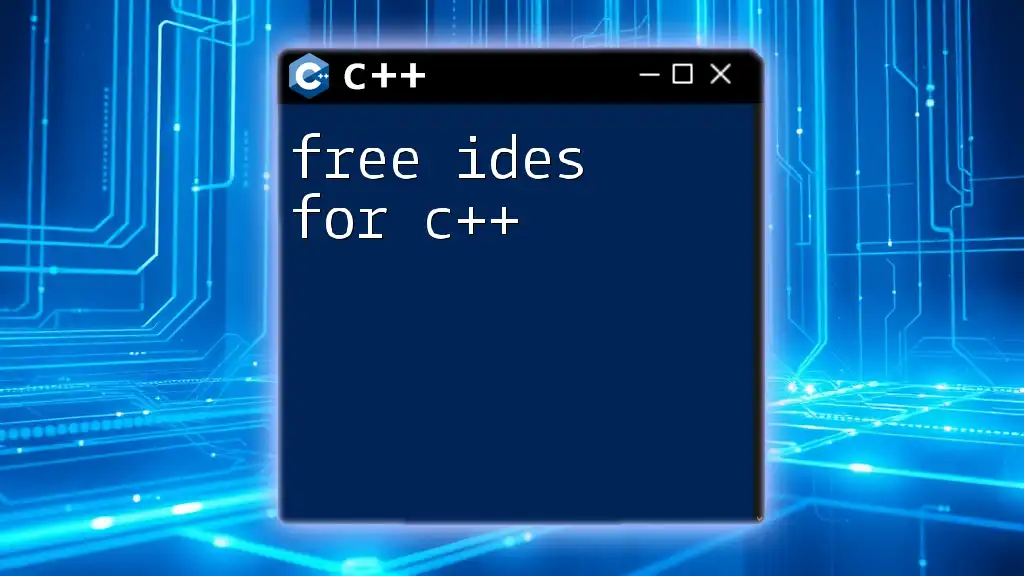
Debugging and Optimization Tips for SDL
Common SDL Issues and Solutions
As with any library, errors can occur. Common SDL issues include:
- Missing library files.
- Incorrect initialization.
- Texture rendering errors (ensure the texture is created after the renderer).
Always check SDL functions for errors to handle issues promptly.
Best Practices for SDL Game Development
Following best practices can enhance your development experience:
- Code Organization: Structure your code logically. Separate concerns—have dedicated classes or modules for handling graphics, input, and sound.
- Asset Management: Load assets only when necessary and free them afterward to manage memory efficiently.
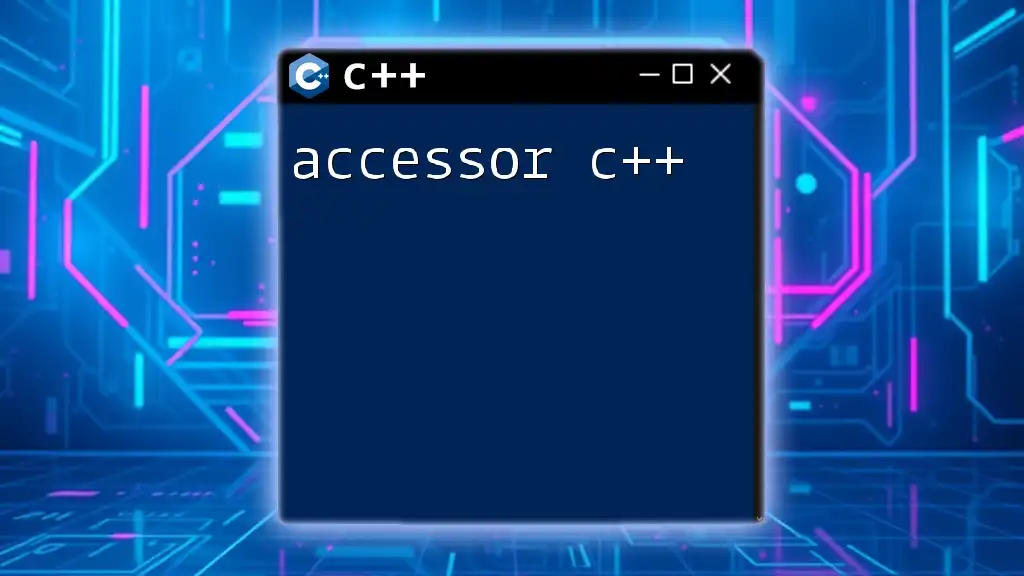
Conclusion
SDL serves as a remarkable tool for developing multimedia applications, particularly in C++. The functionality it provides simplifies numerous aspects of application development, making it a strong choice for both beginners and seasoned developers alike.
Further Learning Resources
To further enhance your SDL skills:
- Explore the [official SDL documentation](https://wiki.libsdl.org/FrontPage) for extensive resources.
- Consider investing in recommended C++ game development books and online courses to dive deeper into SDL.
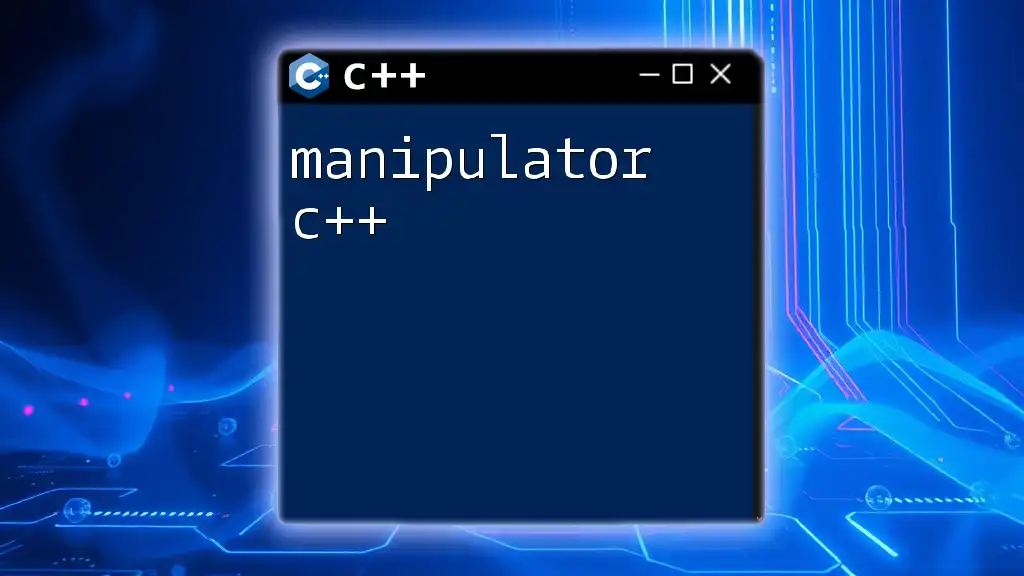
Call to Action
With this guide, you're well on your way to building your own applications using SDL for C++. Get started on your journey, experiment with your own projects, and explore the possibilities SDL has to offer!